Official library for the FRDM-TFC shield
Dependents: TFC-TEST TFC-RACING-DEMO TFC-RACING-DEMO TFC-RACING-FSLMENTORMATTERS ... more
Resources used by the TFC Library
.
More...
Functions | |
void | TFC_Init () |
Initialized the TFC API. | |
uint8_t | TFC_GetDIP_Switch () |
Gets the state of the 4-positiomn DIP switch on the FRDM-TFC. | |
uint8_t | TFC_ReadPushButton (uint8_t Index) |
Reads the state of the pushbuttons (SW1, SW2) on the FRDM-TFC. | |
void | TFC_SetBatteryLED (uint8_t Value) |
Controls the 4 battery level LEDs on the FRDM-TFC boards. | |
void | TFC_SetServo (uint8_t ServoNumber, float Position) |
Sets the servo channels. | |
void | TFC_InitServos (float ServoPulseWidthMin, float ServoPulseWidthMax, float ServoPeriod) |
Initializes TPM for the servoes. | |
void | TFC_InitMotorPWM (float SwitchingFrequency) |
Initialized TPM0 to be used for generating PWM signals for the the dual drive motors. | |
void | TFC_SetMotorPWM (float MotorA, float MotorB) |
Sets the PWM value for each motor. | |
float | TFC_ReadPot (uint8_t Channel) |
Reads the potentiometers. | |
float | TFC_ReadBatteryVoltage () |
Gets the current battery voltage. | |
void | TFC_SetBatteryLED_Level (uint8_t BattLevel) |
Sets the Battery level indiciate. | |
Variables | |
volatile uint32_t | TFC_ServoTicker |
ServoTicker will increment once every servo cycle. | |
volatile uint16_t * | TFC_LineScanImage0 |
Pointer to two channels of line scan camera data. | |
volatile uint8_t | TFC_LineScanImageReady |
This flag will increment when a new frame is ready. |
Detailed Description
Resources used by the TFC Library
.
I/O:
-------------------------------------------------------------------------------------------------
PTB0 (Servo Channel 0 - TPM1)
PTB1 (Servo Channel 1 - TPM1)
PTB8 (Battery LED0)
PTB9 (Battery LED1)
PTB10 (Battery LED2)
PTB11 (Battery LED3)
PTD7 (Camera SI)
PTE0 (Camera CLK)
PTD5 (Camera A0 - ADC_SE6b)
PTD6 (Camera A1 - ADC_SE7b)
PTE2 DIP Switch 0
PTE3 DIP Switch 1
PTE4 DIP Switch 2
PTE5 DIP Switch 3
PTC13 Pushbutton SW1
PTC17 Pushbutton SW2
PTC3 H-Bridge A - 1 FTM0_CH3
PTC4 H-Bridge A - 2 FTM0_CH4
PTC1 H-Bridge B - 1 FTM0_CH1
PTC2 H-Bridge B - 2 FTM0_CH2
PTE21 H-Bridge Enable
PTE20 H-Bridge Fault
PTE23 H-Bridge A - IFB
PTE22 H-Bridge B - IFB
}
Function Documentation
uint8_t TFC_GetDIP_Switch | ( | ) |
void TFC_Init | ( | ) |
void TFC_InitMotorPWM | ( | float | SwitchingFrequency ) |
Initialized TPM0 to be used for generating PWM signals for the the dual drive motors.
This method is called in the TFC constructor with a default value of 4000.0Hz
- Parameters:
-
SwitchingFrequency PWM Switching Frequency in floating point format. Pick something between 1000 and 9000. Maybe you can modulate it and make a tune.
void TFC_InitServos | ( | float | ServoPulseWidthMin, |
float | ServoPulseWidthMax, | ||
float | ServoPeriod | ||
) |
Initializes TPM for the servoes.
It also sets the max and min ranges
- Parameters:
-
ServoPulseWidthMin Minimum pulse width (in seconds) for the servo. The value of -1.0 in SetServo is mapped to this pulse width. I.E. .001 ServoPulseWidthMax Maximum pulse width (in seconds) for the servo. The value of +1.0 in SetServo is mapped to this pulse width. I.E. .002 ServoPeriod Period of the servo pulses (in seconds). I.e. .020 for 20mSec
float TFC_ReadBatteryVoltage | ( | ) |
float TFC_ReadPot | ( | uint8_t | Channel ) |
uint8_t TFC_ReadPushButton | ( | uint8_t | Index ) |
void TFC_SetBatteryLED | ( | uint8_t | Value ) |
void TFC_SetBatteryLED_Level | ( | uint8_t | BattLevel ) |
void TFC_SetMotorPWM | ( | float | MotorA, |
float | MotorB | ||
) |
Sets the PWM value for each motor.
- Parameters:
-
MotorA The PWM value for HBridgeA. The value is normalized to the floating point range of -1.0 to +1.0. -1.0 is 0% (Full Reverse on the H-Bridge) and 1.0 is 100% (Full Forward on the H-Bridge) MotorB The PWM value for HBridgeB. The value is normalized to the floating point range of -1.0 to +1.0. -1.0 is 0% (Full Reverse on the H-Bridge) and 1.0 is 100% (Full Forward on the H-Bridge)
void TFC_SetServo | ( | uint8_t | ServoNumber, |
float | Position | ||
) |
Sets the servo channels.
- Parameters:
-
ServoNumber Which servo channel on the FRDM-TFC to use (0 or 1). 0 is the default channel for steering Position Angle setting for servo in a normalized (-1.0 to 1.0) form. The range of the servo can be changed with the InitServos function. This is called in the TFC constructor with some useful default values--> 20mSec period, 0.5mS min and 2.0mSec max. you may need to adjust these for your own particular setup.
Variable Documentation
volatile uint16_t* TFC_LineScanImage0 |
volatile uint8_t TFC_LineScanImageReady |
volatile uint32_t TFC_ServoTicker |
ServoTicker will increment once every servo cycle.
It can be used to synchronize events to the start of a servo cycle. ServoTicker is a volatile uint32_t and is updated in the TPM1 overlflow interrupt. This means you will see ServoTicker increment on the rising edge of the servo PWM signal
Generated on Thu Jul 14 2022 19:34:25 by
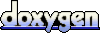