
Example FIR filter using the CMSIS DSP API
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "arm_math.h" 00002 #include "math_helper.h" 00003 #include <stdio.h> 00004 00005 #define BLOCK_SIZE 32 00006 #define NUM_BLOCKS 10 00007 00008 #define TEST_LENGTH_SAMPLES (BLOCK_SIZE * NUM_BLOCKS) 00009 00010 #define SNR_THRESHOLD_F32 140.0f 00011 #define NUM_TAPS 29 00012 00013 /* ------------------------------------------------------------------- 00014 * The input signal and reference output (computed with MATLAB) 00015 * are defined externally in arm_fir_lpf_data.c. 00016 * ------------------------------------------------------------------- */ 00017 extern float32_t testInput_f32_1kHz_15kHz[TEST_LENGTH_SAMPLES]; 00018 extern float32_t refOutput[TEST_LENGTH_SAMPLES]; 00019 00020 /* ------------------------------------------------------------------- 00021 * Declare State buffer of size (numTaps + blockSize - 1) 00022 * ------------------------------------------------------------------- */ 00023 static float32_t firStateF32[BLOCK_SIZE + NUM_TAPS - 1]; 00024 00025 /* ---------------------------------------------------------------------- 00026 * FIR Coefficients buffer generated using fir1() MATLAB function. 00027 * fir1(28, 6/24) 00028 * ------------------------------------------------------------------- */ 00029 const float32_t firCoeffs32[NUM_TAPS] = { 00030 -0.0018225230f, -0.0015879294f, +0.0000000000f, +0.0036977508f, +0.0080754303f, 00031 +0.0085302217f, -0.0000000000f, -0.0173976984f, -0.0341458607f, -0.0333591565f, 00032 +0.0000000000f, +0.0676308395f, +0.1522061835f, +0.2229246956f, +0.2504960933f, 00033 +0.2229246956f, +0.1522061835f, +0.0676308395f, +0.0000000000f, -0.0333591565f, 00034 -0.0341458607f, -0.0173976984f, -0.0000000000f, +0.0085302217f, +0.0080754303f, 00035 +0.0036977508f, +0.0000000000f, -0.0015879294f, -0.0018225230f 00036 }; 00037 00038 /* ---------------------------------------------------------------------- 00039 * FIR LPF Example 00040 * ------------------------------------------------------------------- */ 00041 int32_t main(void) { 00042 /* Call FIR init function to initialize the instance structure. */ 00043 arm_fir_instance_f32 S; 00044 arm_fir_init_f32(&S, NUM_TAPS, (float32_t *)&firCoeffs32[0], &firStateF32[0], BLOCK_SIZE); 00045 00046 /* ---------------------------------------------------------------------- 00047 * Call the FIR process function for every blockSize samples 00048 * ------------------------------------------------------------------- */ 00049 for (uint32_t i=0; i < NUM_BLOCKS; i++) { 00050 float32_t* signal = testInput_f32_1kHz_15kHz + (i * BLOCK_SIZE); 00051 arm_fir_f32(&S, signal, signal, BLOCK_SIZE); 00052 } 00053 00054 /* ---------------------------------------------------------------------- 00055 * Compare the generated output against the reference output computed 00056 * in MATLAB. 00057 * ------------------------------------------------------------------- */ 00058 float32_t snr = arm_snr_f32(refOutput, testInput_f32_1kHz_15kHz, TEST_LENGTH_SAMPLES); 00059 printf("snr: %f\n\r", snr); 00060 if (snr < SNR_THRESHOLD_F32) { 00061 printf("Failed\n\r"); 00062 } else { 00063 printf("Success\n\r"); 00064 } 00065 }
Generated on Tue Jul 12 2022 13:32:57 by
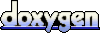