
Update to work with Grove
Dependencies: DigitDisplay RangeFinder Pulse Grove_temperature FXOS8700Q
LED_Bar.h
00001 /* 00002 LED bar library V1.0 00003 2010 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Original Author: LG 00006 Modify: Loovee, 2014-2-26 00007 User can choose which Io to be used. 00008 00009 This library is free software; you can redistribute it and/or 00010 modify it under the terms of the GNU Lesser General Public 00011 License as published by the Free Software Foundation; either 00012 version 2.1 of the License, or (at your option) any later version. 00013 00014 This library is distributed in the hope that it will be useful, 00015 but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00017 Lesser General Public License for more details. 00018 00019 You should have received a copy of the GNU Lesser General Public 00020 License along with this library; if not, write to the Free Software 00021 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00022 */ 00023 00024 #include "mbed.h" 00025 00026 #ifndef LED_Bar_H 00027 #define LED_Bar_H 00028 00029 #define CMDMODE 0x0000 // Work on 8-bit mode 00030 #define ON 0x00ff // 8-bit 1 data 00031 #define SHUT 0x0000 // 8-bit 0 data 00032 00033 /** 00034 * The LED_Bar interface 00035 */ 00036 class LED_Bar 00037 { 00038 00039 public: 00040 LED_Bar(PinName pinClk, PinName pinDta); 00041 00042 /** 00043 * Set led single bit, a bit contrl a led 00044 * @param index_bits which bit. if 0x05, then led 0 and led 3 will on, the others will off 00045 */ 00046 void ledIndexBit(unsigned int index_bits); 00047 00048 /** 00049 * Set level, frm 0 to 10. 00050 * @param level Level 0 means all leds off while level 5 means 5led on and the other will off 00051 */ 00052 void setLevel(int level); 00053 00054 /** 00055 * Control a single led 00056 * @param num which led 00057 * @param st 1: on 0: off 00058 */ 00059 void setSingleLed(int num, int st); 00060 00061 private: 00062 /** 00063 * Pin for clock 00064 */ 00065 DigitalOut __pinClk; 00066 00067 /** 00068 * Pin for data 00069 */ 00070 DigitalOut __pinDta; 00071 00072 /** 00073 * LED State 00074 */ 00075 unsigned int __led_state; 00076 00077 void send16bitData(unsigned int data); 00078 void latchData(void); 00079 00080 }; 00081 00082 #endif
Generated on Wed Jul 13 2022 06:29:10 by
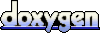