New cellular update
Fork of ublox-at-cellular-interface-ext by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "greentea-client/test_env.h" 00003 #include "unity.h" 00004 #include "utest.h" 00005 #include "UbloxATCellularInterfaceExt.h" 00006 #include "UDPSocket.h" 00007 #ifdef FEATURE_COMMON_PAL 00008 #include "mbed_trace.h" 00009 #define TRACE_GROUP "TEST" 00010 #else 00011 #define tr_debug(format, ...) debug(format "\n", ## __VA_ARGS__) 00012 #define tr_info(format, ...) debug(format "\n", ## __VA_ARGS__) 00013 #define tr_warn(format, ...) debug(format "\n", ## __VA_ARGS__) 00014 #define tr_error(format, ...) debug(format "\n", ## __VA_ARGS__) 00015 #endif 00016 00017 using namespace utest::v1; 00018 00019 // ---------------------------------------------------------------- 00020 // COMPILE-TIME MACROS 00021 // ---------------------------------------------------------------- 00022 00023 // These macros can be overridden with an mbed_app.json file and 00024 // contents of the following form: 00025 // 00026 //{ 00027 // "config": { 00028 // "apn": { 00029 // "value": "\"my_apn\"" 00030 // } 00031 //} 00032 00033 // Whether debug trace is on 00034 #ifndef MBED_CONF_APP_DEBUG_ON 00035 # define MBED_CONF_APP_DEBUG_ON false 00036 #endif 00037 00038 // The credentials of the SIM in the board. 00039 #ifndef MBED_CONF_APP_DEFAULT_PIN 00040 // Note: if PIN is enabled on your SIM, you must define the PIN 00041 // for your SIM jere (e.g. using mbed_app.json to do so). 00042 # define MBED_CONF_APP_DEFAULT_PIN "0000" 00043 #endif 00044 00045 // Network credentials. 00046 #ifndef MBED_CONF_APP_APN 00047 # define MBED_CONF_APP_APN NULL 00048 #endif 00049 #ifndef MBED_CONF_APP_USERNAME 00050 # define MBED_CONF_APP_USERNAME NULL 00051 #endif 00052 #ifndef MBED_CONF_APP_PASSWORD 00053 # define MBED_CONF_APP_PASSWORD NULL 00054 #endif 00055 00056 // The time to wait for a HTTP command to complete 00057 #ifndef MBED_CONF_APP_HTTP_TIMEOUT 00058 #define HTTP_TIMEOUT 10000 00059 #else 00060 #define HTTP_TIMEOUT MBED_CONF_APP_HTTP_TIMEOUT 00061 #endif 00062 00063 // The HTTP echo server, as described in the 00064 // first answer here: 00065 // http://stackoverflow.com/questions/5725430/http-test-server-that-accepts-get-post-calls 00066 // !!! IMPORTANT: this test relies on that server behaving in the same way forever !!! 00067 #define HTTP_ECHO_SERVER "httpbin.org" 00068 00069 // The size of the test file 00070 #define TEST_FILE_SIZE 100 00071 00072 // The maximum number of HTTP profiles 00073 #define MAX_PROFILES 4 00074 00075 // ---------------------------------------------------------------- 00076 // PRIVATE VARIABLES 00077 // ---------------------------------------------------------------- 00078 00079 #ifdef FEATURE_COMMON_PAL 00080 // Lock for debug prints 00081 static Mutex mtx; 00082 #endif 00083 00084 // An instance of the cellular interface 00085 static UbloxATCellularInterfaceExt *pDriver = 00086 new UbloxATCellularInterfaceExt(MDMTXD, MDMRXD, 00087 MBED_CONF_UBLOX_CELL_BAUD_RATE, 00088 MBED_CONF_APP_DEBUG_ON); 00089 // A few buffers for general use 00090 static char buf[2048]; 00091 static char buf1[sizeof(buf)]; 00092 00093 // ---------------------------------------------------------------- 00094 // PRIVATE FUNCTIONS 00095 // ---------------------------------------------------------------- 00096 00097 #ifdef FEATURE_COMMON_PAL 00098 // Locks for debug prints 00099 static void lock() 00100 { 00101 mtx.lock(); 00102 } 00103 00104 static void unlock() 00105 { 00106 mtx.unlock(); 00107 } 00108 #endif 00109 00110 // ---------------------------------------------------------------- 00111 // TESTS 00112 // ---------------------------------------------------------------- 00113 00114 // Test HTTP commands 00115 void test_http_cmd() { 00116 int profile; 00117 char * pData; 00118 00119 TEST_ASSERT(pDriver->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00120 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00121 00122 profile = pDriver->httpAllocProfile(); 00123 TEST_ASSERT(profile >= 0); 00124 00125 pDriver->httpSetTimeout(profile, HTTP_TIMEOUT); 00126 00127 // Set up the server to talk to 00128 TEST_ASSERT(pDriver->httpSetPar(profile, UbloxATCellularInterfaceExt::HTTP_SERVER_NAME, HTTP_ECHO_SERVER)); 00129 00130 // Check HTTP head request 00131 memset(buf, 0, sizeof (buf)); 00132 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_HEAD, 00133 "/headers", 00134 NULL, NULL, 0, NULL, 00135 buf, sizeof (buf)) == NULL); 00136 tr_debug("Received: %s", buf); 00137 TEST_ASSERT(strstr(buf, "Content-Length:") != NULL); 00138 00139 // Check HTTP get request 00140 memset(buf, 0, sizeof (buf)); 00141 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_GET, 00142 "/get", 00143 NULL, NULL, 0, NULL, 00144 buf, sizeof (buf)) == NULL); 00145 tr_debug("Received: %s", buf); 00146 TEST_ASSERT(strstr(buf, "\"http://httpbin.org/get\"") != NULL); 00147 00148 // Check HTTP delete request 00149 memset(buf, 0, sizeof (buf)); 00150 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_DELETE, 00151 "/delete", 00152 NULL, NULL, 0, NULL, 00153 buf, sizeof (buf)) == NULL); 00154 tr_debug("Received: %s", buf); 00155 TEST_ASSERT(strstr(buf, "\"http://httpbin.org/delete\"") != NULL); 00156 00157 // Check HTTP put request (this will fail as the echo server doesn't support it) 00158 memset(buf, 0, sizeof (buf)); 00159 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_PUT, 00160 "/put", 00161 NULL, NULL, 0, NULL, 00162 buf, sizeof (buf)) != NULL); 00163 00164 // Check HTTP post request with data 00165 memset(buf, 0, sizeof (buf)); 00166 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_POST_DATA, 00167 "/post", 00168 NULL, "formData=0123456789", 0, NULL, 00169 buf, sizeof (buf)) == NULL); 00170 tr_debug("Received: %s", buf); 00171 TEST_ASSERT(strstr(buf, "\"http://httpbin.org/post\"") != NULL); 00172 00173 // Check HTTP post request with a file, also checking that writing the response 00174 // to a named file works 00175 for (int x = 0; x < TEST_FILE_SIZE; x++) { 00176 buf[x] = (x % 10) + 0x30; 00177 } 00178 pDriver->delFile("post_test.txt"); 00179 TEST_ASSERT(pDriver->writeFile("post_test.txt", buf, TEST_FILE_SIZE) == TEST_FILE_SIZE); 00180 00181 // This may fail if rsp.txt doesn't happen to be sitting around from a previous run 00182 // so don't check the return value 00183 pDriver->delFile("rsp.txt"); 00184 00185 memset(buf, 0, sizeof (buf)); 00186 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_POST_FILE, 00187 "/post", 00188 "rsp.txt", "post_test.txt", 00189 UbloxATCellularInterfaceExt::HTTP_CONTENT_TEXT, NULL, 00190 buf, sizeof (buf)) == NULL); 00191 tr_debug("Received: %s", buf); 00192 // Find the data in the response and check it 00193 pData = strstr(buf, "\"data\": \""); 00194 TEST_ASSERT(pData != NULL); 00195 pData += 9; 00196 for (int x = 0; x < TEST_FILE_SIZE; x++) { 00197 TEST_ASSERT(*(pData + x) == (x % 10) + 0x30); 00198 } 00199 00200 // Also check that rsp.txt exists and is the same as buf 00201 pDriver->readFile("rsp.txt", buf1, sizeof (buf1)); 00202 memcmp(buf1, buf, sizeof (buf1)); 00203 TEST_ASSERT(pDriver->delFile("rsp.txt")); 00204 TEST_ASSERT(!pDriver->delFile("rsp.txt")); // Should fail 00205 00206 TEST_ASSERT(pDriver->httpFreeProfile(profile)); 00207 TEST_ASSERT(pDriver->disconnect() == 0); 00208 // Wait for printfs to leave the building or the test result string gets messed up 00209 wait_ms(500); 00210 } 00211 00212 // Test HTTP with TLS 00213 void test_http_tls() { 00214 int profile; 00215 SocketAddress address; 00216 00217 TEST_ASSERT(pDriver->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00218 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00219 00220 profile = pDriver->httpAllocProfile(); 00221 TEST_ASSERT(profile >= 0); 00222 00223 pDriver->httpSetTimeout(profile, HTTP_TIMEOUT); 00224 00225 // Set up the server to talk to and TLS, using the IP address this time just for variety 00226 TEST_ASSERT(pDriver->gethostbyname("amazon.com", &address) == 0); 00227 TEST_ASSERT(pDriver->httpSetPar(profile, UbloxATCellularInterfaceExt::HTTP_IP_ADDRESS, address.get_ip_address())); 00228 TEST_ASSERT(pDriver->httpSetPar(profile, UbloxATCellularInterfaceExt::HTTP_SECURE, "1")); 00229 00230 // Check HTTP get request 00231 memset(buf, 0, sizeof (buf)); 00232 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_GET, 00233 "/", 00234 NULL, NULL, 0, NULL, 00235 buf, sizeof (buf)) == NULL); 00236 tr_debug("Received: %s", buf); 00237 // This is what amazon.com returns if TLS is set 00238 TEST_ASSERT(strstr(buf, "503 Service Temporarily Unavailable") != NULL); 00239 00240 // Reset the profile and check that this now fails 00241 TEST_ASSERT(pDriver->httpResetProfile(profile)); 00242 TEST_ASSERT(pDriver->httpSetPar(profile, UbloxATCellularInterfaceExt::HTTP_IP_ADDRESS, address.get_ip_address())); 00243 memset(buf, 0, sizeof (buf)); 00244 TEST_ASSERT(pDriver->httpCommand(profile, UbloxATCellularInterfaceExt::HTTP_GET, 00245 "/", 00246 NULL, NULL, 0, NULL, 00247 buf, sizeof (buf)) == NULL); 00248 tr_debug("Received: %s", buf); 00249 // This is what amazon.com returns if TLS is NOT set 00250 TEST_ASSERT(strstr(buf, "301 Moved Permanently") != NULL); 00251 00252 TEST_ASSERT(pDriver->httpFreeProfile(profile)); 00253 TEST_ASSERT(pDriver->disconnect() == 0); 00254 // Wait for printfs to leave the building or the test result string gets messed up 00255 wait_ms(500); 00256 } 00257 00258 // Allocate max profiles 00259 void test_alloc_profiles() { 00260 int profiles[MAX_PROFILES]; 00261 00262 TEST_ASSERT(pDriver->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00263 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00264 00265 // Allocate first profile and use it 00266 profiles[0] = pDriver->httpAllocProfile(); 00267 TEST_ASSERT(profiles[0] >= 0); 00268 TEST_ASSERT(pDriver->httpSetPar(profiles[0], UbloxATCellularInterfaceExt::HTTP_SERVER_NAME, "raw.githubusercontent.com")); 00269 TEST_ASSERT(pDriver->httpSetPar(profiles[0], UbloxATCellularInterfaceExt::HTTP_SECURE, "1")); 00270 00271 // Check HTTP get request 00272 memset(buf, 0, sizeof (buf)); 00273 TEST_ASSERT(pDriver->httpCommand(profiles[0], UbloxATCellularInterfaceExt::HTTP_GET, 00274 "/u-blox/mbed-os/master/features/cellular/mbed_lib.json", 00275 NULL, NULL, 0, NULL, 00276 buf, sizeof (buf)) == NULL); 00277 tr_debug("Received: %s", buf); 00278 TEST_ASSERT(strstr(buf, "Radio access technology to use. Value in integer: GSM=0, GSM_COMPACT=1, UTRAN=2, EGPRS=3, HSDPA=4, HSUPA=5, HSDPA_HSUPA=6, E_UTRAN=7, CATM1=8 ,NB1=9") != NULL); 00279 00280 // Check that we stop being able to get profiles at the max number 00281 for (int x = 1; x < sizeof (profiles) / sizeof (profiles[0]); x++) { 00282 profiles[x] = pDriver->httpAllocProfile(); 00283 TEST_ASSERT(profiles[0] >= 0); 00284 } 00285 TEST_ASSERT(pDriver->httpAllocProfile() < 0); 00286 00287 // Now use the last one and check that it doesn't affect the first one 00288 TEST_ASSERT(pDriver->httpSetPar(profiles[sizeof (profiles) / sizeof (profiles[0]) - 1], 00289 UbloxATCellularInterfaceExt::HTTP_SERVER_NAME, HTTP_ECHO_SERVER)); 00290 00291 // Check HTTP head request on last profile 00292 memset(buf, 0, sizeof (buf)); 00293 TEST_ASSERT(pDriver->httpCommand(profiles[sizeof (profiles) / sizeof (profiles[0]) - 1], 00294 UbloxATCellularInterfaceExt::HTTP_HEAD, 00295 "/headers", 00296 NULL, NULL, 0, NULL, 00297 buf, sizeof (buf)) == NULL); 00298 tr_debug("Received: %s", buf); 00299 TEST_ASSERT(strstr(buf, "Content-Length:") != NULL); 00300 00301 // Check HTTP get request on first profile once more 00302 memset(buf, 0, sizeof (buf)); 00303 TEST_ASSERT(pDriver->httpCommand(profiles[0], UbloxATCellularInterfaceExt::HTTP_GET, 00304 "/u-blox/mbed-os/master/features/cellular/mbed_lib.json", 00305 NULL, NULL, 0, NULL, 00306 buf, sizeof (buf)) == NULL); 00307 tr_debug("Received: %s", buf); 00308 TEST_ASSERT(strstr(buf, "Radio access technology to use. Value in integer: GSM=0, GSM_COMPACT=1, UTRAN=2, EGPRS=3, HSDPA=4, HSUPA=5, HSDPA_HSUPA=6, E_UTRAN=7, CATM1=8 ,NB1=9") != NULL); 00309 00310 // Free the profiles again 00311 for (int x = 0; x < sizeof (profiles) / sizeof (profiles[0]); x++) { 00312 TEST_ASSERT(pDriver->httpFreeProfile(profiles[x])); 00313 } 00314 00315 TEST_ASSERT(pDriver->disconnect() == 0); 00316 // Wait for printfs to leave the building or the test result string gets messed up 00317 wait_ms(500); 00318 } 00319 00320 // ---------------------------------------------------------------- 00321 // TEST ENVIRONMENT 00322 // ---------------------------------------------------------------- 00323 00324 // Setup the test environment 00325 utest::v1::status_t test_setup(const size_t number_of_cases) { 00326 // Setup Greentea with a timeout 00327 GREENTEA_SETUP(540, "default_auto"); 00328 return verbose_test_setup_handler(number_of_cases); 00329 } 00330 00331 // Test cases 00332 Case cases[] = { 00333 Case("HTTP commands", test_http_cmd), 00334 #ifndef TARGET_UBLOX_C027 00335 // C027 doesn't support TLS 00336 Case("HTTP with TLS", test_http_tls), 00337 #endif 00338 Case("Alloc max profiles", test_alloc_profiles) 00339 }; 00340 00341 Specification specification(test_setup, cases); 00342 00343 // ---------------------------------------------------------------- 00344 // MAIN 00345 // ---------------------------------------------------------------- 00346 00347 int main() { 00348 00349 #ifdef FEATURE_COMMON_PAL 00350 mbed_trace_init(); 00351 00352 mbed_trace_mutex_wait_function_set(lock); 00353 mbed_trace_mutex_release_function_set(unlock); 00354 #endif 00355 00356 // Run tests 00357 return !Harness::run(specification); 00358 } 00359 00360 // End Of File 00361
Generated on Thu Jul 14 2022 10:27:32 by
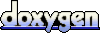