Small library for using circular buffers
Dependents: CircularBufferExample
CircularBuffer< T > Class Template Reference
This class implements a static circular buffer. More...
#include <CircularBuffer.h>
Public Member Functions | |
CircularBuffer () | |
Default constructor. | |
uint32_t | read (uint8_t *data, uint32_t length) |
Reads data from buffer. | |
uint32_t | write (uint8_t *data, uint32_t length) |
Writes data in buffer. | |
uint32_t | getCapacity () const |
Returns the total capacity of this buffer. | |
uint32_t | getSize () const |
Returns the number of bytes available in the buffer. | |
bool | isEmpty () const |
Checks if this buffer is empty. | |
bool | isFull () const |
Checks if this buffer is full. |
Detailed Description
template<size_t T>
class CircularBuffer< T >
This class implements a static circular buffer.
Definition at line 9 of file CircularBuffer.h.
Constructor & Destructor Documentation
CircularBuffer | ( | ) |
Default constructor.
Definition at line 65 of file CircularBuffer.h.
Member Function Documentation
uint32_t getCapacity | ( | ) | const |
Returns the total capacity of this buffer.
- Returns:
- Capacity of buffer
Definition at line 103 of file CircularBuffer.h.
uint32_t getSize | ( | ) | const |
Returns the number of bytes available in the buffer.
- Returns:
- Number of bytes available in the buffer
Definition at line 109 of file CircularBuffer.h.
bool isEmpty | ( | ) | const |
Checks if this buffer is empty.
- Returns:
- True if the buffer is empty, false otherwise
Definition at line 115 of file CircularBuffer.h.
bool isFull | ( | ) | const |
Checks if this buffer is full.
- Returns:
- True if the buffer is full, false otherwise
Definition at line 121 of file CircularBuffer.h.
uint32_t read | ( | uint8_t * | data, |
uint32_t | length | ||
) |
Reads data from buffer.
- Parameters:
-
data output buffer length Maximum number of bytes to read
- Returns:
- Number of bytes read
- Note:
- The return value cannot exceed max(length,capacity)
Definition at line 73 of file CircularBuffer.h.
uint32_t write | ( | uint8_t * | data, |
uint32_t | length | ||
) |
Writes data in buffer.
- Parameters:
-
data input buffer length Maximum number of bytes to write
- Returns:
- Number of bytes wrote
- Note:
- The return value cannot exceed max(length,capacity)
Definition at line 88 of file CircularBuffer.h.
Generated on Tue Jul 19 2022 02:38:08 by
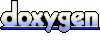