This is the Official SmartGPU 2 processor board library to use with MBED boards!!!
Dependents: BounceBall_SG2 BounceBalls_SG2 EEPROM_SG2 Ellipses_SG2 ... more
SMARTGPU2.h
00001 /********************************************************* 00002 VIZIC TECHNOLOGIES. COPYRIGHT 2014. 00003 THE DATASHEETS, SOFTWARE AND LIBRARIES ARE PROVIDED "AS IS." 00004 VIZIC EXPRESSLY DISCLAIM ANY WARRANTY OF ANY KIND, WHETHER 00005 EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO, THE IMPLIED 00006 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, 00007 OR NONINFRINGEMENT. IN NO EVENT SHALL VIZIC BE LIABLE FOR 00008 ANY INCIDENTAL, SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES, 00009 LOST PROFITS OR LOST DATA, HARM TO YOUR EQUIPMENT, COST OF 00010 PROCUREMENT OF SUBSTITUTE GOODS, TECHNOLOGY OR SERVICES, 00011 ANY CLAIMS BY THIRD PARTIES (INCLUDING BUT NOT LIMITED TO 00012 ANY DEFENCE THEREOF), ANY CLAIMS FOR INDEMNITY OR CONTRIBUTION, 00013 OR OTHER SIMILAR COSTS. 00014 *********************************************************/ 00015 00016 /******************************************************** 00017 MBED SMARTGPU2 LIBRARY VERSION V2.2 00018 IMPORTANT : This library is created for the MBED Software IDE 00019 ********************************************************/ 00020 00021 #ifndef SMARTGPU2_h 00022 #define SMARTGPU2_h 00023 00024 #include <mbed.h> 00025 00026 /*******************USER MODIFABLE**********************/ 00027 //-Uncomment your preferred smartGPU2 board(only 1 at time)---------------- 00028 //#define LCD160X128 //SmartGPU2 1.8" 00029 //#define LCD320X240 //SmartGPU2 2.4" 00030 //#define LCD480X320 //SmartGPU2 3.5" 00031 //#define LCD480X272 //SmartGPU2 4.3" 00032 //#define LCD800X480 //SmartGPU2 7.0" 00033 00034 //General MBED pinout defines 00035 #define TXPIN p13 00036 #define RXPIN p14 00037 //-SmartGPU2-MBED RESET PIN definition 00038 #define RESETPIN p15 //Define for the smartGPU2 RESET pin connected to the MBED board 00039 00040 //delay word compatibility for mbed platform 00041 #define delay wait_ms 00042 /****************END OF USER MODIFABLE******************/ 00043 00044 /**************DON'T MODIFY UP FROM HERE****************/ 00045 #ifdef LCD160X128 //defines for SmartGPU2 LCD160x128 1.8" ONLY 00046 #define LCD_WIDTH 160 00047 #define LCD_HEIGHT 128 00048 #elif defined LCD320X240 //defines for SmartGPU2 LCD320x240 2.4" ONLY 00049 #define LCD_WIDTH 320 00050 #define LCD_HEIGHT 240 00051 #elif defined LCD320X240SH //defines for SmartGPU2 LCD320x240SH 2.4" ONLY 00052 #define LCD_WIDTH 320 00053 #define LCD_HEIGHT 240 00054 #elif defined LCD480X320 //defines for SmartGPU2 LCD480x320 3.5" ONLY 00055 #define LCD_WIDTH 480 00056 #define LCD_HEIGHT 320 00057 #elif defined LCD480X272 //defines for SmartGPU2 LCD480x272 4.3" ONLY 00058 #define LCD_WIDTH 480 00059 #define LCD_HEIGHT 272 00060 #elif defined LCD800X480 //defines for SmartGPU2 LCD800x480 7.0" ONLY 00061 #define LCD_WIDTH 800 00062 #define LCD_HEIGHT 480 00063 #else 00064 #error "No smartGPU2 LCDXXXxXXX board defined in smartGPU2.h file" 00065 #endif 00066 00067 //Max X, Y values depending on orientation definitions 00068 #define MAX_X_LANDSCAPE LCD_WIDTH-1 00069 #define MAX_Y_LANDSCAPE LCD_HEIGHT-1 00070 #define MAX_X_PORTRAIT LCD_HEIGHT-1 00071 #define MAX_Y_PORTRAIT LCD_WIDTH-1 00072 00073 //General definitions 00074 #define OFF 0 00075 #define ON 1 00076 #define GND 0 00077 #define VCC 1 00078 #define SCROLLBUTTONSIZE 25 00079 typedef unsigned int AXIS; 00080 typedef unsigned int COLOUR; 00081 typedef unsigned int RADIUS; 00082 typedef unsigned int NUMBEROFBYTES; 00083 typedef unsigned int ITEMNUMBER; 00084 typedef unsigned int ADDRESS; 00085 typedef unsigned long POINTERPOSITION; 00086 typedef char FILENAME[]; 00087 00088 typedef enum { 00089 DISABLE, //0 00090 ENABLE //1 00091 } STATE; //to set Enable/Disable States 00092 00093 typedef enum { 00094 DESELECTED, //0 00095 SELECTED //1 00096 } ACTIVE; //to set Selected/DeSelected Active objects 00097 00098 typedef enum { 00099 DESELECTEDTRANS, //0 set an unselected top window with transparent center 00100 SELECTEDTRANS, //1 set a selected window with transparent center 00101 SELECTEDGRAY, //2 set a selected window with gray center 00102 SELECTEDWHITE //3 set a selected window with white center 00103 } WINDOWTYPE; //to set type of window to draw 00104 00105 typedef struct { 00106 AXIS x; //X axis 00107 AXIS y; //Y axis 00108 } POINT; //to create a point with point.x and point.y variables 00109 00110 typedef enum { 00111 OK = 'O', //Command successfully executed 00112 FAIL = 'F' //Command Fail 00113 } SMARTGPUREPLY; //to get SmartGPU2 command responses 00114 00115 //Graphics functions definitions 00116 typedef enum { 00117 UNFILL, //0 00118 FILL //1 00119 } FILLGEOM; //to set fill or unfill colour geometry 00120 00121 typedef enum { 00122 HORIZONTAL, //0 00123 VERTICAL //1 00124 } ORIENTATIONPARAMETER;//to set gradient colour fade orientation and objects: scrollBar and Slider orientations 00125 00126 typedef enum { 00127 LANDSCAPE_LEFT, //0 left 00128 PORTRAIT_LOW, //1 low 00129 LANDSCAPE_RIGHT, //2 right 00130 PORTRAIT_TOP //3 top 00131 } LCDORIENTATIONS; //to set LCD orientations 00132 00133 typedef enum { 00134 QUADRANT1 = 1, //1 00135 QUADRANT2, //2 00136 QUADRANT3, //3 00137 QUADRANT4 //4 00138 } ARCQUADRANT; //to set desired arc drawing quadrant 00139 00140 //basic colours definitions 00141 #define BLACK 0x0000 00142 #define WHITE 0xFFFF 00143 #define RED 0xF800 00144 #define GREEN 0x07E0 00145 #define BLUE 0x001F 00146 #define YELLOW 0xFFE0 00147 #define CYAN 0x07FF 00148 #define MAGENTA 0xF81F 00149 00150 //fonts definitions 00151 typedef enum { 00152 FONT0 = 0, 00153 FONT1, 00154 FONT2, 00155 FONT3, 00156 FONT4, 00157 FONT5, 00158 FONT6, 00159 FONT7, 00160 FONT8, 00161 FONT9, 00162 FONT10, 00163 FONT11, 00164 FONT12, 00165 FONT13 00166 } FONTSIZE; //to set text font sizes 00167 00168 typedef enum { 00169 TRANS = 0, 00170 FILLED 00171 } TEXTBACKGROUNDCOLOURFILLUNFILL; //to set text background colour to transparent or filled 00172 00173 //Video 00174 typedef struct { 00175 unsigned int width; //X width 00176 unsigned int height; //Y height 00177 unsigned int framesPerSec; //video frames per second 00178 unsigned int totalFrames; //video total frames in the file 00179 } VIDDATA; //to create a Video Data struct containing, size X, size Y, frames per second and totalframes info 00180 00181 //Touch definitions 00182 typedef enum { 00183 INVALID, //returned touch point is invalid 00184 VALID //returned touch point is valid 00185 } TOUCHREPLY; //to get SmartGPU2 touch responses 00186 00187 typedef enum { 00188 HOUSE = 'H', 00189 MESSAGE = 'M', 00190 BOOK = 'B', 00191 PHONE = 'P', 00192 SONG = 'S', 00193 NONE = 'N' 00194 } ICON; //to get the type of touched icon 00195 00196 //File access definitions 00197 #define BEGINNING 0 00198 #define ALLCONTENTS 0 00199 typedef enum { 00200 READONLY = 1, //1 00201 WRITEONLY, //2 00202 READWRITE //3 00203 } OPENMODE; //to set the file access open mode 00204 00205 //SMARTGPU2 Command Execution responses definitions 00206 typedef enum { 00207 F_OK = 0, /* (0) Succeeded */ 00208 F_DISK_ERR, /* (1) A hard error occurred in the low level disk I/O layer */ 00209 F_INT_ERR, /* (2) Assertion failed */ 00210 F_NOT_READY, /* (3) The physical drive cannot work */ 00211 F_NO_FILE, /* (4) Could not find the file */ 00212 F_NO_PATH, /* (5) Could not find the path */ 00213 F_INVALID_NAME, /* (6) The path name format is invalid */ 00214 F_DENIED, /* (7) Access denied due to prohibited access or directory full */ 00215 F_EXIST, /* (8) Access denied due to prohibited access */ 00216 F_INVALID_OBJECT, /* (9) The file/directory object is invalid */ 00217 F_WRITE_PROTECTED, /* (10) The physical drive is write protected */ 00218 F_INVALID_DRIVE, /* (11) The logical drive number is invalid */ 00219 F_NOT_ENABLED, /* (12) The volume has no work area */ 00220 F_NO_FILESYSTEM, /* (13) There is no valid FAT volume */ 00221 F_MKFS_ABORTED, /* (14) The f_mkfs() aborted due to any parameter error */ 00222 F_TIMEOUT, /* (15) Could not get a grant to access the volume within defined period */ 00223 F_LOCKED, /* (16) The operation is rejected according to the file sharing policy */ 00224 F_NOT_ENOUGH_CORE, /* (17) LFN working buffer could not be allocated */ 00225 F_TOO_MANY_OPEN_FILES, /* (18) Number of open files > _FS_SHARE */ 00226 F_INVALID_PARAMETER /* (19) Given parameter is invalid */ 00227 } FILERESULT; //Gets all FAT functions related responses 00228 00229 //SMARTGPU2 WorkSpaces definitions 00230 typedef enum { 00231 WORKSPACE0 = 0, 00232 WORKSPACE1, 00233 WORKSPACE2, 00234 WORKSPACE3 00235 } WORKSPACEBLOCK; //to set the working workspace 00236 00237 //SMARTGPU2 EEPROM pages definitions, each page is 2048bytes (2Kb) in size 00238 typedef enum { 00239 PAGE0 = 0, 00240 PAGE1, 00241 PAGE2, 00242 PAGE3, 00243 PAGE4, 00244 PAGE5, 00245 PAGE6, 00246 PAGE7 00247 } EEPROMPAGE; //to set the EEPROM page 00248 00249 //Files Time and Date 00250 typedef struct { 00251 unsigned char hour; 00252 unsigned char minute; 00253 unsigned char second; 00254 unsigned char day; 00255 unsigned char month; 00256 unsigned int year; 00257 } TIME; //to create a Time-Date info struct 00258 00259 //JPG images scale factor definitions 00260 typedef enum { 00261 SCALE1_1 = 0, // 1 to 1 00262 SCALE1_2, // 1 to 2 00263 SCALE1_4, // 1 to 4 00264 SCALE1_8 // 1 to 8 00265 } JPGSCALEFACTOR; //to set the desired JPG image decompression scale factor 00266 00267 //Recommended(but not limited to) Mbed-SmartGPU Baud rate definitions 00268 typedef enum{ 00269 BAUD0 = 9600, 00270 BAUD1 = 19200, 00271 BAUD2 = 57600, 00272 BAUD3 = 115200, 00273 BAUD4 = 256000, 00274 BAUD5 = 500000, 00275 BAUD6 = 1000000, 00276 BAUD7 = 2000000 00277 } BAUDRATE; 00278 00279 //************************************************************************** 00280 // class SMARTGPU2 SMARTGPU2.h 00281 // This is the main class. It should be used like this : SMARTGPU2 lcd(p13,p14,p15); 00282 //*************************************************************************** 00283 class SMARTGPU2{ 00284 00285 public: 00286 /****************************************************************/ 00287 //MBED exclusive Functions 00288 /****************************************************************/ 00289 SMARTGPU2(PinName TXPin, PinName RXPin, PinName resetPin); 00290 00291 void init(); 00292 00293 SMARTGPUREPLY reset(); 00294 00295 SMARTGPUREPLY start(); 00296 00297 /****************************************************************/ 00298 //Master Functions 00299 /****************************************************************/ 00300 SMARTGPUREPLY erase(); 00301 00302 SMARTGPUREPLY sleep(STATE); 00303 00304 SMARTGPUREPLY orientation(LCDORIENTATIONS); 00305 00306 SMARTGPUREPLY bright(unsigned char); 00307 00308 SMARTGPUREPLY baudChange(unsigned long); 00309 00310 SMARTGPUREPLY setEraseBackColour(COLOUR); 00311 00312 /****************************************************************/ 00313 //Geometric Functions 00314 /****************************************************************/ 00315 SMARTGPUREPLY putPixel(AXIS, AXIS, COLOUR); 00316 00317 SMARTGPUREPLY drawLine(AXIS, AXIS, AXIS, AXIS, COLOUR); 00318 00319 SMARTGPUREPLY drawRectangle(AXIS, AXIS, AXIS, AXIS, COLOUR, FILLGEOM); 00320 00321 SMARTGPUREPLY drawRoundRect(AXIS, AXIS, AXIS, AXIS, RADIUS, COLOUR, FILLGEOM); 00322 00323 SMARTGPUREPLY drawGradientRect(AXIS, AXIS, AXIS, AXIS, COLOUR, COLOUR, ORIENTATIONPARAMETER); 00324 00325 SMARTGPUREPLY drawTriangle(AXIS, AXIS, AXIS, AXIS, AXIS, AXIS, COLOUR, FILLGEOM); 00326 00327 SMARTGPUREPLY drawArc(AXIS, AXIS, RADIUS, RADIUS, ARCQUADRANT, COLOUR, FILLGEOM); 00328 00329 SMARTGPUREPLY drawCircle(AXIS, AXIS, RADIUS, COLOUR, FILLGEOM); 00330 00331 SMARTGPUREPLY drawEllipse(AXIS, AXIS, RADIUS, RADIUS, COLOUR, FILLGEOM); 00332 00333 /****************************************************************/ 00334 //String Functions 00335 /****************************************************************/ 00336 SMARTGPUREPLY putLetter(AXIS, AXIS, char, AXIS*); 00337 00338 SMARTGPUREPLY printNumber(AXIS, AXIS, float); 00339 00340 SMARTGPUREPLY string(AXIS, AXIS, AXIS, AXIS, char[], NUMBEROFBYTES*); //returns in NUMBEROFBYTES the successfully printed chars or letters 00341 00342 SMARTGPUREPLY stringSD(AXIS, AXIS, AXIS, AXIS, NUMBEROFBYTES, NUMBEROFBYTES, FILENAME, NUMBEROFBYTES*); //returns in NUMBEROFBYTES the successfully printed chars or letters 00343 00344 SMARTGPUREPLY setTextColour(COLOUR); 00345 00346 SMARTGPUREPLY setTextBackColour(COLOUR); 00347 00348 SMARTGPUREPLY setTextSize(FONTSIZE); 00349 00350 SMARTGPUREPLY setTextBackFill(TEXTBACKGROUNDCOLOURFILLUNFILL); 00351 00352 /****************************************************************/ 00353 //Image Functions 00354 /****************************************************************/ 00355 SMARTGPUREPLY drawIcon(AXIS, AXIS, AXIS, AXIS, char[]); 00356 00357 SMARTGPUREPLY imageBMPSD(AXIS, AXIS, FILENAME); 00358 00359 SMARTGPUREPLY imageJPGSD(AXIS, AXIS, JPGSCALEFACTOR, FILENAME); 00360 00361 SMARTGPUREPLY getImageFromMemory(AXIS, AXIS, AXIS, AXIS, char[]); //Read the internal memory of the SMARTGPU2, This command returns 24bit pixels (3 bytes) 00362 00363 SMARTGPUREPLY screenshot(); 00364 00365 /****************************************************************/ 00366 //Video Functions 00367 /****************************************************************/ 00368 SMARTGPUREPLY allocateVideoSD(FILENAME, VIDDATA*); 00369 00370 SMARTGPUREPLY freeVideoSD(); 00371 00372 SMARTGPUREPLY setFrameVideoSD(unsigned int); 00373 00374 SMARTGPUREPLY playVideoSD(AXIS, AXIS, unsigned int); 00375 00376 /****************************************************************/ 00377 //Audio Functions 00378 /****************************************************************/ 00379 SMARTGPUREPLY initDACAudio(STATE); 00380 00381 SMARTGPUREPLY audioBoost(STATE); 00382 00383 SMARTGPUREPLY getWAVPlayState(STATE*); 00384 00385 SMARTGPUREPLY playWAVFile(FILENAME, unsigned int*); //returns in unsigned int* the file duration in seconds 00386 00387 SMARTGPUREPLY pauseWAVFile(); 00388 00389 SMARTGPUREPLY stopWAVFile(); 00390 00391 SMARTGPUREPLY advanceWAVFile(unsigned int); //advance file to the parameter(means seconds) 00392 00393 SMARTGPUREPLY setVolumeWAV(unsigned char); 00394 00395 /****************************************************************/ 00396 //Real Time Clock Functions 00397 /****************************************************************/ 00398 SMARTGPUREPLY setupRTC(STATE*); 00399 00400 SMARTGPUREPLY getRTCTimeDate(TIME*); 00401 00402 SMARTGPUREPLY setRTCTimeDate(TIME*); 00403 00404 /****************************************************************/ 00405 //Objects Functions - Refer to "smartGPU2 Command Set" to learn about minimum width and height objects size. 00406 /****************************************************************/ 00407 SMARTGPUREPLY objButton(AXIS, AXIS, AXIS, AXIS, ACTIVE, char[]); 00408 00409 SMARTGPUREPLY objSwitch(AXIS, AXIS, unsigned int, ACTIVE); 00410 00411 SMARTGPUREPLY objCheckbox(AXIS, AXIS, unsigned int, ACTIVE); 00412 00413 SMARTGPUREPLY objProgressBar(AXIS, AXIS, AXIS, AXIS, unsigned char); 00414 00415 SMARTGPUREPLY objSlider(AXIS, AXIS, AXIS, AXIS, unsigned char, unsigned char, ORIENTATIONPARAMETER); 00416 00417 SMARTGPUREPLY objScrollBar(AXIS, AXIS, AXIS, AXIS, unsigned char, unsigned char, ORIENTATIONPARAMETER, ACTIVE); 00418 00419 SMARTGPUREPLY objWindow(AXIS, AXIS, AXIS, AXIS, FONTSIZE, WINDOWTYPE, char[]); 00420 00421 /****************************************************************/ 00422 //EEPROM-FLASH Functions - Refer to "smartGPU2 Command Set" to learn about READ-WRITE procedure, and page SIZE. 00423 /****************************************************************/ 00424 SMARTGPUREPLY initClearEEPROMBuff(); 00425 00426 SMARTGPUREPLY readEEPROMBuff(char[], ADDRESS, NUMBEROFBYTES, NUMBEROFBYTES*); 00427 00428 SMARTGPUREPLY writeEEPROMBuff(char[], ADDRESS, NUMBEROFBYTES, NUMBEROFBYTES*); 00429 00430 SMARTGPUREPLY saveBuffToEEPROMPage(EEPROMPAGE); 00431 00432 SMARTGPUREPLY fillBuffFromEEPROMPage(EEPROMPAGE); 00433 00434 SMARTGPUREPLY compBuffToEEPROMPage(EEPROMPAGE, unsigned char*); 00435 00436 SMARTGPUREPLY eraseEEPROMPage(EEPROMPAGE); 00437 00438 /****************************************************************/ 00439 //Touch Functions 00440 //Those next Touch Functions return valid or invalid touch coordinates status(TOUCHREPLY) instead of ACK 'O' or NAK 'F'(SMARTGPUREPLY) 00441 /****************************************************************/ 00442 TOUCHREPLY touchScreen(POINT*); 00443 #ifdef LCD320X240 //define function only for the SmartGPU2 LCD320x240 2.4" is selected, as it is the only board with touch icons 00444 TOUCHREPLY touchIcon(ICON*); 00445 #elif defined LCD320X240SH //define function only for the SmartGPU2 LCD320x240SH 2.4" is selected, as it is the only board with touch icons 00446 TOUCHREPLY touchIcon(ICON*); 00447 #endif 00448 00449 /****************************************************************/ 00450 //SD FAT management Functions 00451 //Those next SDF - SD Functions return file execution status(FILERESULT) instead of ACK 'O' or NAK 'F'(SMARTGPUREPLY) 00452 /****************************************************************/ 00453 FILERESULT SDFgetList(unsigned int*, unsigned int*); //get number of dirs and files 00454 00455 FILERESULT SDFgetDirName(ITEMNUMBER, FILENAME); //searches for the "itemNumber" on the SD current folder and updates the buffer with the Dir name ended with NULL character 00456 00457 FILERESULT SDFgetFileName(ITEMNUMBER, FILENAME); //searches for the "itemNumber" on the SD current folder and updates the buffer with the File name ended with NULL character 00458 00459 FILERESULT SDFgetDirPath(char[]); //obtains current dir path and stores on path[] buffer 00460 00461 FILERESULT SDFnewDir(FILENAME); //create a new Directory, fails if already exist 00462 00463 FILERESULT SDFnewFile(FILENAME); //create a new File, fails if already exist 00464 00465 FILERESULT SDFopenDir(FILENAME); //opens an existing Dir 00466 00467 FILERESULT SDFopenFile(FILENAME, OPENMODE, WORKSPACEBLOCK); //opens an existing file in READONLY, WRITEONLY or READWRITE mode on the received object # workspace 00468 00469 FILERESULT SDFcloseFile(WORKSPACEBLOCK); //close and save file object # workspace 00470 00471 FILERESULT SDFsaveFile(WORKSPACEBLOCK); //sync/save file object # workspace 00472 00473 FILERESULT SDFsetFilePointer(POINTERPOSITION, WORKSPACEBLOCK); // set/move file pointer of file object # workspace 00474 00475 FILERESULT SDFgetFilePointer(POINTERPOSITION*, WORKSPACEBLOCK); // get file pointer of file object # workspace 00476 00477 FILERESULT SDFreadFile(char[], NUMBEROFBYTES, NUMBEROFBYTES*, WORKSPACEBLOCK); //Bytes to Read, Succesfully Read Bytes, file object # to read bytes from 00478 00479 FILERESULT SDFwriteFile(char[], NUMBEROFBYTES,NUMBEROFBYTES*, WORKSPACEBLOCK); //Bytes to Write, Succesfully Written Bytes, file object # to write bytes 00480 00481 FILERESULT SDFtestFileError(WORKSPACEBLOCK); //test for an error on file # workspace 00482 00483 FILERESULT SDFtestFileEnd(WORKSPACEBLOCK); //test for an error on file # workspace 00484 00485 FILERESULT SDFtruncateFile(WORKSPACEBLOCK); //truncates the file size to the current file read/write pointer of the file # workspace 00486 00487 FILERESULT SDFeraseDirFile(FILENAME); //Erases an existing Dir or File 00488 00489 FILERESULT SDFsetFileTimeDate(TIME*, FILENAME); //Set Time and Date to an existing File 00490 00491 FILERESULT SDFgetFileTimeDate(TIME*, FILENAME); //Get Time and Date to an existing File 00492 00493 FILERESULT SDFgetFileSize(FILENAME, unsigned long *); //Get Size of an existing File 00494 00495 FILERESULT SDFrenameMoveDirFile(FILENAME, FILENAME); //renames or moves an existing Dir or File 00496 00497 FILERESULT SDFgetFreeTotalSpace(unsigned long *,unsigned long *); //Get free and total space in bytes of the microSD card 00498 00499 protected : 00500 00501 Serial _serialSMARTGPU2; 00502 DigitalOut _resetPin; 00503 00504 }; 00505 00506 #endif
Generated on Thu Jul 14 2022 14:58:56 by
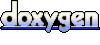