
This is the firmware for the LaOS - Laser Open Source project. You can use it to drive a laser cutter. For hardware and more information, look at our wiki: http://wiki.laoslaser.org
Dependencies: EthernetNetIf mbed
LaosMenu.cpp
00001 /* 00002 * LaosMenu.cpp 00003 * Menu structure and user interface. Uses LaosDisplay 00004 * 00005 * Copyright (c) 2011 Peter Brier & Jaap Vermaas 00006 * 00007 * This file is part of the LaOS project (see: http://wiki.laoslaser.org/) 00008 * 00009 * LaOS is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * LaOS is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00021 * 00022 */ 00023 #include "LaosMenu.h" 00024 00025 static const char *menus[] = { 00026 "STARTUP", //0 00027 "MAIN", //1 00028 "START JOB", //2 00029 "DELETE JOB", //3 00030 "HOME", //4 00031 "MOVE", //5 00032 "FOCUS", //6 00033 "ORIGIN", //7 00034 "REMOVE ALL JOBS", //8 00035 "IP", //9 00036 "REBOOT", //10 00037 // "POWER / SPEED",//11 00038 // "IO", //12 00039 }; 00040 00041 static const char *screens[] = { 00042 //0: main, navigate to MOVE, FOCUS, HOME, ORIGIN, START JOB, IP, 00043 // DELETE JOB, POWER 00044 #define STARTUP (0) 00045 "$$$$$$$$$$$$$$$$" 00046 "$$$$$$$$$$$$$$$$", 00047 00048 #define MAIN (STARTUP+1) 00049 "$$$$$$$$$$$$$$$$" 00050 "<----- 10 ----->", 00051 00052 #define RUN (MAIN+1) 00053 "RUN: " 00054 "$$$$$$$$$$$$$$$$", 00055 00056 #define DELETE (RUN+1) 00057 "DELETE: " 00058 "$$$$$$$$$$$$$$$$", 00059 00060 #define HOME (DELETE+1) 00061 "HOME? " 00062 " [ok] ", 00063 00064 #define MOVE (HOME+1) 00065 "X: +6543210 MOVE" 00066 "Y: +6543210 ", 00067 00068 #define FOCUS (MOVE+1) 00069 "Z: +543210 FOCUS" 00070 " ", 00071 00072 #define ORIGIN (FOCUS+1) 00073 " SET ORIGIN? " 00074 " [ok] ", 00075 00076 #define DELETE_ALL (ORIGIN+1) 00077 "DELETE ALL FILES" 00078 " [ok] ", 00079 00080 #define IP (DELETE_ALL+1) 00081 "210.210.210.210 " 00082 "$$$$$$$$[ok] ", 00083 00084 #define REBOOT (IP+1) 00085 "REBOOTING... " 00086 "Please wait... ", 00087 00088 #define POWER (REBOOT+1) 00089 "$$$$$$$: 6543210" 00090 " [ok] ", 00091 00092 #define IO (POWER+1) 00093 "$$$$$$$$$$$=0 IO" 00094 " [ok] ", 00095 00096 // Intermediate screens 00097 #define DELETE_OK (IO+1) 00098 "DELETE 10? " 00099 " [ok] ", 00100 00101 #define HOMING (DELETE_OK+1) 00102 "HOMING... " 00103 " ", 00104 00105 #define RUNNING (HOMING+1) 00106 "RUNNING... 10%" 00107 "[cancel] ", 00108 00109 #define BUSY (RUNNING+1) 00110 "BUSY: $$$$$$$$$$" 00111 "[cancel][ok] ", 00112 00113 #define PAUSE (BUSY+1) 00114 "PAUSE: $$$$$$$$$" 00115 "[cancel][ok] ", 00116 00117 }; 00118 00119 static const char *ipfields[] = { "IP", "NETMASK", "GATEWAY", "DNS" }; 00120 //static const char *powerfields[] = { "Pmin %", "Pmax %", "Voff", "Von" }; 00121 //static const char *iofields[] = { "o1:PURGE", "o2:EXHAUST", "o3:PUMP", "i1:COVER", "i2:PUMPOK", "i3:LASEROK", "i4:PURGEOK" }; 00122 00123 00124 /** 00125 *** Make new menu object 00126 **/ 00127 LaosMenu::LaosMenu(LaosDisplay *display) { 00128 waitup=timeout=iofield=ipfield=0; 00129 sarg = NULL; 00130 x=y=z=0; 00131 xoff=yoff=zoff=0; 00132 screen=prevscreen=lastscreen=speed=0; 00133 menu=1; 00134 strcpy(jobname, ""); 00135 dsp = display; 00136 if ( dsp == NULL ) dsp = new LaosDisplay(); 00137 dsp->cls(); 00138 SetScreen(NULL); 00139 runfile = NULL; 00140 } 00141 00142 /** 00143 *** Destroy menu object 00144 **/ 00145 LaosMenu::~LaosMenu() { 00146 } 00147 00148 /** 00149 *** Goto specific screen 00150 **/ 00151 void LaosMenu::SetScreen(int screen) { 00152 sarg = NULL; 00153 this->screen = screen; 00154 Handle(); 00155 Handle(); 00156 Handle(); 00157 } 00158 00159 /** 00160 *** Goto specific screen 00161 **/ 00162 void LaosMenu::SetScreen(char *msg) { 00163 if ( msg == NULL ) { 00164 sarg = NULL; 00165 screen = MAIN; 00166 } else if ( msg[0] == 0 ) { 00167 screen = MAIN; 00168 } else { 00169 sarg = msg; 00170 screen = STARTUP; 00171 } 00172 prevscreen = -1; // force update 00173 Handle(); 00174 Handle(); 00175 Handle(); 00176 } 00177 00178 /** 00179 *** Handle menu system 00180 *** Read keys, and plan next action on the screen, output screen if 00181 *** something changed 00182 **/ 00183 void LaosMenu::Handle() { 00184 int xt, yt, zt, cnt=0, nodisplay = 0; 00185 extern LaosFileSystem sd; 00186 extern LaosMotion *mot; 00187 static int count=0; 00188 00189 int c = dsp->read(); 00190 if ( count++ > 10) count = 0; // screen refresh counter (refresh once every 10 cycles( 00191 00192 if ( c ) timeout = 10; // keypress timeout counter 00193 else if ( timeout ) timeout--; 00194 00195 if ( screen != prevscreen ) waitup = 1; // after a screen change: wait for a key release, mask current keypress 00196 if ( waitup && timeout) // if we have to wait for key-up, 00197 c = 0; // cancel the keypress 00198 if ( waitup && !timeout ) waitup=0; 00199 00200 if ( !timeout ) // increase speed if we keep button pressed longer 00201 speed = 5; 00202 else { 00203 speed *= 1.5; 00204 if ( speed >= 100 ) speed = 100; 00205 } 00206 00207 if ( c || screen != prevscreen || count >9 ) { 00208 00209 switch ( screen ) { 00210 case STARTUP: 00211 if ( sarg == NULL ) sarg = (char*) VERSION_STRING; 00212 break; 00213 case MAIN: 00214 switch ( c ) { 00215 case K_RIGHT: menu+=1; waitup=1; break; 00216 case K_LEFT: menu-=1; waitup=1; break; 00217 case K_UP: lastscreen=MAIN; screen=MOVE; menu=MAIN; break; 00218 case K_DOWN: lastscreen=MAIN; screen=MOVE; menu=MAIN; break; 00219 case K_OK: screen=menu; waitup=1; lastscreen=MAIN; break; 00220 case K_CANCEL: menu=MAIN; break; 00221 case K_FUP: lastscreen=MAIN; screen=FOCUS; menu=MAIN; break; 00222 case K_FDOWN: lastscreen=MAIN; screen=FOCUS; menu=MAIN; break; 00223 case K_ORIGIN: lastscreen=MAIN; screen=ORIGIN; waitup=1; break; 00224 } 00225 if (menu==0) menu = (sizeof(menus) / sizeof(menus[0])) -1; 00226 if (menu==(sizeof(menus) / sizeof(menus[0]))) menu = 1; 00227 sarg = (char*)menus[menu]; 00228 args[0] = menu; 00229 break; 00230 00231 case RUN: // START JOB select job to run 00232 if (strlen(jobname) == 0) getprevjob(jobname); 00233 switch ( c ) { 00234 case K_OK: screen=RUNNING; break; 00235 case K_UP: case K_FUP: getprevjob(jobname); waitup = 1; break; // next job 00236 case K_RIGHT: screen=DELETE; waitup=1; break; 00237 case K_DOWN: case K_FDOWN: getnextjob(jobname); waitup = 1; break;// prev job 00238 case K_CANCEL: screen=1; waitup = 1; break; 00239 } 00240 sarg = (char *)&jobname; 00241 break; 00242 00243 case DELETE: // DELETE JOB select job to run 00244 switch ( c ) { 00245 case K_OK: removefile(jobname); screen=lastscreen; waitup = 1; 00246 break; // INSERT: delete current job 00247 case K_UP: case K_FUP: getprevjob(jobname); waitup = 1; break; // next job 00248 case K_DOWN: case K_FDOWN: getnextjob(jobname); waitup = 1; break;// prev job 00249 case K_LEFT: screen=RUN; waitup=1; break; 00250 case K_CANCEL: screen=lastscreen; waitup = 1; break; 00251 } 00252 sarg = (char *)&jobname; 00253 break; 00254 00255 case MOVE: // pos xy 00256 mot->getPosition(&x, &y, &z); 00257 xt = x; yt= y; 00258 switch ( c ) { 00259 case K_DOWN: y+=1000*speed; break; 00260 case K_UP: y-=1000*speed; break; 00261 case K_LEFT: x-=1000*speed; break; 00262 case K_RIGHT: x+=1000*speed; break; 00263 case K_OK: case K_CANCEL: screen=MAIN; waitup=1; break; 00264 case K_FUP: screen=FOCUS; break; 00265 case K_FDOWN: screen=FOCUS; break; 00266 case K_ORIGIN: screen=ORIGIN; break; 00267 } 00268 if ((mot->ready()) && ( (x!=xt) || (y != yt) )) { 00269 mot->moveTo(x, y, z, speed); 00270 } else { 00271 // if (! mot->ready()) 00272 // printf("Buffer vol\n"); 00273 } 00274 args[0]=x-xoff; 00275 args[1]=y-yoff; 00276 break; 00277 00278 case FOCUS: // focus 00279 mot->getPosition(&x, &y, &z); 00280 switch ( c ) { 00281 case K_FUP: z+=speed; if (z>cfg->zmax) z=cfg->zmax; break; 00282 case K_FDOWN: z-=speed; if (z<0) z=0; break; 00283 case K_LEFT: screen=MOVE; break; 00284 case K_RIGHT: screen=MOVE; break; 00285 case K_UP: screen=MOVE; break; 00286 case K_DOWN: screen=MOVE; break; 00287 case K_ORIGIN: screen=ORIGIN; break; 00288 case K_OK: case K_CANCEL: screen=MAIN; waitup=1; break; 00289 case 0: break; 00290 default: screen=MAIN; waitup=1; break; 00291 } 00292 if ( mot->ready() && (z!=zt) ) 00293 mot->moveTo(x, y, z, speed); 00294 00295 args[0]=z-zoff; 00296 break; 00297 00298 case HOME:// home 00299 switch ( c ) { 00300 case K_OK: screen=HOMING; break; 00301 case K_CANCEL: screen=MAIN; menu=MAIN; waitup=1; break; 00302 } 00303 break; 00304 00305 case ORIGIN: // origin 00306 switch ( c ) { 00307 case K_CANCEL: screen=MAIN; menu=MAIN; waitup=1; break; 00308 case K_OK: 00309 case K_ORIGIN: 00310 xoff = x; 00311 yoff = y; 00312 zoff = z; 00313 mot->setOrigin(x,y,z); 00314 screen = lastscreen; 00315 waitup = 1; 00316 break; 00317 } 00318 break; 00319 00320 case DELETE_ALL: // Delete all files 00321 switch ( c ) { 00322 case K_OK: // delete current job 00323 cleandir(); 00324 screen=MAIN; 00325 waitup = 1; 00326 strcpy(jobname, ""); 00327 break; 00328 case K_CANCEL: screen=MAIN; waitup = 1; break; 00329 } 00330 break; 00331 00332 case IP: // IP 00333 switch ( c ) { 00334 case K_RIGHT: ipfield++; waitup=1; break; 00335 case K_LEFT: ipfield--; waitup=1; break; 00336 case K_OK: screen=MAIN; menu=MAIN; break; 00337 case K_CANCEL: screen=MAIN; menu=MAIN; break; 00338 } 00339 ipfield %= 4; 00340 sarg = (char*)ipfields[ipfield]; 00341 switch (ipfield) { 00342 case 0: memcpy(args, cfg->ip, 4*sizeof(int) ); break; 00343 case 1: memcpy(args, cfg->nm, 4*sizeof(int) ); break; 00344 case 2: memcpy(args, cfg->gw, 4*sizeof(int) ); break; 00345 case 3: memcpy(args, cfg->dns, 4*sizeof(int) ); break; 00346 default: memset(args,0,4*sizeof(int)); break; 00347 } 00348 break; 00349 00350 case REBOOT: // RESET MACHINE 00351 mbed_reset(); 00352 break; 00353 00354 /* 00355 case IO: // IO 00356 switch ( c ) { 00357 case K_RIGHT: iofield++; waitup=1; break; 00358 case K_LEFT: iofield--; waitup=1; break; 00359 case K_OK: screen=lastscreen; break; 00360 case K_CANCEL: screen=lastscreen; break; 00361 } 00362 iofield %= sizeof(iofields)/sizeof(char*); 00363 sarg = (char*)iofields[iofield]; 00364 args[0] = ipfield; 00365 args[1] = ipfield; 00366 break; 00367 00368 case POWER: // POWER 00369 switch ( c ) { 00370 case K_RIGHT: powerfield++; waitup=1; break; 00371 case K_LEFT: powerfield--; waitup=1; break; 00372 case K_UP: power[powerfield % 4] += speed; break; 00373 case K_DOWN: power[powerfield % 4] -= speed; break; 00374 case K_OK: screen=lastscreen; break; 00375 case K_CANCEL: screen=lastscreen; break; 00376 } 00377 powerfield %= 4; 00378 args[1] = powerfield; 00379 sarg = (char*)powerfields[powerfield]; 00380 args[0] = power[powerfield]; 00381 break; 00382 */ 00383 case HOMING: // Homing screen 00384 x = cfg->xhome; 00385 y = cfg->yhome; 00386 while ( !mot->isStart() ); 00387 mot->home(cfg->xhome,cfg->yhome,cfg->zhome); 00388 screen=lastscreen; 00389 break; 00390 00391 case RUNNING: // Screen while running 00392 switch ( c ) { 00393 case K_CANCEL: 00394 while (mot->queue()); 00395 mot->reset(); 00396 if (runfile != NULL) fclose(runfile); 00397 runfile=NULL; screen=MAIN; menu=MAIN; 00398 break; 00399 default: 00400 if (runfile == NULL) { 00401 runfile = sd.openfile(jobname, "rb"); 00402 if (! runfile) 00403 screen=MAIN; 00404 else 00405 mot->reset(); 00406 } else { 00407 while ((!feof(runfile)) && mot->ready()) 00408 mot->write(readint(runfile)); 00409 if (feof(runfile) && mot->ready()) { 00410 fclose(runfile); 00411 runfile = NULL; 00412 screen=MAIN; 00413 } else { 00414 nodisplay = 1; 00415 } 00416 } 00417 } 00418 break; 00419 00420 default: 00421 screen = MAIN; 00422 break; 00423 } 00424 //if (nodisplay == 0) { 00425 dsp->ShowScreen(screens[screen], args, sarg); 00426 //} 00427 prevscreen = screen; 00428 } 00429 00430 } 00431 00432 void LaosMenu::SetFileName(char * name) { 00433 strcpy(jobname, name); 00434 }
Generated on Tue Jul 12 2022 21:03:03 by
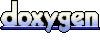