
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
fingerprint_frame.cpp
Go to the documentation of this file.
00001 /** 00002 * @file fingerprint_frame.cpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * @version 0.1 00006 * @date 2020-09-28 00007 * 00008 * @copyright Copyright (c) 2020 00009 * 00010 */ 00011 00012 #include "mbed.h" 00013 #include "fingerprint_frame.hpp " 00014 00015 /** 00016 * @brief 00017 * 00018 */ 00019 00020 // CONSTANTES DE PROTOCOLO DE COMUNICACIÓN 00021 const char SERVER_SEPARATOR[] = ";"; 00022 const char FINGER_CMD_BEGIN[] = "@"; 00023 const char FINGER_CMD_EOF[] = "#"; 00024 00025 // COMANDOS DE HUELLA 00026 const char FINGERPRINT_DIS_CMD[] = "FPF"; // DESHABILITAR HUELLA 00027 const char FINGERPRINT_ENA_CMD[] = "FPN"; // HABILITAR HUELLA 00028 const char ADD_CMD[] = "ADD"; // AGREGAR HUELLA 00029 const char FINGERPRINT_QRY_CMD[] = "ASK"; // INTERROGAR HUELLA 00030 const char Q_CHECK_ENROLLED_ID[] = "CEI"; // query Check Enrolled fingerprint ID 00031 const char DEL_CMD[] = "DEL"; // ELIMINAR HUELLA 00032 const char Q_FINGERPRINT_STATUS[] = "QFS"; // Query Fingerprint Status - 1 Enable, 0 Disable 00033 const char FP_OVERRIDE_ENABLE[] = "FON"; // Fingerprint Override oN 00034 const char FP_OVERRIDE_DISABLE[] = "FOF"; // Fingerprint Override oFF 00035 const char FP_OVERRIDE_COUNT[] = "FOC"; // Fingerprint unauthorized Override Count 00036 const char Q_FP_OVERRIDE_CNT[] = "QFO"; // Query Fingerprint Override 00037 00038 // COMANDOS DE PARLANTE 00039 const char VOL_CMD[] = "VOL"; // SET JQ8400 VOL 00040 const char Q_VOLUME_LEVEL[] = "QVL"; // Query Volume level 00041 00042 // COMANDOS PARA SENSOR DE LLUVIA 00043 const char RAIN_ENA_CMD[] = "RNN"; // RaiN oN 00044 const char RAIN_DIS_CMD[] = "RNF"; // RaiN oFf 00045 const char Q_RAIN_SENSOR_STATUS[] = "QRS"; // Query Rain Sensor Status - 1 Enable, 0 Disable 00046 const char CHANGE_RAIN_TIMER[] = "RST"; // change Rain Sensor Timer 00047 const char Q_RAIN_TIMER[] = "QRT"; // Query Rain Timer 00048 const char CHANGE_WSL_TXT[] = "WSL"; // Wet Speed Limit 00049 const char CHANGE_WSW_TXT[] = "WSW"; // Wet Speed Warning 00050 const char CHANGE_DSL_TXT[] = "DSL"; // Dry Speed Limit 00051 const char CHANGE_DSW_TXT[] = "DSW"; // Dry Speed Warning 00052 const char QUERY_SPEED_LIMIT[] = "QSL"; // Query Speed Limit 00053 const char RAIN_SILENT_ENABLE[] = "RSN"; //* Rain sensor Silent mode oN 00054 const char RAIN_SILENT_DISABLE[] = "RSF"; //* Rain sensor Silent mode ofF 00055 const char RAIN_SILENT_QUERY[] = "RSQ"; //* Rain sensor Silent mode Query 00056 00057 // COMANDOS PARA CINTURONES DE SEGURIDAD 00058 const char SEATBELT_ENA_CMD[] = "SBN"; // SeatBelt reading oN 00059 const char SEATBELT_DIS_CMD[] = "SBF"; // SeatBelt reading oFF 00060 const char SEATBELT_QUERY_CMD[] = "SBQ"; // SeatBelt reading Query 00061 const char FORCE_BUCLKE_ENA_CMD[] = "FBN"; // Force Buclke oN 00062 const char FORCE_BUCLKE_DIS_CMD[] = "FBF"; // Force Buclke oFF 00063 const char Q_FORCE_SEATBELT_STATUS[] = "FSS"; // query Force Seatbelt Status 00064 const char INPUT_NORMALLY_OPEN[] = "INO"; // Inputs Normally Open 00065 const char INPUT_NORMALLY_CLOSE[] = "INC"; // Inputs Normally Close 00066 const char Q_DIGITAL_INPUT_CONF[] = "QDI"; // Query Digital Input Configuration 00067 // Definición de cinturones Individual 00068 const char PILOT_BUCKLE_N_OPEN[4] = "PBO"; // Pilot Buckle normally Open 00069 const char PILOT_BUCKLE_N_CLOSE[4] = "PBC"; // Pilot Buckle normally Close 00070 const char Q_PILOT_BUCLE_TYPE[4] = "QPB"; // Query Pilot Buckle type 00071 const char COPILOT_BUCKLE_N_OPEN[4] = "CBO"; // Copilot Buckle normally Open 00072 const char COPILOT_BUCKLE_N_CLOSE[4] = "CBC"; // Copilot Buckle normally Close 00073 const char Q_COPILOT_BUCLE_TYPE[4] = "QCB"; // Query Copilot Buckle type 00074 const char CREW_BUCKLE_N_OPEN[4] = "TBO"; // Crew Buckle normally Open 00075 const char CREW_BUCKLE_N_CLOSE[4] = "TBC"; // Crew Buckle normally Close 00076 const char Q_CREW_BUCLE_TYPE[4] = "QTB"; // Query Crew Buckle type 00077 00078 // COMANDOS DE RALENTI 00079 const char IDLE_SHUTDOWN_ENA_CMD[] = "ISN"; // Idle Shutdown oN 00080 const char IDLE_SHUTDOWN_DIS_CMD[] = "ISF"; // Idle Shutdown oFF 00081 const char IDLE_SHUTDOWN_QUERY[] = "ISQ"; // Idle Shutdown Query 00082 const char Q_IDLE_SHUTDOWN_STATUS[] = "ISS"; // query Idle Shutdown Status 00083 const char CHANGE_IDLE_TIMER[] = "IST"; // Idle Shutdown Timer 00084 const char QUERY_IDLE_TIMER[] = "QIT"; // Query Idle shutdown Timer 00085 const char IDLE_REMINDER_ENABLE[] = "IRN"; // Idle Reminder oN 00086 const char IDLE_REMINDER_DISABLE[] = "IRF"; // Idle Remider oFF 00087 const char QUERY_IDLE_REMINDER[] = "QIR"; // Query Idle Reminder 00088 const char IDLE_REMINDER_TIMER[] = "IRT"; // Idle Reminer Timer 00089 const char QUERY_IDLE_REMINDER_T[] = "QRT"; // Query idle Reminder Timer 00090 00091 // COMANDOS DE TIP DE SEGURIDAD 00092 const char QUERY_SAFETY_TIP[] = "QST"; // Query Safety Tip Time 00093 const char CHANGE_SAFETY_TIME[] = "STT"; // Safety Tip Time 00094 const char SAFETY_TIP_ENABLE[] = "STN"; // Safety Tip oN 00095 const char SAFETY_TIP_DISABLE[] = "STF"; // Safety Tip oFf 00096 00097 // COMANDOS DE VERSION DE ICS5 00098 const char Q_HARDWARE_VERSION[] = "QHW"; // Query Hardware Version 00099 const char Q_FIRMWARE_VERSION[] = "QFW"; // Query Firmware Version 00100 00101 // COMANDOS DE NOTIFICACION DE GEOCERCA 00102 const char GEO_WARNING_ENABLE[] = "GWN"; //* Geozone Warning oN 00103 const char GEO_WARNING_DISABLE[] = "GWF"; //* Geozone Warning ofF 00104 const char Q_GEO_WARNING_CONF[] = "QGW"; //* Query Geozone Warning Status 00105 const char GEO_WARNING_GETIN[] = "GNI"; //* Geozone Notification Inside 00106 const char GEO_WARNING_GETOUT[] = "GNO"; //* Geozone Notification Outside 00107 const char GEO_WARNING_EVENT[] = "GNE"; //* Geozone Notification Event 00108 00109 // COMANDOS DE AUTORESET BLUETOOTH 00110 const char BLE_AUTORESET_ENABLE[4] = "BAN"; // Bluetooth Autoreset oN 00111 const char BLE_AUTORESET_DISABLE[4] = "BAF"; // Bluetooth Autoreset ofF 00112 const char BLE_AUTORESET_TIME[4] = "BAT"; // Bluetooth Autoreset change Time 00113 const char BLE_AUTORESET_QUERY[4] = "BAQ"; // Bluetooth Autoreset Query 00114 00115 // CONSTANTES DE CAMPOS DE TRAMA 00116 const int FINGER_CMD_START = 1; 00117 const int FINGER_CMD_END = 3; 00118 const int FINGER_ID_BEGIN = 5; 00119 const int FINGER_ID_END = 8; 00120 const int FINGER_PAYLOAD_EOL = 9; 00121 const int FINGER_PAYLOAD_START = 10; 00122 const int FINGER_PAYLOAD_END = 1006; 00123 00124 /** 00125 * @brief 00126 * 00127 * @param cadena 00128 * @return int 00129 */ 00130 int strID_to_intID (char cadena[5]) { 00131 /* 00132 Esta función recibe una cadena de 5 posiciones 00133 multiplica cada posición de la cadena por su peso 00134 decimal y lo acumula en una variable de tipo long. 00135 La posición 5 no se opera por tratarse del caracter 00136 de fin de cadena \0. 00137 */ 00138 int result = 0; 00139 int temp = 0; 00140 00141 temp = (int) cadena[0]; 00142 temp = temp - 48.0; 00143 temp = temp * 1000; 00144 result = result + temp; 00145 00146 temp = (int) cadena[1]; 00147 temp = temp - 48.0; 00148 temp = temp * 100; 00149 result = result + temp; 00150 00151 temp = (int) cadena[2]; 00152 temp = temp - 48.0; 00153 temp = temp * 10; 00154 result = result + temp; 00155 00156 temp = (int) cadena[3]; 00157 temp = temp - 48.0; 00158 result = result + temp; 00159 00160 return(result); 00161 } 00162 00163 /** 00164 * @brief 00165 * 00166 * @param payload 00167 * @return true 00168 * @return false 00169 */ 00170 bool verify_finger_payload (char payload[1011]) { 00171 bool inicio_encontrado = false; // Tipo Bool 00172 bool fin_encontrado = false; // Tipo Bool 00173 char *ret; 00174 00175 // Verificar si se encuentra el inicio del comando @ 00176 ret = strchr(payload, FINGER_CMD_BEGIN[0]); 00177 if (ret!=NULL) { 00178 inicio_encontrado = true; 00179 } 00180 00181 // Verificar si se encuentra el fin de comando # 00182 ret = strchr(payload, FINGER_CMD_EOF[0]); 00183 if (ret!=NULL) { 00184 fin_encontrado = 1; 00185 } 00186 00187 if (inicio_encontrado && fin_encontrado) { // Trama para procesar 00188 return(true); 00189 } else { // Trama para ignorar 00190 return(false); 00191 } 00192 } 00193 00194 /** 00195 * @brief 00196 * 00197 * @param payload 00198 * @param comando 00199 * @param id_huella 00200 * @param huella_hex 00201 * @return true 00202 * @return false 00203 */ 00204 bool parse_finger_payload (char payload[1011], char comando[4], int *id_huella, char huella_hex[997]) { 00205 char id_txt[5]; // Almacenar temporalmente el ID a procesar. 00206 char * pch; 00207 int i = 0; 00208 int j = 0; 00209 memset(id_txt, '\0', sizeof(id_txt)); 00210 memset(huella_hex, '\0', sizeof(huella_hex)); 00211 00212 if (payload[0] == '@') { 00213 00214 pch = strtok (payload, "@;#"); 00215 00216 while (pch != NULL) { 00217 j++; 00218 //debug -> printf("BLE - Puntero-%d = %s\r\n", j, pch); 00219 if (j == 1) { 00220 strcpy(comando, pch); 00221 //debug -> printf("BLE - Comando TXT = %s\r\n", comando); 00222 } else if (j ==2) { 00223 strcpy(id_txt, pch); 00224 //debug -> printf("BLE - ID TXT = %s\r\n", id_txt); 00225 *id_huella = atoi(id_txt); 00226 //debug -> printf("BLE - ID NUM=%d\r\n", *id_huella); 00227 } else if (j == 3) { 00228 strcpy(huella_hex, pch); 00229 //debug -> printf("BLE - Huella ASCII\r\n=%s\r\n\r\n", huella_hex); 00230 } 00231 pch = strtok (NULL, "@;#"); 00232 } 00233 00234 //debug -> printf("BLE - Repeticiones de Lazo = %d\r\n", j); 00235 if (j == 4) { 00236 //debug -> printf("BLE - Trama con Huella TRUE\r\n"); 00237 return true; 00238 } else { 00239 //debug -> printf("BLE - Trama con Huella FALSE\r\n"); 00240 return false; 00241 } 00242 } 00243 return true; 00244 } 00245 00246 00247 /** 00248 * @brief 00249 * 00250 * @param huella_hex 00251 * @param huella_ascii 00252 * @return true 00253 * @return false 00254 */ 00255 bool fingerprint_HEX2CHAR (char huella_hex[997], char huella_ascii[499]) { 00256 /* 00257 Esta función se encarga de analisar el payload de una 00258 huella que es enviada dentro de una cadena (char array) 00259 expresada de manera hexadecimal. en su equivalente de código 00260 ASCII que es guardado en otra cadena. 00261 */ 00262 00263 int i = 0; 00264 int j = 0; 00265 int k = 0; 00266 int limite = strlen(huella_hex); 00267 char pre_buffer[] = "--\0"; 00268 00269 for (i=0; i<limite; i++) { 00270 pre_buffer[j] = huella_hex[i]; 00271 j++; 00272 00273 if ((i%2)&&(i>=1)) { // Si es impar. 00274 j=0; 00275 huella_ascii[k] = (int)strtol(pre_buffer, NULL, 16); 00276 k++; 00277 } 00278 } 00279 return(true); 00280 } 00281 00282 /** 00283 * @brief 00284 * 00285 * @param comando 00286 * @return int 00287 */ 00288 int identify_server_cmd (char comando[4]) { 00289 /* 00290 Esta función se encarga de verificar que el comando a ejecutar es soportado 00291 por el código y que no se ha generado una mala interpretación de la trama 00292 al momento de extraer los caracteres correspondientes al comando. 00293 Esta función retorna 1 si el comando es ADD. Retorna 2 para el comando DEL. 00294 Retorna 0 si no es ninguno de los dos. 00295 */ 00296 char* p; 00297 int comand_int = 0; 00298 00299 00300 /** ********************************** 00301 * COMANDOS DE GEOCERCA 00302 * ********************************** */ 00303 p = strstr(comando, GEO_WARNING_GETIN); 00304 if (p!=NULL) { 00305 comand_int = CMD_GEO_WARNING_GETIN; 00306 return(comand_int); 00307 } 00308 00309 p = strstr(comando, GEO_WARNING_GETOUT); 00310 if (p!=NULL) { 00311 comand_int = CMD_GEO_WARNING_GETOUT; 00312 return(comand_int); 00313 } 00314 00315 p = strstr(comando, GEO_WARNING_EVENT); 00316 if (p!=NULL) { 00317 comand_int = CMD_GEO_WARNING_EVENT; 00318 return(comand_int); 00319 } 00320 00321 p = strstr(comando, GEO_WARNING_ENABLE); 00322 if (p!=NULL) { 00323 comand_int = CMD_GEO_WARNING_ENABLE; 00324 return(comand_int); 00325 } 00326 00327 p = strstr(comando, GEO_WARNING_DISABLE); 00328 if (p!=NULL) { 00329 comand_int = CMD_GEO_WARNING_DISABLE; 00330 return(comand_int); 00331 } 00332 00333 p = strstr(comando, Q_GEO_WARNING_CONF); 00334 if (p!=NULL) { 00335 comand_int = CMD_GEO_WARNING_QUERY; 00336 return(comand_int); 00337 } 00338 00339 00340 00341 /** ********************************** 00342 * COMANDOS DE LECTOR DE HUELLA 00343 * ********************************** */ 00344 p = strstr(comando, ADD_CMD); 00345 if (p!=NULL) { 00346 comand_int = CMD_FINGER_ADD; 00347 return(comand_int); 00348 } 00349 00350 p = strstr(comando, DEL_CMD); 00351 if (p!=NULL) { 00352 comand_int = CMD_FINGER_DELETE; 00353 return(comand_int); 00354 } 00355 00356 p = strstr(comando, FINGERPRINT_DIS_CMD); 00357 if (p!=NULL) { 00358 comand_int = CMD_FINGER_DISABLE; 00359 return(comand_int); 00360 } 00361 00362 p = strstr(comando, FINGERPRINT_ENA_CMD); 00363 if (p!=NULL) { 00364 comand_int = CMD_FINGER_ENABLE; 00365 return(comand_int); 00366 } 00367 00368 p = strstr(comando, Q_FINGERPRINT_STATUS); 00369 if (p!=NULL) { 00370 comand_int = CMD_FINGERPRINT_QUERY; 00371 return(comand_int); 00372 } 00373 00374 p = strstr(comando, FINGERPRINT_QRY_CMD); 00375 if (p!=NULL) { 00376 comand_int = CMD_FINGER_ENROLL_CNT; 00377 return(comand_int); 00378 } 00379 00380 p = strstr(comando, Q_CHECK_ENROLLED_ID); 00381 if (p!=NULL) { 00382 comand_int = CMD_CHECK_ENROLLED_ID; 00383 return(comand_int); 00384 } 00385 00386 p = strstr(comando, FP_OVERRIDE_ENABLE); 00387 if (p!=NULL) { 00388 comand_int = CMD_FP_OVERRIDE_ENABLE; 00389 return(comand_int); 00390 } 00391 00392 p = strstr(comando, FP_OVERRIDE_DISABLE); 00393 if (p!=NULL) { 00394 comand_int = CMD_FP_OVERRIDE_DISABLE; 00395 return(comand_int); 00396 } 00397 00398 p = strstr(comando, FP_OVERRIDE_COUNT); 00399 if (p!=NULL) { 00400 comand_int = CMD_FP_OVERRIDE_COUNT; 00401 return(comand_int); 00402 } 00403 00404 p = strstr(comando, Q_FP_OVERRIDE_CNT); 00405 if (p!=NULL) { 00406 comand_int = CMD_FP_OVERRIDE_QUERY; 00407 return(comand_int); 00408 } 00409 00410 00411 00412 /** ********************************** 00413 * COMANDOS DE PARLANTE 00414 * ********************************** */ 00415 p = strstr(comando, VOL_CMD); 00416 if (p!=NULL) { 00417 comand_int = CMD_SET_VOLUME; 00418 return(comand_int); 00419 } 00420 00421 p = strstr(comando, Q_VOLUME_LEVEL); 00422 if (p!=NULL) { 00423 comand_int = CMD_QUERY_VOLUME_LEVEL; 00424 return(comand_int); 00425 } 00426 00427 00428 00429 /** ********************************** 00430 * COMANDOS DE SENSOR DE LLUVIA 00431 * ********************************** */ 00432 p = strstr(comando, RAIN_ENA_CMD); 00433 if (p!=NULL) { 00434 comand_int = CMD_RAIN_ENABLE; 00435 return(comand_int); 00436 } 00437 00438 p = strstr(comando, RAIN_DIS_CMD); 00439 if (p!=NULL) { 00440 comand_int = CMD_RAIN_DISABLE; 00441 return(comand_int); 00442 } 00443 00444 p = strstr(comando, Q_RAIN_SENSOR_STATUS); 00445 if (p!=NULL) { 00446 comand_int = CMD_RAIN_QUERY; 00447 return(comand_int); 00448 } 00449 00450 p = strstr(comando, CHANGE_RAIN_TIMER); 00451 if (p!=NULL) { 00452 comand_int = CMD_CHANGE_RAIN_TIMER; 00453 return(comand_int); 00454 } 00455 00456 p = strstr(comando, Q_RAIN_TIMER); 00457 if (p!=NULL) { 00458 comand_int = CMD_QUERY_RAIN_TIMER; 00459 return(comand_int); 00460 } 00461 00462 p = strstr(comando, RAIN_SILENT_ENABLE); 00463 if (p!=NULL) { 00464 comand_int = CMD_RAIN_SILENT_MODE_ENA; 00465 return(comand_int); 00466 } 00467 00468 p = strstr(comando, RAIN_SILENT_DISABLE); 00469 if (p!=NULL) { 00470 comand_int = CMD_RAIN_SILENT_MODE_DIS; 00471 return(comand_int); 00472 } 00473 00474 p = strstr(comando, RAIN_SILENT_QUERY); 00475 if (p!=NULL) { 00476 comand_int = CMD_RAIN_SILENT_MODE_Q; 00477 return(comand_int); 00478 } 00479 00480 00481 00482 00483 /** ********************************** 00484 * COMANDOS DE LIMITE DE VELOCIDAD 00485 * ********************************** */ 00486 p = strstr(comando, CHANGE_WSL_TXT); 00487 if (p!=NULL) { 00488 comand_int = CMD_CHANGE_WSL; 00489 return(comand_int); 00490 } 00491 00492 p = strstr(comando, CHANGE_WSW_TXT); 00493 if (p!=NULL) { 00494 comand_int = CMD_CHANGE_WSW; 00495 return(comand_int); 00496 } 00497 00498 p = strstr(comando, CHANGE_DSL_TXT); 00499 if (p!=NULL) { 00500 comand_int = CMD_CHANGE_DSL; 00501 return(comand_int); 00502 } 00503 00504 p = strstr(comando, CHANGE_DSW_TXT); 00505 if (p!=NULL) { 00506 comand_int = CMD_CHANGE_DSW; 00507 return(comand_int); 00508 } 00509 00510 p = strstr(comando, QUERY_SPEED_LIMIT); 00511 if (p!=NULL) { 00512 comand_int = CMD_QUERY_SPEED_LIMIT; 00513 return(comand_int); 00514 } 00515 00516 00517 00518 00519 /** ********************************** 00520 * COMANDOS DE CINTURON DE SEGURIDAD 00521 * ********************************** */ 00522 p = strstr(comando, SEATBELT_ENA_CMD); 00523 if (p!=NULL) { 00524 comand_int = CMD_SEATBELT_ENABLE; 00525 return(comand_int); 00526 } 00527 00528 p = strstr(comando, SEATBELT_DIS_CMD); 00529 if (p!=NULL) { 00530 comand_int = CMD_SEATBELT_DISABLE; 00531 return(comand_int); 00532 } 00533 00534 p = strstr(comando, SEATBELT_QUERY_CMD); 00535 if (p!=NULL) { 00536 comand_int = CMD_SEATBELT_QUERY; 00537 return(comand_int); 00538 } 00539 00540 p = strstr(comando, FORCE_BUCLKE_ENA_CMD); 00541 if (p!=NULL) { 00542 comand_int = CMD_FORCE_BUCLKE_ENABLE; 00543 return(comand_int); 00544 } 00545 00546 p = strstr(comando, FORCE_BUCLKE_DIS_CMD); 00547 if (p!=NULL) { 00548 comand_int = CMD_FORCE_BUCLKE_DISABLE; 00549 return(comand_int); 00550 } 00551 00552 p = strstr(comando, Q_FORCE_SEATBELT_STATUS); 00553 if (p!=NULL) { 00554 comand_int = CMD_QUERY_FORCE_SEATBELT; 00555 return(comand_int); 00556 } 00557 00558 p = strstr(comando, INPUT_NORMALLY_OPEN); 00559 if (p!=NULL) { 00560 comand_int = CMD_SET_INPUT_NORMAL_O; 00561 return(comand_int); 00562 } 00563 00564 p = strstr(comando, INPUT_NORMALLY_CLOSE); 00565 if (p!=NULL) { 00566 comand_int = CMD_SET_INPUT_NORMAL_C; 00567 return(comand_int); 00568 } 00569 00570 p = strstr(comando, Q_DIGITAL_INPUT_CONF); 00571 if (p!=NULL) { 00572 comand_int = CMD_QUERY_DIN_CONFIG; 00573 return(comand_int); 00574 } 00575 00576 p = strstr(comando, PILOT_BUCKLE_N_OPEN); 00577 if (p!=NULL) { 00578 comand_int = CMD_PILOT_BUCKLE_NO; 00579 return(comand_int); 00580 } 00581 00582 p = strstr(comando, PILOT_BUCKLE_N_CLOSE); 00583 if (p!=NULL) { 00584 comand_int = CMD_PILOT_BUCKLE_NC; 00585 return(comand_int); 00586 } 00587 00588 p = strstr(comando, Q_PILOT_BUCLE_TYPE); 00589 if (p!=NULL) { 00590 comand_int = CMD_QUERY_PILOT_BT; 00591 return(comand_int); 00592 } 00593 00594 p = strstr(comando, COPILOT_BUCKLE_N_OPEN); 00595 if (p!=NULL) { 00596 comand_int = CMD_COPILOT_BUCKLE_NO; 00597 return(comand_int); 00598 } 00599 00600 p = strstr(comando, COPILOT_BUCKLE_N_CLOSE); 00601 if (p!=NULL) { 00602 comand_int = CMD_COPILOT_BUCKLE_NC; 00603 return(comand_int); 00604 } 00605 00606 p = strstr(comando, Q_COPILOT_BUCLE_TYPE); 00607 if (p!=NULL) { 00608 comand_int = CMD_QUERY_COPILOT_BT; 00609 return(comand_int); 00610 } 00611 00612 p = strstr(comando, CREW_BUCKLE_N_OPEN); 00613 if (p!=NULL) { 00614 comand_int = CMD_CREW_BUCKLE_NO; 00615 return(comand_int); 00616 } 00617 00618 p = strstr(comando, CREW_BUCKLE_N_CLOSE); 00619 if (p!=NULL) { 00620 comand_int = CMD_CREW_BUCKLE_NC; 00621 return(comand_int); 00622 } 00623 00624 p = strstr(comando, Q_CREW_BUCLE_TYPE); 00625 if (p!=NULL) { 00626 comand_int = CMD_QUERY_CREW_BT; 00627 return(comand_int); 00628 } 00629 00630 00631 00632 00633 /** ********************************** 00634 * COMANDOS DE APAGADO POR RALENTI 00635 * ********************************** */ 00636 p = strstr(comando, IDLE_SHUTDOWN_ENA_CMD); 00637 if (p!=NULL) { 00638 comand_int = CMD_IDLE_SHUTDOWN_ENABLE; 00639 return(comand_int); 00640 } 00641 00642 p = strstr(comando, IDLE_SHUTDOWN_DIS_CMD); 00643 if (p!=NULL) { 00644 comand_int = CMD_IDLE_SHUTDOWN_DISABLE; 00645 return(comand_int); 00646 } 00647 00648 p = strstr(comando, IDLE_SHUTDOWN_QUERY); 00649 if (p!=NULL) { 00650 comand_int = CMD_IDLE_SHUTDOWN_QUERY; 00651 return(comand_int); 00652 } 00653 00654 p = strstr(comando, Q_IDLE_SHUTDOWN_STATUS); 00655 if (p!=NULL) { 00656 comand_int = CMD_IDLE_SHUTDOWN_QUERY; 00657 return(comand_int); 00658 } 00659 00660 p = strstr(comando, CHANGE_IDLE_TIMER); 00661 if (p!=NULL) { 00662 comand_int = CMD_CHANGE_IDLE_SHUTDOWN_T; 00663 return(comand_int); 00664 } 00665 00666 p = strstr(comando, QUERY_IDLE_TIMER); 00667 if (p!=NULL) { 00668 comand_int = CMD_QUERY_IDLE_SHUTDOWN_T; 00669 return(comand_int); 00670 } 00671 00672 00673 00674 00675 /** ********************************** 00676 * COMANDOS DE RECORDATORIO POR RALENTI 00677 * ********************************** */ 00678 p = strstr(comando, IDLE_REMINDER_ENABLE); 00679 if (p!=NULL) { 00680 comand_int = CMD_IDLE_REMINDER_ENABLE; 00681 return(comand_int); 00682 } 00683 00684 p = strstr(comando, IDLE_REMINDER_DISABLE); 00685 if (p!=NULL) { 00686 comand_int = CMD_IDLE_REMINDER_DISABLE; 00687 return(comand_int); 00688 } 00689 00690 p = strstr(comando, QUERY_IDLE_REMINDER); 00691 if (p!=NULL) { 00692 comand_int = CMD_IDLE_REMINDER_QUERY; 00693 return(comand_int); 00694 } 00695 00696 p = strstr(comando, IDLE_REMINDER_TIMER); 00697 if (p!=NULL) { 00698 comand_int = CMD_CHANGE_IDLE_REMINDER_T; 00699 return(comand_int); 00700 } 00701 00702 p = strstr(comando, QUERY_IDLE_REMINDER_T); 00703 if (p!=NULL) { 00704 comand_int = CMD_QUERY_IDLE_REMINDER_T; 00705 return(comand_int); 00706 } 00707 00708 00709 00710 00711 /** ********************************** 00712 * COMANDOS DE TIP DE SEGURIDAD 00713 * ********************************** */ 00714 p = strstr(comando, SAFETY_TIP_ENABLE); 00715 if (p!=NULL) { 00716 comand_int = CMD_SAFETY_TIP_ENABLE; 00717 return(comand_int); 00718 } 00719 00720 p = strstr(comando, SAFETY_TIP_DISABLE); 00721 if (p!=NULL) { 00722 comand_int = CMD_SAFETY_TIP_DISABLE; 00723 return(comand_int); 00724 } 00725 00726 p = strstr(comando, QUERY_SAFETY_TIP); 00727 if (p!=NULL) { 00728 comand_int = CMD_QUERY_SAFETY_TIP_TIME; 00729 return(comand_int); 00730 } 00731 00732 p = strstr(comando, CHANGE_SAFETY_TIME); 00733 if (p!=NULL) { 00734 comand_int = CHANGE_SAFETY_TIP_TIME; 00735 return(comand_int); 00736 } 00737 00738 00739 00740 00741 /** ********************************** 00742 * COMANDOS SOBRE INFO DE ICS5 00743 * ********************************** */ 00744 p = strstr(comando, Q_HARDWARE_VERSION); 00745 if (p!=NULL) { 00746 comand_int = CMD_QUERY_HARDWARE_VER; 00747 return(comand_int); 00748 } 00749 00750 p = strstr(comando, Q_FIRMWARE_VERSION); 00751 if (p!=NULL) { 00752 comand_int = CMD_QUERY_FIRMWARE_VER; 00753 return(comand_int); 00754 } 00755 00756 00757 00758 00759 /** ********************************** 00760 * COMANDOS DE AUTORESET DE BLUETOOTH 00761 * ********************************** */ 00762 p = strstr(comando, BLE_AUTORESET_ENABLE); 00763 if (p!=NULL) { 00764 comand_int = CMD_BLE_AUTORESET_ENABLE; 00765 return(comand_int); 00766 } 00767 00768 p = strstr(comando, BLE_AUTORESET_DISABLE); 00769 if (p!=NULL) { 00770 comand_int = CMD_BLE_AUTORESET_DISABLE; 00771 return(comand_int); 00772 } 00773 00774 p = strstr(comando, BLE_AUTORESET_TIME); 00775 if (p!=NULL) { 00776 comand_int = CMD_BLE_AUTORESET_TIME; 00777 return(comand_int); 00778 } 00779 00780 p = strstr(comando, BLE_AUTORESET_QUERY); 00781 if (p!=NULL) { 00782 comand_int = CMD_BLE_AUTORESET_QUERY; 00783 return(comand_int); 00784 } 00785 00786 return(comand_int); 00787 }
Generated on Thu Jul 28 2022 19:27:02 by
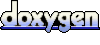