New cellular update
Fork of ublox-at-cellular-interface-ext by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "greentea-client/test_env.h" 00003 #include "unity.h" 00004 #include "utest.h" 00005 #include "UbloxATCellularInterfaceExt.h" 00006 #include "UDPSocket.h" 00007 #ifdef FEATURE_COMMON_PAL 00008 #include "mbed_trace.h" 00009 #define TRACE_GROUP "TEST" 00010 #else 00011 #define tr_debug(format, ...) debug(format "\n", ## __VA_ARGS__) 00012 #define tr_info(format, ...) debug(format "\n", ## __VA_ARGS__) 00013 #define tr_warn(format, ...) debug(format "\n", ## __VA_ARGS__) 00014 #define tr_error(format, ...) debug(format "\n", ## __VA_ARGS__) 00015 #endif 00016 00017 using namespace utest::v1; 00018 00019 // ---------------------------------------------------------------- 00020 // COMPILE-TIME MACROS 00021 // ---------------------------------------------------------------- 00022 00023 // These macros can be overridden with an mbed_app.json file and 00024 // contents of the following form: 00025 // 00026 //{ 00027 // "config": { 00028 // "apn": { 00029 // "value": "\"my_apn\"" 00030 // }, 00031 // "ftp-server": { 00032 // "value": "\"test.rebex.net\"" 00033 // }, 00034 // "ftp-username": { 00035 // "value": "\"demo\"" 00036 // }, 00037 // "ftp-password": { 00038 // "value": "\"password\"" 00039 // }, 00040 // "ftp-use-passive": { 00041 // "value": true 00042 // }, 00043 // "ftp-server-supports-write": { 00044 // "value": false 00045 // }, 00046 // "ftp-filename": { 00047 // "value": "\"readme.txt\"" 00048 // }, 00049 // "ftp-dirname": { 00050 // "value": "\"pub\"" 00051 // } 00052 //} 00053 00054 // Whether debug trace is on 00055 #ifndef MBED_CONF_APP_DEBUG_ON 00056 # define MBED_CONF_APP_DEBUG_ON false 00057 #endif 00058 00059 // The credentials of the SIM in the board. 00060 #ifndef MBED_CONF_APP_DEFAULT_PIN 00061 // Note: if PIN is enabled on your SIM, you must define the PIN 00062 // for your SIM jere (e.g. using mbed_app.json to do so). 00063 # define MBED_CONF_APP_DEFAULT_PIN "0000" 00064 #endif 00065 00066 // Network credentials. 00067 #ifndef MBED_CONF_APP_APN 00068 # define MBED_CONF_APP_APN NULL 00069 #endif 00070 #ifndef MBED_CONF_APP_USERNAME 00071 # define MBED_CONF_APP_USERNAME NULL 00072 #endif 00073 #ifndef MBED_CONF_APP_PASSWORD 00074 # define MBED_CONF_APP_PASSWORD NULL 00075 #endif 00076 00077 // FTP server name 00078 #ifndef MBED_CONF_APP_FTP_SERVER 00079 # error "Must define an FTP server name to run these tests" 00080 #endif 00081 00082 // User name on the FTP server 00083 #ifndef MBED_CONF_APP_FTP_USERNAME 00084 # define MBED_CONF_APP_FTP_SERVER_USERNAME "" 00085 #endif 00086 00087 // Password on the FTP server 00088 #ifndef MBED_CONF_APP_FTP_PASSWORD 00089 # define MBED_CONF_APP_FTP_SERVER_PASSWORD "" 00090 #endif 00091 00092 // Whether to use SFTP or not 00093 #ifndef MBED_CONF_APP_FTP_SECURE 00094 # define MBED_CONF_APP_FTP_SECURE false 00095 #endif 00096 00097 // Port to use on the remote server 00098 #ifndef MBED_CONF_APP_FTP_SERVER_PORT 00099 # if MBED_CONF_APP_FTP_SECURE 00100 # define MBED_CONF_APP_FTP_SERVER_PORT 22 00101 # else 00102 # define MBED_CONF_APP_FTP_SERVER_PORT 21 00103 # endif 00104 #endif 00105 00106 // Whether to use passive or active mode 00107 // default to true as many servers/networks 00108 // require this 00109 #ifndef MBED_CONF_APP_FTP_USE_PASSIVE 00110 # define MBED_CONF_APP_FTP_USE_PASSIVE true 00111 #endif 00112 00113 // Whether the server supports FTP write operations 00114 #ifndef MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00115 # define MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE false 00116 #endif 00117 00118 #if MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00119 // The name of the file to PUT, GET and then delete 00120 # ifndef MBED_CONF_APP_FTP_FILENAME 00121 # define MBED_CONF_APP_FTP_FILENAME "test_file_delete_me" 00122 # endif 00123 // The name of the directory to create, CD to and then remove 00124 // Must not be a substring of MBED_CONF_APP_FTP_FILENAME. 00125 # ifndef MBED_CONF_APP_FTP_DIRNAME 00126 # define MBED_CONF_APP_FTP_DIRNAME "test_dir_delete_me" 00127 # endif 00128 #else 00129 // The name of the file to GET 00130 # ifndef MBED_CONF_APP_FTP_FILENAME 00131 # error "Must define the name of a file you know exists on the FTP server" 00132 # endif 00133 // The name of the directory to CD to 00134 # ifndef MBED_CONF_APP_FTP_DIRNAME 00135 # error "Must define the name of a directory you know exists on the FTP server" 00136 # endif 00137 #endif 00138 00139 // The size of file when testing PUT/GET 00140 #ifndef MBED_CONF_APP_FTP_FILE_SIZE 00141 # define MBED_CONF_APP_FTP_FILE_SIZE 1200 00142 #endif 00143 00144 // ---------------------------------------------------------------- 00145 // PRIVATE VARIABLES 00146 // ---------------------------------------------------------------- 00147 00148 #ifdef FEATURE_COMMON_PAL 00149 // Lock for debug prints 00150 static Mutex mtx; 00151 #endif 00152 00153 // An instance of the cellular interface 00154 static UbloxATCellularInterfaceExt *pDriver = 00155 new UbloxATCellularInterfaceExt(MDMTXD, MDMRXD, 00156 MBED_CONF_UBLOX_CELL_BAUD_RATE, 00157 MBED_CONF_APP_DEBUG_ON); 00158 // A buffer for general use 00159 static char buf[MBED_CONF_APP_FTP_FILE_SIZE]; 00160 00161 // ---------------------------------------------------------------- 00162 // PRIVATE FUNCTIONS 00163 // ---------------------------------------------------------------- 00164 00165 #ifdef FEATURE_COMMON_PAL 00166 // Locks for debug prints 00167 static void lock() 00168 { 00169 mtx.lock(); 00170 } 00171 00172 static void unlock() 00173 { 00174 mtx.unlock(); 00175 } 00176 #endif 00177 00178 // Write a file to the module's file system with known contents 00179 void createFile(const char * filename) { 00180 00181 for (unsigned int x = 0; x < sizeof (buf); x++) { 00182 buf[x] = (char) x; 00183 } 00184 00185 TEST_ASSERT(pDriver->writeFile(filename, buf, sizeof (buf)) == sizeof (buf)); 00186 tr_debug("%d bytes written to file \"%s\"", sizeof (buf), filename); 00187 } 00188 00189 // Read a file back from the module's file system and check the contents 00190 void checkFile(const char * filename) { 00191 memset(buf, 0, sizeof (buf)); 00192 00193 int x = pDriver->readFile(filename, buf, sizeof (buf)); 00194 tr_debug ("File is %d bytes big", x); 00195 TEST_ASSERT(x == sizeof (buf)); 00196 00197 tr_debug("%d bytes read from file \"%s\"", sizeof (buf), filename); 00198 00199 for (unsigned int x = 0; x < sizeof (buf); x++) { 00200 TEST_ASSERT(buf[x] == (char) x); 00201 } 00202 } 00203 00204 // ---------------------------------------------------------------- 00205 // TESTS 00206 // ---------------------------------------------------------------- 00207 00208 // Test the setting up of parameters, connection and login to an FTP session 00209 void test_ftp_login() { 00210 SocketAddress address; 00211 char portString[10]; 00212 00213 sprintf(portString, "%d", MBED_CONF_APP_FTP_SERVER_PORT); 00214 00215 TEST_ASSERT(pDriver->init(MBED_CONF_APP_DEFAULT_PIN)); 00216 00217 // Reset parameters to default to begin with 00218 pDriver->ftpResetPar(); 00219 00220 // Set a timeout for FTP commands 00221 TEST_ASSERT(pDriver->ftpSetTimeout(60000)); 00222 00223 // Set up the FTP server parameters 00224 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_SERVER_NAME, 00225 MBED_CONF_APP_FTP_SERVER)); 00226 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_SERVER_PORT, 00227 portString)); 00228 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_USER_NAME, 00229 MBED_CONF_APP_FTP_USERNAME)); 00230 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_PASSWORD, 00231 MBED_CONF_APP_FTP_PASSWORD)); 00232 #ifdef MBED_CONF_APP_FTP_ACCOUNT 00233 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_ACCOUNT, 00234 MBED_CONF_APP_FTP_ACCOUNT)); 00235 #endif 00236 #if MBED_CONF_APP_FTP_SECURE 00237 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_SECURE, "1")); 00238 #endif 00239 #if MBED_CONF_APP_FTP_USE_PASSIVE 00240 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_MODE, "1")); 00241 #endif 00242 00243 // Now connect to the network 00244 TEST_ASSERT(pDriver->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00245 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00246 00247 // Get the server IP address, purely to make sure it's there 00248 TEST_ASSERT(pDriver->gethostbyname(MBED_CONF_APP_FTP_SERVER, &address) == 0); 00249 tr_debug ("Using FTP \"%s\", which is at %s", MBED_CONF_APP_FTP_SERVER, 00250 address.get_ip_address()); 00251 00252 // Log into the FTP server 00253 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LOGIN) == NULL); 00254 } 00255 00256 // Test FTP directory listing 00257 void test_ftp_dir() { 00258 // Get a directory listing 00259 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00260 NULL, NULL, buf, sizeof (buf)) == NULL); 00261 tr_debug("Listing:\n%s", buf); 00262 00263 // The file we will GET should appear in the directory listing 00264 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME) > NULL); 00265 // As should the directory name we will change to 00266 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) > NULL); 00267 } 00268 00269 // Test FTP file information 00270 void test_ftp_fileinfo() { 00271 // Get the info 00272 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_FILE_INFO, 00273 MBED_CONF_APP_FTP_FILENAME, NULL, 00274 buf, sizeof (buf)) == NULL); 00275 tr_debug("File info:\n%s", buf); 00276 00277 // The file info string should at least include the file name 00278 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME) > NULL); 00279 } 00280 00281 #if MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00282 00283 // In case a previous test failed half way, do some cleaning up first 00284 // Note: don't check return values as these operations will fail 00285 // if there's nothing to clean up 00286 void test_ftp_write_cleanup() { 00287 pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_DELETE_FILE, 00288 MBED_CONF_APP_FTP_FILENAME); 00289 pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_DELETE_FILE, 00290 MBED_CONF_APP_FTP_FILENAME "_2"); 00291 pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_RMDIR, 00292 MBED_CONF_APP_FTP_DIRNAME); 00293 pDriver->delFile(MBED_CONF_APP_FTP_FILENAME); 00294 pDriver->delFile(MBED_CONF_APP_FTP_FILENAME "_1"); 00295 } 00296 00297 // Test FTP put and then get 00298 void test_ftp_put_get() { 00299 // Create the file 00300 createFile(MBED_CONF_APP_FTP_FILENAME); 00301 00302 // Put the file 00303 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_PUT_FILE, 00304 MBED_CONF_APP_FTP_FILENAME) == NULL); 00305 00306 // Get the file 00307 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_GET_FILE, 00308 MBED_CONF_APP_FTP_FILENAME, 00309 MBED_CONF_APP_FTP_FILENAME "_1") == NULL); 00310 00311 // Check that it is the same as we sent 00312 checkFile(MBED_CONF_APP_FTP_FILENAME "_1"); 00313 } 00314 00315 // Test FTP rename file 00316 void test_ftp_rename() { 00317 // Get a directory listing 00318 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00319 NULL, NULL, buf, sizeof (buf)) == NULL); 00320 tr_debug("Listing:\n%s", buf); 00321 00322 // The file we are renaming to should not appear 00323 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00324 00325 // Rename the file 00326 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_RENAME_FILE, 00327 MBED_CONF_APP_FTP_FILENAME, 00328 MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00329 00330 // Get a directory listing 00331 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00332 NULL, NULL, buf, sizeof (buf)) == NULL); 00333 tr_debug("Listing:\n%s", buf); 00334 00335 // The new file should now exist 00336 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") > NULL); 00337 00338 } 00339 00340 // Test FTP delete file 00341 void test_ftp_delete() { 00342 // Get a directory listing 00343 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00344 NULL, NULL, buf, sizeof (buf)) == NULL); 00345 tr_debug("Listing:\n%s", buf); 00346 00347 // The file we are to delete should appear in the list 00348 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") > NULL); 00349 00350 // Delete the file 00351 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_DELETE_FILE, 00352 MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00353 00354 // Get a directory listing 00355 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00356 NULL, NULL, buf, sizeof (buf)) == NULL); 00357 tr_debug("Listing:\n%s", buf); 00358 00359 // The file we deleted should no longer appear in the list 00360 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00361 } 00362 00363 // Test FTP MKDIR 00364 void test_ftp_mkdir() { 00365 // Get a directory listing 00366 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00367 NULL, NULL, buf, sizeof (buf)) == NULL); 00368 tr_debug("Listing:\n%s", buf); 00369 00370 // The directory we are to create should not appear in the list 00371 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) == NULL); 00372 00373 // Create the directory 00374 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_MKDIR, 00375 MBED_CONF_APP_FTP_DIRNAME) == NULL); 00376 00377 // Get a directory listing 00378 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00379 NULL, NULL, buf, sizeof (buf)) == NULL); 00380 tr_debug("Listing:\n%s", buf); 00381 00382 // The directory we created should now appear in the list 00383 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) > NULL); 00384 } 00385 00386 // Test FTP RMDIR 00387 void test_ftp_rmdir() { 00388 // Get a directory listing 00389 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00390 NULL, NULL, buf, sizeof (buf)) == NULL); 00391 tr_debug("Listing:\n%s", buf); 00392 00393 // The directory we are to remove should appear in the list 00394 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) > NULL); 00395 00396 // Remove the directory 00397 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_RMDIR, 00398 MBED_CONF_APP_FTP_DIRNAME) == NULL); 00399 00400 // Get a directory listing 00401 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00402 NULL, NULL, buf, sizeof (buf)) == NULL); 00403 tr_debug("Listing:\n%s", buf); 00404 00405 // The directory we removed should no longer appear in the list 00406 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) == NULL); 00407 } 00408 00409 #endif // MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00410 00411 // Test FTP get 00412 void test_ftp_get() { 00413 // Make sure that the 'get' filename we're going to use 00414 // isn't already here (but don't assert on this one 00415 // as, if the file isn't there, we will get an error) 00416 pDriver->delFile(MBED_CONF_APP_FTP_FILENAME); 00417 00418 // Get the file 00419 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_GET_FILE, 00420 MBED_CONF_APP_FTP_FILENAME) == NULL); 00421 00422 // Check that it has arrived 00423 TEST_ASSERT(pDriver->fileSize(MBED_CONF_APP_FTP_FILENAME) > 0); 00424 } 00425 00426 // Test FTP change directory 00427 void test_ftp_cd() { 00428 // Get a directory listing 00429 *buf = 0; 00430 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00431 NULL, NULL, buf, sizeof (buf)) == NULL); 00432 00433 tr_debug("Listing:\n%s", buf); 00434 00435 // The listing should include the directory name we are going to move to 00436 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) > NULL); 00437 00438 // Change directories 00439 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_CD, 00440 MBED_CONF_APP_FTP_DIRNAME) == NULL); 00441 // Get a directory listing 00442 *buf = 0; 00443 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00444 NULL, NULL, buf, sizeof (buf)) == NULL); 00445 tr_debug("Listing:\n%s", buf); 00446 00447 // The listing should no longer include the directory name we have moved 00448 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) == NULL); 00449 00450 // Go back to where we were 00451 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_CD, "..") 00452 == NULL); 00453 00454 // Get a directory listing 00455 *buf = 0; 00456 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00457 NULL, NULL, buf, sizeof (buf)) == NULL); 00458 tr_debug("Listing:\n%s", buf); 00459 00460 // The listing should include the directory name we went to once more 00461 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) > NULL); 00462 } 00463 00464 #ifdef MBED_CONF_APP_FTP_FOTA_FILENAME 00465 // Test FTP FOTA 00466 // TODO: test not tested as I don't have a module that supports the FTP FOTA operation 00467 void test_ftp_fota() { 00468 *buf = 0; 00469 // Do FOTA on a file 00470 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_FOTA_FILE, 00471 MBED_CONF_APP_FTP_FOTA_FILENAME, NULL, 00472 buf, sizeof (buf)) == NULL); 00473 tr_debug("MD5 sum: %s\n", buf); 00474 00475 // Check that the 128 bit MD5 sum is now there 00476 TEST_ASSERT(strlen (buf) == 32); 00477 } 00478 #endif 00479 00480 // Test logout and disconnect from an FTP session 00481 void test_ftp_logout() { 00482 // Log out from the FTP server 00483 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LOGOUT) == NULL); 00484 00485 TEST_ASSERT(pDriver->disconnect() == 0); 00486 00487 // Wait for printfs to leave the building or the test result string gets messed up 00488 wait_ms(500); 00489 } 00490 00491 // ---------------------------------------------------------------- 00492 // TEST ENVIRONMENT 00493 // ---------------------------------------------------------------- 00494 00495 // Setup the test environment 00496 utest::v1::status_t test_setup(const size_t number_of_cases) { 00497 // Setup Greentea with a timeout 00498 GREENTEA_SETUP(540, "default_auto"); 00499 return verbose_test_setup_handler(number_of_cases); 00500 } 00501 00502 // Test cases 00503 Case cases[] = { 00504 Case("FTP log in", test_ftp_login), 00505 #if MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00506 Case("Clean-up", test_ftp_write_cleanup), 00507 Case("FTP put and get", test_ftp_put_get), 00508 Case("FTP file info", test_ftp_fileinfo), 00509 Case("FTP rename", test_ftp_rename), 00510 Case("FTP make directory", test_ftp_mkdir), 00511 Case("FTP directory list", test_ftp_dir), Case("FTP delete", test_ftp_delete), 00512 Case("FTP change directory", test_ftp_cd), 00513 Case("FTP delete directory", test_ftp_rmdir), 00514 #else 00515 Case("FTP directory list", test_ftp_dir), 00516 Case("FTP file info", test_ftp_fileinfo), 00517 Case("FTP get", test_ftp_get), 00518 Case("FTP change directory", test_ftp_cd), 00519 #endif 00520 #ifdef MBED_CONF_APP_FTP_FOTA_FILENAME 00521 Case("FTP FOTA", test_ftp_fota), 00522 #endif 00523 Case("FTP log out", test_ftp_logout) 00524 }; 00525 00526 Specification specification(test_setup, cases); 00527 00528 // ---------------------------------------------------------------- 00529 // MAIN 00530 // ---------------------------------------------------------------- 00531 00532 int main() { 00533 00534 #ifdef FEATURE_COMMON_PAL 00535 mbed_trace_init(); 00536 00537 mbed_trace_mutex_wait_function_set(lock); 00538 mbed_trace_mutex_release_function_set(unlock); 00539 #endif 00540 00541 // Run tests 00542 return !Harness::run(specification); 00543 } 00544 00545 // End Of File 00546
Generated on Thu Jul 14 2022 10:27:32 by
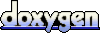