
f
Dependencies: TS_DISCO_F746NG mbed LCD_DISCO_F746NG mbed-rtos BSP_DISCO_F746NG FatFS X_NUCLEO_IHM02A1
main.cpp
00001 #include "mbed.h" 00002 #include "TS_DISCO_F746NG.h" 00003 #include "LCD_DISCO_F746NG.h" 00004 #include "eeprom.h" 00005 #include <ctype.h> 00006 #include "rtos.h" 00007 #define USE_STM32746G_DISCOVERY 00008 #include "stm32f7xx_hal.h" 00009 #include "stm32746g_discovery.h" 00010 #include "stm32746g_discovery_sd.h" 00011 #include "string.h" 00012 #include "stm32746g_discovery_lcd.h" 00013 #include "ff_gen_drv.h" 00014 #include "sd_diskio.h" 00015 #include "XNucleoIHM02A1.h" 00016 #include "wolfman.h" 00017 #include "hfl.h" 00018 #include "PCF85263AT.h" 00019 00020 #define FRAME_BUFFER 0xC0000000 00021 #define SD_IN_BUFFER 0xC0140000 00022 #define SD_OUT_BUFFER 0xC0280000 00023 00024 00025 I2C i2c(D14,D15); // sda, scl 00026 00027 char cmd[32]; 00028 dt_dat dt; 00029 00030 FATFS SDFatFs; 00031 FIL MyFile; 00032 00033 Thread thread; 00034 Thread thread2; 00035 Thread thread3; 00036 00037 00038 LCD_DISCO_F746NG lcd; 00039 TS_DISCO_F746NG ts; 00040 00041 extern SD_HandleTypeDef uSdHandle; 00042 DMA2D_HandleTypeDef hdma2d_disco; 00043 Serial pc(USBTX, USBRX); 00044 00045 char SDPath[4]; 00046 //char text [40]; 00047 char c; 00048 00049 TS_StateTypeDef TS_State; 00050 00051 uint16_t x, y; 00052 uint8_t text1[30]; 00053 uint8_t text2[30]; 00054 uint8_t text3[30]; 00055 uint8_t text4[60]; 00056 uint8_t text5[60]; 00057 uint8_t textn1[30]; 00058 uint8_t textn2[30]; 00059 uint8_t textn3[30]; 00060 uint8_t textn4[60]; 00061 uint8_t textn5[60]; 00062 uint8_t savereading[120]; 00063 uint8_t currenttime[60]; 00064 uint8_t idx; 00065 uint8_t cleared = 0; 00066 uint8_t prev_nb_touches = 0; 00067 static uint8_t nus_buffer[20]; 00068 double Silver = 0.0; 00069 double Copper = 0.0; 00070 double LEDCUR = 0.0; 00071 uint8_t cyclestep[7]; 00072 bool CuMODE = true; 00073 bool AgMODE = true; 00074 int screenstate = 0; 00075 int MODE = 0; 00076 int ZERO = 0.0; 00077 int DARK = 0.0; 00078 int READ = 0.0; 00079 int LVL = 0.0; 00080 double RAW = 0.0; 00081 double SilverRAW = 0.0; 00082 double CopperRAW = 0.0; 00083 int writefail = 0; 00084 int* toucharrow; 00085 int* touchbutt; 00086 bool ManMode = false; 00087 FRESULT ress; 00088 uint32_t bytesread; 00089 double TempCurrent = 0.0; 00090 00091 //PwmOut pump1(PA_8); 00092 00093 DigitalOut pump1(D0); // PA_8 00094 DigitalOut pump2(D1); //PA_15; 00095 DigitalOut pump3(A3); 00096 //DigitalOut pump4(A2); 00097 DigitalOut pump5(A4); 00098 DigitalOut pump6(A5); 00099 00100 PwmOut LED515(D10); 00101 PwmOut LED480(A2); 00102 DigitalOut LEDLD(D8); 00103 DigitalOut LEDBL(D9); 00104 DigitalOut Tempcs(A0); 00105 DigitalOut Heat(A1); 00106 00107 00108 SPI spi(D11, D12, D13); // mosi, miso, sclk 00109 DigitalOut cs(D7); 00110 00111 00112 00113 /* Number of movements per revolution. */ 00114 #define MPR_1 4 00115 00116 /* Number of steps. */ 00117 #define STEPS_1 (200 * 128) /* 1 revolution given a 400 steps motor configured at 1/128 microstep mode. */ 00118 #define STEPS_2 (STEPS_1 * 2) 00119 00120 /* Delay in milliseconds. */ 00121 #define DELAY_1 1000 00122 #define DELAY_2 2000 00123 #define DELAY_3 5000 00124 00125 00126 ///XNucleoIHM02A1 *x_nucleo_ihm02a1; 00127 L6470_init_t init[L6470DAISYCHAINSIZE] = { 00128 /* First Motor. */ 00129 { 00130 /* Ic configuration. */ 00131 }, 00132 00133 /* Second Motor. */ 00134 { 00135 12.0, /* Motor supply voltage in V. */ 00136 200, /* Min number of steps per revolution for the motor. */ 00137 1.5, /* Max motor phase voltage in A. */ 00138 4.2, /* Max motor phase voltage in V. */ 00139 100.0, /* Motor initial speed [step/s]. */ 00140 400.0, /* Motor acceleration [step/s^2] (comment for infinite acceleration mode). */ 00141 400.0, /* Motor deceleration [step/s^2] (comment for infinite deceleration mode). */ 00142 900.0, /* Motor maximum speed [step/s]. */ 00143 0.0, /* Motor minimum speed [step/s]. */ 00144 902.7, /* Motor full-step speed threshold [step/s]. */ 00145 1.5, /* Holding kval [V]. */ 00146 5.2, /* Constant speed kval [V]. */ 00147 5.2, /* Acceleration starting kval [V]. */ 00148 5.2, /* Deceleration starting kval [V]. */ 00149 371.52, /* Intersect speed for bemf compensation curve slope changing [step/s]. */ 00150 392.1569e-6, /* Start slope [s/step]. */ 00151 643.1372e-6, /* Acceleration final slope [s/step]. */ 00152 643.1372e-6, /* Deceleration final slope [s/step]. */ 00153 0, /* Thermal compensation factor (range [0, 15]). */ 00154 3.06 * 1000 * 1.10, /* Ocd threshold [ma] (range [375 ma, 6000 ma]). */ 00155 3.06 * 1000 * 1.00, /* Stall threshold [ma] (range [31.25 ma, 4000 ma]). */ 00156 StepperMotor::STEP_MODE_1_128, /* Step mode selection. */ 00157 0xFF, /* Alarm conditions enable. */ 00158 0x2E88 /* Ic configuration. */ 00159 } 00160 }; 00161 00162 XNucleoIHM02A1 *x_nucleo_ihm02a1 = new XNucleoIHM02A1(&init[0], &init[1], D5/*D14*/, D4/*D15*/, D2/*D4*/, D6/*A2*/, D11, D12, D13); 00163 L6470 **motors = x_nucleo_ihm02a1->get_components(); 00164 00165 00166 00167 /* Virtual address defined by the user: 0xFFFF value is prohibited */ 00168 uint16_t VirtAddVarTab[NB_OF_VAR] = {0x5555, 0x6666, 0x7777, 0x8888, 0x8899, 0x5655, 0x6776, 0x7887, 0x7117}; 00169 uint16_t VarDataTab[] = {2, 1, 5, 1, 1, 60, 1, 0, 0}; 00170 00171 00172 //ADC Struct for sensor 00173 union LTC2400 { 00174 unsigned int raw; 00175 unsigned char byte[4]; 00176 struct { 00177 unsigned int 00178 sub:4, 00179 reading:24, 00180 exr:1, 00181 sgn:1, 00182 dmy:1, 00183 eoc:1; 00184 }; 00185 } adc; 00186 00187 //Prime Button 00188 int primeon[4]; 00189 int pcount = 0; 00190 //Man Button 00191 int Manonoff[4]; 00192 //CuMODE Button 00193 int CuMODEonoff[4]; 00194 //AgMODE Button 00195 int AgMODEonoff[4]; 00196 //screen mode 00197 int screenmode[4]; 00198 //All Button 00199 int Aonoff[4]; 00200 bool Aon = 0; 00201 //pump1 00202 double p1count = 3; 00203 int p1up[4]; 00204 int p1down[4]; 00205 int p1onoff[4]; 00206 bool p1on = false; 00207 //pump2 00208 double p2count = 5; 00209 int p2up[4]; 00210 int p2down[4]; 00211 int p2onoff[4]; 00212 bool p2on = false; 00213 //pump3 00214 double p3count = 1; 00215 int p3up[4]; 00216 int p3down[4]; 00217 int p3onoff[4]; 00218 bool p3on = false; 00219 //pump4 00220 double p4count = 1; 00221 int p4up[4]; 00222 int p4down[4]; 00223 int p4onoff[4]; 00224 bool p4on = false; 00225 //Sole5 00226 double p5count = 1; 00227 int p5up[4]; 00228 int p5down[4]; 00229 int p5onoff[4]; 00230 bool p5on = false; 00231 //Pump6 00232 double p6count = 6; 00233 int p6up[4]; 00234 int p6down[4]; 00235 int p6onoff[4]; 00236 bool p6on = false; 00237 //Pump7 00238 double p7count = 1; 00239 int p7up[4]; 00240 int p7down[4]; 00241 int p7onoff[4]; 00242 bool p7on = false; 00243 00244 //date/time 00245 double d1count = 1; 00246 int d1up[4]; 00247 int d1down[4]; 00248 double d2count = 1; 00249 int d2up[4]; 00250 int d2down[4]; 00251 double d3count = 1; 00252 int d3up[4]; 00253 int d3down[4]; 00254 double d4count = 1; 00255 int d4up[4]; 00256 int d4down[4]; 00257 double d5count = 1; 00258 int d5up[4]; 00259 int d5down[4]; 00260 int savedate[4]; 00261 double d6count = 0; 00262 int d6up[4]; 00263 int d6down[4]; 00264 00265 00266 void set_ch(char sel) 00267 { // PCA9541のサンプル 00268 // MST_0側の自分にスレーブ側の制御権を得る場合 00269 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00270 i2c.write( 0xe2, cmd, 1); // Cont Regを指定 00271 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00272 wait(0.1); // 0.1s待つ 00273 switch(cmd[0] & 0xf) 00274 { 00275 case 0: // bus off, has control 00276 case 1: // bus off, no control 00277 case 5: // bus on, no control 00278 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00279 cmd[1] = 4; // bus on, has control 00280 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00281 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00282 break; 00283 case 2: // bus off, no control 00284 case 3: // bus off, has control 00285 case 6: // bus on, no control 00286 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00287 cmd[1] = 5; // bus on, has control 00288 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00289 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00290 break; 00291 case 9: // bus on, no control 00292 case 0xc: // bus on, no control 00293 case 0xd: // bus off, no control 00294 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00295 cmd[1] = 0; // bus on, has control 00296 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00297 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00298 break; 00299 case 0xa: // bus on, no control 00300 case 0xe: // bus off, no control 00301 case 0xf: // bus on, has control 00302 cmd[0] = 1; // PCA9541 コマンドコード Cont Reg 00303 cmd[1] = 1; // bus on, has control 00304 i2c.write( 0xe2, cmd, 2); // Cont Regにcmd[1]を書込み 00305 i2c.read( 0xe2, cmd, 1); // Cont Regを読込み 00306 break; 00307 default: 00308 break; 00309 } 00310 00311 cmd[0] = sel; // PCA9546 Cont Reg sel channel enabled 00312 i2c.write( 0xe8, cmd, 1); // Send command string 00313 } 00314 00315 void get_time(dt_dat *dt) // 日時の取得 00316 { 00317 cmd[0] = Seconds_100th; // 取得はレジスタSecondsから 00318 i2c.write(PCF85263AT_ADDR, cmd, 1); // レジスタの設定 00319 i2c.read(PCF85263AT_ADDR, cmd, 8); // SecondsからYearsまで取得 00320 cmd[1] &= 0x7f; // 有効なのは下位7ビット 00321 dt->s = (cmd[1] >> 4) * 10 + (cmd[1] & 0xf); // BCDの数値化 00322 cmd[2] &= 0x7f; // 有効なのは下位7ビット 00323 dt->m = (cmd[2] >> 4) * 10 + (cmd[2] & 0xf); // BCDの数値化 00324 cmd[3] &= 0x3f; // 有効なのは下位6ビット 00325 dt->h = (cmd[3] >> 4) * 10 + (cmd[3] & 0xf); // BCDの数値化 00326 cmd[4] &= 0x3f; // 有効なのは下位6ビット 00327 dt->d = (cmd[4] >> 4) * 10 + (cmd[4] & 0xf); // BCDの数値化 00328 dt->wd = (cmd[5] & 0x3); // BCDの数値化 00329 cmd[6] &= 0x1f; // 有効なのは下位5ビット 00330 dt->mm = (cmd[6] >> 4) * 10 + (cmd[6] & 0xf); // BCDの数値化 00331 dt->y = (cmd[7] >> 4) * 10 + (cmd[7] & 0xf); // BCDの数値化 00332 } 00333 00334 void set_time(dt_dat *dt) // 日時の設定 00335 { 00336 cmd[0] = Seconds_100th; // 設定はレジスタSeconds_100thから 00337 cmd[1] = ((dt->s100th / 10) << 4) + (dt->s100th % 10);// 0.01秒のBCD化 00338 cmd[2] = ((dt->s / 10) << 4) + (dt->s % 10) + 0x80; // 秒のBCD化 00339 cmd[3] = ((dt->m / 10) << 4) + (dt->m % 10); // 分のBCD化 00340 cmd[4] = ((dt->h / 10) << 4) + (dt->h % 10); // 時のBCD化 00341 cmd[5] = ((dt->d / 10) << 4) + (dt->d % 10); // 日のBCD化 00342 cmd[7] = ((dt->mm / 10) << 4) + (dt->mm % 10); // 月のBCD化 00343 dt->y = dt->y - 2000; 00344 cmd[8] = ((dt->y / 10) << 4) + (dt->y % 10); // 年のBCD化 00345 i2c.write(PCF85263AT_ADDR, cmd, 9); // 日時の設定 00346 } 00347 00348 00349 00350 00351 int * DrawButton(int x, int y, int w, int h){ 00352 int* pointer; 00353 int butttouch[4]; 00354 pointer = butttouch; 00355 lcd.FillRect(x, y, w, h); 00356 butttouch[0] = x; 00357 butttouch[1] = x+w; 00358 butttouch[2] = y; 00359 butttouch[3] = y+h; 00360 return pointer; 00361 } 00362 00363 int * DrawArrow(int x, int y, int d, int s){ 00364 int* pointer; 00365 int arrowtouch[4]; 00366 pointer = arrowtouch; 00367 if (d == 1) { 00368 Point points[] = {{x-10*s,y+10*s},{x,y},{x+10*s,y+10*s},{x+2*s,y+10*s},{x+2*s,y+20*s},{x-2*s,y+20*s},{x-2*s,y+10*s}}; 00369 lcd.FillPolygon(points,7); 00370 arrowtouch[0] = x-10*s; 00371 arrowtouch[1] = x+10*s; 00372 arrowtouch[2] = y; 00373 arrowtouch[3] = y+20*s; 00374 } else if (d == 2) { 00375 Point points[] = {{x+10*s,y-10*s},{x,y},{x-10*s,y-10*s},{x-2*s,y-10*s},{x-2*s,y-20*s},{x+2*s,y-20*s},{x+2*s,y-10*s}}; 00376 lcd.FillPolygon(points,7); 00377 arrowtouch[0] = x-10*s; 00378 arrowtouch[1] = x+10*s; 00379 arrowtouch[2] = y-20*s; 00380 arrowtouch[3] = y; 00381 } else if (d == 3) { 00382 Point points[] = {{x,y},{x-10*s,y-10*s},{x-10*s,y-2*s},{x-20*s,y-2*s},{x-20*s,y+2*s},{x-10*s,y+2*s},{x-10*s,y+10*s}}; 00383 lcd.FillPolygon(points,7); 00384 arrowtouch[0] = x-10*s; 00385 arrowtouch[1] = x+10*s; 00386 arrowtouch[2] = y-20*s; 00387 arrowtouch[3] = y; 00388 } else if (d == 4) { 00389 Point points[] = {{x,y},{x+10*s,y+10*s},{x+10*s,y+2*s},{x+20*s,y+2*s},{x+20*s,y-2*s},{x+10*s,y-2*s},{x+10*s,y-10*s}}; 00390 lcd.FillPolygon(points,7); 00391 arrowtouch[0] = x-10*s; 00392 arrowtouch[1] = x+10*s; 00393 arrowtouch[2] = y-20*s; 00394 arrowtouch[3] = y; 00395 } 00396 return pointer; 00397 } 00398 void runpump(){ 00399 if (ManMode == true) { 00400 while (1) { 00401 //pump1 00402 if (p1on == true) { 00403 for (int j = 1; j<=p1count; j++) { 00404 pump1 = 1; 00405 Thread::wait(400); 00406 pump1 = 0; 00407 Thread::wait(600); 00408 } 00409 } 00410 //pump2 00411 if (p2on == true) { 00412 for (int j = 1; j<=p2count; j++) { 00413 pump2 = 1; 00414 Thread::wait(400); 00415 pump2 = 0; 00416 Thread::wait(600); 00417 } 00418 } 00419 //pump3 00420 if (p3on == true) { 00421 for (int j = 1; j<=p3count; j++) { 00422 pump3 = 1; 00423 Thread::wait(400); 00424 pump3 = 0; 00425 Thread::wait(600); 00426 } 00427 } 00428 /* //pump4 00429 if (p4on == true) { 00430 for (int j = 1; j<=p4count; j++) { 00431 pump4 = 1; 00432 Thread::wait(400); 00433 pump4 = 0; 00434 Thread::wait(600); 00435 } 00436 } */ 00437 //Sole5 00438 if (p5on == true) { 00439 for (int j = 1; j<=p5count; j++) { 00440 pump5 = 1; 00441 Thread::wait(5000); 00442 pump5 = 0; 00443 Thread::wait(300); 00444 } 00445 } 00446 //Pump6 00447 if (p6on == true) { 00448 for (int j = 1; j<=p6count; j++) { 00449 pump6 = 1; 00450 Thread::wait(100); 00451 pump6 = 0; 00452 Thread::wait(100); 00453 } 00454 } 00455 //Pump7 00456 if (p7on == true) { 00457 for (int j = 1; j<=p7count; j++) { 00458 motors[1]->move(StepperMotor::BWD, STEPS_1*6.15); 00459 //motors[1]->wait_while_active(); 00460 Thread::wait(300); 00461 } 00462 } 00463 Thread::wait(2000); 00464 } 00465 } else { 00466 //pump1 00467 if (p1on == true) { 00468 for (int j = 1; j<=p1count; j++) { 00469 pump1 = 1; 00470 Thread::wait(400); 00471 pump1 = 0; 00472 Thread::wait(600); 00473 } 00474 } 00475 //pump2 00476 if (p2on == true) { 00477 for (int j = 1; j<=p2count; j++) { 00478 pump2 = 1; 00479 Thread::wait(400); 00480 pump2 = 0; 00481 Thread::wait(600); 00482 } 00483 } 00484 //pump3 00485 if (p3on == true) { 00486 for (int j = 1; j<=p3count; j++) { 00487 pump3 = 1; 00488 Thread::wait(400); 00489 pump3 = 0; 00490 Thread::wait(600); 00491 } 00492 } 00493 /* //pump4 00494 if (p4on == true) { 00495 for (int j = 1; j<=p4count; j++) { 00496 pump4 = 1; 00497 Thread::wait(400); 00498 pump4 = 0; 00499 Thread::wait(600); 00500 } 00501 } */ 00502 //Sole5 00503 if (p5on == true) { 00504 for (int j = 1; j<=p5count; j++) { 00505 pump5 = 1; 00506 Thread::wait(5000); 00507 //Wash cycle will go here 00508 pump5 = 0; 00509 Thread::wait(300); 00510 } 00511 } 00512 //Pump6 00513 if (p6on == true) { 00514 for (int j = 1; j<=p6count; j++) { 00515 pump6 = 1; 00516 Thread::wait(100); 00517 pump6 = 0; 00518 Thread::wait(100); 00519 } 00520 } 00521 //Pump7 00522 if (p7on == true) { 00523 for (int j = 1; j<=p7count; j++) { 00524 motors[1]->move(StepperMotor::BWD, STEPS_1*6.15); 00525 //motors[1]->wait_while_active(); 00526 Thread::wait(4000); 00527 } 00528 } 00529 00530 } 00531 00532 } 00533 void fill() { 00534 if (MODE != 6) { MODE = 5; } 00535 p1on = false; 00536 p2on = false; 00537 p3on = false; 00538 p4on = false; 00539 p5on = false; 00540 p6on = false; 00541 p7on = true; 00542 runpump(); 00543 if (MODE != 6) { MODE = 4; } 00544 } 00545 void drain() { 00546 p1on = false; 00547 p2on = false; 00548 p3on = false; 00549 p4on = false; 00550 p5on = true; 00551 p6on = false; 00552 p7on = false; 00553 runpump(); 00554 } 00555 void wash() { 00556 MODE = 6; 00557 pump5 = 1; 00558 motors[1]->move(StepperMotor::BWD, STEPS_1*18.45); 00559 Thread::wait(4000); 00560 //motors[1]->wait_while_active(); 00561 pump5 = 0; 00562 motors[1]->move(StepperMotor::BWD, STEPS_1*18.45); 00563 Thread::wait(4000); 00564 //motors[1]->wait_while_active(); 00565 pump5 = 1; 00566 Thread::wait(3000); 00567 pump5 = 0; 00568 MODE = 4; 00569 /* drain(); 00570 Thread::wait(100); 00571 fill(); 00572 Thread::wait(100); 00573 drain(); 00574 Thread::wait(100) ; 00575 fill(); 00576 Thread::wait(100); 00577 drain(); 00578 Thread::wait(100) ; 00579 fill(); 00580 Thread::wait(100); 00581 drain(); 00582 Thread::wait(100); 00583 fill(); 00584 Thread::wait(100); 00585 fill(); 00586 Thread::wait(100); 00587 fill(); 00588 Thread::wait(100); 00589 drain(); 00590 Thread::wait(100); 00591 MODE = 4; */ 00592 } 00593 void mix() { 00594 MODE = 7; 00595 p1on = false; 00596 p2on = false; 00597 p3on = false; 00598 p4on = false; 00599 p5on = false; 00600 p6on = true; 00601 p7on = false; 00602 runpump(); 00603 Thread::wait(1000); 00604 MODE = 4; 00605 00606 } 00607 void Agbuffer() { 00608 MODE = 8; 00609 p1on = true; 00610 p2on = false; 00611 p3on = false; 00612 p4on = false; 00613 p5on = false; 00614 p6on = false; 00615 p7on = false; 00616 runpump(); 00617 MODE = 4; 00618 } 00619 void Agindicator() { 00620 MODE = 9; 00621 p1on = false; 00622 p2on = true; 00623 p3on = false; 00624 p4on = false; 00625 p5on = false; 00626 p6on = false; 00627 p7on = false; 00628 runpump(); 00629 MODE = 4; 00630 } 00631 void CuIndicator() { 00632 MODE = 10; 00633 p1on = false; 00634 p2on = false; 00635 p3on = true; 00636 p4on = false; 00637 p5on = false; 00638 p6on = false; 00639 p7on = false; 00640 runpump(); 00641 MODE = 4; 00642 } 00643 void ClSoln() { 00644 MODE = 11; 00645 p1on = false; 00646 p2on = false; 00647 p3on = false; 00648 p4on = true; 00649 p5on = false; 00650 p6on = false; 00651 p7on = false; 00652 runpump(); 00653 MODE = 4; 00654 } 00655 unsigned int read() { 00656 spi.format(8,0); 00657 cs = 0; 00658 Thread::wait(200); 00659 for( int i = 3; i >= 0; i-- ) { 00660 adc.byte[i] = spi.write( 0xFF ); 00661 } 00662 cs = 1; 00663 00664 return adc.reading; 00665 00666 } 00667 unsigned int readtemp() { 00668 spi.format(8,0); 00669 Tempcs = 0; 00670 Thread::wait(200); 00671 for( int i = 3; i >= 0; i-- ) { 00672 adc.byte[i] = spi.write( 0xFF ); 00673 } 00674 Tempcs = 1; 00675 00676 return adc.reading; 00677 00678 } 00679 unsigned int readl(int take) { 00680 LED515.write(0.0); 00681 LED480.write(0.0); 00682 LEDLD = 0; 00683 LEDBL = 0; 00684 unsigned int res = 1; 00685 unsigned int resp = 0; 00686 if (take == 0) { 00687 MODE = 0; 00688 LEDCUR=0.0; 00689 Thread::wait(2000); 00690 while (resp < res) { 00691 resp = read(); 00692 res = read(); 00693 } 00694 Thread::wait(2000); 00695 unsigned int DF = read(); 00696 unsigned int basereading = 0; 00697 double i = 0.0; 00698 while (basereading <= DF) { 00699 i = i + 0.001; 00700 LED515.write(i); 00701 res = 1; 00702 resp = 0; 00703 while (resp < res) { 00704 resp = read(); 00705 res = read(); 00706 } 00707 basereading = read(); 00708 } 00709 LEDCUR = i + 0.001f; 00710 LED515.write(i + 0.001f); 00711 Thread::wait(2000); 00712 while (resp < res) { 00713 resp = read(); 00714 res = read(); 00715 } 00716 res = read(); 00717 MODE = 4; 00718 pc.printf("DARK: %d LEDCUR: %f\r\n", res, LEDCUR); 00719 00720 } 00721 if (take == 1) { 00722 MODE = 1; 00723 LED515.write(LEDCUR); 00724 while (read() <= 12000000) { 00725 LEDCUR = LEDCUR + 0.001f; 00726 LED515.write(LEDCUR); 00727 } 00728 Thread::wait(2000); 00729 while (resp < res) { 00730 resp = read(); 00731 res = read(); 00732 } 00733 res = read(); 00734 MODE = 4; 00735 pc.printf("ZERO: %d\r\n", res); 00736 } 00737 if (take == 2) { 00738 MODE = 2; 00739 LED515.write(LEDCUR); 00740 Thread::wait(2000); 00741 while (resp < res) { 00742 resp = read(); 00743 res = read(); 00744 } 00745 res = read(); 00746 MODE = 4; 00747 pc.printf("READ: %d\r\n", res); 00748 } 00749 if (take == 3) { 00750 MODE = 12; 00751 LEDLD=1; 00752 Thread::wait(2000); 00753 while (resp < res) { 00754 resp = read(); 00755 res = read(); 00756 } 00757 res = read(); 00758 LEDLD=0; 00759 MODE = 4; 00760 pc.printf("Level: %d\r\n", res); 00761 } 00762 00763 00764 /// 00765 if (take == 4) { 00766 MODE = 0; 00767 LEDCUR=0.0; 00768 Thread::wait(2000); 00769 while (resp < res) { 00770 resp = read(); 00771 res = read(); 00772 } 00773 Thread::wait(2000); 00774 unsigned int DF = read(); 00775 unsigned int basereading = 0; 00776 double i = 0.0; 00777 while (basereading <= DF) { 00778 i = i + 0.001; 00779 LED480.write(i); 00780 res = 1; 00781 resp = 0; 00782 while (resp < res) { 00783 resp = read(); 00784 res = read(); 00785 } 00786 basereading = read(); 00787 } 00788 LEDCUR = i + 0.001f; 00789 LED480.write(i + 0.001f); 00790 Thread::wait(2000); 00791 while (resp < res) { 00792 resp = read(); 00793 res = read(); 00794 } 00795 res = read(); 00796 LED480.write(0.0); 00797 MODE = 4; 00798 pc.printf("DARK: %d LEDCUR: %f\r\n", res, LEDCUR); 00799 00800 } 00801 if (take == 5) { 00802 MODE = 1; 00803 LED480.write(LEDCUR); 00804 while (read() <= 12000000) { 00805 LEDCUR = LEDCUR + 0.001f; 00806 LED480.write(LEDCUR); 00807 } 00808 Thread::wait(2000); 00809 while (resp < res) { 00810 resp = read(); 00811 res = read(); 00812 } 00813 res = read(); 00814 LED480.write(0.0); 00815 MODE = 4; 00816 pc.printf("ZERO: %d\r\n", res); 00817 } 00818 if (take == 6) { 00819 MODE = 2; 00820 LED480.write(LEDCUR); 00821 Thread::wait(2000); 00822 while (resp < res) { 00823 resp = read(); 00824 res = read(); 00825 } 00826 res = read(); 00827 LED480.write(0.0); 00828 MODE = 4; 00829 pc.printf("READ: %d\r\n", res); 00830 } 00831 /// 00832 LEDBL=1; 00833 return res; 00834 } 00835 00836 void saveit() { 00837 Thread::wait(200); 00838 get_time(&dt); 00839 Thread::wait(200); 00840 memset((char*)savereading,0,sizeof(savereading)); 00841 Thread::wait(1000); 00842 sprintf((char*)savereading, "%04d/%02d/%02d %02d:%02d:%02d,%0.1f,%0.5f,%0.1f,%0.5f,%0.5f,%0.5f,%0.5f\n", 2000 + dt.y, dt.mm, dt.d, dt.h, dt.m, dt.s, Silver, SilverRAW, Copper, CopperRAW, ((double)DARK/16777216)*3.302, ((double)ZERO/16777216)*3.302, ((double)READ/16777216)*3.302); 00843 Thread::wait(1000); 00844 00845 ress = f_open(&MyFile, "Log.csv", FA_WRITE); 00846 Thread::wait(200); 00847 if(ress!= FR_OK) { 00848 pc.printf("Failed to open file\n"); 00849 ress = f_open(&MyFile, "Log.csv", FA_CREATE_ALWAYS); 00850 if(ress!= FR_OK) { 00851 pc.printf("Failed to open file\n"); 00852 } 00853 } 00854 Thread::wait(200); 00855 f_lseek(&MyFile, f_size(&MyFile)); 00856 Thread::wait(200); 00857 ress = f_write(&MyFile, savereading, sizeof(savereading), &bytesread); 00858 Thread::wait(200); 00859 if(ress!= FR_OK) { 00860 pc.printf("Failed to write file 1\n"); 00861 MODE = 13; 00862 writefail = 1; 00863 Thread::wait(3000); 00864 } else { 00865 pc.printf("Done!\n"); 00866 MODE = 11; 00867 writefail = 0; 00868 } 00869 Thread::wait(200); 00870 f_close(&MyFile); 00871 /* Thread::wait(200); 00872 FATFS_UnLinkDriver(SDPath); */ 00873 } 00874 00875 void ReadSilver() { 00876 sprintf((char*)cyclestep, " - Ag"); 00877 wash(); 00878 //Thread::wait(30000); //to give heater time to warm water 00879 LVL = readl(3); 00880 while (LVL > 9000000) { 00881 fill(); 00882 LVL = readl(3); 00883 } 00884 Thread::wait(2000); 00885 DARK = readl(0); 00886 Thread::wait(2000); 00887 ZERO = readl(1); 00888 Agbuffer(); 00889 mix(); 00890 Agindicator(); 00891 mix(); 00892 Thread::wait(60000); 00893 sprintf((char*)cyclestep, " - Ag"); 00894 READ = readl(2); 00895 double x = abs(((float)READ-(float)DARK)/((float)ZERO-(float)DARK)); 00896 x = 2-log10(x*100); 00897 RAW = x; 00898 SilverRAW = RAW; 00899 //y = 10726x^2 – 301.98x – 5.1693 00900 x = 10726*pow(x,2) - 301.98*x - 5.1693; 00901 if (x<0.0){ x=0.0;} 00902 Silver = x; 00903 sprintf((char*)cyclestep, ""); 00904 saveit(); 00905 } 00906 void ReadCopper() { 00907 sprintf((char*)cyclestep, " - Cu"); 00908 wash(); 00909 //Thread::wait(30000); //to give heater time to warm water 00910 LVL = readl(3); 00911 while (LVL > 9000000) { 00912 fill(); 00913 LVL = readl(3); 00914 } 00915 Thread::wait(2000); 00916 DARK = readl(4); 00917 Thread::wait(2000); 00918 ZERO = readl(5); 00919 CuIndicator(); 00920 mix(); 00921 Thread::wait(60000); 00922 sprintf((char*)cyclestep, " - Cu"); 00923 READ = readl(6); 00924 double x = abs(((float)READ-(float)DARK)/((float)ZERO-(float)DARK)); 00925 x = 2-log10(x*100); 00926 RAW = x; 00927 CopperRAW = RAW; 00928 //y = 4852.2x – 9.5947 00929 x = 4852.2*x - 9.5947; 00930 if (x<0.0){ x=0.0;} 00931 Copper = x; 00932 sprintf((char*)cyclestep, ""); 00933 saveit(); 00934 } 00935 void ThermoStat() { 00936 TempCurrent = ((readtemp()/16777216.0) - 0.3756) * 660; 00937 pc.printf("%0.2f",TempCurrent); 00938 if (TempCurrent >= 41.0) { Heat = 0;} 00939 if (TempCurrent < 38.0) { Heat = 1;} 00940 } 00941 void reprime(); 00942 void takereading() { 00943 while (1) { 00944 LED515.write(0.0); 00945 LED480.write(0.0); 00946 LEDLD = 0; 00947 LEDBL = 0; 00948 LEDCUR = 0.0; 00949 if (ManMode == true) { 00950 DARK = readl(0); 00951 ZERO = readl(1); 00952 Thread::wait(5000); 00953 READ = readl(2); 00954 double x = abs(((float)READ-(float)DARK)/((float)ZERO-(float)DARK)); 00955 x = 2-log10(x*100); 00956 //x = 10.297*pow(x,2) - 21.909*x + 11.453; 00957 if (x < 0.0) { x = 0.0; } 00958 Copper = x; 00959 Silver = x; 00960 } 00961 if (ManMode == false) { 00962 ThermoStat(); 00963 if (AgMODE == true) { ReadSilver(); } 00964 if (CuMODE == true) { ReadCopper(); } 00965 drain(); 00966 for (int d6chld = 0; d6chld < d6count*56; d6chld++){ 00967 Thread::wait(60000); 00968 } 00969 if (d6count > 0) { reprime(); } 00970 } 00971 } 00972 } 00973 00974 void prime(){ 00975 MODE = 3; 00976 thread3.terminate(); 00977 pump5 = 1; 00978 for (pcount = 0; pcount < 25; pcount++) { 00979 00980 pump1 = 1; 00981 Thread::wait(200); 00982 pump1 = 0; 00983 00984 pump2 = 1; 00985 Thread::wait(200); 00986 pump2 = 0; 00987 00988 pump3 = 1; 00989 Thread::wait(200); 00990 pump3 = 0; 00991 00992 /* pump4 = 1; 00993 Thread::wait(200); 00994 pump4 = 0; */ 00995 00996 pump6 = 1; 00997 Thread::wait(200); 00998 pump6 = 0; 00999 01000 motors[1]->move(StepperMotor::BWD, STEPS_1*6.15); 01001 //motors[1]->wait_while_active(); 01002 01003 01004 } 01005 Thread::wait(5000); 01006 pump5 = 0; 01007 Thread::wait(300); 01008 if (ManMode == true) { thread2.start(runpump); } 01009 thread3.start(takereading); 01010 pcount = 0; 01011 MODE = 4; 01012 } 01013 01014 void reprime(){ 01015 MODE = 3; 01016 pump5 = 1; 01017 for (pcount = 0; pcount < 5; pcount++) { 01018 01019 pump1 = 1; 01020 Thread::wait(200); 01021 pump1 = 0; 01022 01023 pump2 = 1; 01024 Thread::wait(200); 01025 pump2 = 0; 01026 01027 pump3 = 1; 01028 Thread::wait(200); 01029 pump3 = 0; 01030 01031 /* pump4 = 1; 01032 Thread::wait(200); 01033 pump4 = 0; */ 01034 01035 pump6 = 1; 01036 Thread::wait(200); 01037 pump6 = 0; 01038 01039 } 01040 Thread::wait(2000); 01041 pump5 = 0; 01042 Thread::wait(300); 01043 pcount = 0; 01044 MODE = 4; 01045 } 01046 01047 void screencycle(); 01048 void setdatetime() { 01049 cmd[0] = Oscillator; // Aging_offset 01050 cmd[1] = 0x80 + 0x40 + 0x1; // -1ppm 01051 i2c.write(PCF85263AT_ADDR, cmd, 2); // Aging_offset 01052 01053 cmd[0] = Function; // Aging_offset 01054 cmd[1] = 0x1; // -1ppm 01055 i2c.write(PCF85263AT_ADDR, cmd, 2); // Aging_offset 01056 01057 cmd[0] = Offset; // Aging_offset 01058 cmd[1] = 60; // -1ppm 01059 i2c.write(PCF85263AT_ADDR, cmd, 2); // Aging_offse 01060 01061 01062 dt.y = 2000+(int)d3count; 01063 dt.mm = (int)d1count; 01064 dt.d = (int)d2count; 01065 dt.h = (int)d4count; 01066 dt.m = (int)d5count; 01067 dt.s = 0; 01068 set_time(&dt); 01069 01070 } 01071 void screen() { 01072 while(1) { 01073 if (p1count<0){p1count=0;} 01074 if (p2count<0){p2count=0;} 01075 if (p3count<0){p3count=0;} 01076 if (p4count<0){p4count=0;} 01077 if (p5count<0){p5count=0;} 01078 if (p6count<0){p6count=0;} 01079 if (p7count<0){p7count=0;} 01080 //pump1.pulsewidth(p1count/750000); 01081 01082 if (p1on == true || p2on == true || p3on == true || p4on == true || p5on == true || p6on == true || p7on == true) { Aon = true; } 01083 if (p1on == false && p2on == false && p3on == false && p4on == false && p5on == false && p6on == false && p7on == false) { Aon = false; } 01084 01085 01086 if (writefail == 1) { 01087 lcd.SetTextColor(LCD_COLOR_RED); 01088 lcd.FillCircle(235,175,10); 01089 } 01090 01091 //General Display 01092 lcd.SetFont(&Font16); 01093 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01094 sprintf((char*)textn1, "%0.1f/%0.5f Ag", Silver, SilverRAW); 01095 if (strcmp((char*)text1,(char*)textn1) != 0) { 01096 sprintf((char*)text1, "%0.1f/%0.5f Ag", Silver, SilverRAW); 01097 lcd.ClearStringLine(13); 01098 } 01099 lcd.DisplayStringAt(0, LINE(13), (uint8_t *)&text1, CENTER_MODE); 01100 01101 01102 lcd.SetFont(&Font16); 01103 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01104 sprintf((char*)textn4, "%0.1f/%0.5f Cu L:%d", Copper, CopperRAW, LVL); 01105 if (strcmp((char*)text4,(char*)textn4) != 0) { 01106 sprintf((char*)text4, "%0.1f/%0.5f Cu L:%d", Copper, CopperRAW, LVL); 01107 lcd.ClearStringLine(14); 01108 } 01109 lcd.DisplayStringAt(0, LINE(14), (uint8_t *)&text4, CENTER_MODE); 01110 01111 01112 01113 lcd.SetTextColor(LCD_COLOR_DARKGRAY); 01114 sprintf((char*)textn4, "RAW=%0.4f | %d | %d | %d", RAW, DARK, ZERO, READ); 01115 if (strcmp((char*)text4,(char*)textn4) != 0) { 01116 sprintf((char*)text4, "RAW=%0.4f | %d | %d | %d", RAW, DARK, ZERO, READ); 01117 lcd.ClearStringLine(15); 01118 } 01119 lcd.DisplayStringAt(0, LINE(15), (uint8_t *)&text4, CENTER_MODE); 01120 01121 sprintf((char*)textn5, "RAW Volts=%0.3f | %0.3f | %0.3f", ((double)DARK/16777216)*3.302, ((double)ZERO/16777216)*3.302, ((double)READ/16777216)*3.302); 01122 if (strcmp((char*)text5,(char*)textn5) != 0) { 01123 sprintf((char*)text5, "RAW Volts=%0.3f | %0.3f | %0.3f", ((double)DARK/16777216)*3.302, ((double)ZERO/16777216)*3.302, ((double)READ/16777216)*3.302); 01124 lcd.ClearStringLine(16); 01125 } 01126 lcd.DisplayStringAt(0, LINE(16), (uint8_t *)&text5, CENTER_MODE); 01127 01128 01129 01130 if (MODE == 0 && (strcmp((char*)text3,"Dark Reading") != 0)) { 01131 lcd.ClearStringLine(12); 01132 sprintf((char*)text3, "Dark Reading"); 01133 } 01134 if (MODE == 1 && (strcmp((char*)text3,"Zeroing") != 0)) { 01135 lcd.ClearStringLine(12); 01136 sprintf((char*)text3, "Zeroing"); 01137 } 01138 if (MODE == 2 && (strcmp((char*)text3,"Reading") != 0)) { 01139 lcd.ClearStringLine(12); 01140 sprintf((char*)text3, "Reading"); 01141 } 01142 if (MODE == 3 && (strcmp((char*)text3,"Priming") != 0)) { 01143 lcd.ClearStringLine(12); 01144 sprintf((char*)text3, "Priming"); 01145 } 01146 sprintf((char*)textn3, "Waiting%s", cyclestep); 01147 if (MODE == 4 && (strcmp((char*)text3,(char*)textn3) != 0)) { 01148 lcd.ClearStringLine(12); 01149 sprintf((char*)text3, "Waiting%s", cyclestep); 01150 } 01151 if (MODE == 5 && (strcmp((char*)text3,"Sampling") != 0)) { 01152 lcd.ClearStringLine(12); 01153 sprintf((char*)text3, "Sampling"); 01154 } 01155 if (MODE == 6 && (strcmp((char*)text3,"Washing") != 0)) { 01156 lcd.ClearStringLine(12); 01157 sprintf((char*)text3, "Washing"); 01158 } 01159 if (MODE == 7 && (strcmp((char*)text3,"Mixing") != 0)) { 01160 lcd.ClearStringLine(12); 01161 sprintf((char*)text3, "Mixing"); 01162 } 01163 if (MODE == 8 && (strcmp((char*)text3,"Ag Buffer Dosing") != 0)) { 01164 lcd.ClearStringLine(12); 01165 sprintf((char*)text3, "Ag Buffer Dosing"); 01166 } 01167 if (MODE == 9 && (strcmp((char*)text3,"Ag Indicator Dosing") != 0)) { 01168 lcd.ClearStringLine(12); 01169 sprintf((char*)text3, "Ag Indicator Dosing"); 01170 } 01171 if (MODE == 10 && (strcmp((char*)text3,"Cu Indicator Dosing") != 0)) { 01172 lcd.ClearStringLine(12); 01173 sprintf((char*)text3, "Cu Indicator Dosing"); 01174 } 01175 if (MODE == 11 && (strcmp((char*)text3,"Write Success") != 0)) { 01176 lcd.ClearStringLine(12); 01177 sprintf((char*)text3, "Write Success"); 01178 } 01179 if (MODE == 12 && (strcmp((char*)text3,"Level") != 0)) { 01180 lcd.ClearStringLine(12); 01181 sprintf((char*)text3, "Level"); 01182 } 01183 if (MODE == 13 && (strcmp((char*)text3,"Write Fail") != 0)) { 01184 lcd.ClearStringLine(12); 01185 sprintf((char*)text3, "Write Fail"); 01186 } 01187 01188 lcd.SetTextColor(LCD_COLOR_LIGHTRED); 01189 lcd.DisplayStringAt(0, LINE(12), (uint8_t *)&text3, CENTER_MODE); 01190 01191 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01192 lcd.SetFont(&Font24); 01193 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)"WOLFMAN", CENTER_MODE); 01194 01195 01196 //Prime Button 01197 lcd.SetFont(&Font12); 01198 if (pcount == 0) { 01199 lcd.SetTextColor(LCD_COLOR_GREEN); 01200 lcd.SetBackColor(LCD_COLOR_GREEN); 01201 touchbutt = DrawButton(20, 2, 50, 15); 01202 for( int i = 0 ; i < 4 ; ++i ){ primeon[ i ] = touchbutt[ i ]; } 01203 lcd.SetTextColor(LCD_COLOR_BLACK); 01204 lcd.DisplayStringAt(20, 2, (uint8_t *)"Prime", LEFT_MODE); 01205 } else { 01206 lcd.SetTextColor(LCD_COLOR_RED); 01207 lcd.SetBackColor(LCD_COLOR_RED); 01208 touchbutt = DrawButton(20, 2, 50, 15); 01209 for( int i = 0 ; i < 4 ; ++i ){ primeon[ i ] = touchbutt[ i ]; } 01210 lcd.SetTextColor(LCD_COLOR_BLACK); 01211 sprintf((char*)nus_buffer, "%d", (int)pcount); 01212 lcd.DisplayStringAt(20, 2, (uint8_t *)nus_buffer, LEFT_MODE); 01213 } 01214 01215 //Screen Mode 01216 //lcd.SetTextColor(LCD_COLOR_GREEN); 01217 //lcd.SetBackColor(LCD_COLOR_GREEN); 01218 touchbutt = DrawButton(230, 30, 50, 15); 01219 for( int i = 0 ; i < 4 ; ++i ){ screenmode[ i ] = touchbutt[ i ]; } 01220 01221 01222 //Cu Only Button 01223 lcd.SetFont(&Font12); 01224 if (CuMODE == false) { 01225 lcd.SetTextColor(LCD_COLOR_RED); 01226 lcd.SetBackColor(LCD_COLOR_RED); 01227 touchbutt = DrawButton(90, 2, 25, 15); 01228 for( int i = 0 ; i < 4 ; ++i ){ CuMODEonoff[ i ] = touchbutt[ i ]; } 01229 lcd.SetTextColor(LCD_COLOR_BLACK); 01230 lcd.DisplayStringAt(90, 2, (uint8_t *)"Cu", LEFT_MODE); 01231 } else { 01232 lcd.SetTextColor(LCD_COLOR_GREEN); 01233 lcd.SetBackColor(LCD_COLOR_GREEN); 01234 touchbutt = DrawButton(90, 2, 25, 15); 01235 for( int i = 0 ; i < 4 ; ++i ){ CuMODEonoff[ i ] = touchbutt[ i ]; } 01236 lcd.SetTextColor(LCD_COLOR_BLACK); 01237 lcd.DisplayStringAt(90, 2, (uint8_t *)"Cu", LEFT_MODE); 01238 } 01239 //Ag Only Button 01240 lcd.SetFont(&Font12); 01241 if (AgMODE == false) { 01242 lcd.SetTextColor(LCD_COLOR_RED); 01243 lcd.SetBackColor(LCD_COLOR_RED); 01244 touchbutt = DrawButton(130, 2, 25, 15); 01245 for( int i = 0 ; i < 4 ; ++i ){ AgMODEonoff[ i ] = touchbutt[ i ]; } 01246 lcd.SetTextColor(LCD_COLOR_BLACK); 01247 lcd.DisplayStringAt(130, 2, (uint8_t *)"Ag", LEFT_MODE); 01248 } else { 01249 lcd.SetTextColor(LCD_COLOR_GREEN); 01250 lcd.SetBackColor(LCD_COLOR_GREEN); 01251 touchbutt = DrawButton(130, 2, 25, 15); 01252 for( int i = 0 ; i < 4 ; ++i ){ AgMODEonoff[ i ] = touchbutt[ i ]; } 01253 lcd.SetTextColor(LCD_COLOR_BLACK); 01254 lcd.DisplayStringAt(130, 2, (uint8_t *)"Ag", LEFT_MODE); 01255 } 01256 01257 01258 01259 //Mode Button 01260 lcd.SetFont(&Font12); 01261 if (ManMode == true) { 01262 lcd.SetTextColor(LCD_COLOR_GREEN); 01263 lcd.SetBackColor(LCD_COLOR_GREEN); 01264 touchbutt = DrawButton(340, 2, 50, 15); 01265 for( int i = 0 ; i < 4 ; ++i ){ Manonoff[ i ] = touchbutt[ i ]; } 01266 lcd.SetTextColor(LCD_COLOR_BLACK); 01267 lcd.DisplayStringAt(346, 5, (uint8_t *)"Manual", LEFT_MODE); 01268 } else { 01269 lcd.SetTextColor(LCD_COLOR_RED); 01270 lcd.SetBackColor(LCD_COLOR_RED); 01271 touchbutt = DrawButton(340, 2, 50, 15); 01272 for( int i = 0 ; i < 4 ; ++i ){ Manonoff[ i ] = touchbutt[ i ]; } 01273 lcd.SetTextColor(LCD_COLOR_BLACK); 01274 lcd.DisplayStringAt(348, 5, (uint8_t *)"Auto", LEFT_MODE); 01275 } 01276 01277 01278 01279 01280 //All Button 01281 lcd.SetFont(&Font12); 01282 if (Aon == true) { 01283 lcd.SetTextColor(LCD_COLOR_GREEN); 01284 lcd.SetBackColor(LCD_COLOR_GREEN); 01285 touchbutt = DrawButton(410, 2, 50, 15); 01286 for( int i = 0 ; i < 4 ; ++i ){ Aonoff[ i ] = touchbutt[ i ]; } 01287 lcd.SetTextColor(LCD_COLOR_BLACK); 01288 lcd.DisplayStringAt(411, 5, (uint8_t *)"All ON", LEFT_MODE); 01289 } else { 01290 lcd.SetTextColor(LCD_COLOR_RED); 01291 lcd.SetBackColor(LCD_COLOR_RED); 01292 touchbutt = DrawButton(410, 2, 50, 15); 01293 for( int i = 0 ; i < 4 ; ++i ){ Aonoff[ i ] = touchbutt[ i ]; } 01294 lcd.SetTextColor(LCD_COLOR_BLACK); 01295 lcd.DisplayStringAt(410, 5, (uint8_t *)"All OFF", LEFT_MODE); 01296 } 01297 01298 //pump 1 controls 01299 lcd.SetFont(&Font16); 01300 lcd.SetBackColor(LCD_COLOR_BLACK); 01301 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01302 lcd.DisplayStringAt(10, 30, (uint8_t *)"AgBuf", LEFT_MODE); 01303 lcd.SetFont(&Font12); 01304 lcd.SetTextColor(LCD_COLOR_YELLOW); 01305 toucharrow = DrawArrow(30,50,1,2); 01306 for( int i = 0 ; i < 4 ; ++i ){ p1up[ i ] = toucharrow[ i ]; } 01307 lcd.SetTextColor(LCD_COLOR_GREEN); 01308 toucharrow = DrawArrow(30,150,2,2); 01309 for( int i = 0 ; i < 4 ; ++i ){ p1down[ i ] = toucharrow[ i ]; } 01310 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01311 sprintf((char*)nus_buffer, "%d", (int)p1count); 01312 lcd.DisplayStringAt(30, 95, (uint8_t *)nus_buffer, LEFT_MODE); 01313 if (p1on == true) { 01314 lcd.SetTextColor(LCD_COLOR_GREEN); 01315 lcd.SetBackColor(LCD_COLOR_GREEN); 01316 touchbutt = DrawButton(10, 165, 40, 15); 01317 for( int i = 0 ; i < 4 ; ++i ){ p1onoff[ i ] = touchbutt[ i ]; } 01318 lcd.SetTextColor(LCD_COLOR_BLACK); 01319 lcd.DisplayStringAt(20, 168, (uint8_t *)"ON", LEFT_MODE); 01320 } else { 01321 lcd.SetTextColor(LCD_COLOR_RED); 01322 lcd.SetBackColor(LCD_COLOR_RED); 01323 touchbutt = DrawButton(10, 165, 40, 15); 01324 for( int i = 0 ; i < 4 ; ++i ){ p1onoff[ i ] = touchbutt[ i ]; } 01325 lcd.SetTextColor(LCD_COLOR_BLACK); 01326 lcd.DisplayStringAt(20, 168, (uint8_t *)"OFF", LEFT_MODE); 01327 } 01328 01329 01330 01331 //pump 2 controls 01332 lcd.SetFont(&Font16); 01333 lcd.SetBackColor(LCD_COLOR_BLACK); 01334 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01335 lcd.DisplayStringAt(80, 30, (uint8_t *)"AgInd", LEFT_MODE); 01336 lcd.SetFont(&Font12); 01337 lcd.SetTextColor(LCD_COLOR_YELLOW); 01338 toucharrow = DrawArrow(100,50,1,2); 01339 for( int i = 0 ; i < 4 ; ++i ){ p2up[ i ] = toucharrow[ i ]; } 01340 lcd.SetTextColor(LCD_COLOR_GREEN); 01341 toucharrow = DrawArrow(100,150,2,2); 01342 for( int i = 0 ; i < 4 ; ++i ){ p2down[ i ] = toucharrow[ i ]; } 01343 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01344 sprintf((char*)nus_buffer, "%d", (int)p2count); 01345 lcd.DisplayStringAt(100, 95, (uint8_t *)nus_buffer, LEFT_MODE); 01346 if (p2on == true) { 01347 lcd.SetTextColor(LCD_COLOR_GREEN); 01348 lcd.SetBackColor(LCD_COLOR_GREEN); 01349 touchbutt = DrawButton(80, 165, 40, 15); 01350 for( int i = 0 ; i < 4 ; ++i ){ p2onoff[ i ] = touchbutt[ i ]; } 01351 lcd.SetTextColor(LCD_COLOR_BLACK); 01352 lcd.DisplayStringAt(90, 168, (uint8_t *)"ON", LEFT_MODE); 01353 } else { 01354 lcd.SetTextColor(LCD_COLOR_RED); 01355 lcd.SetBackColor(LCD_COLOR_RED); 01356 touchbutt = DrawButton(80, 165, 40, 15); 01357 for( int i = 0 ; i < 4 ; ++i ){ p2onoff[ i ] = touchbutt[ i ]; } 01358 lcd.SetTextColor(LCD_COLOR_BLACK); 01359 lcd.DisplayStringAt(90, 168, (uint8_t *)"OFF", LEFT_MODE); 01360 } 01361 01362 01363 01364 //pump 3 controls 01365 lcd.SetFont(&Font16); 01366 lcd.SetBackColor(LCD_COLOR_BLACK); 01367 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01368 lcd.DisplayStringAt(150, 30, (uint8_t *)"CuInd", LEFT_MODE); 01369 lcd.SetFont(&Font12); 01370 lcd.SetTextColor(LCD_COLOR_YELLOW); 01371 toucharrow = DrawArrow(170,50,1,2); 01372 for( int i = 0 ; i < 4 ; ++i ){ p3up[ i ] = toucharrow[ i ]; } 01373 lcd.SetTextColor(LCD_COLOR_GREEN); 01374 toucharrow = DrawArrow(170,150,2,2); 01375 for( int i = 0 ; i < 4 ; ++i ){ p3down[ i ] = toucharrow[ i ]; } 01376 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01377 sprintf((char*)nus_buffer, "%d", (int)p3count); 01378 lcd.DisplayStringAt(170, 95, (uint8_t *)nus_buffer, LEFT_MODE); 01379 if (p3on == true) { 01380 lcd.SetTextColor(LCD_COLOR_GREEN); 01381 lcd.SetBackColor(LCD_COLOR_GREEN); 01382 touchbutt = DrawButton(150, 165, 40, 15); 01383 for( int i = 0 ; i < 4 ; ++i ){ p3onoff[ i ] = touchbutt[ i ]; } 01384 lcd.SetTextColor(LCD_COLOR_BLACK); 01385 lcd.DisplayStringAt(160, 168, (uint8_t *)"ON", LEFT_MODE); 01386 } else { 01387 lcd.SetTextColor(LCD_COLOR_RED); 01388 lcd.SetBackColor(LCD_COLOR_RED); 01389 touchbutt = DrawButton(150, 165, 40, 15); 01390 for( int i = 0 ; i < 4 ; ++i ){ p3onoff[ i ] = touchbutt[ i ]; } 01391 lcd.SetTextColor(LCD_COLOR_BLACK); 01392 lcd.DisplayStringAt(160, 168, (uint8_t *)"OFF", LEFT_MODE); 01393 } 01394 01395 01396 01397 /* 01398 //pump 4 controls 01399 lcd.SetFont(&Font16); 01400 lcd.SetBackColor(LCD_COLOR_BLACK); 01401 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01402 lcd.DisplayStringAt(220, 30, (uint8_t *)"OCl", LEFT_MODE); 01403 lcd.SetFont(&Font12); 01404 lcd.SetTextColor(LCD_COLOR_YELLOW); 01405 toucharrow = DrawArrow(240,50,1,2); 01406 for( int i = 0 ; i < 4 ; ++i ){ p4up[ i ] = toucharrow[ i ]; } 01407 lcd.SetTextColor(LCD_COLOR_GREEN); 01408 toucharrow = DrawArrow(240,150,2,2); 01409 for( int i = 0 ; i < 4 ; ++i ){ p4down[ i ] = toucharrow[ i ]; } 01410 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01411 sprintf((char*)nus_buffer, "%d", (int)p4count); 01412 lcd.DisplayStringAt(240, 95, (uint8_t *)nus_buffer, LEFT_MODE); 01413 if (p4on == true) { 01414 lcd.SetTextColor(LCD_COLOR_GREEN); 01415 lcd.SetBackColor(LCD_COLOR_GREEN); 01416 touchbutt = DrawButton(220, 165, 40, 15); 01417 for( int i = 0 ; i < 4 ; ++i ){ p4onoff[ i ] = touchbutt[ i ]; } 01418 lcd.SetTextColor(LCD_COLOR_BLACK); 01419 lcd.DisplayStringAt(230, 168, (uint8_t *)"ON", LEFT_MODE); 01420 } else { 01421 lcd.SetTextColor(LCD_COLOR_RED); 01422 lcd.SetBackColor(LCD_COLOR_RED); 01423 touchbutt = DrawButton(220, 165, 40, 15); 01424 for( int i = 0 ; i < 4 ; ++i ){ p4onoff[ i ] = touchbutt[ i ]; } 01425 lcd.SetTextColor(LCD_COLOR_BLACK); 01426 lcd.DisplayStringAt(230, 168, (uint8_t *)"OFF", LEFT_MODE); 01427 } 01428 */ 01429 01430 //Solenoid 5 controls 01431 lcd.SetFont(&Font16); 01432 lcd.SetBackColor(LCD_COLOR_BLACK); 01433 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01434 lcd.DisplayStringAt(285, 30, (uint8_t *)"Drain", LEFT_MODE); 01435 lcd.SetFont(&Font12); 01436 lcd.SetTextColor(LCD_COLOR_YELLOW); 01437 toucharrow = DrawArrow(310,50,1,2); 01438 for( int i = 0 ; i < 4 ; ++i ){ p5up[ i ] = toucharrow[ i ]; } 01439 lcd.SetTextColor(LCD_COLOR_GREEN); 01440 toucharrow = DrawArrow(310,150,2,2); 01441 for( int i = 0 ; i < 4 ; ++i ){ p5down[ i ] = toucharrow[ i ]; } 01442 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01443 sprintf((char*)nus_buffer, "%d", (int)p5count); 01444 lcd.DisplayStringAt(310, 95, (uint8_t *)nus_buffer, LEFT_MODE); 01445 if (p5on == true) { 01446 lcd.SetTextColor(LCD_COLOR_GREEN); 01447 lcd.SetBackColor(LCD_COLOR_GREEN); 01448 touchbutt = DrawButton(290, 165, 40, 15); 01449 for( int i = 0 ; i < 4 ; ++i ){ p5onoff[ i ] = touchbutt[ i ]; } 01450 lcd.SetTextColor(LCD_COLOR_BLACK); 01451 lcd.DisplayStringAt(300, 168, (uint8_t *)"ON", LEFT_MODE); 01452 } else { 01453 lcd.SetTextColor(LCD_COLOR_RED); 01454 lcd.SetBackColor(LCD_COLOR_RED); 01455 touchbutt = DrawButton(290, 165, 40, 15); 01456 for( int i = 0 ; i < 4 ; ++i ){ p5onoff[ i ] = touchbutt[ i ]; } 01457 lcd.SetTextColor(LCD_COLOR_BLACK); 01458 lcd.DisplayStringAt(300, 168, (uint8_t *)"OFF", LEFT_MODE); 01459 } 01460 //Pump 6 controls 01461 lcd.SetFont(&Font16); 01462 lcd.SetBackColor(LCD_COLOR_BLACK); 01463 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01464 lcd.DisplayStringAt(360, 30, (uint8_t *)"Mix", LEFT_MODE); 01465 lcd.SetFont(&Font12); 01466 lcd.SetTextColor(LCD_COLOR_YELLOW); 01467 toucharrow = DrawArrow(380,50,1,2); 01468 for( int i = 0 ; i < 4 ; ++i ){ p6up[ i ] = toucharrow[ i ]; } 01469 lcd.SetTextColor(LCD_COLOR_GREEN); 01470 toucharrow = DrawArrow(380,150,2,2); 01471 for( int i = 0 ; i < 4 ; ++i ){ p6down[ i ] = toucharrow[ i ]; } 01472 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01473 sprintf((char*)nus_buffer, "%d", (int)p6count); 01474 lcd.DisplayStringAt(380, 95, (uint8_t *)nus_buffer, LEFT_MODE); 01475 if (p6on == true) { 01476 lcd.SetTextColor(LCD_COLOR_GREEN); 01477 lcd.SetBackColor(LCD_COLOR_GREEN); 01478 touchbutt = DrawButton(360, 165, 40, 15); 01479 for( int i = 0 ; i < 4 ; ++i ){ p6onoff[ i ] = touchbutt[ i ]; } 01480 lcd.SetTextColor(LCD_COLOR_BLACK); 01481 lcd.DisplayStringAt(370, 168, (uint8_t *)"ON", LEFT_MODE); 01482 } else { 01483 lcd.SetTextColor(LCD_COLOR_RED); 01484 lcd.SetBackColor(LCD_COLOR_RED); 01485 touchbutt = DrawButton(360, 165, 40, 15); 01486 for( int i = 0 ; i < 4 ; ++i ){ p6onoff[ i ] = touchbutt[ i ]; } 01487 lcd.SetTextColor(LCD_COLOR_BLACK); 01488 lcd.DisplayStringAt(370, 168, (uint8_t *)"OFF", LEFT_MODE); 01489 } 01490 //Pump 7 controls 01491 lcd.SetFont(&Font16); 01492 lcd.SetBackColor(LCD_COLOR_BLACK); 01493 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01494 lcd.DisplayStringAt(430, 30, (uint8_t *)"Fill", LEFT_MODE); 01495 lcd.SetFont(&Font12); 01496 lcd.SetTextColor(LCD_COLOR_YELLOW); 01497 toucharrow = DrawArrow(450,50,1,2); 01498 for( int i = 0 ; i < 4 ; ++i ){ p7up[ i ] = toucharrow[ i ]; } 01499 lcd.SetTextColor(LCD_COLOR_GREEN); 01500 toucharrow = DrawArrow(450,150,2,2); 01501 for( int i = 0 ; i < 4 ; ++i ){ p7down[ i ] = toucharrow[ i ]; } 01502 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01503 sprintf((char*)nus_buffer, "%d", (int)p7count); 01504 lcd.DisplayStringAt(450, 95, (uint8_t *)nus_buffer, LEFT_MODE); 01505 if (p7on == true) { 01506 lcd.SetTextColor(LCD_COLOR_GREEN); 01507 lcd.SetBackColor(LCD_COLOR_GREEN); 01508 touchbutt = DrawButton(430, 165, 40, 15); 01509 for( int i = 0 ; i < 4 ; ++i ){ p7onoff[ i ] = touchbutt[ i ]; } 01510 lcd.SetTextColor(LCD_COLOR_BLACK); 01511 lcd.DisplayStringAt(440, 168, (uint8_t *)"ON", LEFT_MODE); 01512 } else { 01513 lcd.SetTextColor(LCD_COLOR_RED); 01514 lcd.SetBackColor(LCD_COLOR_RED); 01515 touchbutt = DrawButton(430, 165, 40, 15); 01516 for( int i = 0 ; i < 4 ; ++i ){ p7onoff[ i ] = touchbutt[ i ]; } 01517 lcd.SetTextColor(LCD_COLOR_BLACK); 01518 lcd.DisplayStringAt(440, 168, (uint8_t *)"OFF", LEFT_MODE); 01519 } 01520 01521 lcd.SetBackColor(LCD_COLOR_BLACK); 01522 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01523 ts.GetState(&TS_State); 01524 if (TS_State.touchDetected) { 01525 // Clear lines corresponding to old touches coordinates 01526 if (TS_State.touchDetected < prev_nb_touches) { 01527 for (idx = (TS_State.touchDetected + 1); idx <= 5; idx++) { 01528 lcd.ClearStringLine(idx); 01529 } 01530 } 01531 prev_nb_touches = TS_State.touchDetected; 01532 01533 cleared = 0; 01534 01535 /* sprintf((char*)text, "Touches: %d", TS_State.touchDetected); 01536 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); */ 01537 01538 01539 01540 for (idx = 0; idx < TS_State.touchDetected; idx++) { 01541 x = TS_State.touchX[idx]; 01542 y = TS_State.touchY[idx]; 01543 double touchsensativity = 0.075; //(0.055 originaly) 01544 01545 01546 if (( x >= primeon[0] && x <= primeon[1] ) && (y >= primeon[2] && y <= primeon[3])) { 01547 thread2.terminate(); 01548 if (pcount != 0) { 01549 pcount = 0; 01550 thread2.start(takereading); 01551 } 01552 else { 01553 ManMode = false; 01554 Aon = false; 01555 p1on = false; 01556 p2on = false; 01557 p3on = false; 01558 p4on = false; 01559 p5on = false; 01560 p6on = false; 01561 p7on = false; 01562 thread2.start(prime); 01563 } 01564 Thread::wait(300); 01565 } 01566 01567 01568 if (( x >= Manonoff[0] && x <= Manonoff[1] ) && (y >= Manonoff[2] && y <= Manonoff[3])){ 01569 ManMode = !ManMode; 01570 //motors[1]->wait_while_active(); 01571 if (ManMode == true) { 01572 thread2.terminate(); 01573 p1on = false; 01574 p2on = false; 01575 p3on = false; 01576 p4on = false; 01577 p5on = false; 01578 p6on = false; 01579 p7on = false; 01580 thread2.start(runpump); 01581 thread3.terminate(); 01582 thread3.start(takereading); 01583 } else { 01584 thread2.terminate(); 01585 thread3.terminate(); 01586 thread3.start(takereading); 01587 } 01588 Thread::wait(200); 01589 } 01590 01591 if (( x >= CuMODEonoff[0] && x <= CuMODEonoff[1] ) && (y >= CuMODEonoff[2] && y <= CuMODEonoff[3])){ CuMODE = !CuMODE; } 01592 if (( x >= AgMODEonoff[0] && x <= AgMODEonoff[1] ) && (y >= AgMODEonoff[2] && y <= AgMODEonoff[3])){ AgMODE = !AgMODE; } 01593 01594 if (( x >= screenmode[0] && x <= screenmode[1] ) && (y >= screenmode[2] && y <= screenmode[3])){ 01595 screenstate = screenstate + 1; 01596 if (screenstate > 2){ screenstate = 0; } 01597 Thread::wait(200); 01598 EE_WriteVariable(VirtAddVarTab[7], (uint16_t)screenstate); 01599 screencycle(); 01600 return; 01601 } 01602 01603 01604 if (( x >= Aonoff[0] && x <= Aonoff[1] ) && (y >= Aonoff[2] && y <= Aonoff[3])){ 01605 Aon = !Aon; 01606 if (Aon == true) { 01607 p1on = true; 01608 p2on = true; 01609 p3on = true; 01610 p4on = true; 01611 p5on = true; 01612 p6on = true; 01613 p7on = true; 01614 } else { 01615 p1on = false; 01616 p2on = false; 01617 p3on = false; 01618 p4on = false; 01619 p5on = false; 01620 p6on = false; 01621 p7on = false; 01622 } 01623 Thread::wait(200); 01624 //EE_WriteVariable(VirtAddVarTab[0], (uint16_t)p1count); 01625 } 01626 01627 //pump1 01628 if (( x >= p1up[0] && x <= p1up[1] ) && (y >= p1up[2] && y <= p1up[3])){ 01629 p1count = p1count + touchsensativity; 01630 Thread::wait(2); 01631 EE_WriteVariable(VirtAddVarTab[0], (uint16_t)p1count); 01632 01633 } 01634 if (( x >= p1down[0] && x <= p1down[1] ) && (y >= p1down[2] && y <= p1down[3])){ 01635 p1count = p1count - touchsensativity; 01636 Thread::wait(2); 01637 EE_WriteVariable(VirtAddVarTab[0], (uint16_t)p1count); 01638 } 01639 if (( x >= p1onoff[0] && x <= p1onoff[1] ) && (y >= p1onoff[2] && y <= p1onoff[3])){ 01640 p1on = !p1on; 01641 Thread::wait(200); 01642 } 01643 //pump2 01644 if (( x >= p2up[0] && x <= p2up[1] ) && (y >= p2up[2] && y <= p2up[3])){ 01645 p2count = p2count + touchsensativity; 01646 Thread::wait(2); 01647 EE_WriteVariable(VirtAddVarTab[1], (uint16_t)p2count); 01648 01649 } 01650 if (( x >= p2down[0] && x <= p2down[1] ) && (y >= p2down[2] && y <= p2down[3])){ 01651 p2count = p2count - touchsensativity; 01652 Thread::wait(2); 01653 EE_WriteVariable(VirtAddVarTab[1], (uint16_t)p2count); 01654 } 01655 if (( x >= p2onoff[0] && x <= p2onoff[1] ) && (y >= p2onoff[2] && y <= p2onoff[3])){ 01656 p2on = !p2on; 01657 Thread::wait(200); 01658 } 01659 //pump3 01660 if (( x >= p3up[0] && x <= p3up[1] ) && (y >= p3up[2] && y <= p3up[3])){ 01661 p3count = p3count + touchsensativity; 01662 Thread::wait(2); 01663 EE_WriteVariable(VirtAddVarTab[2], (uint16_t)p3count); 01664 01665 } 01666 if (( x >= p3down[0] && x <= p3down[1] ) && (y >= p3down[2] && y <= p3down[3])){ 01667 p3count = p3count - touchsensativity; 01668 Thread::wait(2); 01669 EE_WriteVariable(VirtAddVarTab[2], (uint16_t)p3count); 01670 } 01671 if (( x >= p3onoff[0] && x <= p3onoff[1] ) && (y >= p3onoff[2] && y <= p3onoff[3])){ 01672 p3on = !p3on; 01673 Thread::wait(200); 01674 } 01675 /* //pump4 01676 if (( x >= p4up[0] && x <= p4up[1] ) && (y >= p4up[2] && y <= p4up[3])){ 01677 p4count = p4count + touchsensativity; 01678 Thread::wait(2); 01679 EE_WriteVariable(VirtAddVarTab[3], (uint16_t)p4count); 01680 01681 } 01682 if (( x >= p4down[0] && x <= p4down[1] ) && (y >= p4down[2] && y <= p4down[3])){ 01683 p4count = p4count - touchsensativity; 01684 Thread::wait(2); 01685 EE_WriteVariable(VirtAddVarTab[3], (uint16_t)p4count); 01686 } 01687 if (( x >= p4onoff[0] && x <= p4onoff[1] ) && (y >= p4onoff[2] && y <= p4onoff[3])){ 01688 p4on = !p4on; 01689 Thread::wait(200); 01690 } */ 01691 //Sole5 01692 if (( x >= p5up[0] && x <= p5up[1] ) && (y >= p5up[2] && y <= p5up[3])){ 01693 p5count = p5count + touchsensativity; 01694 Thread::wait(2); 01695 EE_WriteVariable(VirtAddVarTab[4], (uint16_t)p5count); 01696 01697 } 01698 if (( x >= p5down[0] && x <= p5down[1] ) && (y >= p5down[2] && y <= p5down[3])){ 01699 p5count = p5count - touchsensativity; 01700 Thread::wait(2); 01701 EE_WriteVariable(VirtAddVarTab[4], (uint16_t)p5count); 01702 } 01703 if (( x >= p5onoff[0] && x <= p5onoff[1] ) && (y >= p5onoff[2] && y <= p5onoff[3])){ 01704 p5on = !p5on; 01705 Thread::wait(200); 01706 } 01707 //Pump6 01708 if (( x >= p6up[0] && x <= p6up[1] ) && (y >= p6up[2] && y <= p6up[3])){ 01709 p6count = p6count + touchsensativity; 01710 Thread::wait(2); 01711 EE_WriteVariable(VirtAddVarTab[5], (uint16_t)p6count); 01712 01713 } 01714 if (( x >= p6down[0] && x <= p6down[1] ) && (y >= p6down[2] && y <= p6down[3])){ 01715 p6count = p6count - touchsensativity; 01716 Thread::wait(2); 01717 EE_WriteVariable(VirtAddVarTab[5], (uint16_t)p6count); 01718 } 01719 if (( x >= p6onoff[0] && x <= p6onoff[1] ) && (y >= p6onoff[2] && y <= p6onoff[3])){ 01720 p6on = !p6on; 01721 Thread::wait(200); 01722 } 01723 //Pump7 01724 if (( x >= p7up[0] && x <= p7up[1] ) && (y >= p7up[2] && y <= p7up[3])){ 01725 p7count = p7count + touchsensativity; 01726 Thread::wait(2); 01727 EE_WriteVariable(VirtAddVarTab[6], (uint16_t)p7count); 01728 01729 } 01730 if (( x >= p7down[0] && x <= p7down[1] ) && (y >= p7down[2] && y <= p7down[3])){ 01731 p7count = p7count - touchsensativity; 01732 Thread::wait(2); 01733 EE_WriteVariable(VirtAddVarTab[6], (uint16_t)p7count); 01734 } 01735 if (( x >= p7onoff[0] && x <= p7onoff[1] ) && (y >= p7onoff[2] && y <= p7onoff[3])){ 01736 p7on = !p7on; 01737 Thread::wait(200); 01738 } 01739 01740 /* sprintf((char*)text, "Touch %d: x=%d y=%d ", idx+1, x, y); 01741 lcd.DisplayStringAt(0, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); */ 01742 } 01743 lcd.DrawPixel(TS_State.touchX[0], TS_State.touchY[0], LCD_COLOR_ORANGE); 01744 } else { 01745 if (!cleared) { 01746 lcd.Clear(LCD_COLOR_BLACK); 01747 /* sprintf((char*)text, "Touches: 0"); 01748 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); */ 01749 cleared = 1; 01750 } 01751 } 01752 01753 } 01754 } 01755 01756 //////////// 01757 void screen2() { 01758 while(1) { 01759 if (p1count<0){p1count=0;} 01760 if (p2count<0){p2count=0;} 01761 if (p3count<0){p3count=0;} 01762 if (p4count<0){p4count=0;} 01763 if (p5count<0){p5count=0;} 01764 if (p6count<0){p6count=0;} 01765 if (p7count<0){p7count=0;} 01766 //pump1.pulsewidth(p1count/750000); 01767 01768 if (p1on == true || p2on == true || p3on == true || p4on == true || p5on == true || p6on == true || p7on == true) { Aon = true; } 01769 if (p1on == false && p2on == false && p3on == false && p4on == false && p5on == false && p6on == false && p7on == false) { Aon = false; } 01770 01771 if (writefail == 1) { 01772 lcd.SetTextColor(LCD_COLOR_RED); 01773 lcd.FillCircle(235,190,10); 01774 } 01775 01776 //General Display 01777 lcd.SetFont(&Font20); 01778 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01779 sprintf((char*)textn1, "%0.1f ppb Ag+", Silver); 01780 /* if (strcmp((char*)text1,(char*)textn1) != 0) { 01781 sprintf((char*)text1, "%0.1f ppm Ag+", Silver); 01782 lcd.ClearStringLine(4); 01783 } */ 01784 lcd.DisplayStringAt(0, LINE(4), (uint8_t *)&textn1, CENTER_MODE); 01785 01786 lcd.SetFont(&Font20); 01787 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01788 sprintf((char*)textn1, "%0.1f ppb Cu2+", Copper); 01789 /* if (strcmp((char*)text1,(char*)textn1) != 0) { 01790 sprintf((char*)text1, "%0.1f ppm Cu2+", Copper); 01791 lcd.ClearStringLine(9); 01792 } */ 01793 lcd.DisplayStringAt(0, LINE(7), (uint8_t *)&textn1, CENTER_MODE); 01794 01795 01796 01797 01798 lcd.SetFont(&Font16); 01799 if (MODE == 0 && (strcmp((char*)text3,"Dark Reading") != 0)) { 01800 lcd.ClearStringLine(15); 01801 sprintf((char*)text3, "Dark Reading"); 01802 } 01803 if (MODE == 1 && (strcmp((char*)text3,"Zeroing") != 0)) { 01804 lcd.ClearStringLine(15); 01805 sprintf((char*)text3, "Zeroing"); 01806 } 01807 if (MODE == 2 && (strcmp((char*)text3,"Reading") != 0)) { 01808 lcd.ClearStringLine(15); 01809 sprintf((char*)text3, "Reading"); 01810 } 01811 if (MODE == 3 && (strcmp((char*)text3,"Priming") != 0)) { 01812 lcd.ClearStringLine(15); 01813 sprintf((char*)text3, "Priming"); 01814 } 01815 sprintf((char*)textn3, "Waiting%s", cyclestep); 01816 if (MODE == 4 && (strcmp((char*)text3,(char*)textn3) != 0)) { 01817 lcd.ClearStringLine(15); 01818 sprintf((char*)text3, "Waiting%s", cyclestep); 01819 } 01820 if (MODE == 5 && (strcmp((char*)text3,"Sampling") != 0)) { 01821 lcd.ClearStringLine(15); 01822 sprintf((char*)text3, "Sampling"); 01823 } 01824 if (MODE == 6 && (strcmp((char*)text3,"Washing") != 0)) { 01825 lcd.ClearStringLine(15); 01826 sprintf((char*)text3, "Washing"); 01827 } 01828 if (MODE == 7 && (strcmp((char*)text3,"Mixing") != 0)) { 01829 lcd.ClearStringLine(15); 01830 sprintf((char*)text3, "Mixing"); 01831 } 01832 if (MODE == 8 && (strcmp((char*)text3,"Ag Buffer Dosing") != 0)) { 01833 lcd.ClearStringLine(15); 01834 sprintf((char*)text3, "Ag Buffer Dosing"); 01835 } 01836 if (MODE == 9 && (strcmp((char*)text3,"Ag Indicator Dosing") != 0)) { 01837 lcd.ClearStringLine(15); 01838 sprintf((char*)text3, "Ag Indicator Dosing"); 01839 } 01840 if (MODE == 10 && (strcmp((char*)text3,"Cu Indicator Dosing") != 0)) { 01841 lcd.ClearStringLine(15); 01842 sprintf((char*)text3, "Cu Indicator Dosing"); 01843 } 01844 if (MODE == 11 && (strcmp((char*)text3,"Write Success") != 0)) { 01845 lcd.ClearStringLine(15); 01846 sprintf((char*)text3, "Write Success"); 01847 } 01848 if (MODE == 12 && (strcmp((char*)text3,"Level") != 0)) { 01849 lcd.ClearStringLine(15); 01850 sprintf((char*)text3, "Level"); 01851 } 01852 if (MODE == 13 && (strcmp((char*)text3,"Write Fail") != 0)) { 01853 lcd.ClearStringLine(15); 01854 sprintf((char*)text3, "Write Fail"); 01855 } 01856 01857 lcd.SetTextColor(LCD_COLOR_LIGHTRED); 01858 lcd.DisplayStringAt(0, LINE(15), (uint8_t *)&text3, CENTER_MODE); 01859 01860 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01861 lcd.SetFont(&Font24); 01862 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)"Cu/Ag Ion Monitor", CENTER_MODE); 01863 01864 01865 //Prime Button 01866 lcd.SetFont(&Font12); 01867 if (pcount == 0) { 01868 lcd.SetTextColor(LCD_COLOR_GREEN); 01869 lcd.SetBackColor(LCD_COLOR_GREEN); 01870 touchbutt = DrawButton(20, 2, 50, 15); 01871 for( int i = 0 ; i < 4 ; ++i ){ primeon[ i ] = touchbutt[ i ]; } 01872 lcd.SetTextColor(LCD_COLOR_BLACK); 01873 lcd.DisplayStringAt(20, 2, (uint8_t *)"Prime", LEFT_MODE); 01874 } else { 01875 lcd.SetTextColor(LCD_COLOR_RED); 01876 lcd.SetBackColor(LCD_COLOR_RED); 01877 touchbutt = DrawButton(20, 2, 50, 15); 01878 for( int i = 0 ; i < 4 ; ++i ){ primeon[ i ] = touchbutt[ i ]; } 01879 lcd.SetTextColor(LCD_COLOR_BLACK); 01880 sprintf((char*)nus_buffer, "%d", (int)pcount); 01881 lcd.DisplayStringAt(20, 2, (uint8_t *)nus_buffer, LEFT_MODE); 01882 } 01883 01884 01885 //Screen Mode 01886 //lcd.SetTextColor(LCD_COLOR_GREEN); 01887 //lcd.SetBackColor(LCD_COLOR_GREEN); 01888 touchbutt = DrawButton(230, 30, 50, 15); 01889 for( int i = 0 ; i < 4 ; ++i ){ screenmode[ i ] = touchbutt[ i ]; } 01890 01891 01892 01893 01894 lcd.SetBackColor(LCD_COLOR_BLACK); 01895 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 01896 ts.GetState(&TS_State); 01897 if (TS_State.touchDetected) { 01898 // Clear lines corresponding to old touches coordinates 01899 if (TS_State.touchDetected < prev_nb_touches) { 01900 for (idx = (TS_State.touchDetected + 1); idx <= 5; idx++) { 01901 lcd.ClearStringLine(idx); 01902 } 01903 } 01904 prev_nb_touches = TS_State.touchDetected; 01905 01906 cleared = 0; 01907 01908 /* sprintf((char*)text, "Touches: %d", TS_State.touchDetected); 01909 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); */ 01910 01911 01912 01913 for (idx = 0; idx < TS_State.touchDetected; idx++) { 01914 x = TS_State.touchX[idx]; 01915 y = TS_State.touchY[idx]; 01916 double touchsensativity = 0.075; //(0.055 originaly) 01917 01918 01919 if (( x >= primeon[0] && x <= primeon[1] ) && (y >= primeon[2] && y <= primeon[3])) { 01920 thread2.terminate(); 01921 if (pcount != 0) { 01922 pcount = 0; 01923 thread2.start(takereading); 01924 } 01925 else { 01926 ManMode = false; 01927 Aon = false; 01928 p1on = false; 01929 p2on = false; 01930 p3on = false; 01931 p4on = false; 01932 p5on = false; 01933 p6on = false; 01934 p7on = false; 01935 thread2.start(prime); 01936 } 01937 Thread::wait(300); 01938 } 01939 01940 01941 if (( x >= Manonoff[0] && x <= Manonoff[1] ) && (y >= Manonoff[2] && y <= Manonoff[3])){ 01942 ManMode = !ManMode; 01943 //motors[1]->wait_while_active(); 01944 if (ManMode == true) { 01945 thread2.terminate(); 01946 p1on = false; 01947 p2on = false; 01948 p3on = false; 01949 p4on = false; 01950 p5on = false; 01951 p6on = false; 01952 p7on = false; 01953 thread2.start(runpump); 01954 thread3.terminate(); 01955 thread3.start(takereading); 01956 } else { 01957 thread2.terminate(); 01958 thread3.terminate(); 01959 thread3.start(takereading); 01960 } 01961 Thread::wait(200); 01962 } 01963 01964 if (( x >= CuMODEonoff[0] && x <= CuMODEonoff[1] ) && (y >= CuMODEonoff[2] && y <= CuMODEonoff[3])){ CuMODE = !CuMODE; } 01965 if (( x >= AgMODEonoff[0] && x <= AgMODEonoff[1] ) && (y >= AgMODEonoff[2] && y <= AgMODEonoff[3])){ AgMODE = !AgMODE; } 01966 01967 if (( x >= screenmode[0] && x <= screenmode[1] ) && (y >= screenmode[2] && y <= screenmode[3])){ 01968 screenstate = screenstate + 1; 01969 if (screenstate > 2){ screenstate = 0; } 01970 Thread::wait(200); 01971 EE_WriteVariable(VirtAddVarTab[7], (uint16_t)screenstate); 01972 screencycle(); 01973 return; 01974 } 01975 01976 01977 if (( x >= Aonoff[0] && x <= Aonoff[1] ) && (y >= Aonoff[2] && y <= Aonoff[3])){ 01978 Aon = !Aon; 01979 if (Aon == true) { 01980 p1on = true; 01981 p2on = true; 01982 p3on = true; 01983 p4on = true; 01984 p5on = true; 01985 p6on = true; 01986 p7on = true; 01987 } else { 01988 p1on = false; 01989 p2on = false; 01990 p3on = false; 01991 p4on = false; 01992 p5on = false; 01993 p6on = false; 01994 p7on = false; 01995 } 01996 Thread::wait(200); 01997 //EE_WriteVariable(VirtAddVarTab[0], (uint16_t)p1count); 01998 } 01999 02000 02001 02002 /* sprintf((char*)text, "Touch %d: x=%d y=%d ", idx+1, x, y); 02003 lcd.DisplayStringAt(0, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); */ 02004 } 02005 lcd.DrawPixel(TS_State.touchX[0], TS_State.touchY[0], LCD_COLOR_ORANGE); 02006 } else { 02007 if (!cleared) { 02008 lcd.Clear(LCD_COLOR_BLACK); 02009 /* sprintf((char*)text, "Touches: 0"); 02010 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); */ 02011 cleared = 1; 02012 } 02013 } 02014 02015 } 02016 } 02017 02018 void screen3() { 02019 while(1) { 02020 if (d1count<0){d1count=0;} 02021 if (d2count<0){d2count=0;} 02022 if (d3count<0){d3count=0;} 02023 if (d4count<0){d4count=0;} 02024 if (d5count<0){d5count=0;} 02025 if (d6count<0){d6count=0;} 02026 02027 02028 02029 02030 /* //Prime Button 02031 lcd.SetFont(&Font12); 02032 if (pcount == 0) { 02033 lcd.SetTextColor(LCD_COLOR_GREEN); 02034 lcd.SetBackColor(LCD_COLOR_GREEN); 02035 touchbutt = DrawButton(20, 2, 50, 15); 02036 for( int i = 0 ; i < 4 ; ++i ){ primeon[ i ] = touchbutt[ i ]; } 02037 lcd.SetTextColor(LCD_COLOR_BLACK); 02038 lcd.DisplayStringAt(20, 2, (uint8_t *)"Prime", LEFT_MODE); 02039 } else { 02040 lcd.SetTextColor(LCD_COLOR_RED); 02041 lcd.SetBackColor(LCD_COLOR_RED); 02042 touchbutt = DrawButton(20, 2, 50, 15); 02043 for( int i = 0 ; i < 4 ; ++i ){ primeon[ i ] = touchbutt[ i ]; } 02044 lcd.SetTextColor(LCD_COLOR_BLACK); 02045 sprintf((char*)nus_buffer, "%d", (int)pcount); 02046 lcd.DisplayStringAt(20, 2, (uint8_t *)nus_buffer, LEFT_MODE); 02047 } */ 02048 02049 //Screen Mode 02050 lcd.SetTextColor(LCD_COLOR_GREEN); 02051 lcd.SetBackColor(LCD_COLOR_GREEN); 02052 touchbutt = DrawButton(230, 2, 50, 15); 02053 for( int i = 0 ; i < 4 ; ++i ){ screenmode[ i ] = touchbutt[ i ]; } 02054 lcd.SetTextColor(LCD_COLOR_BLACK); 02055 lcd.DisplayStringAt(230, 2, (uint8_t *)"Exit", LEFT_MODE); 02056 02057 02058 02059 //Save 02060 lcd.SetFont(&Font12); 02061 lcd.SetTextColor(LCD_COLOR_GREEN); 02062 lcd.SetBackColor(LCD_COLOR_GREEN); 02063 touchbutt = DrawButton(90, 2, 50, 15); 02064 for( int i = 0 ; i < 4 ; ++i ){ savedate[ i ] = touchbutt[ i ]; } 02065 lcd.SetTextColor(LCD_COLOR_BLACK); 02066 lcd.DisplayStringAt(90, 2, (uint8_t *)"Save", LEFT_MODE); 02067 02068 02069 get_time(&dt); 02070 sprintf((char*)currenttime, "%02d/%02d/%04d %02d:%02d:%02d\n", dt.mm, dt.d, 2000 + dt.y, dt.h, dt.m, dt.s); 02071 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02072 lcd.SetFont(&Font12); 02073 lcd.DisplayStringAt(0, LINE(13), (uint8_t *)currenttime, CENTER_MODE); 02074 02075 02076 02077 02078 //Month 02079 lcd.SetFont(&Font16); 02080 lcd.SetBackColor(LCD_COLOR_BLACK); 02081 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02082 lcd.DisplayStringAt(10, 30, (uint8_t *)"Month", LEFT_MODE); 02083 lcd.SetFont(&Font12); 02084 lcd.SetTextColor(LCD_COLOR_YELLOW); 02085 toucharrow = DrawArrow(30,50,1,2); 02086 for( int i = 0 ; i < 4 ; ++i ){ d1up[ i ] = toucharrow[ i ]; } 02087 lcd.SetTextColor(LCD_COLOR_GREEN); 02088 toucharrow = DrawArrow(30,150,2,2); 02089 for( int i = 0 ; i < 4 ; ++i ){ d1down[ i ] = toucharrow[ i ]; } 02090 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02091 sprintf((char*)nus_buffer, "%d", (int)d1count); 02092 lcd.DisplayStringAt(30, 95, (uint8_t *)nus_buffer, LEFT_MODE); 02093 02094 02095 02096 //Day 02097 lcd.SetFont(&Font16); 02098 lcd.SetBackColor(LCD_COLOR_BLACK); 02099 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02100 lcd.DisplayStringAt(80, 30, (uint8_t *)"Day", LEFT_MODE); 02101 lcd.SetFont(&Font12); 02102 lcd.SetTextColor(LCD_COLOR_YELLOW); 02103 toucharrow = DrawArrow(100,50,1,2); 02104 for( int i = 0 ; i < 4 ; ++i ){ d2up[ i ] = toucharrow[ i ]; } 02105 lcd.SetTextColor(LCD_COLOR_GREEN); 02106 toucharrow = DrawArrow(100,150,2,2); 02107 for( int i = 0 ; i < 4 ; ++i ){ d2down[ i ] = toucharrow[ i ]; } 02108 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02109 sprintf((char*)nus_buffer, "%d", (int)d2count); 02110 lcd.DisplayStringAt(100, 95, (uint8_t *)nus_buffer, LEFT_MODE); 02111 02112 02113 02114 //Year 02115 lcd.SetFont(&Font16); 02116 lcd.SetBackColor(LCD_COLOR_BLACK); 02117 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02118 lcd.DisplayStringAt(150, 30, (uint8_t *)"Year", LEFT_MODE); 02119 lcd.SetFont(&Font12); 02120 lcd.SetTextColor(LCD_COLOR_YELLOW); 02121 toucharrow = DrawArrow(170,50,1,2); 02122 for( int i = 0 ; i < 4 ; ++i ){ d3up[ i ] = toucharrow[ i ]; } 02123 lcd.SetTextColor(LCD_COLOR_GREEN); 02124 toucharrow = DrawArrow(170,150,2,2); 02125 for( int i = 0 ; i < 4 ; ++i ){ d3down[ i ] = toucharrow[ i ]; } 02126 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02127 sprintf((char*)nus_buffer, "%d", (int)d3count); 02128 lcd.DisplayStringAt(170, 95, (uint8_t *)nus_buffer, LEFT_MODE); 02129 02130 02131 02132 02133 02134 //Hour 02135 lcd.SetFont(&Font16); 02136 lcd.SetBackColor(LCD_COLOR_BLACK); 02137 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02138 lcd.DisplayStringAt(220, 30, (uint8_t *)"Hour", LEFT_MODE); 02139 lcd.SetFont(&Font12); 02140 lcd.SetTextColor(LCD_COLOR_YELLOW); 02141 toucharrow = DrawArrow(240,50,1,2); 02142 for( int i = 0 ; i < 4 ; ++i ){ d4up[ i ] = toucharrow[ i ]; } 02143 lcd.SetTextColor(LCD_COLOR_GREEN); 02144 toucharrow = DrawArrow(240,150,2,2); 02145 for( int i = 0 ; i < 4 ; ++i ){ d4down[ i ] = toucharrow[ i ]; } 02146 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02147 sprintf((char*)nus_buffer, "%d", (int)d4count); 02148 lcd.DisplayStringAt(240, 95, (uint8_t *)nus_buffer, LEFT_MODE); 02149 02150 02151 02152 //Min 02153 lcd.SetFont(&Font16); 02154 lcd.SetBackColor(LCD_COLOR_BLACK); 02155 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02156 lcd.DisplayStringAt(285, 30, (uint8_t *)"Min", LEFT_MODE); 02157 lcd.SetFont(&Font12); 02158 lcd.SetTextColor(LCD_COLOR_YELLOW); 02159 toucharrow = DrawArrow(310,50,1,2); 02160 for( int i = 0 ; i < 4 ; ++i ){ d5up[ i ] = toucharrow[ i ]; } 02161 lcd.SetTextColor(LCD_COLOR_GREEN); 02162 toucharrow = DrawArrow(310,150,2,2); 02163 for( int i = 0 ; i < 4 ; ++i ){ d5down[ i ] = toucharrow[ i ]; } 02164 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02165 sprintf((char*)nus_buffer, "%d", (int)d5count); 02166 lcd.DisplayStringAt(310, 95, (uint8_t *)nus_buffer, LEFT_MODE); 02167 02168 02169 //Interval 02170 lcd.SetFont(&Font16); 02171 lcd.SetBackColor(LCD_COLOR_BLACK); 02172 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02173 lcd.DisplayStringAt(360, 30, (uint8_t *)"Interval(h)", LEFT_MODE); 02174 lcd.SetFont(&Font12); 02175 lcd.SetTextColor(LCD_COLOR_YELLOW); 02176 toucharrow = DrawArrow(380,50,1,2); 02177 for( int i = 0 ; i < 4 ; ++i ){ d6up[ i ] = toucharrow[ i ]; } 02178 lcd.SetTextColor(LCD_COLOR_GREEN); 02179 toucharrow = DrawArrow(380,150,2,2); 02180 for( int i = 0 ; i < 4 ; ++i ){ d6down[ i ] = toucharrow[ i ]; } 02181 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02182 sprintf((char*)nus_buffer, "%d", (int)d6count); 02183 lcd.DisplayStringAt(380, 95, (uint8_t *)nus_buffer, LEFT_MODE); 02184 02185 02186 02187 lcd.SetBackColor(LCD_COLOR_BLACK); 02188 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE); 02189 ts.GetState(&TS_State); 02190 if (TS_State.touchDetected) { 02191 // Clear lines corresponding to old touches coordinates 02192 if (TS_State.touchDetected < prev_nb_touches) { 02193 for (idx = (TS_State.touchDetected + 1); idx <= 5; idx++) { 02194 lcd.ClearStringLine(idx); 02195 } 02196 } 02197 prev_nb_touches = TS_State.touchDetected; 02198 02199 cleared = 0; 02200 02201 /* sprintf((char*)text, "Touches: %d", TS_State.touchDetected); 02202 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); */ 02203 02204 02205 02206 for (idx = 0; idx < TS_State.touchDetected; idx++) { 02207 x = TS_State.touchX[idx]; 02208 y = TS_State.touchY[idx]; 02209 double touchsensativity = 0.075; //(0.055 originaly) 02210 02211 02212 02213 02214 if (( x >= screenmode[0] && x <= screenmode[1] ) && (y >= screenmode[2] && y <= screenmode[3])){ 02215 screenstate = screenstate + 1; 02216 if (screenstate > 2){ screenstate = 0; } 02217 Thread::wait(200); 02218 EE_WriteVariable(VirtAddVarTab[7], (uint16_t)screenstate); 02219 screencycle(); 02220 return; 02221 } 02222 02223 if (( x >= savedate[0] && x <= savedate[1] ) && (y >= savedate[2] && y <= savedate[3])){ setdatetime(); } 02224 02225 //Month 02226 if (( x >= d1up[0] && x <= d1up[1] ) && (y >= d1up[2] && y <= d1up[3])){ 02227 d1count = d1count + touchsensativity; 02228 } 02229 if (( x >= d1down[0] && x <= d1down[1] ) && (y >= d1down[2] && y <= d1down[3])){ 02230 d1count = d1count - touchsensativity; 02231 } 02232 02233 //Day 02234 if (( x >= d2up[0] && x <= d2up[1] ) && (y >= d2up[2] && y <= d2up[3])){ 02235 d2count = d2count + touchsensativity; 02236 02237 } 02238 if (( x >= d2down[0] && x <= d2down[1] ) && (y >= d2down[2] && y <= d2down[3])){ 02239 d2count = d2count - touchsensativity; 02240 02241 } 02242 02243 //year 02244 if (( x >= d3up[0] && x <= d3up[1] ) && (y >= d3up[2] && y <= d3up[3])){ 02245 d3count = d3count + touchsensativity; 02246 02247 } 02248 if (( x >= d3down[0] && x <= d3down[1] ) && (y >= d3down[2] && y <= d3down[3])){ 02249 d3count = d3count - touchsensativity; 02250 } 02251 02252 //hour 02253 if (( x >= d4up[0] && x <= d4up[1] ) && (y >= d4up[2] && y <= d4up[3])){ 02254 d4count = d4count + touchsensativity; 02255 02256 } 02257 if (( x >= d4down[0] && x <= d4down[1] ) && (y >= d4down[2] && y <= d4down[3])){ 02258 d4count = d4count - touchsensativity; 02259 } 02260 02261 //Min 02262 if (( x >= d5up[0] && x <= d5up[1] ) && (y >= d5up[2] && y <= d5up[3])){ 02263 d5count = d5count + touchsensativity; 02264 } 02265 if (( x >= d5down[0] && x <= d5down[1] ) && (y >= d5down[2] && y <= d5down[3])){ 02266 d5count = d5count - touchsensativity; 02267 } 02268 02269 //Interval 02270 if (( x >= d6up[0] && x <= d6up[1] ) && (y >= d6up[2] && y <= d6up[3])){ 02271 d6count = d6count + touchsensativity; 02272 Thread::wait(2); 02273 EE_WriteVariable(VirtAddVarTab[8], (uint16_t)d6count); 02274 } 02275 if (( x >= d6down[0] && x <= d6down[1] ) && (y >= d6down[2] && y <= d6down[3])){ 02276 d6count = d6count - touchsensativity; 02277 Thread::wait(2); 02278 EE_WriteVariable(VirtAddVarTab[8], (uint16_t)d6count); 02279 } 02280 02281 02282 /* sprintf((char*)text, "Touch %d: x=%d y=%d ", idx+1, x, y); 02283 lcd.DisplayStringAt(0, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); */ 02284 } 02285 lcd.DrawPixel(TS_State.touchX[0], TS_State.touchY[0], LCD_COLOR_ORANGE); 02286 } else { 02287 if (!cleared) { 02288 lcd.Clear(LCD_COLOR_BLACK); 02289 /* sprintf((char*)text, "Touches: 0"); 02290 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); */ 02291 cleared = 1; 02292 } 02293 } 02294 02295 } 02296 } 02297 02298 02299 02300 void screencycle() { 02301 if (screenstate == 1) {screen();} 02302 if (screenstate == 0) {screen2();} 02303 if (screenstate == 2) {screen3();} 02304 } 02305 ///////////// 02306 02307 int main() 02308 { 02309 i2c.frequency(100000); 02310 set_ch(1); 02311 pc.baud(9600); 02312 LED515.write(0.0); 02313 LED480.write(0.0); 02314 /* sd.mount(); 02315 FILE *fp = fopen("/sd/Readings.txt", "w"); 02316 if (fp == NULL) 02317 { 02318 fprintf(stderr, "Open error for writing!!\r\n"); 02319 while (true) {} 02320 } 02321 fprintf(fp, "Hello!\n"); 02322 fprintf(fp, "Example of writing and reading of text file.\n"); 02323 fclose(fp); */ 02324 //Setup LED515 02325 //LED515.period(-1.0f); // 4 second period 02326 //LED515.write(0); // 50% duty cycle, relative to period 02327 HAL_FLASH_Unlock(); 02328 EE_Init(); 02329 //pump1 02330 EE_ReadVariable(VirtAddVarTab[0], &VarDataTab[0]); 02331 p1count = (double)VarDataTab[0]; 02332 //pump2 02333 EE_ReadVariable(VirtAddVarTab[1], &VarDataTab[1]); 02334 p2count = (double)VarDataTab[1]; 02335 //pump3 02336 EE_ReadVariable(VirtAddVarTab[2], &VarDataTab[2]); 02337 p3count = (double)VarDataTab[2]; 02338 //pump4 02339 EE_ReadVariable(VirtAddVarTab[3], &VarDataTab[3]); 02340 p4count = (double)VarDataTab[3]; 02341 //Sole5 02342 EE_ReadVariable(VirtAddVarTab[4], &VarDataTab[4]); 02343 p5count = (double)VarDataTab[4]; 02344 //Pump6 02345 EE_ReadVariable(VirtAddVarTab[5], &VarDataTab[5]); 02346 p6count = (double)VarDataTab[5]; 02347 //Pump7 02348 EE_ReadVariable(VirtAddVarTab[6], &VarDataTab[6]); 02349 p7count = (double)VarDataTab[6]; 02350 //Screen 02351 EE_ReadVariable(VirtAddVarTab[7], &VarDataTab[7]); 02352 screenstate = (int)VarDataTab[7]; 02353 //Interval 02354 EE_ReadVariable(VirtAddVarTab[8], &VarDataTab[8]); 02355 d6count = (int)VarDataTab[8]; 02356 02357 //Draw WolfMan 02358 /* lcd.Clear(LCD_COLOR_RED); 02359 lcd.SetTextColor(LCD_COLOR_BLACK); 02360 lcd.SetBackColor(LCD_COLOR_RED); 02361 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)"WolfMan", RIGHT_MODE); 02362 lcd.SetTextColor(LCD_COLOR_LIGHTBLUE);*/ 02363 lcd.Clear(LCD_COLOR_WHITE); 02364 lcd.SetBackColor(LCD_COLOR_WHITE); 02365 lcd.DrawBitmap(150,25,(uint8_t *)hfl); 02366 02367 //motors[1]->reset_device(); 02368 wait(2); 02369 02370 FATFS_LinkDriver(&SD_Driver, SDPath); 02371 ress = f_mount(&SDFatFs, (TCHAR const*)SDPath, 0); 02372 Thread::wait(200); 02373 if(ress!= FR_OK){ pc.printf("Failed to mount SD\n"); } 02374 02375 saveit(); 02376 ts.Init(lcd.GetXSize(), lcd.GetYSize()); 02377 lcd.SetFont(&Font12); 02378 lcd.SetBackColor(LCD_COLOR_BLACK); 02379 lcd.SetTextColor(LCD_COLOR_WHITE); 02380 thread.start(screencycle); 02381 sprintf((char*)cyclestep, ""); 02382 //reprime(); 02383 LED515.period(1.0/10000); 02384 LED480.period(1.0/10000); 02385 thread3.start(takereading); 02386 02387 02388 } 02389
Generated on Thu Jul 14 2022 06:12:33 by
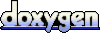