
Code Demonstrate most of the feature of application board/ /like LED, Speaker,RGB,Joystick,LCD,POT,Accelerometer,temperature sensor/ /by using component of real time operating system such as Mutex, Threads/ Signals,Queue and Memory pool Video at https://www.youtube.com/watch?v=c0pRzfZGfV0
Dependencies: C12832_lcd LCD_fonts LM75B MMA7660 MMA7660FC mbed-rtos mbed
main.cpp
00001 00002 /************Author embedded nerd**********************/ 00003 /*********www.embedded.zettamonk.com*******************/ 00004 /**************Free to use Lic************************/ 00005 /*************Date 28 Aug 2014***********************/ 00006 /* Code Demonstarte most of the feature of application board/ 00007 /like LED, Speaker,RGB,Joystick,LCD,POT,Accelomenter,temprature sensor/ 00008 /by using component of real time oprating system such as Mutex, Threads/ 00009 Signals,Queue and Memorypool//////////////////////////////////////////*/ 00010 00011 /////////////////////////////////////////Header Files////////////////////// 00012 #include "mbed.h" 00013 #include "rtos.h" // real time os 00014 #include "Small_6.h" // LCD 00015 #include "Small_7.h" // LCD 00016 #include "Arial_9.h" // LCD 00017 #include "stdio.h" 00018 #include "C12832_lcd.h" // LCD 00019 #include "LM75B.h" // temprature 00020 #include "MMA7660FC.h" 00021 #include "MMA7660.h" // Axix 00022 #include <string> 00023 #define SET 1 00024 #define RESET 0 00025 #define ADDR_MMA7660 0x98 //// I2C SLAVE ADDR MMA7660FC 00026 00027 00028 /****************************Defination and Object Creation*****************************/ 00029 C12832_LCD LCD; // LCD object 00030 BusOut leds(LED1,LED2,LED3,LED4); // Led decration 00031 AnalogIn Pot1(p19); // Analog pin name decration 00032 AnalogIn Pot2(p20); // Analog pin name decration 00033 BusIn Up(p15); //Joy stick 00034 BusIn Down(p12); //Joy stick 00035 BusIn Left(p13); //Joy stick 00036 BusIn Right(p16); //Joy stick 00037 BusOut Reset(p14); //Joy stick 00038 PwmOut spkr(p26); //Speaker 00039 PwmOut r (p23); //RGB red 00040 PwmOut g (p24); //RGB green 00041 PwmOut b (p25); // RGB blue 00042 LM75B sensor(p28,p27); // I2c 00043 MMA7660FC Acc(p28, p27, ADDR_MMA7660); //sda, scl, Addr 00044 MMA7660 MMA(p28, p27); 00045 /*****************************************************************************************/ 00046 //r.period(0); 00047 00048 // 0 1 2 3 4 5 6 7 00049 const string display_menu[] = {" ","LED ","POT ","Temp ","Axix ","Spker","RGB ","POT2"}; // aray to display modules on LCD 00050 unsigned char oprate_menu[] = {0, 0, 0, 0, 0, 0, 0, 0}; // Store Flags for module 00051 00052 00053 typedef struct { 00054 float voltage; 00055 float current; 00056 uint32_t counter; 00057 } message_t; 00058 MemoryPool<message_t, 16> mpool; 00059 Queue<message_t, 16> queue; 00060 00061 00062 //Mutex decleration of LCD 00063 Mutex lcd_mutex; 00064 00065 // Semaphore Declearation of Calcualtion with two slots 00066 Semaphore calculate(2); 00067 int count= 0,menu = 0; 00068 /*////////////////////// Thread led///////////////////////// 00069 Input: 00070 Output: LED 00071 if module flag is set than it will turn led on from 0X00 to 0X0F 00072 //////////////////////////////////////////////////////////*/ 00073 void led(void const *args) 00074 { 00075 00076 char out =0; 00077 while(true) { // thread loop 00078 if (oprate_menu[1] == SET) // Checking flag for module 00079 { 00080 leds = out; // Turning LEd on 00081 out++; 00082 if(out>16) out = 0; // Reseting count 00083 } 00084 else leds = 0; // If flag not set than turn of the module 00085 Thread::wait(100); // Thread wait .1s 00086 } 00087 00088 } // thread close 00089 00090 /*////////////////////// Thread menu_sel///////////////////////// 00091 Input: Joustick 00092 Output: Set flag in oprate_menu 00093 By using left right key user in enable to change module and by up 00094 cown key user is able to set module on or off bye pressinf enter key 00095 //////////////////////////////////////////////////////////*/ 00096 void menu_sel(void const *args) 00097 { 00098 int k; 00099 while(true) { // thread loop 00100 //LCD.locate(0,5);LCD.printf(" "); 00101 00102 LCD.set_font((unsigned char*) Small_7); 00103 if (Left) // Checking keys 00104 { 00105 count = 0 ; 00106 menu--; // manipulating menue number as per key response 00107 if(menu<1){ menu = 7;} // 00108 lcd_mutex.lock(); 00109 LCD.locate(50,10); 00110 LCD.printf(" %s",display_menu[menu]); // displaying menue on LCD 00111 lcd_mutex.unlock(); 00112 wait(1); 00113 } 00114 if (Right) 00115 { 00116 count = 0 ; 00117 menu++; 00118 if(menu>7) {menu = 1;} 00119 00120 lcd_mutex.lock(); 00121 LCD.locate(50,10); 00122 LCD.printf(" %s",display_menu[menu]); 00123 lcd_mutex.unlock(); 00124 wait(1); 00125 //while(Right); 00126 } 00127 if(Up) 00128 { 00129 count = 1 ; // Manipulating flag as per selection from user 00130 LCD.set_font((unsigned char*) Small_7); 00131 lcd_mutex.lock(); 00132 LCD.locate(50,20); 00133 LCD.printf(" ON "); 00134 lcd_mutex.unlock(); 00135 } 00136 if(Down) 00137 { 00138 count = 0; 00139 LCD.set_font((unsigned char*) Small_7); 00140 lcd_mutex.lock(); 00141 LCD.locate(50,20); 00142 LCD.printf(" OFF"); 00143 lcd_mutex.unlock(); 00144 } 00145 if (Reset) 00146 { 00147 LCD.set_font((unsigned char*) Small_7); 00148 lcd_mutex.lock(); 00149 LCD.locate(50,20); 00150 LCD.printf(" "); 00151 lcd_mutex.unlock(); 00152 oprate_menu[menu] = count; 00153 //if menu = 1 thread.signal_set(0x1); 00154 } 00155 00156 Thread::wait(10); // wait 0.5s 00157 } 00158 } 00159 00160 00161 /*////////////////////// Thread spk///////////////////////// 00162 Input: 00163 Output: Turn on speaker on board 00164 Turn on PWM on speaker pin for specific period of time 00165 //////////////////////////////////////////////////////////*/ 00166 void spk(void const *args) 00167 { 00168 while (1) { 00169 if (oprate_menu[5] == SET) 00170 { 00171 for (float i=2000.0; i<10000.0; i+=500) { 00172 spkr.period(1.0/i); 00173 spkr=0.5; 00174 wait(0.1);} 00175 } 00176 else {spkr.period(0); spkr=0;} 00177 Thread::wait(50); 00178 } 00179 00180 } 00181 00182 00183 /*////////////////////// Thread pot///////////////////////// 00184 Input: Analog in 00185 Output: 00186 Read the analog value and manupulate it for further use 00187 //////////////////////////////////////////////////////////*/ 00188 void pot(void const *args) 00189 { 00190 int k; 00191 while(true) // thread loop 00192 { 00193 if (oprate_menu[2] == SET) 00194 { 00195 k = Pot1.read_u16(); // get the value of poti 2 00196 k = k >> 5; // we need only 6 bit for contrast 00197 lcd_mutex.lock(); 00198 LCD.set_font((unsigned char*) Small_6); 00199 LCD.locate(0,10); 00200 LCD.printf("Pot: %d",k); 00201 lcd_mutex.unlock(); 00202 //Thread::wait(500); // wait 0.5s 00203 } 00204 else 00205 { 00206 lcd_mutex.lock(); 00207 LCD.set_font((unsigned char*) Small_6); 00208 LCD.locate(0,10); 00209 LCD.printf(" "); 00210 lcd_mutex.unlock(); 00211 } 00212 Thread::wait(50); 00213 } 00214 } 00215 /*////////////////////// Thread pot///////////////////////// 00216 Input: 00217 Output: PWM output to RGB led Pin 00218 Multicolor LED display diffrent colors as per PWM cycle in set 00219 //////////////////////////////////////////////////////////*/ 00220 void rgb(void const *args) 00221 { 00222 while(1) { 00223 if (oprate_menu[6] == SET) 00224 { 00225 00226 r = 0.5 + (rand() % 11)/20.0; 00227 g = 0.5 + (rand() % 11)/20.0; 00228 b = 0.5 + (rand() % 11)/20.0; 00229 00230 } 00231 00232 else {r =.1;g=0;b=0;} 00233 Thread::wait(500); 00234 } 00235 } 00236 00237 /*////////////////////// Thread temp///////////////////////// 00238 Input: 00239 Output: PWM output to RGB led Pin 00240 Multicolor LED display diffrent colors as per PWM cycle in set 00241 //////////////////////////////////////////////////////////*/ 00242 00243 void temp(void const *args) 00244 { 00245 00246 int k; 00247 while(true) // thread loop 00248 { 00249 if (oprate_menu[3] == SET) 00250 { 00251 lcd_mutex.lock(); 00252 LCD.set_font((unsigned char*) Small_6); 00253 LCD.locate(0,20); 00254 LCD.printf("Temp:%.1f", (float)sensor); 00255 lcd_mutex.unlock(); 00256 Thread::wait(50); 00257 } 00258 else 00259 { 00260 lcd_mutex.lock(); 00261 LCD.set_font((unsigned char*) Small_6); 00262 LCD.locate(0,20); 00263 LCD.printf(" "); 00264 lcd_mutex.unlock(); 00265 Thread::wait(50); 00266 } 00267 00268 00269 Thread::wait(50); // wait 0.5s 00270 } 00271 } 00272 00273 /*////////////////////// Thread AXIX///////////////////////// 00274 Input: I2C data 00275 Output: 00276 Draw a circle and a buble in it. Buble move with respond to 00277 change in axix of board 00278 //////////////////////////////////////////////////////////*/ 00279 void axix(void const *args) 00280 { 00281 // Acc.init(); 00282 float x=0, y=0, z=0; 00283 while(1) 00284 { 00285 //int x=0,y=0; 00286 if (oprate_menu[4] == SET) 00287 { 00288 00289 Acc.read_Tilt(&x, &y, &z); 00290 lcd_mutex.lock(); 00291 x = (x + MMA.x() * 32.0)/2.0; 00292 y = (y -(MMA.y() * 16.0))/2.0; 00293 // if(y>25) y = 0; //else if(y<0) y = 0; 00294 if(y>8) y =8; if(y<-8) y =-8; if(x>8) x =8; if(x<-8) x =-8; // confining 00295 LCD.fillcircle(x+115, y+17, 2, 1); //draw bubble 00296 LCD.circle(115, 17, 8, 1); 00297 wait(.1); //time delay 00298 LCD.fillcircle(x+115, y+17, 2, 0); //erase bubble 00299 00300 lcd_mutex.unlock(); 00301 // LCD.locate(0,3); 00302 wait_ms(100); 00303 } 00304 else 00305 { 00306 lcd_mutex.lock(); 00307 LCD.circle(115, 17, 8, 0); 00308 // LCD.locate(0,3); 00309 lcd_mutex.unlock(); 00310 } 00311 Thread::wait(10); 00312 } 00313 } 00314 00315 /*////////////////////// Memory pool example and Semaphore/////////////// 00316 Output: 00317 00318 //////////////////////////////////////////////////////////*/ 00319 void calci(void const *args) { 00320 uint32_t i = 0; 00321 while (true) { 00322 calculate.wait(); //semaphore 00323 i = Pot2.read_u16();; 00324 i = (i>>5)/2; 00325 message_t *message = mpool.alloc(); 00326 message->voltage = (i * 3.3) / 1024; 00327 message->current = ((i * 3.3) / 2048); 00328 message->counter = i; 00329 queue.put(message); 00330 calculate.release(); 00331 Thread::wait(100000); 00332 } 00333 } 00334 00335 // print the actual contrast 00336 int main() 00337 { 00338 int j; 00339 // r =0; b=0;g=0; 00340 LCD.cls(); 00341 LCD.set_font((unsigned char*) Small_7); 00342 LCD.locate(0,0); 00343 LCD.printf(" embedded.zettamonk.com"); 00344 /////////////////////////////Threads/////////////////// 00345 Thread t1(led); //start thread1 00346 Thread t2(menu_sel); //start thread2 00347 Thread t3(spk); //start thread3 00348 Thread t4(pot); //start thread4 00349 Thread t5(rgb); //start thread4 00350 Thread t6(temp); //start thread4 00351 Thread t7(axix); 00352 Thread t8(calci); 00353 Thread t9(calci); 00354 //////////////////////////////////////////////// 00355 while(true) { // main is the next thread 00356 00357 if (oprate_menu[7] == SET) 00358 { 00359 LCD.set_font((unsigned char*) Small_7); 00360 LCD.locate(0,0); 00361 00362 osEvent evt = queue.get(); 00363 if (evt.status == osEventMessage) { 00364 message_t *message = (message_t*)evt.value.p; 00365 lcd_mutex.lock(); 00366 LCD.printf(" V:%.2fv" , message->voltage); 00367 LCD.printf(" I:%.2fA" , message->current); 00368 LCD.printf(" R: %u ", message->counter); 00369 lcd_mutex.unlock(); 00370 mpool.free(message); 00371 00372 00373 // wait(.2); 00374 } 00375 else 00376 { LCD.set_font((unsigned char*) Small_7); 00377 LCD.locate(0,0); 00378 lcd_mutex.lock(); 00379 LCD.printf("embedded.zettamonk.com"); 00380 lcd_mutex.unlock(); 00381 } 00382 00383 Thread::wait(100); // wait 0.5s 00384 } 00385 } 00386 }
Generated on Tue Jul 19 2022 03:49:22 by
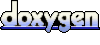