Library to control the EM027BS013 ePaper display from Pervasive Display.
Dependents: app_epaper_EM027BS013_LPC1549 lpc4088_ebb_epaper EaEpaper_EM027BS013 app_epaper_EM027BS013 ... more
EPD_hardware_gpio.h
00001 /** 00002 * \file 00003 * 00004 * \brief The definition of EPD GPIO pins 00005 * 00006 * Copyright (c) 2012-2014 Pervasive Displays Inc. All rights reserved. 00007 * 00008 * Authors: Pervasive Displays Inc. 00009 * 00010 * Redistribution and use in source and binary forms, with or without 00011 * modification, are permitted provided that the following conditions 00012 * are met: 00013 * 00014 * 1. Redistributions of source code must retain the above copyright 00015 * notice, this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright 00017 * notice, this list of conditions and the following disclaimer in 00018 * the documentation and/or other materials provided with the 00019 * distribution. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00022 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00023 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00024 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00025 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00026 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00027 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00028 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00029 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00030 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 */ 00033 00034 #include "Pervasive_Displays_small_EPD.h" 00035 00036 #ifndef DISPLAY_HARDWARE_GPIO_H_INCLUDED 00037 #define DISPLAY_HARDWARE_GPIO_H_INCLUDED 00038 00039 #define _BV(bit) (1 << (bit)) /**< left shift 1 bit */ 00040 #define _HIGH 1 /**< signal high */ 00041 #define _LOW !_HIGH /**< signal low */ 00042 00043 ////FOR MSP430 00044 //#define DIR_(x) x ## DIR 00045 //#define DIR(x) (DIR_(x)) 00046 //#define OUT_(x) x ## OUT 00047 //#define OUTPORT(x) (OUT_(x)) 00048 //#define SEL_(x) x ## SEL 00049 //#define SEL(x) (SEL_(x)) 00050 //#define SEL2_(x) x ## SEL2 00051 //#define SEL2(x) (SEL2_(x)) 00052 //#define IN_(x) x ## IN 00053 //#define INPORT(x) (IN_(x)) 00054 //#define REN_(x) x ## REN 00055 //#define REN(x) (REN_(x)) 00056 //#define BITSET(x,y) ((x) |= (y)) 00057 //#define BITCLR(x,y) ((x) &= ~(y)) 00058 //#define BITINV(x,y) ((x) ^= (y)) 00059 00060 //#define config_gpio_dir_o(Port,Pin) BITSET(DIR (Port), Pin) /**< set output direction for an IOPORT pin */ 00061 //#define config_gpio_dir_i(Port,Pin) BITCLR(DIR (Port), Pin) /**< set input direction for an IOPORT pin */ 00062 //#define set_gpio_high(Port,Pin) BITSET (OUTPORT (Port), Pin) /**< set HIGH for an IOPORT pin */ 00063 //#define set_gpio_low(Port,Pin) BITCLR (OUTPORT (Port), Pin) /**< set LOW for an IOPORT pin */ 00064 //#define set_gpio_invert(Port,Pin) BITINV(OUTPORT (Port),Pin) /**< toggle the value of an IOPORT pin */ 00065 //#define input_get(Port,Pin) (INPORT (Port) & Pin ) /**< get current value of an IOPORT pin */ 00066 00067 /****************************************************************************** 00068 * GPIO Defines 00069 *****************************************************************************/ 00070 //#define Temper_PIN BIT4 00071 //#define Temper_PORT P1 /**< LaunchPad P1.4 */ 00072 //#define SPICLK_PIN BIT5 00073 //#define SPICLK_PORT P1 /**< LaunchPad P1.5 */ 00074 //#define EPD_BUSY_PIN BIT0 00075 //#define EPD_BUSY_PORT P2 /**< LaunchPad P2.0 */ 00076 //#define PWM_PIN BIT1 00077 //#define PWM_PORT P2 /**< LaunchPad P2.1 */ 00078 //#define EPD_RST_PIN BIT2 00079 //#define EPD_RST_PORT P2 /**< LaunchPad P2.2 */ 00080 //#define EPD_PANELON_PIN BIT3 00081 //#define EPD_PANELON_PORT P2 /**< LaunchPad P2.3 */ 00082 //#define EPD_DISCHARGE_PIN BIT4 00083 //#define EPD_DISCHARGE_PORT P2 /**< LaunchPad P2.4 */ 00084 //#define EPD_BORDER_PIN BIT5 00085 //#define EPD_BORDER_PORT P2 /**< LaunchPad P2.5 */ 00086 //#define SPIMISO_PIN BIT6 00087 //#define SPIMISO_PORT P1 /**< LaunchPad P1.6 */ 00088 //#define SPIMOSI_PIN BIT7 00089 //#define SPIMOSI_PORT P1 /**< LaunchPad P1.7 */ 00090 //#define Flash_CS_PIN BIT7 00091 //#define Flash_CS_PORT P2 /**< LaunchPad P2.7 */ 00092 //#define Flash_CS_PORT_SEL (SEL (Flash_CS_PORT)) 00093 //#define Flash_CS_PORT_SEL2 (SEL2(Flash_CS_PORT)) 00094 //#define EPD_CS_PIN BIT6 00095 //#define EPD_CS_PORT P2 /**< LaunchPad P2.6 */ 00096 //#define CS_PORT_SEL (SEL (EPD_CS_PORT)) 00097 //#define CS_PORT_SEL2 (SEL2(EPD_CS_PORT)) 00098 00099 bool EPD_IsBusy(void); 00100 void EPD_cs_high (void); 00101 void EPD_cs_low (void); 00102 void EPD_flash_cs_high(void); 00103 void EPD_flash_cs_low (void); 00104 void EPD_rst_high (void); 00105 void EPD_rst_low (void); 00106 void EPD_discharge_high (void); 00107 void EPD_discharge_low (void); 00108 void EPD_Vcc_turn_off (void); 00109 void EPD_Vcc_turn_on (void); 00110 void EPD_border_high(void); 00111 void EPD_border_low (void); 00112 void EPD_pwm_low (void); 00113 void EPD_pwm_high(void); 00114 void SPIMISO_low(void); 00115 void SPIMOSI_low(void); 00116 void SPICLK_low(void); 00117 void EPD_initialize_gpio(void); 00118 00119 #endif //DISPLAY_HARDWARE_GPIO_H_INCLUDED 00120 00121 00122 00123
Generated on Thu Jul 14 2022 17:10:35 by
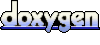