
Example showing the ublox Cellular GPS/GNSS module with the HTTPClient library to fetch and upload web pages.
Dependencies: C027_Support HTTPClient mbed
main.cpp
00001 #include "mbed.h" 00002 #include "HTTPClient.h" 00003 00004 //------------------------------------------------------------------------------------ 00005 // You need to configure these cellular modem / SIM parameters. 00006 // These parameters are ignored for LISA-C200 variants and can be left NULL. 00007 //------------------------------------------------------------------------------------ 00008 #include "MDM.h" 00009 //! Set your secret SIM pin here (e.g. "1234"). Check your SIM manual. 00010 #define SIMPIN NULL 00011 /*! The APN of your network operator SIM, sometimes it is "internet" check your 00012 contract with the network operator. You can also try to look-up your settings in 00013 google: https://www.google.de/search?q=APN+list */ 00014 #define APN "online.telia.se" 00015 //! Set the user name for your APN, or NULL if not needed 00016 #define USERNAME NULL 00017 //! Set the password for your APN, or NULL if not needed 00018 #define PASSWORD NULL 00019 //------------------------------------------------------------------------------------ 00020 00021 char str[512]; 00022 00023 int main() 00024 { 00025 // turn on the supplies of the Modem 00026 MDMSerial mdm; 00027 //mdm.setDebug(4); // enable this for debugging issues 00028 if (!mdm.connect(SIMPIN, APN,USERNAME,PASSWORD)) 00029 return -1; 00030 HTTPClient http; 00031 00032 //GET data 00033 printf("\nTrying to fetch page...\n"); 00034 int ret = http.get("http://mbed.org/media/uploads/donatien/hello.txt", str, 128); 00035 if (!ret) 00036 { 00037 printf("Page fetched successfully - read %d characters\n", strlen(str)); 00038 printf("Result: %s\n", str); 00039 } 00040 else 00041 { 00042 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00043 } 00044 00045 //POST data 00046 HTTPMap map; 00047 HTTPText inText(str, 512); 00048 map.put("Hello", "World"); 00049 map.put("test", "1234"); 00050 printf("\nTrying to post data...\n"); 00051 ret = http.post("http://httpbin.org/post", map, &inText); 00052 if (!ret) 00053 { 00054 printf("Executed POST successfully - read %d characters\n", strlen(str)); 00055 printf("Result: %s\n", str); 00056 } 00057 else 00058 { 00059 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00060 } 00061 00062 //PUT data 00063 strcpy(str, "This is a PUT test!"); 00064 HTTPText outText(str); 00065 //HTTPText inText(str, 512); 00066 printf("\nTrying to put resource...\n"); 00067 ret = http.put("http://httpbin.org/put", outText, &inText); 00068 if (!ret) 00069 { 00070 printf("Executed PUT successfully - read %d characters\n", strlen(str)); 00071 printf("Result: %s\n", str); 00072 } 00073 else 00074 { 00075 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00076 } 00077 00078 //DELETE data 00079 //HTTPText inText(str, 512); 00080 printf("\nTrying to delete resource...\n"); 00081 ret = http.del("http://httpbin.org/delete", &inText); 00082 if (!ret) 00083 { 00084 printf("Executed DELETE successfully - read %d characters\n", strlen(str)); 00085 printf("Result: %s\n", str); 00086 } 00087 else 00088 { 00089 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00090 } 00091 00092 mdm.disconnect(); 00093 mdm.powerOff(); 00094 00095 while(true); 00096 }
Generated on Tue Jul 12 2022 21:17:12 by
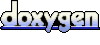