
printing text onto the screen
Embed:
(wiki syntax)
Show/hide line numbers
MAX7456.cpp
00001 #include "mbed.h" 00002 #include "MAX7456.h" 00003 00004 namespace mbed { 00005 00006 00007 00008 MAX7456::MAX7456(PinName mosi, PinName miso, PinName clk, PinName ncs, PinName nrst, const char* name) 00009 : Stream(name), _spi(mosi, miso, clk), _ncs(ncs), _nrst(nrst) { 00010 00011 // initialisation code here 00012 00013 _nrst = 0; 00014 wait (0.5); 00015 _nrst = 1; 00016 wait (0.5); 00017 00018 // Setup the spi for 8 bit data, high steady state clock, 00019 // second edge capture 00020 _spi.format(8,0); 00021 00022 // 1MHz clock 00023 _spi.frequency(1000000); 00024 00025 00026 } 00027 00028 00029 00030 00031 void MAX7456::cls() { 00032 int tmp=0; 00033 tmp = _read(DMM); 00034 tmp &= 0xFB; 00035 _write(DMM,tmp); //Make sure that DMM[2]=0 so that there can be write operations 00036 00037 tmp = _read(DMM); 00038 tmp |= 0x04; 00039 _write (DMM,tmp); //set DMM[2]=1 to clear all locations 00040 00041 // should wait until DMM[2] goes back to zero, so we know the reset it finished 00042 00043 } 00044 00045 00046 void MAX7456::locate(int x, int y) { //not sure if I understand the last line 00047 if ( (x<30) && (y<16) ) { 00048 int add = y*30+x; //formula for converting coordinates into denary location 00049 _write(DMAL,add); 00050 _write(DMAH,add>>8); // what does the ">>" mean? 00051 } 00052 } 00053 00054 00055 00056 int MAX7456::_getc(int character) { 00057 for (int i = 0 ; i < 479 ; i++) { 00058 int location= _read(DMDI,i) 00059 00060 if((location == character - 0x30){ //characters from 1-9 00061 found= "true"; 00062 } 00063 else if(location == character - 0x36){ // characters from A-Z 00064 found = "true"; 00065 } 00066 else if(location == character - 0x3C){ // characters from a-z 00067 found = "true"; 00068 } 00069 else if(location == character + 0x17){ //brackets () 00070 found = "true"; 00071 } 00072 else{ 00073 found = "false"; 00074 00075 if found = "true"{ 00076 return(i); 00077 } 00078 else{ 00079 return("character not found"); 00080 } 00081 } 00082 00083 00084 int MAX7456::_putc(int c) { 00085 00086 if((c >= 0x31)&&(c <= 0x39)) { //characters from 1-9 00087 c= c-0x30; 00088 } 00089 if((c >= 0x41)&&(c <= 0x5A)){ // characters from A-Z 00090 c= c-0x36; 00091 } 00092 if((c >= 0x61)&&(c <= 0x7A)){ // characters from a-z 00093 c= c-0x3C; 00094 } 00095 if((c == 0x28)&&(c == 0x29)){ //brackets () 00096 c= c + 0x17; 00097 } 00098 _write(DMDI,c); 00099 return(c); 00100 } 00101 00102 00103 00104 00105 void MAX7456::vtrim(int v) { 00106 } 00107 00108 void MAX7456::htrim(int h) { 00109 } 00110 00111 void MAX7456::format(char mode){ 00112 if (mode == "P"){ 00113 write(VM0,0x78); // internal sync, OSD enable, PAL 00114 } 00115 } 00116 00117 int MAX7456::_read(int address) { 00118 // force bit 7 to 1 for a read 00119 address |= 0x80; 00120 _ncs=0; // select device 00121 _spi.write(address); // send address 00122 int value = _spi.write(0x00); // send dummy 00123 _ncs=1; //deselect device 00124 return (value); 00125 } 00126 00127 void MAX7456::_write(int address, int data) { 00128 // force bit 7 to 0 for a write 00129 address &= 0x7f; 00130 // select the device 00131 _ncs = 0; 00132 // write VM1 00133 _spi.write(address); // send address 00134 _spi.write(data); // send some data 00135 // Deselect the device 00136 _ncs = 1; 00137 }
Generated on Tue Jul 12 2022 18:24:27 by
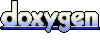