
Just a little example how to use the pushover.net service in mbed and generally POST
Dependencies: EthernetInterface mbed-rtos mbed
Fork of TCPSocket_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 00004 00005 /* 00006 By Andrea Campanella 00007 Just a small example how to use pushover.net 00008 with mbed and generally ho to use POST in mbed. 00009 */ 00010 00011 00012 int main() 00013 { 00014 EthernetInterface eth; 00015 eth.init(); //Use DHCP 00016 eth.connect(); 00017 printf("IP Address is %s\n", eth.getIPAddress()); 00018 // Pushover settings 00019 char pushoversite[] = "api.pushover.net"; 00020 char apitoken[] = "APIKEY"; 00021 char userkey [] = "USERKEY"; 00022 char message [] = "Hello mate"; 00023 TCPSocketConnection sock; 00024 sock.connect(pushoversite, 80); 00025 00026 char http_cmd[300] = "POST /1/messages.json HTTP/1.1\r\n" ; 00027 char length[10]; 00028 sprintf(length, "%d", 113 + sizeof(message)-1); 00029 00030 //strcat( http_cmd , "POST /1/messages.json HTTP/1.1" ); 00031 strcat( http_cmd , "Host: api.pushover.net\r\n"); 00032 strcat( http_cmd , "Connection: close\r\n "); 00033 strcat( http_cmd , "Content-Type: application/x-www-form-urlencoded\r\n"); 00034 strcat( http_cmd , "Content-Length: "); 00035 strcat( http_cmd , length); 00036 strcat( http_cmd , "\r\n\r\n");; 00037 strcat( http_cmd , "token="); 00038 strcat( http_cmd , apitoken); 00039 strcat( http_cmd , "&user="); 00040 strcat( http_cmd , userkey); 00041 strcat( http_cmd , "&message="); 00042 strcat( http_cmd , message); 00043 strcat( http_cmd , "&priority="); 00044 strcat( http_cmd , "0"); 00045 strcat( http_cmd , "&retry=60"); 00046 strcat( http_cmd , "&expire=3600"); 00047 printf("Sending :%s\n", http_cmd); 00048 00049 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00050 00051 00052 char buffer[300]; 00053 int ret; 00054 while (true) { 00055 ret = sock.receive(buffer, sizeof(buffer)-1); 00056 if (ret <= 0) 00057 break; 00058 buffer[ret] = '\0'; 00059 printf("Received %d chars from server:\n%s\n", ret, buffer); 00060 } 00061 00062 sock.close(); 00063 00064 eth.disconnect(); 00065 00066 while(1) {} 00067 }
Generated on Sun Jul 24 2022 15:30:38 by
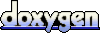