
f
Dependencies: TS_DISCO_F746NG mbed LCD_DISCO_F746NG mbed-rtos BSP_DISCO_F746NG FatFS X_NUCLEO_IHM02A1
eeprom.h
00001 /** 00002 ****************************************************************************** 00003 * @file EEPROM/EEPROM_Emulation/inc/eeprom.h 00004 * @author MCD Application Team 00005 * @version V1.0.1 00006 * @date 29-January-2016 00007 * @brief This file contains all the functions prototypes for the EEPROM 00008 * emulation firmware library. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __EEPROM_H 00041 #define __EEPROM_H 00042 00043 /* Includes ------------------------------------------------------------------*/ 00044 #include "stm32f7xx_hal.h" 00045 00046 /* Exported constants --------------------------------------------------------*/ 00047 /* EEPROM emulation firmware error codes */ 00048 #define EE_OK (uint32_t)HAL_OK 00049 #define EE_ERROR (uint32_t)HAL_ERROR 00050 #define EE_BUSY (uint32_t)HAL_BUSY 00051 #define EE_TIMEOUT (uint32_t)HAL_TIMEOUT 00052 00053 /* Define the size of the sectors to be used */ 00054 #define PAGE_SIZE (uint32_t)0x4000 /* Page size = 16KByte */ 00055 00056 /* Device voltage range supposed to be [2.7V to 3.6V], the operation will 00057 be done by word */ 00058 #define VOLTAGE_RANGE (uint8_t)VOLTAGE_RANGE_3 00059 00060 /* EEPROM start address in Flash */ 00061 #define EEPROM_START_ADDRESS ((uint32_t)0x08008000) /* EEPROM emulation start address: 00062 from sector2 : after 16KByte of used 00063 Flash memory */ 00064 00065 /* Pages 0 and 1 base and end addresses */ 00066 #define PAGE0_BASE_ADDRESS ((uint32_t)(EEPROM_START_ADDRESS + 0x0000)) 00067 #define PAGE0_END_ADDRESS ((uint32_t)(EEPROM_START_ADDRESS + (PAGE_SIZE - 1))) 00068 #define PAGE0_ID FLASH_SECTOR_2 00069 00070 #define PAGE1_BASE_ADDRESS ((uint32_t)(EEPROM_START_ADDRESS + 0x4000)) 00071 #define PAGE1_END_ADDRESS ((uint32_t)(EEPROM_START_ADDRESS + (2 * PAGE_SIZE - 1))) 00072 #define PAGE1_ID FLASH_SECTOR_3 00073 00074 /* Used Flash pages for EEPROM emulation */ 00075 #define PAGE0 ((uint16_t)0x0000) 00076 #define PAGE1 ((uint16_t)0x0001) /* Page nb between PAGE0_BASE_ADDRESS & PAGE1_BASE_ADDRESS*/ 00077 00078 /* No valid page define */ 00079 #define NO_VALID_PAGE ((uint16_t)0x00AB) 00080 00081 /* Page status definitions */ 00082 #define ERASED ((uint16_t)0xFFFF) /* Page is empty */ 00083 #define RECEIVE_DATA ((uint16_t)0xEEEE) /* Page is marked to receive data */ 00084 #define VALID_PAGE ((uint16_t)0x0000) /* Page containing valid data */ 00085 00086 /* Valid pages in read and write defines */ 00087 #define READ_FROM_VALID_PAGE ((uint8_t)0x00) 00088 #define WRITE_IN_VALID_PAGE ((uint8_t)0x01) 00089 00090 /* Page full define */ 00091 #define PAGE_FULL ((uint8_t)0x80) 00092 00093 /* Variables' number */ 00094 #define NB_OF_VAR ((uint8_t)0x09) 00095 00096 /* Exported types ------------------------------------------------------------*/ 00097 /* Exported macro ------------------------------------------------------------*/ 00098 /* Exported functions ------------------------------------------------------- */ 00099 uint16_t EE_Init(void); 00100 uint16_t EE_ReadVariable(uint16_t VirtAddress, uint16_t* Data); 00101 uint16_t EE_WriteVariable(uint16_t VirtAddress, uint16_t Data); 00102 00103 #endif /* __EEPROM_H */ 00104 00105 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jul 14 2022 06:12:32 by
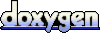