
Update to work with Grove
Dependencies: DigitDisplay RangeFinder Pulse Grove_temperature FXOS8700Q
LED_Bar.cpp
00001 /* 00002 LED bar library V1.0 00003 2010 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Original Author: LG 00006 00007 Modify: Mihail Stoyanov (mihail.stoyanov@arm.com) for ARM mbed, 2014-07-30 00008 User can choose which Io to be used. 00009 This library is free software; you can redistribute it and/or 00010 modify it under the terms of the GNU Lesser General Public 00011 License as published by the Free Software Foundation; either 00012 version 2.1 of the License, or (at your option) any later version. 00013 00014 This library is distributed in the hope that it will be useful, 00015 but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00017 Lesser General Public License for more details. 00018 00019 You should have received a copy of the GNU Lesser General Public 00020 License along with this library; if not, write to the Free Software 00021 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00022 */ 00023 00024 #include "LED_Bar.h" 00025 00026 LED_Bar::LED_Bar(PinName pinClk, PinName pinDta) : __pinClk(pinClk), __pinDta(pinDta) 00027 { 00028 __led_state = 0x00; 00029 } 00030 00031 void LED_Bar::latchData() 00032 { 00033 __pinDta = 0; 00034 wait_us(10); 00035 00036 for(int i=0; i<4; i++) { 00037 __pinDta = 1; 00038 __pinDta = 0; 00039 } 00040 00041 } 00042 00043 void LED_Bar::send16bitData(unsigned int data) 00044 { 00045 for(int i=0; i<16; i++) { 00046 unsigned int state = data & 0x8000 ? 1 : 0; 00047 __pinDta = state; 00048 00049 state = __pinClk ? 0 : 1; 00050 __pinClk = state; 00051 00052 data <<= 1; 00053 } 00054 } 00055 00056 void LED_Bar::ledIndexBit(unsigned int index_bits) 00057 { 00058 00059 send16bitData(CMDMODE); 00060 00061 for (int i=0; i<12; i++) { 00062 unsigned int state = (index_bits&0x0001) ? ON : SHUT; 00063 send16bitData(state); 00064 00065 index_bits = index_bits>>1; 00066 } 00067 00068 latchData(); 00069 } 00070 00071 void LED_Bar::setLevel(int level) 00072 { 00073 00074 if(level>10)return; 00075 00076 send16bitData(CMDMODE); 00077 00078 for(int i=0; i<12; i++) { 00079 unsigned int state1 = (i<level) ? ON : SHUT; 00080 00081 send16bitData(state1); 00082 } 00083 00084 latchData(); 00085 } 00086 00087 void LED_Bar::setSingleLed(int num, int st) 00088 { 00089 if(num>10)return; 00090 __led_state = st ? (__led_state | (0x01<<num)) : (__led_state & ~(0x01<<num)); 00091 ledIndexBit(__led_state); 00092 00093 }
Generated on Wed Jul 13 2022 06:29:10 by
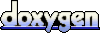