
ok
Dependencies: mbed AnalogIn_Diff_ok MovingAverage_ok
trms.h
00001 /* 00002 * Copyright (c) 2014 LAAS-CNRS 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef TRMS_H 00018 #define TRMS_H 00019 00020 #include "mbed.h" 00021 #include "AnalogIn_Diff.h" 00022 #include "math.h" 00023 #include "MovingAverage.h" 00024 00025 00026 #define FREQ 50//en HZ 00027 #define TSAMPLE 125 //en µS 00028 #define R1 1.0E6 00029 #define R2 510.0 00030 #define ADCVREF 3.3 00031 #define GAIN_ACPL_C78 0.125 00032 #define GAIN ((double)((R1+R2)*2.0*GAIN_ACPL_C78*ADCVREF/R2)/65535.0) 00033 #define UAC_NON 230.0 00034 #define UAC_MON_i32 ((int32_t)((double)UAC_NON/(double)GAIN)) 00035 #define UAC_MAX ((int32_t)((double)UAC_NON*1.1/(double)GAIN)) 00036 #define UAC_MIN ((int32_t)((double)UAC_NON*0.9/(double)GAIN)) 00037 #define UAC_NON2 ((int32_t)((double)UAC_NON/(double)GAIN*(double)UAC_NON/(double)GAIN)) 00038 #define UAC_MAX2 ((int32_t)((double)UAC_MAX*(double)UAC_MAX)) 00039 #define UAC_MIN2 ((int32_t)((double)UAC_MIN*(double)UAC_MIN)) 00040 #define UAC_MAX2STOP ((int32_t)((double)UAC_MAX*(double)UAC_MAX)*0.95) 00041 #define UAC_MIN2STOP ((int32_t)((double)UAC_MIN*(double)UAC_MIN)*1.05) 00042 #define NSAMPLE ((int32_t)(1/(double)FREQ *1.0E6/(double)TSAMPLE)) 00043 //#define max(a,b) ((a)>=(b)?(a):(b)) 00044 //#define min(a,b) ((a)<=(b)?(a):(b)) 00045 00046 #define MAX(a,b) ({ typeof(a) aa = (a); typeof(b) bb = (b); aa>=bb? aa: bb; }) 00047 #define MIN(a,b) ({ typeof(a) aa = (a); typeof(b) bb = (b); aa<=bb? aa: bb; }) 00048 00049 #define VERSION_TRMS "2014_12_10" 00050 00051 00052 class trms : public AnalogIn_Diff 00053 { 00054 00055 public: 00056 /** 00057 * Constructor 00058 * 00059 * @param a2d_number_chan is ADC_DIFF(#adc, #ch) 00060 * @return true if successful 00061 */ 00062 00063 trms(int adc_Diff_number_chan) ; 00064 00065 /** 00066 * destructor 00067 */ 00068 ~trms(); 00069 00070 void start(); 00071 float read_rms(); 00072 float get_gain(); 00073 void set_gain(float gain); 00074 float get_offset(); 00075 void set_offset(float offset); 00076 bool flag(float *rms, uint32_t *time); 00077 00078 private: 00079 volatile bool _flag_trms; 00080 volatile bool _flag_max; 00081 volatile bool _flag_min; 00082 00083 volatile int32_t _min; 00084 volatile int32_t _max; 00085 volatile float gain; 00086 volatile float offset; 00087 volatile bool F_timer_min; 00088 volatile bool F_timer_max; 00089 volatile uint32_t _time_min; 00090 volatile uint32_t _time_max; 00091 00092 Timer timer_min; 00093 Timer timer_max; 00094 void flipadc_Diff(); 00095 MovingAverage <uint32_t>vtrms; 00096 Ticker flipperadc_Diff; 00097 00098 00099 }; 00100 00101 #endif //TRMS_H
Generated on Tue Jul 12 2022 19:14:37 by
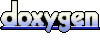