
Varijanta TFT banggood za PAI
Dependencies: SDFileSystem SPI_TFT_ILI9341 TFT_fonts mbed
Fork of TFT_banggood by
main.cpp
00001 // example to test the TFT Display from banggood.com 00002 // Thanks to the GraphicsDisplay and TextDisplay classes 00003 // test.bmp has to be on the mbed file system 00004 // and on the sd-card 00005 00006 #include "stdio.h" 00007 #include "mbed.h" 00008 #include "SPI_TFT_ILI9341.h" 00009 #include "string" 00010 #include "Arial12x12.h" 00011 #include "Arial24x23.h" 00012 #include "Arial28x28.h" 00013 #include "font_big.h" 00014 #include "SDFileSystem.h" 00015 #define dp23 P0_0 00016 00017 // the SD-connector is connected to SPI pin 11-13 00018 //SDFileSystem sd(p11, p12, p13, p14, "sd"); // mosi,miso,sck,cs 00019 00020 //LocalFileSystem local("local"); 00021 00022 //extern unsigned char p1[]; // the mbed logo graphic 00023 00024 // the display has a backlight switch on board 00025 //DigitalOut LCD_LED(p21); 00026 00027 extern unsigned char p1[]; // the mbed logo graphic 00028 00029 00030 // the TFT is connected to SPI pin 5-7 00031 //SPI_TFT_ILI9341 TFT(p5, p6, p7, p8, p9, p10,"TFT"); // mosi, miso, sclk, cs, reset, dc 00032 SPI_TFT_ILI9341 TFT(dp2,dp1,dp6,dp24,dp23,dp25,"TFT"); // mosi, miso, sclk, cs, reset, dc 00033 00034 int main() 00035 { 00036 int i=0; 00037 TFT.claim(stdout); // send stdout to the TFT display 00038 TFT.set_orientation(1); 00039 TFT.background(Black); // set background to black 00040 TFT.foreground(White); // set chars to white 00041 TFT.cls(); // clear the screen 00042 00043 while(1){ 00044 //first show the 4 directions 00045 TFT.set_orientation(0); 00046 TFT.background(Black); 00047 TFT.cls(); 00048 00049 TFT.set_font((unsigned char*) Arial12x12); 00050 TFT.locate(0,0); 00051 printf(" Hello Mbed 0"); 00052 00053 wait(5); // wait two seconds 00054 00055 // draw some graphics 00056 TFT.cls(); 00057 TFT.set_font((unsigned char*) Arial24x23); 00058 TFT.locate(100,100); 00059 TFT.printf("Graphic"); 00060 00061 TFT.line(0,0,100,0,Green); 00062 TFT.line(0,0,0,200,Green); 00063 TFT.line(0,0,100,200,Green); 00064 wait(5); // wait two seconds 00065 TFT.rect(100,50,150,100,Red); 00066 TFT.fillrect(180,25,220,70,Blue); 00067 00068 00069 TFT.circle(80,150,33,White); 00070 TFT.fillcircle(160,190,20,Yellow); 00071 00072 double s; 00073 00074 for (i=0; i<320; i++) { 00075 s =20 * sin((long double) i / 10 ); 00076 TFT.pixel(i,100 + (int)s ,Red); 00077 } 00078 00079 00080 wait(5); // wait two seconds 00081 00082 /* 00083 // bigger text 00084 TFT.foreground(White); 00085 TFT.background(Blue); 00086 TFT.cls(); 00087 TFT.set_font((unsigned char*) Arial24x23); 00088 TFT.locate(0,0); 00089 TFT.printf("Different Fonts :"); 00090 00091 TFT.set_font((unsigned char*) Neu42x35); 00092 TFT.locate(10,30); 00093 TFT.printf("Hello Mbed 1"); 00094 TFT.set_font((unsigned char*) Arial24x23); 00095 TFT.locate(20,80); 00096 TFT.printf("Hello Mbed 2"); 00097 TFT.set_font((unsigned char*) Arial12x12); 00098 TFT.locate(35,120); 00099 TFT.printf("Hello Mbed 3"); 00100 00101 */ 00102 00103 wait(5); 00104 00105 TFT.background(Black); 00106 TFT.cls(); 00107 TFT.locate(10,10); 00108 TFT.printf("Graphic from Flash"); 00109 00110 // mbed logo 00111 // defined in graphics.c 00112 //__align(4) 00113 //unsigned char p1[18920] = { 00114 //0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, .... 00115 // 00116 00117 TFT.Bitmap(90,90,172,55,p1); 00118 00119 wait(5); 00120 TFT.cls(); 00121 00122 /* 00123 // to compare the speed of the internal file system and a SD-card 00124 TFT.locate(10,10); 00125 TFT.printf("Graphic from internal File System"); 00126 TFT.locate(10,20); 00127 TFT.printf("open test.bmp"); 00128 int err = TFT.BMP_16(1,50,"/local/test.bmp"); 00129 if (err != 1) TFT.printf(" - Err: %d",err); 00130 */ 00131 /* TFT.locate(10,110); 00132 TFT.printf("Graphic from external SD-card"); 00133 TFT.locate(10,120); 00134 err = TFT.BMP_16(1,140,"/sd/test.bmp"); 00135 if (err != 1) TFT.printf(" - Err: %d",err); 00136 */ 00137 } 00138 00139 00140 00141 }
Generated on Fri Jul 15 2022 07:34:38 by
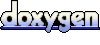