
Proyecto ABInBev para la tarjeta Guaria 1/2.
Embed:
(wiki syntax)
Show/hide line numbers
eeprom_exe.cpp
00001 /** 00002 * @file flash_exe.cpp 00003 * @author Felícito Manzano (felicito.manzano@detektor.com.sv) 00004 * @brief 00005 * @version 0.1 00006 * @date 2021-02-08 00007 * 00008 * @copyright Copyright (c) 2021 00009 * 00010 */ 00011 00012 #include "mbed.h" 00013 #include "eeprom_exe.hpp" 00014 #include "eeprom.h" 00015 #include "USBSerial.h" 00016 #include <ctype.h> 00017 00018 /** * INTERFACES * **/ 00019 extern USBSerial myPC_debug; 00020 extern DigitalOut flashLED; 00021 extern EEPROM ep; 00022 00023 /** * VARIABLES * **/ 00024 // Manejo de EEPROM FLASH 00025 uint32_t eprom_AddressArray[] = { 00026 EE_Address_FINGERPRINT, 00027 EE_Address_OVERRIDE_FP, 00028 EE_Address_OVERRIDE_LIMIT, 00029 EE_Address_IDLE_SHUTDOWN, 00030 EE_Address_IDLE_REMINDER, 00031 EE_Address_IDLE_S_TIME, 00032 EE_Address_IDLE_R_TIME, 00033 EE_Address_SEATBELT_READ, 00034 EE_Address_ENFORCE_SB, 00035 EE_Address_PILOT_BTYPE, 00036 EE_Address_COPILOT_BTYPE, 00037 EE_Address_CREW_BTYPE, 00038 EE_Address_RAINSENSOR, 00039 EE_Address_RS_SILENTMODE, 00040 EE_Address_RS_SAMPLE_T, 00041 EE_Address_WET_LIMIT, 00042 EE_Address_WET_WARNING, 00043 EE_Address_DRY_LIMIT, 00044 EE_Address_DRY_WARNING, 00045 EE_Address_SAFETY_TIP, 00046 EE_Address_SAFETY_TIP_T, 00047 EE_Address_GEOZONE, 00048 EE_Address_VOLUME, 00049 EE_Address_BLE_RESET, 00050 EE_Address_BLE_RESET_T, 00051 EE_Address_FINGERPRINT_RE 00052 }; 00053 00054 // Lector de Huellas 00055 extern bool functionality_fingerprint_reader; 00056 extern bool fingerprint_remotly_disable; 00057 extern bool fingerprint_override; 00058 extern int fp_override_limit; // 4 intentos 00059 00060 // Ralentí 00061 extern bool functionality_idle_shutdown; 00062 extern bool functionality_idle_reminder; 00063 extern float wait_idle_shutdown; //600.0 segundos 00064 extern float wait_idle_reminder; // 180.0 segundos 00065 00066 // Cinturones 00067 extern bool functionality_seatbelt_reading; 00068 extern bool functionality_force_driver_buclke; 00069 extern bool pilot_buckle_type; 00070 extern int pilot_buckleUp; 00071 extern int pilot_unfasten; 00072 extern bool copilot_buckle_type; 00073 extern int copilot_buckleUp; 00074 extern int copilot_unfasten; 00075 extern bool crew_buckle_type; 00076 extern int crew_buckleUp; 00077 extern int crew_unfasten; 00078 00079 // Sensor de Lluvia 00080 extern bool functionality_rain_sensor; 00081 extern bool functionality_rainSensor_silentMode; 00082 extern float time_sample_rain; // 6.0 segundos 00083 00084 // Limites de Velocidad en Mojado y seco 00085 extern int wet_Speed_Warning; // 50 Km/h 00086 extern int wet_Speed_Limit; // 55 Km/h 00087 extern int dry_Speed_Warning; // 70 Km/h 00088 extern int dry_Speed_Limit; // 80 Km/h 00089 00090 // Tip de Seguridad y Geocercas 00091 extern bool functionality_geo_warning; 00092 extern bool functionality_safety_tip; 00093 extern float time_safety_tip; // 900.0 segundos 00094 00095 // Volumen de Parlante 00096 extern int temp_JQ8400_Volume; // 4 HIGH 00097 00098 // Autoreset de BLE 00099 extern bool functionality_ble_autoreset; 00100 extern float time_ble_autoreset; 00101 00102 #define DEBUG_FLASH_CPP 00103 00104 /** 00105 * @brief 00106 * 00107 */ 00108 void load_eepromVar() { 00109 //myPC_debug.printf("\r\n FLASH - Cargando datos en Variables..."); 00110 int16_t tempData; 00111 00112 // Lector de Huellas 00113 ep.read(eprom_AddressArray[EE_Address_FINGERPRINT], tempData); 00114 functionality_fingerprint_reader = (bool) tempData; 00115 00116 ep.read(eprom_AddressArray[EE_Address_FINGERPRINT_RE], tempData); 00117 fingerprint_remotly_disable = (bool) tempData; 00118 00119 ep.read(eprom_AddressArray[EE_Address_OVERRIDE_FP], tempData); 00120 fingerprint_override = (bool) tempData; 00121 00122 ep.read(eprom_AddressArray[EE_Address_OVERRIDE_LIMIT], tempData); 00123 fp_override_limit = (int) tempData; 00124 00125 // Ralentí 00126 ep.read(eprom_AddressArray[EE_Address_IDLE_SHUTDOWN], tempData); 00127 functionality_idle_shutdown = (bool) tempData; 00128 00129 ep.read(eprom_AddressArray[EE_Address_IDLE_REMINDER], tempData); 00130 functionality_idle_reminder = (bool) tempData; 00131 00132 ep.read(eprom_AddressArray[EE_Address_IDLE_S_TIME], tempData); 00133 wait_idle_shutdown = ((int) tempData) * 1.0; 00134 00135 ep.read(eprom_AddressArray[EE_Address_IDLE_R_TIME], tempData); 00136 wait_idle_reminder = ((int) tempData) * 1.0; 00137 00138 // Sensor de Lluvia 00139 ep.read(eprom_AddressArray[EE_Address_RAINSENSOR], tempData); 00140 functionality_rain_sensor = (bool) tempData; 00141 00142 ep.read(eprom_AddressArray[EE_Address_RS_SILENTMODE], tempData); 00143 functionality_rainSensor_silentMode = (bool) tempData; 00144 00145 ep.read(eprom_AddressArray[EE_Address_RS_SAMPLE_T], tempData); 00146 time_sample_rain = ((int) tempData) * 1.0; 00147 00148 // Limites de Velocidad en Mojado y seco 00149 ep.read(eprom_AddressArray[EE_Address_WET_WARNING], tempData); 00150 wet_Speed_Warning = (int) tempData; 00151 00152 ep.read(eprom_AddressArray[EE_Address_WET_LIMIT], tempData); 00153 wet_Speed_Limit = (int) tempData; 00154 00155 ep.read(eprom_AddressArray[EE_Address_DRY_WARNING], tempData); 00156 dry_Speed_Warning = (int) tempData; 00157 00158 ep.read(eprom_AddressArray[EE_Address_DRY_LIMIT], tempData); 00159 dry_Speed_Limit = (int) tempData; 00160 00161 // Tip de Seguridad y Geocercas 00162 ep.read(eprom_AddressArray[EE_Address_SAFETY_TIP], tempData); 00163 functionality_safety_tip = (bool) tempData; 00164 00165 ep.read(eprom_AddressArray[EE_Address_SAFETY_TIP_T], tempData); // 900.0 segundos 00166 time_safety_tip = ((int) tempData) * 1.0; 00167 00168 ep.read(eprom_AddressArray[EE_Address_GEOZONE], tempData); 00169 functionality_geo_warning = (bool) tempData; 00170 00171 // Volumen de Parlante 00172 ep.read(eprom_AddressArray[EE_Address_VOLUME], tempData); 00173 temp_JQ8400_Volume = (int) tempData; 00174 00175 // Autoreset BLE 00176 ep.read(eprom_AddressArray[EE_Address_BLE_RESET], tempData); 00177 functionality_ble_autoreset = (bool) tempData; 00178 00179 ep.read(eprom_AddressArray[EE_Address_BLE_RESET_T], tempData); 00180 time_ble_autoreset = ((int) tempData) * 1.0; 00181 00182 // Cinturones 00183 ep.read(eprom_AddressArray[EE_Address_SEATBELT_READ], tempData); 00184 functionality_seatbelt_reading = (bool) tempData; 00185 00186 ep.read(eprom_AddressArray[EE_Address_ENFORCE_SB], tempData); 00187 functionality_force_driver_buclke = (bool) tempData; 00188 00189 ep.read(eprom_AddressArray[EE_Address_PILOT_BTYPE], tempData); 00190 pilot_buckle_type = (bool) tempData; 00191 00192 ep.read(eprom_AddressArray[EE_Address_COPILOT_BTYPE], tempData); 00193 copilot_buckle_type = (bool) tempData; 00194 00195 ep.read(eprom_AddressArray[EE_Address_CREW_BTYPE], tempData); 00196 crew_buckle_type = (bool) tempData; 00197 00198 // Pilot Seatbelt Buckle Type Definition 00199 if (pilot_buckle_type) { // N.O. - Honduras 00200 pilot_buckleUp = 1; 00201 pilot_unfasten = -1; 00202 } else { // N.C. - Panamá 00203 pilot_buckleUp = -1; 00204 pilot_unfasten = 1; 00205 } 00206 00207 // Copilot Seatbelt Buckle Type Definition 00208 if (copilot_buckle_type) { // N.O. - Honduras 00209 copilot_buckleUp = 1; 00210 copilot_unfasten = -1; 00211 } else { // N.C. - Panamá 00212 copilot_buckleUp = -1; 00213 copilot_unfasten = 1; 00214 } 00215 00216 // Crew Seatbelt Buckle Type Definition 00217 if (crew_buckle_type) { // N.O. - Honduras 00218 crew_buckleUp = 1; 00219 crew_unfasten = -1; 00220 } else { // N.C. - Panamá 00221 crew_buckleUp = -1; 00222 crew_unfasten = 1; 00223 } 00224 00225 #ifdef DEBUG_FLASH_CPP 00226 myPC_debug.printf("\r\n FLASH - Completado!"); 00227 myPC_debug.printf("\r\n FLASH - Valores cargados:"); 00228 myPC_debug.printf("\r\n Lector de Huellas: %s", functionality_fingerprint_reader?"TRUE":"FALSE"); 00229 myPC_debug.printf("\r\n Lector de Huellas Deshabilitado Remoto: %s", functionality_fingerprint_reader?"TRUE":"FALSE"); 00230 myPC_debug.printf("\r\n Anulacion de Lector: %s", fingerprint_override?"TRUE":"FALSE"); 00231 myPC_debug.printf("\r\n Cantidad para Anulacion: %d\r\n", fp_override_limit); 00232 myPC_debug.printf("\r\n Apagado por Ralenti: %s", functionality_idle_shutdown?"TRUE":"FALSE"); 00233 myPC_debug.printf("\r\n Recordatorio de Ralenti: %s", functionality_idle_reminder?"TRUE":"FALSE"); 00234 myPC_debug.printf("\r\n Tiempo Apagado por Ralenti: %.1f", wait_idle_shutdown); 00235 myPC_debug.printf("\r\n Tiempo Recordatorio de Ralenti: %.1f\r\n", wait_idle_reminder); 00236 myPC_debug.printf("\r\n Lectura de Cinturones: %s", functionality_seatbelt_reading?"TRUE":"FALSE"); 00237 myPC_debug.printf("\r\n Forzar Conductor Abrochado: %s\r\n", functionality_force_driver_buclke?"TRUE":"FALSE"); 00238 myPC_debug.printf("\r\n Tipo de Hebilla Piloto: %s", pilot_buckle_type?"N. OPEN":"N. CLOSE"); 00239 myPC_debug.printf("\r\n Tipo de Hebilla Copiloto: %s", copilot_buckle_type?"N. OPEN":"N. CLOSE"); 00240 myPC_debug.printf("\r\n Tipo de Hebilla Tripulante: %s", crew_buckle_type?"N. OPEN":"N. CLOSE"); 00241 myPC_debug.printf("\r\n Sensor de Lluvia: %s", functionality_rain_sensor?"TRUE":"FALSE"); 00242 myPC_debug.printf("\r\n Modo Silencioso de Lluvia: %s", functionality_rainSensor_silentMode?"TRUE":"FALSE"); 00243 myPC_debug.printf("\r\n Temporizador para Lluvia: %.1f\r\n", time_sample_rain); 00244 myPC_debug.printf("\r\n Advertencia Mojado: %d", wet_Speed_Warning); 00245 myPC_debug.printf("\r\n Limite Mojado: %d", wet_Speed_Limit); 00246 myPC_debug.printf("\r\n Advertencia Seco: %d", dry_Speed_Warning); 00247 myPC_debug.printf("\r\n Limite Seco: %d\r\n", dry_Speed_Limit); 00248 myPC_debug.printf("\r\n Notificacion Geocerca: %s", functionality_geo_warning?"TRUE":"FALSE"); 00249 myPC_debug.printf("\r\n Tip de Seguridad: %s", functionality_safety_tip?"TRUE":"FALSE"); 00250 myPC_debug.printf("\r\n Tiempo entre Tip de Seguridad: %.1f\r\n", time_safety_tip); 00251 myPC_debug.printf("\r\n Volumen Parlante: %d\r\n", temp_JQ8400_Volume); 00252 myPC_debug.printf("\r\n BLE Autoreset: %s", functionality_ble_autoreset?"TRUE":"FALSE"); 00253 myPC_debug.printf("\r\n BLE Temporizador: %.1f\r\n\r\n", time_ble_autoreset); 00254 #endif 00255 } 00256 00257 void eeprom_Default() { 00258 00259 //myPC_debug.printf("\r\n FLASH - Cargando valores por defecto"); 00260 #define SEATBELT_CONFIG 7 00261 00262 ep.write(eprom_AddressArray[EE_Address_FINGERPRINT], ((uint16_t) true)); // Fingerprint Enable 00263 ep.write(eprom_AddressArray[EE_Address_OVERRIDE_FP], ((uint16_t) true)); // Override Fingerprint Authentication 00264 ep.write(eprom_AddressArray[EE_Address_OVERRIDE_LIMIT], ((uint16_t) 4)); // Override Fingerprint Quantity 00265 ep.write(eprom_AddressArray[EE_Address_IDLE_SHUTDOWN], ((uint16_t) true)); // Idle Shutdown Enable 00266 ep.write(eprom_AddressArray[EE_Address_IDLE_REMINDER], ((uint16_t) true)); // Idle Shutdown Reminder 00267 ep.write(eprom_AddressArray[EE_Address_IDLE_S_TIME], ((uint16_t) 600)); // Idle Shutdown Cutoff Time 00268 ep.write(eprom_AddressArray[EE_Address_IDLE_R_TIME], ((uint16_t) 180)); // Idle Shutdown Reminder Time 00269 ep.write(eprom_AddressArray[EE_Address_SEATBELT_READ], ((uint16_t) true)); // Seatbelt reading Enable 00270 ep.write(eprom_AddressArray[EE_Address_ENFORCE_SB], ((uint16_t) true)); // Force Driver Buckleup 00271 #if (SEATBELT_CONFIG == 0) 00272 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00273 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00274 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00275 #elif (SEATBELT_CONFIG == 1) 00276 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00277 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00278 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00279 #elif (SEATBELT_CONFIG == 2) 00280 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00281 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00282 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00283 #elif (SEATBELT_CONFIG == 3) 00284 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00285 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00286 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00287 #elif (SEATBELT_CONFIG == 4) 00288 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00289 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00290 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00291 #elif (SEATBELT_CONFIG == 5) 00292 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00293 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00294 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00295 #elif (SEATBELT_CONFIG == 6) 00296 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00297 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00298 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) false)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00299 #elif (SEATBELT_CONFIG == 7) 00300 ep.write(eprom_AddressArray[EE_Address_PILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00301 ep.write(eprom_AddressArray[EE_Address_COPILOT_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00302 ep.write(eprom_AddressArray[EE_Address_CREW_BTYPE], ((uint16_t) true)); // Seatbelt reading Type (FALSE = PA | TRUE = HN) 00303 #endif 00304 ep.write(eprom_AddressArray[EE_Address_RAINSENSOR], ((uint16_t) true)); // Rain Sensor Enable 00305 ep.write(eprom_AddressArray[EE_Address_RS_SILENTMODE], ((uint16_t) false)); // Silent mode Rain Sensor 00306 ep.write(eprom_AddressArray[EE_Address_RS_SAMPLE_T], ((uint16_t) 6)); // Sample Time Rain Sensor 00307 ep.write(eprom_AddressArray[EE_Address_WET_LIMIT], ((uint16_t) 55)); // Wet Speed Limit 00308 ep.write(eprom_AddressArray[EE_Address_WET_WARNING], ((uint16_t) 50)); // Wet Speed Warning 00309 ep.write(eprom_AddressArray[EE_Address_DRY_LIMIT], ((uint16_t) 80)); // Dry Speed Limit 00310 ep.write(eprom_AddressArray[EE_Address_DRY_WARNING], ((uint16_t) 70)); // Dry Speed Warning 00311 ep.write(eprom_AddressArray[EE_Address_SAFETY_TIP], ((uint16_t) true)); // Enable Safety Tip 00312 ep.write(eprom_AddressArray[EE_Address_SAFETY_TIP_T], ((uint16_t) 1800)); // Time for Safety Tip 00313 ep.write(eprom_AddressArray[EE_Address_GEOZONE], ((uint16_t) false)); // Geozone alert 00314 ep.write(eprom_AddressArray[EE_Address_VOLUME], ((uint16_t) 5)); // Volume Level 6=Maximum 00315 ep.write(eprom_AddressArray[EE_Address_BLE_RESET], ((uint16_t) true)); // BLE Autoreset 00316 ep.write(eprom_AddressArray[EE_Address_BLE_RESET_T], ((uint16_t) 3600)); // BLE Autoreset Time 00317 ep.write(eprom_AddressArray[EE_Address_FINGERPRINT_RE], ((uint16_t) false)); // Fingerprint Enable 00318 00319 // LED 00320 flashLED = 1; 00321 wait_ms(3750); 00322 flashLED = 0; 00323 #ifdef DEBUG_FLASH_CPP 00324 myPC_debug.printf("\r\n FLASH - Completado!"); 00325 #endif 00326 } 00327 00328 void writeEE(int myAddress, int myValue) { 00329 ep.write(((uint32_t) myAddress), ((uint16_t) myValue)); 00330 }
Generated on Thu Jul 28 2022 19:27:02 by
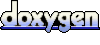