
Update to work with Grove
Dependencies: DigitDisplay RangeFinder Pulse Grove_temperature FXOS8700Q
main.cpp
00001 #include "mbed.h" 00002 #include "Grove_temperature.h" 00003 #include "LED_Bar.h" 00004 #include "DigitDisplay.h" 00005 #include "RangeFinder.h" 00006 #include "FXOS8700Q.h" 00007 00008 #define V_SERVO_CENTER 1400 00009 #define V_SERVO_MAX 2200 00010 #define V_SERVO_MIN 800 00011 00012 Serial pc(USBTX, USBRX); // Serial Port 115200 00013 00014 I2C i2c( PTE25, PTE24 ); 00015 FXOS8700QAccelerometer acc( i2c, FXOS8700CQ_SLAVE_ADDR1 ); // Accelerometer 00016 00017 Grove_temperature temp(A3); // Temperature GPIO 00018 DigitalOut myled(LED2); //Red LED 00019 DigitDisplay display(D7, D8); // Digital Display GPIO 00020 00021 AnalogIn xAxis(A0); // Joystick x GPIO 00022 AnalogIn yAxis(A1); // Joystick y GPIO 00023 00024 // Servo 00025 PwmOut v_servo(D3); // D3 output 00026 00027 LED_Bar bar(D6, D5); // LED_Bar GPIO 00028 00029 int x,y,button; // global variables to hold values 00030 00031 // Seeed ultrasound range finder 00032 RangeFinder rf(A2, 10, 5800.0, 100000); 00033 DigitalOut led(LED1); 00034 00035 00036 Ticker joystick; // recurring interrupt to get joystick data 00037 Ticker tick; // Digital display clock 00038 00039 // Digital Display variables 00040 uint8_t hour = 20; 00041 uint8_t minute = 14; 00042 uint8_t second = 0; 00043 00044 // Digital Display Algorythm Function 00045 void beat() 00046 { 00047 static uint8_t colon = 0; 00048 00049 display.setColon(colon); 00050 if (colon) { 00051 second++; 00052 if (second >= 60) { 00053 second = 0; 00054 minute++; 00055 if (minute >= 60) { 00056 minute = 0; 00057 00058 hour++; 00059 if (hour >= 24) { 00060 hour = 0; 00061 } 00062 display.write(0, hour / 10); 00063 display.write(1, hour % 10); 00064 } 00065 display.write(2, minute / 10); 00066 display.write(3, minute % 10); 00067 } 00068 } 00069 colon = 1 - colon; 00070 } 00071 00072 // Joystick Algorythm Function 00073 void joystick_Int_Handler() 00074 { 00075 x = xAxis.read() * 1000; // float (0->1) to int (0-1000) 00076 y = yAxis.read() * 1000; 00077 if ( (x > 900) || (y > 900) ) 00078 button = 1; 00079 else 00080 button = 0; 00081 } 00082 00083 // main() runs in its own thread in the OS 00084 int main() { 00085 int i; 00086 pc.baud(115200); // Serial Port 115200 00087 00088 led = 1; //Rangefinder 00089 float d; //Rangefinder 00090 00091 float acc_x, acc_y, acc_z; //Accelerometer 00092 00093 // Servo 00094 int v_pulse = V_SERVO_CENTER; 00095 v_servo.period_us(20000); // servo requires a 20ms period 00096 v_servo.pulsewidth_us(v_pulse); // servo position determined by a pulsewidth between 1-2ms 00097 wait(1); 00098 00099 // Joystick interrupt, call every .2s 00100 joystick.attach(joystick_Int_Handler,0.2); 00101 display.write(0, hour / 10); 00102 display.write(1, hour % 10); 00103 display.write(2, minute / 10); 00104 display.write(3, minute % 10); 00105 display.setColon(true); 00106 00107 // Dig Display interrupt, call every .5s 00108 tick.attach(&beat, 0.5); 00109 00110 // Enable Accelerometer 00111 acc.enable(); 00112 00113 while (true) { 00114 printf("\r\nTemperature Measurement\r\n"); 00115 pc.printf("\rtemperature = %2.2f\n", temp.getTemperature()); 00116 00117 printf("\r\nJoystick Status, Meters\r\n"); 00118 pc.printf("\rX=%3d, Y=%3d, Button=%d\n",x,y,button); 00119 00120 //for (i=0; i<=10; i++) { 00121 //bar.setLevel(i); 00122 //wait(0.1); 00123 //} 00124 bar.setLevel((x-250)/50); 00125 00126 00127 //Servo 00128 v_pulse = v_pulse - 20; 00129 if (v_pulse <= V_SERVO_MIN) v_pulse = V_SERVO_MIN; 00130 if (v_pulse >= V_SERVO_MAX) v_pulse = V_SERVO_MAX; 00131 v_servo.pulsewidth_us(v_pulse); // servo position determined by a pulsewidth between 1-2ms 00132 //wait(3); 00133 printf("\n\rPulse = %d\n\r",v_pulse); 00134 00135 00136 //Range sensor 00137 d = rf.read_m(); 00138 if (d == -1.0) { 00139 printf("\rTimeout Error.\n"); 00140 } else if (d > 5.0) { 00141 // Seeed's sensor has a maximum range of 4m, it returns 00142 // something like 7m if the ultrasound pulse isn't reflected. 00143 printf("\rNo object within detection range.\n"); 00144 } else { 00145 printf("\r\nUltra Sonic Rangefinder, Meters\r\n"); 00146 printf("\rDistance = %f m.\n", d); 00147 } 00148 00149 // get Accelerometer values 00150 acc.getX( acc_x ); 00151 acc.getY( acc_y ); 00152 acc.getZ( acc_z ); 00153 00154 printf("\r\nAccelerometer Values\r\n"); 00155 printf("X:%6.1f, Y:%6.1f, Z:%6.1f\r\n\r\n", acc_x * 90.0, acc_y * 90.0, acc_z * 90.0 ); 00156 00157 myled = 1; 00158 wait(0.5); 00159 myled = 0; 00160 wait(0.5); 00161 00162 led = !led; 00163 } 00164 } 00165 00166
Generated on Wed Jul 13 2022 06:29:10 by
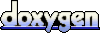