Library for debouncing inputs, originally by Andres Mora Bedoya. Updated to include PinMode capability and class documentation.
Fork of DebouncedIn by
DebouncedIn.cpp
00001 /* 00002 * DebouncedIn class version 1.0 00003 * Created by Andres Moya Bedoya, updated by Ben Faucher 00004 */ 00005 00006 #include "DebouncedIn.h" 00007 #include "mbed.h" 00008 00009 DebouncedIn::DebouncedIn(PinName in) 00010 : _in(in) { 00011 00012 // Reset all the flags and counters 00013 _samples = 0; 00014 _output = 0; 00015 _output_last = 0; 00016 _rising_flag = 0; 00017 _falling_flag = 0; 00018 _state_counter = 0; 00019 00020 // Attach ticker 00021 _ticker.attach(this, &DebouncedIn::_sample, 0.005); 00022 } 00023 00024 DebouncedIn::DebouncedIn(PinName in, PinMode mode) 00025 : _in(in, mode) { 00026 00027 // Reset all the flags and counters 00028 _samples = 0; 00029 _output = 0; 00030 _output_last = 0; 00031 _rising_flag = 0; 00032 _falling_flag = 0; 00033 _state_counter = 0; 00034 00035 // Attach ticker 00036 _ticker.attach(this, &DebouncedIn::_sample, 0.005); 00037 } 00038 00039 // Public member functions 00040 00041 int DebouncedIn::read(void) { 00042 return(_output); 00043 } 00044 00045 DebouncedIn::operator int() { 00046 return read(); 00047 } 00048 00049 // return number of rising edges 00050 int DebouncedIn::rising(void) { 00051 int return_value = _rising_flag; 00052 _rising_flag = 0; 00053 return(return_value); 00054 } 00055 00056 // return number of falling edges 00057 int DebouncedIn::falling(void) { 00058 int return_value = _falling_flag; 00059 _falling_flag = 0; 00060 return(return_value); 00061 } 00062 00063 // return number of ticks we've bene steady for 00064 int DebouncedIn::steady(void) { 00065 return(_state_counter); 00066 } 00067 00068 // Private member functions 00069 void DebouncedIn::_sample() { 00070 00071 // take a sample 00072 _samples = _samples >> 1; // shift right 1 bit 00073 00074 if (_in) { 00075 _samples |= 0x80; 00076 } 00077 00078 // examine the sample window, look for steady state 00079 if (_samples == 0x00) { 00080 _output = 0; 00081 } 00082 else if (_samples == 0xFF) { 00083 _output = 1; 00084 } 00085 00086 00087 // Rising edge detection 00088 if ((_output == 1) && (_output_last == 0)) { 00089 _rising_flag++; 00090 _state_counter = 0; 00091 } 00092 00093 // Falling edge detection 00094 else if ((_output == 0) && (_output_last == 1)) { 00095 _falling_flag++; 00096 _state_counter = 0; 00097 } 00098 00099 // steady state 00100 else { 00101 _state_counter++; 00102 } 00103 00104 // update the output 00105 _output_last = _output; 00106 00107 }
Generated on Sun Jul 24 2022 09:39:58 by
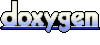