
Echo Server based on the legacy EthernetNetIf libraries used for a performance comparison with the new networking libraries
Dependencies: EthernetNetIf mbed
Fork of EchoServer by
EchoServer.h
00001 #ifndef ECHO_SERVER_H 00002 #define ECHO_SERVER_H 00003 00004 #include "mbed.h" 00005 #include "TCPSocket.h" 00006 #include "UDPSocket.h" 00007 #include "EthernetNetIf.h" 00008 00009 #include "TCPEchoHandler.h" 00010 #include <netservice.h> 00011 /* 00012 Class: EchoServer 00013 Binds itself to port 7 on TCP and UDP and listens for 00014 incoming connections 00015 */ 00016 class EchoServer { 00017 public: 00018 // Constructor: EchoServer 00019 // Creates the TCP and UDP sockets and wires up 00020 // the event callback methods 00021 EchoServer(); 00022 // Destructor: ~EchoServer 00023 // Deletes the TCP and UDP sockets 00024 ~EchoServer(); 00025 /* 00026 Function: bind 00027 Binds the sockets to the specified ports 00028 Parameters: 00029 tcpPort - The TCP port to bind to (default 7 as per RFC862) 00030 udpPort - The UDP port to bind to (default 7 as per RFC862) 00031 */ 00032 void bind(int tcpPort=7, int udpPort=7); 00033 private: 00034 // Variable: tcpSock 00035 // The TCP server socket 00036 TCPSocket* tcpSock; 00037 // Variable: udpSock 00038 // The UDP socket 00039 UDPSocket* udpSock; 00040 00041 // Function: onNetTcpSocketEvent 00042 // The callback function called by the network stack whenever an 00043 // event occurs on the TCP socket 00044 // Parameter: e - The event that has occurred 00045 void onNetTcpSocketEvent(TCPSocketEvent e); 00046 // Function: onNetUdpSocketEvent 00047 // The callback function called by the network stack whenever an 00048 // event occurs on the UDP socket 00049 // Parameter: e - The event that has occurred 00050 void onNetUdpSocketEvent(UDPSocketEvent e); 00051 }; 00052 00053 #endif
Generated on Tue Jul 26 2022 23:07:02 by
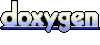