
ok
Dependencies: mbed AnalogIn_Diff_ok MovingAverage_ok
trms.cpp
00001 #include "trms.h" 00002 00003 #define DEBUG 1 00004 //Debug is disabled by default 00005 #if (defined(DEBUG)) 00006 00007 #define DBG(x, ...) std::printf("[trms : DBG]"x"\r\n", ##__VA_ARGS__); 00008 #define WARN(x, ...) std::printf("[trms : WARN]"x"\r\n", ##__VA_ARGS__); 00009 #define ERR(x, ...) std::printf("[trms : ERR]"x"\r\n", ##__VA_ARGS__); 00010 #define INFO(x, ...) std::printf("[trms : INFO]"x"\r\n", ##__VA_ARGS__); 00011 00012 #else 00013 00014 #define DBG(x, ...) 00015 #define WARN(x, ...) 00016 #define ERR(x, ...) 00017 #define INFO(x, ...) 00018 #endif 00019 00020 trms::trms(int adc_Diff_number_chan) : AnalogIn_Diff(adc_Diff_number_chan), vtrms(NSAMPLE,UAC_NON2) 00021 { 00022 00023 timer_min.stop(); 00024 timer_max.stop(); 00025 timer_min.reset(); 00026 timer_max.reset(); 00027 _time_min=0; 00028 F_timer_min=false; 00029 _time_max=0; 00030 F_timer_max=false; 00031 _min=UAC_NON2; 00032 _max=UAC_NON2; 00033 gain=GAIN; 00034 offset=0.0; 00035 _flag_trms=false; 00036 DBG("Unon =%0.0fV",UAC_NON); 00037 DBG("RAW Umax2 =%d",UAC_MAX2); 00038 DBG("RAW Umin2 =%d",UAC_MIN2); 00039 DBG("Gain =%f",gain); 00040 00041 00042 DBG("Freq UAC=%dHz",FREQ); 00043 DBG("Sample =%d",NSAMPLE); 00044 DBG("Sample =%dus",TSAMPLE); 00045 00046 00047 00048 } 00049 00050 00051 00052 float trms::read_rms() 00053 { 00054 return (sqrt((float)vtrms.GetAverage())*gain-offset); 00055 00056 } 00057 00058 /** 00059 * @brief Destructor. 00060 */ 00061 trms::~trms() 00062 { 00063 00064 flipperadc_Diff.detach(); 00065 } 00066 00067 void trms::start() 00068 { 00069 flipperadc_Diff.attach_us<trms>(this,&trms::flipadc_Diff, TSAMPLE); 00070 } 00071 00072 00073 00074 void trms::flipadc_Diff() 00075 { 00076 int16_t val_i16; 00077 uint32_t val2_ui32; 00078 00079 val_i16=read_raws16(); 00080 00081 vtrms.Insert(val_i16*val_i16); 00082 00083 val2_ui32=vtrms.GetAverage(); 00084 00085 //MIN 00086 if(val2_ui32<UAC_MIN2 && F_timer_min ==false) { 00087 00088 timer_min.start(); 00089 F_timer_min = true; 00090 00091 } 00092 00093 if(F_timer_min ==true) { 00094 if(val2_ui32>UAC_MIN2STOP ) { 00095 00096 timer_min.stop(); 00097 F_timer_min = false; 00098 _flag_trms=true; //????????????????? 00099 _flag_min=true; 00100 } 00101 00102 00103 else if(timer_min.read_ms()>20) { 00104 00105 _time_min=timer_min.read_ms(); 00106 _min=MIN(val2_ui32,_min); 00107 00108 } 00109 //max 00110 if(val2_ui32<UAC_MAX2STOP && F_timer_max ==true) { 00111 timer_max.stop(); 00112 F_timer_max = false; 00113 _flag_trms=true; 00114 _flag_max=true; 00115 } 00116 if(val2_ui32>UAC_MAX2 && F_timer_max ==false) { 00117 00118 timer_max.start(); 00119 F_timer_max = true; 00120 00121 } 00122 if(timer_max.read_ms()>20 && F_timer_max ==true) { 00123 00124 _time_max=timer_max.read_ms(); 00125 _max=MAX(val2_ui32,_max); 00126 00127 } 00128 } 00129 00130 } 00131 00132 bool trms::flag (float *rms, uint32_t *time) 00133 { 00134 bool old_flag =_flag_trms; 00135 00136 if(_flag_trms==true) { 00137 _flag_trms=false; 00138 if(_flag_min==true) { 00139 00140 *time=_time_min; 00141 timer_min.reset(); 00142 *rms=(sqrt((float)_min)*gain-offset); 00143 _min=UAC_NON2; 00144 _flag_min=false; 00145 00146 } 00147 00148 else if(_flag_max==true) { 00149 00150 *time=_time_max; 00151 timer_max.reset(); 00152 *rms=(sqrt((float)_max)*gain-offset); 00153 _max=UAC_NON2; 00154 _flag_max=false; 00155 00156 } 00157 00158 } else { 00159 00160 *rms=read_rms(); 00161 *time=0; 00162 } 00163 00164 return old_flag; 00165 00166 } 00167 00168 00169 void trms::set_gain(float _gain) 00170 { 00171 gain=_gain; 00172 } 00173 00174 void trms::set_offset(float _offset) 00175 { 00176 offset=_offset; 00177 } 00178 float trms::get_gain() 00179 { 00180 return gain; 00181 } 00182 00183 float trms::get_offset() 00184 { 00185 return offset; 00186 }
Generated on Tue Jul 12 2022 19:14:37 by
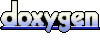