
This is a test program for the LaOS - Laser Open Source driverboard, stepper drivers, sensors and I2C display.
Dependencies: mbed SDFileSystem
main.cpp
00001 /* 00002 * main.cpp 00003 * 00004 * Copyright (c) 2011 Peter Brier 00005 * 00006 * This file is part of the LaOS project (see: http://laoslaser.org) 00007 * 00008 * LaOS is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * LaOS is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License 00019 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * This program tests the inputs and outputs of the laoslaser v1 board 00022 * LED1 = xhome 00023 * LED2 = yhome 00024 * LED3 = zmin 00025 * LED4 = zmax 00026 * 00027 */ 00028 #include "mbed.h" 00029 #include "SDFileSystem.h" 00030 00031 // USB Serial 00032 Serial pc(USBTX, USBRX); // tx, rx 00033 00034 // SD card 00035 SDFileSystem sd(p11, p12, p13, p14, "sd"); 00036 00037 // Analog in/out (cover sensor) + NC 00038 DigitalIn cover(p19); 00039 00040 // I2C 00041 I2C i2c(p9, p10); // sda, scl 00042 #define _I2C_ADDRESS 0x04 00043 #define _I2C_HOME 0xFE 00044 #define _I2C_CLS 0xFF 00045 #define _I2C_BAUD 10000 00046 00047 // status leds 00048 DigitalOut led1(LED1); 00049 DigitalOut led2(LED2); 00050 DigitalOut led3(LED3); 00051 DigitalOut led4(LED4); 00052 00053 // Inputs; 00054 DigitalIn xhome(p8); 00055 DigitalIn yhome(p17); 00056 DigitalIn zmin(p15); 00057 DigitalIn zmax(p16); 00058 00059 00060 // motors 00061 DigitalOut enable(p7); 00062 DigitalOut xdir(p23); 00063 DigitalOut xstep(p24); 00064 DigitalOut ydir(p25); 00065 DigitalOut ystep(p26); 00066 DigitalOut zdir(p27); 00067 DigitalOut zstep(p28); 00068 00069 // laser 00070 DigitalOut o1(p22); 00071 DigitalOut o2(p21); 00072 DigitalOut o3(p6); 00073 DigitalOut o4(p5); 00074 00075 00076 // CAN bus 00077 CAN can(p30, p29); 00078 00079 00080 void home() 00081 { 00082 printf("Homing with x,y,z dir=0\n"); 00083 int i=0; 00084 xdir = 0; 00085 ydir = 0; 00086 zdir = 0; 00087 led1 = 0; 00088 while ( 1 ) 00089 { 00090 xstep = ystep = 0; 00091 wait(0.0001); 00092 xstep = xhome; 00093 ystep = yhome; 00094 zstep = zmin; 00095 wait(0.0001); 00096 led1 = !xhome; 00097 led2 = !yhome; 00098 led3 = !zmin; 00099 led4 = ((i++) & 0x10000); 00100 if ( (!xhome) && (!yhome) ) return; 00101 } 00102 } 00103 00104 #define TEST(c,x) case c: io = &x; x = !x; printf("Test %c: " #x " is now %s \n\r", c, (x ? "ON" : "OFF")); break 00105 00106 /** 00107 *** main() 00108 **/ 00109 int main() 00110 { 00111 led1 = led2 = led3 = led4 = 1; 00112 Timer t; 00113 DigitalOut *io = NULL; 00114 CANMessage msg; 00115 00116 i2c.frequency(_I2C_BAUD); 00117 pc.baud(115200); 00118 //pc.baud(9600); 00119 00120 xhome.mode(PullUp); 00121 yhome.mode(PullUp); 00122 zmin.mode(PullUp); 00123 zmax.mode(PullUp); 00124 00125 char buf[512]; 00126 char *help = "\n\r\n\r\n\rLaOS Test program\n\r" 00127 __DATE__ " " __TIME__ "\n\r" 00128 "++++++++++++++++++\n\r" 00129 "Use these keys to test the functionality of the board:\n\r" 00130 "xX: X Step/Dir\t" 00131 "yY: Y Step/Dir\t" 00132 "zZ: Z Step/Dir\t" 00133 "tT: Ext Step/Dir (o1/o2)\n\r" 00134 "e: Toggle Stepper enable\n\r" 00135 "c: Can bus test\n\r" 00136 "sS: SD-Card test (s=test file, S=speed test)\n\r" 00137 "i: I2C\n\r" 00138 "1/2/3/4: Toggle outputs\n\r" 00139 "f: apply 1Khz frequency to last IO line (1 second)\n\r" 00140 "h: X/Y homing (note: direction and home sensor polarity may be wrong\n\r"; 00141 char iline[] = " LAOS-IOTEST V1.1"; 00142 iline[0] = 0xFF; 00143 //i2c.write(_I2C_ADDRESS, iline, 17); 00144 printf(help); 00145 00146 int i=0; 00147 int test=0; 00148 char key=0; 00149 while( 1 ) 00150 { 00151 i++; 00152 led1 = xhome; 00153 led2 = yhome; 00154 led3 = zmin; 00155 led4 = zmax; 00156 if ( pc.readable() ) 00157 test = pc.getc(); 00158 else 00159 test = 0; 00160 switch( test ) 00161 { 00162 TEST('e',enable); 00163 TEST('X',xdir); 00164 TEST('x',xstep); 00165 TEST('Y',ydir); 00166 TEST('y',ystep); 00167 TEST('Z',zdir); 00168 TEST('z',zstep); 00169 TEST('t',o2); 00170 TEST('T',o1); 00171 TEST('1',o1); 00172 TEST('2',o2); 00173 TEST('3',o3); 00174 TEST('4',o4); 00175 case 'h': 00176 home(); 00177 break; 00178 case 'f': 00179 case 'F': 00180 if (io != NULL ) 00181 { 00182 printf("pulsing output for 1000 pulses sec at 500Hz or 10kHz...\n"); 00183 for(i=0;i<1000;i++) 00184 { 00185 wait((test == 'f' ? 0.001 : 0.00005)); 00186 *io = 1; 00187 wait((test == 'f' ? 0.001 : 0.00005)); 00188 *io = 0; 00189 } 00190 00191 } 00192 break; 00193 case 's': 00194 memset(buf,0,sizeof(buf)); 00195 printf("Testing IO board SD card: Write...\n\r"); 00196 FILE *test1 = fopen("/sd/text.txt", "wb"); 00197 fwrite("bla bla\n",8, 1, test1); 00198 fclose(test1); 00199 printf("Testing IO board SD card: Read...\n\r"); 00200 test1 = fopen("/sd/text.txt", "rb"); 00201 fread(&buf,8, 1, test1); 00202 printf("Result: '%s'\n\r", buf); 00203 fclose(test1); 00204 break; 00205 case 'S': 00206 printf("SD card speed test (writing and reading 1MByte file\n"); 00207 memset(buf,23,sizeof(buf)); 00208 FILE *test2 = fopen("/sd/test.bin", "wb"); 00209 t.reset(); 00210 t.start(); 00211 for(i=0;i<sizeof(buf);i++) 00212 buf[i] = (char)t.read_ms(); 00213 t.stop(); t.reset(); t.start(); 00214 for(i=0;i<200;i++) 00215 fwrite(buf,sizeof(buf), 1, test2); 00216 t.stop(); 00217 fclose(test2); 00218 printf("Write 1MByte: %d msec\n\r", (int)t.read_ms()); 00219 test2 = fopen("/sd/test.bin", "rb"); 00220 t.reset(); 00221 t.start(); 00222 while( fread(&buf, sizeof(buf), 1, test2) > 0 ); 00223 t.stop(); 00224 printf("Read 1MByte: %d msec\n\r", (int)t.read_ms()); 00225 case 'i': 00226 printf("I2C test:\n\r"); 00227 char s[48]; 00228 sprintf(s," 0123456789ABCDEF1234567890ABCDEF"); 00229 s[0] = 0xFF; 00230 i2c.write(_I2C_ADDRESS, s, strlen(s)); 00231 wait(3); 00232 i2c.read(_I2C_ADDRESS, s, 48); 00233 sprintf(iline," Key read: %c", s[0]); 00234 i2c.write(_I2C_ADDRESS, iline, strlen(iline)); 00235 break; 00236 case 'c': 00237 printf("CAN bus test, sending and receiving characters, press any key to quit\n\r"); 00238 can.frequency(500000); 00239 can.reset(); 00240 t.start(); 00241 can.reset(); 00242 while( !pc.readable() ) 00243 { 00244 if ( t > 1.0 ) 00245 { 00246 t.reset(); 00247 can.write(CANMessage(10, &key, 1)); 00248 key++; 00249 } 00250 wait(0.1); 00251 if(can.read(msg)) 00252 printf("Message received: id=%d, len=%d, data[0]=%d\n\n\r", (int)msg.id, (int)msg.len, (int)msg.data[0]); 00253 00254 printf("value=%d, rderror=%d, tderror=%d\n\r", (int)key, can.rderror(), can.tderror()); 00255 00256 } 00257 break; 00258 case 0: 00259 case '\n': 00260 case '\r': break; 00261 default: printf(help); break; 00262 } 00263 //i2c.read(_I2C_ADDRESS,&key, 1); 00264 if (key != 0) { 00265 char s[48]; 00266 printf("I2C key read: %c\n\r", key); 00267 sprintf(s," Key read: %c", key); 00268 s[0] = 0xFF; 00269 //i2c.write(_I2C_ADDRESS, s, strlen(s)); 00270 wait(0.2); 00271 if (key == '7') { 00272 wait(1); 00273 //i2c.write(_I2C_ADDRESS, iline, 17); 00274 } 00275 //i2c.read(_I2C_ADDRESS, s, 48); 00276 key=0; 00277 } 00278 if ( test ) 00279 { 00280 printf("xhome=%d, yhome=%d, zmin=%d, zmax=%d, cover=%s\n\r", (int)xhome, (int)yhome, (int)zmin, (int)zmax, (cover ? "OPEN" : "CLOSED") ); 00281 } 00282 } 00283 }
Generated on Wed Jul 13 2022 03:18:52 by
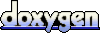