
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
main.cpp
00001 /* 00002 * main.cpp 00003 * Laos Controller, main function 00004 * 00005 * Copyright (c) 2011 Peter Brier & Jaap Vermaas 00006 * 00007 * This file is part of the LaOS project (see: http://wiki.laoslaser.org) 00008 * 00009 * LaOS is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * LaOS is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00021 * 00022 * This program consists of a few parts: 00023 * 00024 * ConfigFile Read configuration files 00025 * EthConfig Initialize an ethernet stack, based on a configuration file (includes link status monitoring) 00026 * LaosDisplay User interface functions (read keys, display text and menus on LCD) 00027 * LaosMenu User interface stuctures (menu navigation) 00028 * LaosServer TCP/IP server, accept connections read/write data 00029 * LaosMotion Motion control functions (X,Y,Z motion, homing) 00030 * LaosIO Input/Output functions 00031 * LaosFile File based jobs (read/write/delete) 00032 * 00033 * Program functions: 00034 * 1) Read config file 00035 * 2) Enable TCP/IP stack (Fixed ip or DHCP) 00036 * 3) Instantiate tcp/ip port and accept connections 00037 * 4) Show menus, peform user actions 00038 * 5) Controll laser 00039 * 6) Controll motion 00040 * 7) Set and read IO, check status (e.g. interlocks) 00041 * 00042 */ 00043 #include "global.h" 00044 #include "ConfigFile.h" 00045 #include "EthConfig.h" 00046 #include "TFTPServer.h" 00047 #include "LaosMenu.h" 00048 #include "LaosMotion.h" 00049 #include "SDFileSystem.h" 00050 #include "laosfilesystem.h" 00051 00052 // MBED blue status leds 00053 DigitalOut led1(LED1); 00054 DigitalOut led2(LED2); 00055 DigitalOut led3(LED3); 00056 DigitalOut led4(LED4); 00057 00058 // Status and communication 00059 DigitalOut eth_link(p29); // green 00060 DigitalOut eth_speed(p30); // yellow 00061 EthernetNetIf *eth; // Ethernet, tcp/ip 00062 00063 // Filesystems 00064 LocalFileSystem local("local"); //File System 00065 LaosFileSystem sd(p11, p12, p13, p14, "sd"); 00066 00067 // Laos objects 00068 LaosDisplay *dsp; 00069 LaosMenu *mnu; 00070 TFTPServer *srv; 00071 LaosMotion *mot; 00072 Timer systime; 00073 00074 // Config 00075 GlobalConfig *cfg; 00076 00077 // Protos 00078 void GetFile(void); 00079 void main_nodisplay(); 00080 void main_menu(); 00081 00082 // for debugging: 00083 extern void plan_get_current_position_xyz(float *x, float *y, float *z); 00084 extern PwmOut pwm; 00085 extern "C" void mbed_reset(); 00086 00087 /** 00088 *** Main function 00089 **/ 00090 int main() 00091 { 00092 systime.start(); 00093 //float x, y, z; 00094 eth_speed = 1; 00095 00096 dsp = new LaosDisplay(); 00097 printf( VERSION_STRING "...\nBOOT...\n" ); 00098 mnu = new LaosMenu(dsp); 00099 eth_speed=0; 00100 00101 printf("TEST SD...\n"); 00102 FILE *fp = sd.openfile("test.txt", "wb"); 00103 if ( fp == NULL ) 00104 { 00105 mnu->SetScreen("SD NOT READY!"); 00106 wait(2.0); 00107 mbed_reset(); 00108 } 00109 else 00110 { 00111 printf("SD: READY...\n"); 00112 fclose(fp); 00113 removefile("test.txt"); 00114 } 00115 00116 // See if there's a .bin file on the SD 00117 // if so, put it on the MBED and reboot 00118 if (SDcheckFirmware()) mbed_reset(); 00119 00120 mnu->SetScreen(VERSION_STRING); 00121 printf("START...\n"); 00122 cfg = new GlobalConfig("config.txt"); 00123 mnu->SetScreen("CONFIG OK...."); 00124 printf("CONFIG OK...\n"); 00125 if (!cfg->nodisplay) 00126 dsp->testI2C(); 00127 00128 printf("MOTION...\n"); 00129 mot = new LaosMotion(); 00130 00131 eth = EthConfig(); 00132 eth_speed=1; 00133 00134 printf("SERVER...\n"); 00135 srv = new TFTPServer("/sd", cfg->port); 00136 mnu->SetScreen("SERVER OK...."); 00137 wait(0.5); 00138 mnu->SetScreen(9); // IP 00139 wait(1.0); 00140 00141 printf("RUN...\n"); 00142 00143 // Wait for key, and then home 00144 00145 if ( cfg->autohome ) 00146 { 00147 printf("HOME...\n"); 00148 wait(1); 00149 00150 mnu->SetScreen("HOME...."); 00151 00152 // Start homing 00153 while ( !mot->isStart() ); 00154 mot->home(cfg->xhome,cfg->yhome, cfg->zhome); 00155 // if ( !mot->isHome ) exit(1); 00156 printf("HOME DONE. (%d,%d, %d)\n",cfg->xhome,cfg->yhome,cfg->zhome); 00157 } 00158 else 00159 printf("Homing skipped: %d\n", cfg->autohome); 00160 00161 // clean sd card? 00162 if (cfg->cleandir) cleandir(); 00163 mnu->SetScreen(NULL); 00164 00165 if (cfg->nodisplay) { 00166 printf("No display set\n\r"); 00167 main_nodisplay(); 00168 } else { 00169 printf("Entering display\n\r"); 00170 main_menu(); 00171 } 00172 } 00173 00174 void main_nodisplay() { 00175 float x, y, z = 0; 00176 00177 // main loop 00178 while(1) 00179 { 00180 led1=led2=led3=led4=0; 00181 mnu->SetScreen("Wait for file ..."); 00182 while (srv->State() == listen) 00183 Net::poll(); 00184 GetFile(); 00185 00186 plan_get_current_position_xyz(&x, &y, &z); 00187 printf("%f %f\n", x,y); 00188 mnu->SetScreen("Laser BUSY..."); 00189 00190 char name[32]; 00191 srv->getFilename(name); 00192 printf("Now processing file: '%s'\n\r", name); 00193 FILE *in = sd.openfile(name, "r"); 00194 while (!feof(in)) 00195 { 00196 while (!mot->ready() ); 00197 mot->write(readint(in)); 00198 } 00199 fclose(in); 00200 removefile(name); 00201 // done 00202 printf("DONE!...\n"); 00203 mot->moveTo(cfg->xrest, cfg->yrest, cfg->zrest); 00204 } 00205 } 00206 00207 00208 void main_menu() { 00209 // main loop 00210 while (1) { 00211 led1=led2=led3=led4=0; 00212 00213 mnu->SetScreen(1); 00214 while (1) {; 00215 mnu->Handle(); 00216 Net::poll(); 00217 if (srv->State() != listen) { 00218 GetFile(); 00219 char myname[32]; 00220 srv->getFilename(myname); 00221 if (isFirmware(myname)) { 00222 installFirmware(myname); 00223 mnu->SetScreen(1); 00224 } else { 00225 if (strcmp("config.txt", myname) == 0) { 00226 // it's a config file! 00227 mnu->SetScreen(1); 00228 } else { 00229 if (isLaosFile(myname)) { 00230 mnu->SetFileName(myname); 00231 mnu->SetScreen(2); 00232 } 00233 } 00234 } 00235 } 00236 } 00237 } 00238 } 00239 00240 /** 00241 *** Get file from network and save on SDcard 00242 *** Ascii data is read from the network, and saved on the SD card in binary int32 format 00243 **/ 00244 void GetFile(void) { 00245 Timer t; 00246 printf("Main::GetFile()\n\r" ); 00247 mnu->SetScreen("Receive file..."); 00248 t.start(); 00249 while (srv->State() != listen) { 00250 Net::poll(); 00251 switch ((int)t.read()) { 00252 case 1: 00253 mnu->SetScreen("Receive file"); 00254 break; 00255 case 2: 00256 mnu->SetScreen("Receive file."); 00257 break; 00258 case 3: 00259 mnu->SetScreen("Receive file.."); 00260 break; 00261 case 4: 00262 mnu->SetScreen("Receive file..."); 00263 t.reset(); 00264 break; 00265 } 00266 } 00267 mnu->SetScreen("Received file."); 00268 } // GetFile 00269
Generated on Tue Jul 12 2022 21:03:03 by
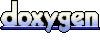