
Simple HTTP Client with JSON parser
Dependencies: MbedJSONValue WIZnetInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "MbedJSONValue.h" 00004 00005 MbedJSONValue parser; 00006 EthernetInterface eth; 00007 TCPSocketConnection sock; 00008 00009 int main() 00010 { 00011 int http_tx_msg_sz=800; 00012 char http_tx_msg[http_tx_msg_sz]; 00013 int http_rx_msg_sz=500; 00014 char http_rx_msg[http_rx_msg_sz]; 00015 int returnCode = 0; 00016 00017 // Enter a MAC address for your controller below. 00018 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x00, 0x01, 0x02}; 00019 00020 printf("initializing Ethernet\r\n"); 00021 // initializing MAC address 00022 eth.init(mac_addr, "192.168.0.34", "255.255.255.0", "192.168.0.1"); 00023 00024 // Check Ethenret Link 00025 if(eth.link() == true) printf("- Ethernet PHY Link-Done \r\n"); 00026 else printf("- Ethernet PHY Link- Fail\r\n"); 00027 00028 // Start Ethernet connecting: Trying to get an IP address using DHCP 00029 if (eth.connect()<0) printf("Fail - Ethernet Connecing"); 00030 00031 // Print your local IP address: 00032 printf("IP=%s\n\r",eth.getIPAddress()); 00033 printf("MASK=%s\n\r",eth.getNetworkMask()); 00034 printf("GW=%s\n\r",eth.getGateway()); 00035 00036 while(1) { 00037 sock.connect("192.168.0.223", 8000); 00038 00039 snprintf(http_tx_msg, http_tx_msg_sz, "GET / HTTP/1.1\r\nHost: 192.168.0.223:8000\r\nUser-Agent: WIZwiki-W7500ECO\r\nConection: close\r\n\r\n"); 00040 sock.send_all(http_tx_msg, http_tx_msg_sz-1); 00041 00042 while ( (returnCode = sock.receive(http_rx_msg, http_rx_msg_sz-1)) > 0) { 00043 http_rx_msg[returnCode] = '\0'; 00044 printf("Received %d chars from server:\n\r%s\n", returnCode, http_rx_msg); 00045 } 00046 00047 sock.close(); 00048 00049 parse(parser, http_rx_msg); 00050 printf("name =%s\r\n" , parser["name"].get<string>().c_str()); 00051 printf("age =%d\r\n" , parser["age"].get<int>()); 00052 printf("gender =%s\r\n" , parser["gender"].get<string>().c_str()); 00053 00054 wait(10); 00055 } 00056 00057 }
Generated on Thu Jul 14 2022 19:59:59 by
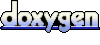