
Bus Can
Dependencies: mbed ssd1306_library
main_Carte2.cpp
00001 /* 00002 * Copyright (c) 2017-2020 Arm Limited and affiliates. 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 #if !DEVICE_CAN 00007 #error [NOT_SUPPORTED] CAN not supported for this target 00008 #endif 00009 00010 00011 #include "mbed.h" 00012 #include "ssd1306.h" 00013 00014 SSD1306 OLED (I2C_SDA, I2C_SCL); // assumes default I2C address of 0x78 00015 00016 00017 //Ticker ticker; 00018 00019 DigitalOut ledD9(PA_4); 00020 DigitalOut ledD8(PA_5); // NON, désouder SB16 si utilisation I2c 00021 DigitalOut ledD7(PA_6); // NON, désouder SB18 si utilisation I2c 00022 DigitalOut ledD6(PA_7); 00023 00024 DigitalIn SW4_1(PA_1); 00025 DigitalIn SW4_0(PA_3); 00026 DigitalIn SW1(PB_4, PullUp); 00027 DigitalIn SW2(PB_5, PullUp); 00028 //DigitalIn SW3(PA_8, PullUp); 00029 00030 //InterruptIn SW1(PB_4, PullUp); 00031 //InterruptIn SW2(PB_5, PullUp); 00032 InterruptIn SW3(PA_8, PullUp); 00033 00034 /** The constructor takes in RX, and TX pin respectively. 00035 * These pins, for this example, are defined in mbed_app.json 00036 */ 00037 //CAN can1(MBED_CONF_APP_CAN1_RD, MBED_CONF_APP_CAN1_TD); 00038 00039 CAN can(PA_11, PA_12); 00040 //Serial pc(USBTX, USBRX); 00041 00042 char counter = 123; 00043 char Carte2[] = "K2 "; 00044 char Carte2bb[] = "K2bb"; 00045 int Nb_messT = 0; 00046 int value_SW4=0; 00047 00048 int detectionFrontSW1(void) { 00049 int frontDescendant = 0; 00050 static int etatPrecedent1=1; 00051 int bp = SW1.read(); 00052 if(bp!=etatPrecedent1 && !bp) 00053 frontDescendant = 1; 00054 etatPrecedent1= bp; 00055 return frontDescendant; 00056 } 00057 00058 int detectionFrontSW2(void) { 00059 int frontDescendant = 0; 00060 static int etatPrecedent1=1; 00061 int bp = SW2.read(); 00062 if(bp!=etatPrecedent1 && !bp) 00063 frontDescendant = 1; 00064 etatPrecedent1= bp; 00065 return frontDescendant; 00066 } 00067 00068 /* 00069 int detectionFrontSW3(void) { 00070 int frontDescendant = 0; 00071 static int etatPrecedent1=1; 00072 int bp = SW3.read(); 00073 if(bp!=etatPrecedent1 && !bp) 00074 frontDescendant = 1; 00075 etatPrecedent1= bp; 00076 return frontDescendant; 00077 } 00078 */ 00079 00080 /* Test Arbitrage */ 00081 void AppuiSW3() { 00082 if (value_SW4 == 3) { 00083 if (can.write(CANMessage(60, Carte2bb, 5 , CANData, CANStandard))) { // Rmq : tableau = pointeur 00084 ledD8 = !ledD8; 00085 Nb_messT++; 00086 } 00087 } 00088 if (value_SW4 == 2) { 00089 if (can.write(CANMessage(48, Carte2bb, 5 , CANData, CANStandard))) { // Rmq : tableau = pointeur 00090 ledD8 = !ledD8; 00091 Nb_messT++; 00092 } 00093 } 00094 } 00095 00096 00097 int LectureSW4(){ 00098 int ETAT; 00099 int value_SW4_0 = SW4_0.read(); 00100 int value_SW4_1 = SW4_1.read(); 00101 00102 if (value_SW4_1 == 1) { 00103 if (value_SW4_0 == 1) 00104 ETAT = 3; 00105 else 00106 ETAT = 2; 00107 } 00108 else { 00109 if (value_SW4_0 == 1) 00110 ETAT = 1; 00111 else 00112 ETAT = 0; 00113 } 00114 return ETAT; 00115 } 00116 00117 00118 int main() 00119 { 00120 //pc.baud(115200); 00121 //pc.printf("main()\n"); 00122 00123 can.frequency(500000); 00124 CANMessage msg; 00125 00126 char Donnees[8]=""; 00127 static int Nb_messR = 0; 00128 00129 ledD6 = 1; ledD7 = 1; ledD8 = 1; ledD9 = 1; 00130 00131 OLED.speed(SSD1306::Medium); // set working frequency 00132 OLED.init(); // initialize SSD1306 00133 OLED.cls(); // clear frame buffer 00134 00135 OLED.locate (0,0); 00136 OLED.printf ("NbRx = 0"); 00137 OLED.locate (1,0); 00138 OLED.printf ("NbTx = 0"); 00139 00140 SW3.fall(&AppuiSW3); 00141 00142 while (1) { 00143 //pc.printf("Reception message \n"); 00144 value_SW4 = LectureSW4(); 00145 00146 if (detectionFrontSW1()) 00147 { 00148 if (value_SW4 == 0) { 00149 if (can.write(CANMessage(2021, Carte2, 5 , CANData, CANStandard))) { // Rmq : tableau = pointeur 00150 ledD6 = !ledD6; 00151 Nb_messT++; 00152 } 00153 } 00154 } 00155 /* 00156 if (detectionFrontSW2()) 00157 { 00158 if (value_SW4 == 0) { 00159 if (can.write(CANMessage(2021, CANStandard))) { // Remote frame 00160 ledD7 = !ledD7; 00161 Nb_messT++; 00162 } 00163 } 00164 } 00165 */ 00166 /* 00167 if (detectionFrontSW3()) 00168 { 00169 if (value_SW4 == 3) { 00170 if (can.write(CANMessage(60, Carte2bb, 5 , CANData, CANStandard))) { // Rmq : tableau = pointeur 00171 ledD8 = !ledD8; 00172 Nb_messT++; 00173 } 00174 } 00175 if (value_SW4 == 2) { 00176 if (can.write(CANMessage(48, Carte2bb, 5 , CANData, CANStandard))) { // Rmq : tableau = pointeur 00177 ledD8 = !ledD8; 00178 Nb_messT++; 00179 } 00180 } 00181 } 00182 */ 00183 00184 if (can.read(msg)) { 00185 Nb_messR++; 00186 ledD9 = !ledD9; 00187 00188 for (int i =0; i < msg.len; i++) 00189 Donnees[i] = msg.data[i]; 00190 00191 //pc.printf("ID = 0x%.3x\r\n", msg.id); //ID sous forme 0x suivi de l'id 00192 //pc.printf("Length = %d\r\n", msg.len); 00193 //pc.printf("CAN rderrors : %d, CAN tderrors : %d\n", can.rderror(), can.tderror()); 00194 00195 } 00196 OLED.locate (0,0); 00197 OLED.printf ("NbRx = %d", Nb_messR); 00198 OLED.locate (1,0); 00199 OLED.printf ("NbTx = %d", Nb_messT); 00200 OLED.locate (3,0); 00201 OLED.printf ("Message recu "); 00202 OLED.locate (4,0); 00203 OLED.printf ("=> "); 00204 OLED.puts(Donnees); 00205 OLED.locate (6,0); 00206 OLED.printf("Valeur REC=%d", can.rderror()); 00207 OLED.locate (7,0); 00208 OLED.printf("Valeur TEC=%d", can.tderror()); 00209 OLED.redraw(); 00210 wait(0.2); 00211 } 00212 } 00213 00214 00215 /* Envoi d'une trame de requete */ 00216 /* 00217 void AppuiSW2() { 00218 if (can.write(CANMessage(1620, CANStandard ))) { 00219 ledD7 = !ledD7; 00220 } 00221 } 00222 */ 00223 /* Reset controleur après court-circuit */ 00224 /* 00225 void AppuiSW3() { 00226 if (can.write(CANMessage(2021, Reset, 6 , CANData, CANStandard))) // Rmq : tableau = pointeur 00227 ledD8 = !ledD8; 00228 else 00229 can.reset(); 00230 } 00231 */ 00232 /* Reset controleur après court-circuit */ 00233 /* 00234 void AppuiSW3() { 00235 can.reset(); 00236 OLED.cls(); 00237 OLED.locate (0,0); // set text cursor to line 3, column 1 00238 OLED.printf ("GEII - Bus CAN"); // print to frame buffer 00239 OLED.locate (2,0); // set text cursor to line 3, column 1 00240 OLED.printf ("SW1"); // print to frame buffer 00241 OLED.locate (3,0); // set text cursor to line 3, column 1 00242 OLED.printf ("Envoi trame"); // print to frame buffer 00243 OLED.redraw(); 00244 } 00245 */ 00246 /* 00247 char *conversionIntChaine(int longueurChaine, int Valeur_int, char *ChaineAffichage) 00248 { 00249 while (longueurChaine >= 0) 00250 { 00251 ChaineAffichage[longueurChaine] = (Valeur_int % 10) + 48; 00252 Valeur_int /= 10; 00253 longueurChaine--; 00254 } 00255 return (ChaineAffichage); 00256 } 00257 */ 00258 00259 /* 00260 OLED.locate (0,0); // set text cursor to line 3, column 1 00261 OLED.printf ("GEII - Bus CAN"); // print to frame buffer 00262 OLED.locate (2,0); // set text cursor to line 3, column 1 00263 OLED.printf ("SW1"); // print to frame buffer 00264 OLED.locate (3,0); // set text cursor to line 3, column 1 00265 OLED.printf ("Envoi trame"); // print to frame buffer 00266 OLED.redraw(); 00267 */ 00268 00269 /* 00270 char * 00271 itoa (int n) 00272 { 00273 00274 char *a = malloc (long_int (n) + 1); 00275 int i; 00276 for (i = 0; n > 0; i++) 00277 { 00278 a[i] = n % 10 + 48; 00279 n = n / 10; 00280 } 00281 a[i + 1] = '\0'; 00282 return retourne (a); 00283 } 00284 00285 char *create_str(int len, int n, int neg, char *str) 00286 { 00287 while (len >= 0) 00288 { 00289 str[len] = (n % 10) + 48; 00290 n /= 10; 00291 len--; 00292 } 00293 if (neg < 0) 00294 str[0] = '-'; 00295 return (str); 00296 } 00297 */
Generated on Wed Aug 31 2022 04:18:02 by
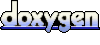