
Example project for the Rioux Chem control box
Embed:
(wiki syntax)
Show/hide line numbers
CHEM_BOX_INTERFACE.h
00001 #include "MODSERIAL.h" 00002 00003 #ifndef CHEM_BOX_INTERFACE 00004 #define CHEM_BOX_INTERFACE 00005 00006 #define API_VERSION "1.1" 00007 00008 // _____ _ _ ______ __ __ ____ ______ __ _____ _____ 00009 // / ____| | | | ____| \/ | | _ \ / __ \ \ / / /\ | __ \_ _| 00010 // | | | |__| | |__ | \ / | | |_) | | | \ V / / \ | |__) || | 00011 // | | | __ | __| | |\/| | | _ <| | | |> < / /\ \ | ___/ | | 00012 // | |____| | | | |____| | | | | |_) | |__| / . \ / ____ \| | _| |_ 00013 // \_____|_| |_|______|_| |_| |____/ \____/_/ \_\ /_/ \_\_| |_____| 00014 // 00015 // 00016 00017 //Initializes the hardware for the Chem box. Must be called before any other CHEM box functions are called. 00018 void InitChemBox(); 00019 00020 extern DigitalOut RED_LED; 00021 00022 extern DigitalOut GREEN_LED; 00023 00024 //Special Note.... All of the Digital IO functions are queued. This means that they do not take effect 00025 //until the function FlushDigitalIO() is called. 00026 00027 00028 //Enables one of the 8 heater channels. Just pass a number between 0 and 7 00029 void EnableHeater(uint8_t RelayIndex); 00030 00031 00032 //Disables one of the 8 heater channels. Just pass a number between 0 and 7 00033 void DisableHeater(uint8_t RelayIndex); 00034 00035 //Enables one of the 12 Solenoid valve channels. Just pass a number between 0 and 12 00036 void EnableSolenoidValve(uint8_t SolenoidIndex); 00037 00038 //Disables one of the 12 Solenoid valve channels. Just pass a number between 0 and 12 00039 void DisableSolenoidValue(uint8_t SolenoidIndex); 00040 00041 //Enables one of the 4 digital I/O channels. Just pass a number between 0 and 3 00042 void EnableMiscDigitalOutput(uint8_t DigitalOutIndex); 00043 00044 //Disables one of the 4 digital I/O channels. Just pass a number between 0 and 3 00045 void DisableMiscDigitalOutput(uint8_t DigitalOutIndex); 00046 00047 //Updates all of the Digital I/O, heater and solenoid channels. Call this function to enable all the queued up I/O commands 00048 void FlushDigitalIO(); 00049 00050 //Immediatelly disables all heaters and Solenoids. FlushDigitalIO() does not need to be called for this to have an effect 00051 void DisableAllHeatersAndSolenoids(); 00052 00053 //Enables the +/-15v power to the MFC Channels 00054 void EnableMFC_Power(); 00055 00056 //Disbales the +/-15v power to the MFC Channels 00057 void DisableMFC_Power(); 00058 00059 //Writes to one of the MFC Analog output channels. The Value given to the function is in volts 00060 void WriteMFC_AnalogOut(uint8_t Channel,float Value); 00061 00062 //Reads on the MFC Analog input channels. The value returned is in volts 00063 float ReadMFC_AnalogInput(uint8_t Channel); 00064 00065 //Reads a 4 to 20mA channel. value returned is in amps (.004 to .020) 00066 float Read4to20(uint8_t Channel); 00067 00068 //Turns the Fan on 00069 void EnableFan(); 00070 00071 //Turns the Fan off(); 00072 void DisableFan(); 00073 00074 // Makes the buzzer ring for a time period (in seconds) 00075 //Note that this function immediately exits and the beeping is done in the background 00076 //If you want to beep a couple times, you will need to delay for a certain time period after you call this function 00077 //before you can call it again 00078 void Buzz(float Time); 00079 00080 //reads a thermocouple. Returns temperature in degrees C 00081 float ReadThermocouple(uint8_t ThermocoupleIndex); 00082 00083 //Determines if a thermocouple is disconnected. Each BIT in the return will be set if the Thermocouple is open. 00084 //so, to see if a particular thermocouple is no connected, you need to bitmask the result. 00085 /* 00086 OC_State = ReadThermocouple_OC(); 00087 00088 if(OC_State & (1<<5)) 00089 { 00090 //we are here if thermocouple 5 is not connected. The if statement does a bitwise AND to check the bit 00091 } 00092 00093 */ 00094 uint16_t ReadThermocouple_OC(); 00095 00096 00097 00098 //Determines if a thermocouple is shorted to ground. Each BIT in the return will be set if the Thermocouple is sorted to ground. 00099 //so, to see if a particular thermocouple is shorted to ground, you need to bitmask the result. 00100 /* 00101 SCG_State = ReadThermocouple_SCG(); 00102 00103 if(SCG_State & (1<<7)) 00104 { 00105 //we are here if thermocouple 7 is shorted to ground. The if statement does a bitwise AND to check the bit 00106 } 00107 00108 */ 00109 uint16_t ReadThermocouple_SCG(); 00110 00111 //Determines if a thermocouple is shorted to VCC. Each BIT in the return will be set if the Thermocouple is shorted to VCC. 00112 //so, to see if a particular thermocouple is shorted to VCC, you need to bitmask the result. 00113 /* 00114 SCV_State = ReadThermocouple_SCG(); 00115 00116 if(SCv_State & (1<<4)) 00117 { 00118 //we are here if thermocouple 5 is shorted to VCC. The if statement does a bitwise AND to check the bit 00119 } 00120 00121 */ 00122 uint16_t ReadThermocouple_SCV(); 00123 00124 00125 00126 //Determines if a thermocouple is faulted (see MAX31855 Datasheet). Each BIT in the return will be set if the Thermocouple is faulted 00127 //so, to see if a particular thermocouple is faulted, you need to bitmask the result. 00128 /* 00129 Faulted_State = ReadThermocouple_FAULT(); 00130 00131 if(Faulted_State & (1<<10)) 00132 { 00133 //we are here if thermocouple 10 is faulted The if statement does a bitwise AND to check the bit 00134 } 00135 00136 */ 00137 00138 00139 uint16_t ReadThermocouple_FAULT(); 00140 00141 /* 00142 Read the internal reference temperature 00143 */ 00144 float ReadInternalTemperature(uint8_t ThermocoupleIndex); 00145 00146 //Writes to one of the Misc Analog Output channels. The Value shoul dbe in Volts (from 0 to 5v) 00147 void WriteMISC_AnalogOut(uint8_t Channel,float Value); 00148 00149 //Reads one of the MISC Analog Input channels. The value returned will be in volts (from 0 to 5v) 00150 float ReadMISC_AnalogInput(uint8_t Channel); 00151 00152 //This function must be called in your main loop if you want the USB terminal interface to work! 00153 void ProcessTerminal(); 00154 00155 00156 //This is the Serial object (see MODSERIAL Documentation on the MBED site for details) for RS-232 channel 0 00157 extern MODSERIAL RS232_0; 00158 00159 //This is the Serial object (see MODSERIAL Documentation on the MBED site for details) for RS-232 channel 0 00160 extern MODSERIAL RS232_1; 00161 00162 //This is the serial object for the USB connection. Used by the Serial terminal 00163 extern MODSERIAL PC; 00164 00165 //Process incomings lines from the PUmp 00166 void ProcessRS232(); 00167 00168 #endif
Generated on Sun Jul 24 2022 04:18:19 by
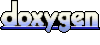