Library for debouncing inputs, originally by Andres Mora Bedoya. Updated to include PinMode capability and class documentation.
Fork of DebouncedIn by
DebouncedIn.h
00001 /** 00002 * DebouncedIn class version 1.0 00003 * Created by Andres Moya Bedoya, updated by Ben Faucher 00004 */ 00005 00006 #include "mbed.h" 00007 00008 #ifndef _DEBOUNCED_IN_H_ 00009 #define _DEBOUNCED_IN_H_ 00010 00011 /** 00012 * DebouncedIn object, uses software tickers to debounce mechanical inputs. 00013 */ 00014 00015 class DebouncedIn { 00016 public: 00017 /** Create a DebouncedIn connected to the specified pin 00018 * 00019 * @param in DigitalIn pin to connect to 00020 */ 00021 DebouncedIn(PinName in); 00022 00023 /** Same as before, with option to specify pin mode 00024 * 00025 * @param in DigitalIn pin to connect to 00026 * @param mode (optional) Set pull mode - PullUp, PullDown, PullNone, OpenDrain 00027 */ 00028 DebouncedIn(PinName in, PinMode mode); 00029 00030 /** Read the input state, represented as 0 or 1 (int) 00031 * 00032 * @returns 00033 * An integer representing the state of the input pin, 00034 * 0 for logical 0, 1 for logical 1. State changes when input 00035 * has been steady for at least 40ms (8 ticker cycles of 5ms). 00036 */ 00037 int read (void); 00038 00039 /** An operator shorthand for read() 00040 */ 00041 operator int(); 00042 00043 /** Rising edge count (int) 00044 * 00045 * @returns 00046 * An integer representing the number of times the switch has 00047 * changed from low to high. Count resets to zero when this 00048 * function is called. 00049 */ 00050 int rising(void); 00051 00052 /** Falling edge count (int) 00053 * 00054 * @returns 00055 * An integer representing the number of times the switch has 00056 * changed from high to low. Count resets to zero when this 00057 * function is called. 00058 */ 00059 int falling(void); 00060 00061 /** Steady state tick count (int) 00062 * 00063 * @returns 00064 * An integer representing how many ticker cycles the input has been 00065 * steady for. Ticker cycles every 5ms. 00066 */ 00067 int steady(void); 00068 00069 private : 00070 // objects 00071 DigitalIn _in; 00072 Ticker _ticker; 00073 00074 // function to take a sample, and update flags 00075 void _sample(void); 00076 00077 // counters and flags 00078 int _samples; 00079 int _output; 00080 int _output_last; 00081 int _rising_flag; 00082 int _falling_flag; 00083 int _state_counter; 00084 00085 }; 00086 00087 #endif
Generated on Sun Jul 24 2022 09:39:58 by
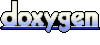