
wifi only 基準コード
Dependencies: RemoteIR TextLCD
main.cpp
00001 #include "mbed.h" 00002 #include "ReceiverIR.h" 00003 #include "rtos.h" 00004 #include <stdint.h> 00005 #include "platform/mbed_thread.h" 00006 #include "TextLCD.h" 00007 RawSerial pc(USBTX, USBRX); 00008 //Serial esp(p28, p27); // tx, rx 00009 00010 RawSerial esp(p13, p14); // tx, rx 00011 // Standard Mbed LED definitions 00012 DigitalOut led1(LED1); 00013 DigitalOut led2(LED2); 00014 DigitalOut led3(LED3); 00015 DigitalOut led4(LED4); 00016 00017 Timer t1; 00018 Timer t2; 00019 00020 struct tm t; 00021 00022 00023 int bufflen, DataRX, count_wifi, getcount, replycount, servreq, timeout; 00024 int bufl, ipdLen, linkID, weberror, webcounter,click_flag; 00025 float temperature, AdcIn, Ht; 00026 float R1=100000, R2=10000; // resistor values to give a 10:1 reduction of measured AnalogIn voltage 00027 char Vcc[10]; 00028 char webcount[8]; 00029 char type[16]; 00030 char type1[16]; 00031 char channel[2]; 00032 char cmdbuff[32]; 00033 char replybuff[1024]; 00034 00035 char webdata[1024]; // This may need to be bigger depending on WEB browser used 00036 char webbuff[4096*4]; // Currently using 1986 characters, Increase this if more web page data added 00037 00038 00039 void SendCMD(),getreply(),ReadWebData(),startserver(),sendpage(),sendpage2(),SendWEB(),sendcheck(),touchuan(); 00040 void wifi(void const *argument); 00041 00042 Thread wifi_thread(wifi, NULL, osPriorityNormal); // decodeIRをスレッド化 :+3 00043 int count_test=1; //test 00044 char battery_ch[8]; 00045 int port =80; // set server port 00046 int SERVtimeout =5; // set server timeout in seconds in case link breaks. 00047 00048 // Serial Interrupt read ESP data 00049 void callback() 00050 { 00051 //pc.printf("\n\r------------ callback is being called --------------\n\r"); 00052 led3=1; 00053 while (esp.readable()) { 00054 webbuff[count_wifi] = esp.getc(); 00055 count_wifi++; 00056 } 00057 if(strlen(webbuff)>bufflen) { 00058 pc.printf("\f\n\r------------ webbuff over bufflen --------------\n\r"); 00059 DataRX=1; 00060 led3=0; 00061 } 00062 } 00063 00064 void wifi(void const *argument){ 00065 pc.baud(115200); 00066 00067 pc.printf("\f\n\r------------ ESP8266 Hardware Reset psq --------------\n\r"); 00068 wait(0.5); 00069 led1=1,led2=0,led3=0; 00070 timeout=6000; 00071 getcount=500; 00072 getreply(); 00073 esp.baud(115200); // ESP8266 baudrate. Maximum on KLxx' is 115200, 230400 works on K20 and K22F 00074 startserver(); 00075 00076 while(1) { 00077 if(DataRX==1) { 00078 pc.printf("\f\n\r------------ main while > if --------------\n\r"); 00079 click_flag = 1; 00080 ReadWebData(); 00081 pc.printf("\f\n\r------------ click_flag=%d --------------\n\r",click_flag); 00082 //if ((servreq == 1 && weberror == 0) && click_flag == 1) { 00083 if (servreq == 1 && weberror == 0) { 00084 pc.printf("\f\n\r------------ befor send page --------------\n\r"); 00085 sendpage(); 00086 } 00087 pc.printf("\f\n\r------------ send_check begin --------------\n\r"); 00088 00089 //sendcheck(); 00090 pc.printf("\f\n\r------------ ssend_check end--------------\n\r"); 00091 00092 esp.attach(&callback); 00093 pc.printf(" IPD Data:\r\n\n Link ID = %d,\r\n IPD Header Length = %d \r\n IPD Type = %s\r\n", linkID, ipdLen, type); 00094 pc.printf("\n\n HTTP Packet: \n\n%s\n", webdata); 00095 pc.printf(" Web Characters sent : %d\n\n", bufl); 00096 pc.printf(" -------------------------------------\n\n"); 00097 servreq=0; 00098 } 00099 } 00100 } 00101 int main() 00102 { 00103 while(1){ 00104 Thread::wait(100); 00105 } 00106 } 00107 // Static WEB page 00108 void sendpage() 00109 { 00110 // WEB page data 00111 00112 strcpy(webbuff, "<!DOCTYPE html>"); 00113 strcat(webbuff, "<html><head><title>RobotCar</title><meta name='viewport' content='width=device-width'/>"); 00114 strcat(webbuff, "</head><body><center><p><strong>Robot Car Remot Controller"); 00115 strcat(webbuff, "</strong></p><td style='vertical-align:top;'><strong>Battery level "); 00116 strcat(webbuff, "<input type=\"text\" id=\"leftms\" size=4 value=250>%</strong>"); 00117 strcat(webbuff, "</td></p><tr ><strong>Now speed : </strong></tr><nobr id=\"speprint\">ready"); 00118 strcat(webbuff, "</nobr><br><tr ><strong>Now action : </strong></tr>"); 00119 strcat(webbuff, "<nobr id=\"funprint\">ready</nobr><table><tr><td></td><td>"); 00120 00121 strcat(webbuff, "<button id='gobtn' type='button' style='width:100px;height:60px' value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00122 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00123 strcat(webbuff, "<button id='leftbtn' type='button' style='width:100px;height:60px' value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00124 strcat(webbuff, "</button></td><td>"); 00125 00126 strcat(webbuff, "<button id='stopbtn' type='button' style='width:100px;height:60px' value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00127 strcat(webbuff, "</button></td><td>"); 00128 strcat(webbuff, "<button id='rightbtn' type='button' style='width:100px;height:60px' value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00129 strcat(webbuff, "</button></td></tr><td></td><td>"); 00130 strcat(webbuff, "<button id='backbtn' type='button' style='width:100px;height:60px' value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00131 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00132 00133 00134 00135 //begin 00136 strcat(webbuff, "<strong>Addition functions:</strong><table>"); 00137 strcat(webbuff, "<td>");//ok 00138 strcat(webbuff, "<button id='avoidbtn' type='button' style='width:100px;height:60px' value=\"AVOIDANCE\" onClick='send_mes_fun(this.id,this.value)' >"); 00139 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00140 strcat(webbuff, "<button id='tracebtn' type='button' style='width:100px;height:60px' value=\"LINETRACE\" onClick='send_mes_fun(this.id,this.value)' >LINE TRACE"); 00141 strcat(webbuff, "</button></td></table><table><td>"); 00142 strcat(webbuff, "</td><strong>Speed level:</strong>"); 00143 strcat(webbuff, "</table><table><td>"); 00144 strcat(webbuff, "<button id='sp1btn' type='button' style='width:100px;height:60px' value=\"SLOW\" onClick='send_mes_spe(this.id,this.value)' >SLOW"); 00145 strcat(webbuff, "</button></td><td>"); 00146 strcat(webbuff, "<button id='sp2btn' type='button' style='width:100px;height:60px' value=\"FAST\" onClick='send_mes_spe(this.id,this.value)' >FAST"); 00147 strcat(webbuff, "</button>"); 00148 strcat(webbuff, "</td>"); 00149 strcat(webbuff, "<td>"); 00150 strcat(webbuff, "<button id='sp3btn' type='button' style='width:100px;height:60px' value=\"VERYFAST\" onClick='send_mes_spe(this.id,this.value)' >FLY"); 00151 strcat(webbuff, "</button>"); 00152 strcat(webbuff, "</td></table>"); 00153 strcat(webbuff, "<table>"); 00154 strcat(webbuff, "<td>"); 00155 strcat(webbuff, "</td>"); 00156 strcat(webbuff, "</table>"); //end 00157 00158 00159 00160 strcat(webbuff, "</center>"); 00161 strcat(webbuff, "</body>"); 00162 strcat(webbuff, "</html>"); 00163 strcat(webbuff, "<script language=\"javascript\" type=\"text/javascript\">"); 00164 strcat(webbuff, "function send_mes(btnmes,btnval){"); 00165 strcat(webbuff, "var url = \"http://\" + window.location.hostname + \"/cargo?a=\" + btnval;"); 00166 strcat(webbuff, "htmlacs(url);"); 00167 strcat(webbuff, "console.log(url);"); 00168 strcat(webbuff, "}"); 00169 00170 strcat(webbuff, "function htmlacs(url) {"); 00171 strcat(webbuff, "var xhr = new XMLHttpRequest();"); 00172 strcat(webbuff, "xhr.open(\"GET\", url ,true);"); 00173 strcat(webbuff, "xhr.send();"); 00174 strcat(webbuff, "}"); 00175 00176 00177 //0824 battery update auto 00178 strcat(webbuff, "function battery_update() {"); 00179 strcat(webbuff, "var url1 = \"http://\" + window.location.hostname+ \"/cargo?a=responseBattery\";"); 00180 strcat(webbuff, "var xhr1 = new XMLHttpRequest();"); 00181 strcat(webbuff, "xhr1.open(\"GET\", url1);"); 00182 //0820 00183 strcat(webbuff, "xhr1.onreadystatechange = function(){"); 00184 //strcat(webbuff, "console.log(\"onready function is being reload!\");"); 00185 strcat(webbuff, "if(this.readyState == 4 && this.status == 200){"); 00186 //strcat(webbuff, "console.log(\"state is being reload!\");"); 00187 strcat(webbuff, "var res1 = xhr1.responseText;"); 00188 strcat(webbuff, "document.getElementById('leftms').value=res1;}};"); 00189 //strcat(webbuff, "console.log(res);}};"); 00190 //0820 00191 00192 strcat(webbuff, "xhr1.send();"); 00193 strcat(webbuff, "}"); 00194 strcat(webbuff, "setInterval(battery_update,10000);"); 00195 00196 //0824 battery update auto 00197 /* 00198 strcat(webbuff, "function send_mes_spe(btnmes,btnval){"); 00199 strcat(webbuff, "var url = \"http://\" + window.location.hostname + \"/cargo?a=\" + btnval;"); 00200 strcat(webbuff, "htmlacs(url);"); 00201 strcat(webbuff, "if(document.getElementById(\"speprint\")){"); 00202 strcat(webbuff, "document.getElementById(\"speprint\").innerHTML=btnval;"); 00203 strcat(webbuff, "}"); 00204 strcat(webbuff, "}"); 00205 strcat(webbuff, "function send_mes_fun(btnmes,btnval){"); 00206 strcat(webbuff, "var url = \"http://\" + window.location.hostname + \"/cargo?a=\" + btnval;"); 00207 strcat(webbuff, "htmlacs(url);"); 00208 strcat(webbuff, "if(document.getElementById(\"funprint\")){"); 00209 strcat(webbuff, "document.getElementById(\"funprint\").innerHTML=btnval;"); 00210 strcat(webbuff, "}"); 00211 strcat(webbuff, "}"); 00212 */ 00213 strcat(webbuff, "</script>"); 00214 // end of WEB page data 00215 bufl = strlen(webbuff); // get total page buffer length 00216 //sprintf(cmdbuff,"AT+CIPSEND=%d,%d\r\n", linkID, bufl); // send IPD link channel and buffer character length. 00217 00218 sprintf(cmdbuff,"AT+CIPSENDBUF=%d,%d\r\n", linkID, (bufl>2048?2048:bufl)); // send IPD link channel and buffer character length. 00219 timeout=500; 00220 getcount=40; 00221 SendCMD(); 00222 getreply(); 00223 pc.printf(replybuff); 00224 pc.printf("\n++++++++++ AT+CIPSENDBUF=%d,%d+++++++++\r\n", linkID, (bufl>2048?2048:bufl)); 00225 00226 pc.printf("\n++++++++++ bufl is %d ++++++++++\r\n",bufl); 00227 00228 //pastthrough mode 00229 SendWEB(); // send web page 00230 pc.printf("\n++++++++++ webbuff clear ++++++++++\r\n"); 00231 00232 memset(webbuff, '\0', sizeof(webbuff)); 00233 sendcheck(); 00234 } 00235 00236 00237 void sendpage2() 00238 { 00239 // WEB page data 00240 00241 //strcpy(webbuff, "<script language=\"javascript\" type=\"text/javascript\">"); 00242 //strcat(webbuff, "document.getElementById('leftms').value=\"100\";"); 00243 count_test++; 00244 sprintf(battery_ch,"%d",count_test); 00245 strcpy(webbuff, battery_ch); 00246 00247 //strcpy(webbuff, "document.getElementById('leftms').value=\"100\";"); 00248 00249 //strcat(webbuff, "</script>"); 00250 // end of WEB page data 00251 bufl = strlen(webbuff); // get total page buffer length 00252 //sprintf(cmdbuff,"AT+CIPSEND=%d,%d\r\n", linkID, bufl); // send IPD link channel and buffer character length. 00253 00254 sprintf(cmdbuff,"AT+CIPSENDBUF=%d,%d\r\n", linkID, (bufl>2048?2048:bufl)); // send IPD link channel and buffer character length. 00255 timeout=500; 00256 getcount=40; 00257 SendCMD(); 00258 getreply(); 00259 pc.printf(replybuff); 00260 pc.printf("\n++++++++++ AT+CIPSENDBUF=%d,%d+++++++++\r\n", linkID, (bufl>2048?2048:bufl)); 00261 00262 pc.printf("\n++++++++++ bufl is %d ++++++++++\r\n",bufl); 00263 00264 //pastthrough mode 00265 SendWEB(); // send web page 00266 pc.printf("\n++++++++++ webbuff clear ++++++++++\r\n"); 00267 00268 memset(webbuff, '\0', sizeof(webbuff)); 00269 sendcheck(); 00270 } 00271 00272 00273 // Reads and processes GET and POST web data 00274 void ReadWebData() 00275 { 00276 pc.printf("+++++++++++++++++Read Web Data+++++++++++++++++++++\r\n"); 00277 wait_ms(200); 00278 esp.attach(NULL); 00279 count_wifi=0; 00280 DataRX=0; 00281 weberror=0; 00282 memset(webdata, '\0', sizeof(webdata)); 00283 int x = strcspn (webbuff,"+"); 00284 if(x) { 00285 strcpy(webdata, webbuff + x); 00286 weberror=0; 00287 int numMatched = sscanf(webdata,"+IPD,%d,%d:%s", &linkID, &ipdLen, type); 00288 //int i=0; 00289 pc.printf("+++++++++++++++++succed rec begin+++++++++++++++++++++\r\n"); 00290 pc.printf("%s",webdata); 00291 pc.printf("+++++++++++++++++succed rec end+++++++++++++++++++++\r\n"); 00292 if( strstr(webdata, "responseBattery") != NULL ) { 00293 click_flag = 0; 00294 led4=!led4; 00295 pc.printf("\r\n++++++++++++++mode = LEFT++++++++++++++\r\n"); 00296 sendpage2(); 00297 /* 00298 strcpy(webbuff, "<script language=\"javascript\" type=\"text/javascript\">"); 00299 strcat(webbuff, "document.getElementById('leftms').value=\"96\";"); 00300 strcat(webbuff, "let request = new XMLHttpRequest();"); 00301 strcat(webbuff, "request.open('GET', url);request.responseType = 'text';"); 00302 strcat(webbuff, "request.onload = function() { poemDisplay.textContent = request.response;};"); 00303 00304 strcat(webbuff, "request.send();"); 00305 strcat(webbuff, "</script>"); 00306 bufl = strlen(webbuff); // get total page buffer length 00307 sprintf(cmdbuff,"AT+CIPSEND=%d,%d\r\n", linkID, bufl); // send IPD link channel and buffer character length. 00308 timeout=500; 00309 getcount=40; 00310 SendCMD(); 00311 getreply(); 00312 SendWEB(); 00313 memset(webbuff, '\0', sizeof(webbuff)); 00314 sendcheck(); 00315 pc.printf("\r\n++++++++++++++mode = LEFT++++++++++++++\r\n"); 00316 */ 00317 00318 /* mode = LEFT; // 左折モード 00319 run = LEFT; // 左折 00320 display(); */ 00321 00322 } 00323 if( strstr(webdata, "LEFT") != NULL ) { 00324 led4=!led4; 00325 pc.printf("mode = RIGHT\r\n"); 00326 00327 } 00328 /* 00329 if( strstr(webdata, "RIGHT") != NULL ) { 00330 led4=!led4; 00331 pc.printf("mode = RIGHT\r\n"); 00332 mode = RIGHT; // 右折モード 00333 run = RIGHT; // 右折 00334 display(); // ディスプレイ表示 00335 } 00336 00337 if( strstr(webdata, "GO") != NULL ) { 00338 led4=!led4; 00339 pc.printf("mode = ADVANCE\r\n"); 00340 mode = ADVANCE; // 前進モード 00341 run = ADVANCE; // 前進 00342 display(); // ディスプレイ表示 00343 } 00344 00345 if( strstr(webdata, "BACK") != NULL ) { 00346 led4=!led4; 00347 pc.printf("mode = BACK\r\n"); 00348 mode = BACK; // 後退モード 00349 run = BACK; // 後退 00350 display(); // ディスプレイ表示 00351 } 00352 00353 if( strstr(webdata, "STOP") != NULL ) { 00354 led4=!led4; 00355 pc.printf("mode = STOP\r\n"); 00356 mode = STOP; // 停止モード 00357 run = STOP; // 停止 00358 display(); // ディスプレイ表示 00359 } 00360 00361 if( strstr(webdata, "AVOIDANCE") != NULL ) { 00362 led4=!led4; 00363 pc.printf("mode = AVOIDANCE\r\n"); 00364 mode=AVOIDANCE; // 障害物回避モード 00365 run = ADVANCE; // 前進 00366 display(); 00367 } 00368 00369 if( strstr(webdata, "LINETRACE") != NULL ) { 00370 led4=!led4; 00371 pc.printf("mode = LINE_TRACE\r\n"); 00372 mode=LINE_TRACE; // ライントレースモード 00373 display(); 00374 } 00375 00376 if( strstr(webdata, "SLOW") != NULL ) { 00377 led4=!led4; 00378 pc.printf("\r\n+++++++++++++++++bbutton right clicked+++++++++++++++++++++\r\n"); 00379 } 00380 00381 if( strstr(webdata, "FAST") != NULL ) { 00382 led4=!led4; 00383 pc.printf("\r\n+++++++++++++++++bbutton right clicked+++++++++++++++++++++\r\n"); 00384 } 00385 00386 if( strstr(webdata, "VERYFAST") != NULL ) { 00387 led4=!led4; 00388 pc.printf("\r\n+++++++++++++++++bbutton right clicked+++++++++++++++++++++\r\n"); 00389 }*/ 00390 sprintf(channel, "%d",linkID); 00391 if (strstr(webdata, "GET") != NULL) { 00392 servreq=1; 00393 pc.printf("\r\n+++++++++++++++++GET+++++++++++++++++++++\r\n"); 00394 00395 } 00396 if (strstr(webdata, "POST") != NULL) { 00397 servreq=1; 00398 pc.printf("\r\n+++++++++++++++++POST+++++++++++++++++++++\r\n"); 00399 } 00400 webcounter++; 00401 sprintf(webcount, "%d",webcounter); 00402 } else { 00403 pc.printf("\n++++++++++ webbuff clear ++++++++++\r\n"); 00404 memset(webbuff, '\0', sizeof(webbuff)); 00405 esp.attach(&callback); 00406 weberror=1; 00407 } 00408 } 00409 // Large WEB buffer data send 00410 void SendWEB() 00411 { 00412 int i=0; 00413 if(esp.writeable()) { 00414 while(webbuff[i]!='\0') { 00415 esp.putc(webbuff[i]); 00416 00417 //**** 00418 //output at command when 2000 00419 if(((i%2047)==0) && (i>0)){ 00420 //wait_ms(10); 00421 sprintf(cmdbuff,"AT+CIPSENDBUF=%d,%d\r\n", linkID, (bufl-2048)>2048?2048:(bufl-2048)); // send IPD link channel and buffer character length. 00422 pc.printf("\r\n++++++++++ AT+CIPSENDBUF=%d,%d ++++++++++\r\n", linkID, (bufl-2048)>2048?2048:(bufl-2048)); 00423 timeout=600; 00424 getcount=50; 00425 SendCMD(); 00426 getreply(); 00427 pc.printf(replybuff); 00428 pc.printf("\r\n+++++++++++++++++++\r\n"); 00429 } 00430 //**** 00431 i++; 00432 pc.printf("%c",webbuff[i]); 00433 } 00434 } 00435 pc.printf("\n++++++++++ send web i= %dinfo ++++++++++\r\n",i); 00436 } 00437 00438 00439 00440 void sendcheck() 00441 { 00442 weberror=1; 00443 timeout=500; 00444 getcount=24; 00445 t2.reset(); 00446 t2.start(); 00447 00448 /* 00449 while(weberror==1 && t2.read() <5) { 00450 getreply(); 00451 if (strstr(replybuff, "SEND OK") != NULL) { 00452 weberror=0; // wait for valid SEND OK 00453 } 00454 } 00455 */ 00456 if(weberror==1) { // restart connection 00457 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); 00458 timeout=500; 00459 getcount=10; 00460 SendCMD(); 00461 getreply(); 00462 pc.printf(replybuff); 00463 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 00464 timeout=500; 00465 getcount=10; 00466 SendCMD(); 00467 getreply(); 00468 pc.printf(replybuff); 00469 } else { 00470 sprintf(cmdbuff, "AT+CIPCLOSE=%s\r\n",channel); // close current connection 00471 SendCMD(); 00472 getreply(); 00473 pc.printf(replybuff); 00474 } 00475 t2.reset(); 00476 } 00477 00478 // Starts and restarts webserver if errors detected. 00479 void startserver() 00480 { 00481 pc.printf("++++++++++ Resetting ESP ++++++++++\r\n"); 00482 strcpy(cmdbuff,"AT+RST\r\n"); 00483 timeout=8000; 00484 getcount=1000; 00485 SendCMD(); 00486 getreply(); 00487 pc.printf(replybuff); 00488 pc.printf("%d",count_wifi); 00489 if (strstr(replybuff, "OK") != NULL) { 00490 pc.printf("\n++++++++++ Starting Server ++++++++++\r\n"); 00491 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); // set multiple connections. 00492 timeout=500; 00493 getcount=20; 00494 SendCMD(); 00495 getreply(); 00496 pc.printf(replybuff); 00497 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 00498 timeout=500; 00499 getcount=20; 00500 SendCMD(); 00501 getreply(); 00502 pc.printf(replybuff); 00503 wait(1); 00504 sprintf(cmdbuff,"AT+CIPSTO=%d\r\n",SERVtimeout); 00505 timeout=500; 00506 getcount=50; 00507 SendCMD(); 00508 getreply(); 00509 pc.printf(replybuff); 00510 //wait(5); 00511 wait(1); 00512 pc.printf("\n Getting Server IP \r\n"); 00513 strcpy(cmdbuff, "AT+CIFSR\r\n"); 00514 timeout=2500; 00515 getcount=200; 00516 while(weberror==0) { 00517 SendCMD(); 00518 getreply(); 00519 if (strstr(replybuff, "0.0.0.0") == NULL) { 00520 weberror=1; // wait for valid IP 00521 } 00522 } 00523 pc.printf("\n Enter WEB address (IP) found below in your browser \r\n\n"); 00524 pc.printf("\n The MAC address is also shown below,if it is needed \r\n\n"); 00525 replybuff[strlen(replybuff)-1] = '\0'; 00526 //char* IP = replybuff + 5; 00527 sprintf(webdata,"%s", replybuff); 00528 pc.printf(webdata); 00529 led2=1; 00530 bufflen=200; 00531 //bufflen=100; 00532 count_wifi=0; 00533 pc.printf("\n\n++++++++++ Ready ++++++++++\r\n\n"); 00534 esp.attach(&callback); 00535 } else { 00536 pc.printf("\n++++++++++ ESP8266 error, check power/connections ++++++++++\r\n"); 00537 led1=1; 00538 led2=1; 00539 led3=1; 00540 led4=1; 00541 while(1) { 00542 led1=!led1; 00543 led2=!led2; 00544 led3=!led3; 00545 led4=!led4; 00546 wait(1); 00547 } 00548 } 00549 t2.reset(); 00550 t2.start(); 00551 } 00552 // ESP Command data send 00553 void SendCMD() 00554 { 00555 esp.printf("%s", cmdbuff); 00556 } 00557 // Get Command and ESP status replies 00558 void getreply() 00559 { 00560 memset(replybuff, '\0', sizeof(replybuff)); 00561 t1.reset(); 00562 t1.start(); 00563 replycount=0; 00564 while(t1.read_ms()< timeout && replycount < getcount) { 00565 if(esp.readable()) { 00566 replybuff[replycount] = esp.getc(); 00567 replycount++; 00568 } 00569 } 00570 t1.stop(); 00571 }
Generated on Sun Jul 17 2022 13:33:53 by
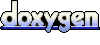