
max32630
Dependencies: OLED USBDevice max32630fthr
Fork of FTHR_OLED by
main.cpp
00001 #include "mbed.h" 00002 #include "max32630fthr.h" 00003 #include "Adafruit_SSD1306.h" 00004 #include "USBSerial.h" 00005 00006 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00007 00008 I2C oledI2C(P3_4, P3_5); // SDA, SCL 00009 00010 // Hardware serial port over DAPLink 00011 Serial daplink(P2_1, P2_0); 00012 00013 // serial port for pm1003 00014 Serial pmSerial(P3_1, P3_0); 00015 //for 595 drive LED bar 00016 DigitalOut ledbarClk(P5_3); 00017 DigitalOut ledbardat(P5_4); 00018 DigitalOut ledbarlatch(P5_5); 00019 DigitalOut ledEnable(P5_6); 00020 int airBufferPrcess( float a, float b); 00021 void shirftDate(unsigned int date); 00022 /* Analog inputs 0 and 1 have internal dividers to allow measuring 5V signals 00023 * The dividers are selected by using inputs AIN_5 and AIN_5 respectively. 00024 * The full scale range for AIN0-3 is 1.2V 00025 * The full scale range for AIN4-5 is 6.0V 00026 */ 00027 AnalogIn fireAir(AIN_4); 00028 AnalogIn alcohol(AIN_5); 00029 00030 // main() runs in its own thread in the OS 00031 // (note the calls to Thread::wait below for delays) 00032 int main() 00033 { 00034 char incomeByte[32]; 00035 unsigned int pm25; 00036 unsigned int pm1; 00037 unsigned int pm10; 00038 unsigned int airDisplayDate; 00039 //unsigned int alcoholDate,fireAirDate; 00040 00041 //daplink.printf("start \r\n"); 00042 pmSerial.baud(9600); 00043 00044 Thread::wait(50); // Give the supplies time to settle before initializing the display 00045 Adafruit_SSD1306_I2c OLED(oledI2C); 00046 //OLED.printf("%ux%u OLED Display\r\n", OLED.width(), OLED.height()); 00047 //OLED.printf("HelloWorld \r"); 00048 //OLED.display(); 00049 00050 //daplink.printf("OLED init over \r\n"); 00051 while(1) { 00052 Thread::wait(250); 00053 00054 while(pmSerial.getc() !=0x42){} 00055 if(pmSerial.getc() == 0x4d){ 00056 for(int i = 2; i < 32; i++){ 00057 incomeByte[i] = pmSerial.getc(); 00058 } 00059 00060 unsigned int calcsum = 0; 00061 incomeByte[0] = 0x42; 00062 incomeByte[1] = 0x4d; 00063 unsigned int exptsum = (incomeByte[30]<<8) + incomeByte[31]; 00064 for(int i = 0; i < 30; i++){ 00065 calcsum += incomeByte[i]; 00066 // daplink.printf("income[%d],%d\n",i,incomeByte[i]); 00067 } 00068 if( calcsum == exptsum) 00069 { 00070 //daplink.printf("check ok \n"); 00071 pm1 = incomeByte[10] + incomeByte[11]; 00072 pm25 = incomeByte[12] + incomeByte[13]; 00073 pm10 = incomeByte[14] + incomeByte[15]; 00074 00075 //daplink.printf("pm1:%d\n",pm1); 00076 //daplink.printf("pm2.5:%d\n",pm25); 00077 //daplink.printf("pm10:%d\n",pm10); 00078 00079 } 00080 00081 } 00082 00083 Thread::wait(10); 00084 OLED.clearDisplay(); 00085 OLED.setTextCursor(0,0); 00086 OLED.printf("Car air memter \n"); 00087 OLED.printf("CO: %1.2f\n", (6.0f * fireAir) ); // fireAir inputs 4 00088 OLED.printf("alcohol: %1.2f\n", (6.0f * alcohol) ); // alcohol inputs 5 00089 OLED.printf("PM2.5: %d \n", pm25 ); // 00090 OLED.printf("PM10 : %d \n", pm10 ); // 00091 OLED.printf("PM1 : %d \n", pm1 ); // 00092 OLED.display(); 00093 daplink.printf("CO : %1.2f\n", (6.0f * fireAir) ); 00094 daplink.printf("alcohol: %1.2f\n", (6.0f * alcohol) ); 00095 00096 airDisplayDate = airBufferPrcess( (6.0f * fireAir), (6.0f *alcohol) ); 00097 daplink.printf("date for shirtf:%d\n",airDisplayDate); 00098 ledEnable = 1; 00099 ledEnable = 0; 00100 shirftDate( (~airDisplayDate)<<4 ); 00101 Thread::wait(500); 00102 //shirftDate( 0x00ffff); 00103 00104 while((pm25 > 100)||(fireAir > 1)||(alcohol > 1)){ 00105 00106 OLED.clearDisplay(); 00107 Thread::wait(50); 00108 OLED.printf("ERROR \n"); 00109 OLED.printf("ERROR \n"); 00110 OLED.printf("ERROR \n"); 00111 OLED.printf("ERROR \n"); 00112 OLED.printf("ERROR \n"); 00113 OLED.printf("ERROR \n"); 00114 OLED.display(); 00115 Thread::wait(500); 00116 } 00117 00118 00119 } 00120 } 00121 00122 /*传入LB数据,共24位取低位24*/ 00123 /*低20位用于两个LED bar*/ 00124 void shirftDate(unsigned int date){ 00125 unsigned int _date = date; 00126 //_date &= 0xfffff; 00127 for( int i = 24; i >= 0; i-- ){ 00128 if( _date & (0x01 << i ) ){ 00129 ledbardat = 1; 00130 }else{ 00131 ledbardat = 0; 00132 } 00133 // daplink.printf("date ledbardat:%d\n",ledbardat); 00134 Thread::wait(0.5); 00135 ledbarClk = 0; 00136 Thread::wait(0.5); 00137 ledbarClk = 1; 00138 } 00139 // /*补充4位空白*/ 00140 // for(int i= 0; i < 4 ; i++){ 00141 // ledbardat = 0; 00142 // ledbarClk = 1; 00143 // Thread::wait(0.5); 00144 // ledbarClk = 0; 00145 // } 00146 00147 ledbarlatch = 0; 00148 Thread::wait(0.5); 00149 ledbarlatch = 1; 00150 00151 } 00152 00153 //空气模拟数据处理,a,b分别表示 CO与acohol; 00154 //采用对数级浓度增加对应一位, 00155 int airBufferPrcess( float a, float b) 00156 { 00157 unsigned int _a , _b; 00158 _a = (unsigned int)( a / 0.1); 00159 _b = (unsigned int)( b / 0.1); 00160 daplink.printf("airDisplayDate:%d\n",_a); 00161 daplink.printf("airDisplayDate:%d\n",_b); 00162 unsigned int _ProcessDate[3]={1,1,0}; 00163 for(int i=0;i<10;i++){ 00164 _a >>= 1; 00165 _b >>= 1; 00166 if(_a > 0){ 00167 _ProcessDate[0] <<= 1; 00168 _ProcessDate[0]++; 00169 } 00170 if(_b > 0){ 00171 _ProcessDate[1] <<= 1; 00172 _ProcessDate[1]++; 00173 } 00174 _ProcessDate[2] = ( _ProcessDate[0] << 10); 00175 _ProcessDate[2] += _ProcessDate[1]; 00176 } 00177 00178 return _ProcessDate[2]; 00179 }
Generated on Tue Jul 12 2022 21:39:29 by
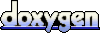