
Echo Server based on the legacy EthernetNetIf libraries used for a performance comparison with the new networking libraries
Dependencies: EthernetNetIf mbed
Fork of EchoServer by
main.cpp
00001 /* 00002 * Echo server 00003 * Listens on TCP and UDP ports 7 for any incoming connections 00004 * Re-transmits any incoming bytes 00005 */ 00006 00007 #include "mbed.h" 00008 #include "EthernetNetIf.h" 00009 00010 #include "EchoServer.h" 00011 00012 // Our Echo server 00013 EchoServer server; 00014 00015 /* 00016 Function: main 00017 00018 Sets up the Ethernet interface using DHCP, reports the assigned 00019 IP address via serial, binds the Echo server to port 7 on 00020 TCP and UDP and then sits in a loop calling Net::poll() to 00021 keep the network stack doing its thing 00022 */ 00023 int main() { 00024 printf("\r\nSetting up...\r\n"); 00025 //use DHCP 00026 00027 // Our Ethernet interface 00028 EthernetNetIf eth; 00029 EthernetErr ethErr = eth.setup(); 00030 if (ethErr) { 00031 printf("Error %d in setup.\n", ethErr); 00032 return -1; 00033 } 00034 IpAddr ip = eth.getIp(); 00035 00036 printf("mbed IP Address is %d.%d.%d.%d\r\n", ip[0], ip[1], ip[2], ip[3]); 00037 00038 server.bind(7,7); 00039 00040 printf("Entering while loop Net::poll()ing\r\n"); 00041 while (1) { 00042 Net::poll(); 00043 } 00044 }
Generated on Tue Jul 26 2022 23:07:02 by
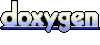