
Test code. Blinks by default at 2 seconds. Receives single characters from PC (sensor) and transmit to "nRF Connect" on droid. Droid can send ascii to Nano. When droid sends "A", blinking is twice per second. "B" returns to slow.
Dependencies: BLE_API mbed nRF51822
Fork of IofT_comm by
main.cpp
00001 00002 /* 00003 * The application works with the BLEController iOS/Android App. 00004 * Type something from the Terminal to send 00005 * to the BLEController App or vice verse. 00006 * Characteristics received from App will print on Terminal. 00007 */ 00008 00009 #include "mbed.h" 00010 #include "ble/BLE.h" 00011 00012 00013 #define BLE_UUID_TXRX_SERVICE 0x0000 /**< The UUID of the Nordic UART Service. */ 00014 #define BLE_UUID_TX_CHARACTERISTIC 0x0002 /**< The UUID of the TX Characteristic. */ 00015 #define BLE_UUIDS_RX_CHARACTERISTIC 0x0003 /**< The UUID of the RX Characteristic. */ 00016 00017 #define TXRX_BUF_LEN 4 00018 00019 BLE ble; 00020 DigitalOut led1(LED1); 00021 Serial pc(USBTX, USBRX); 00022 00023 00024 // The Nordic UART Service 00025 static const uint8_t uart_base_uuid[] = {0x71, 0x3D, 0, 0, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00026 static const uint8_t uart_tx_uuid[] = {0x71, 0x3D, 0, 3, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00027 static const uint8_t uart_rx_uuid[] = {0x71, 0x3D, 0, 2, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00028 static const uint8_t uart_base_uuid_rev[] = {0x1E, 0x94, 0x8D, 0xF1, 0x48, 0x31, 0x94, 0xBA, 0x75, 0x4C, 0x3E, 0x50, 0, 0, 0x3D, 0x71}; 00029 00030 00031 uint8_t txPayload[TXRX_BUF_LEN] = {0,}; 00032 uint8_t rxPayload[TXRX_BUF_LEN] = {0,}; 00033 00034 //static uint8_t rx_buf[TXRX_BUF_LEN]; 00035 static uint8_t rx_buf[4] = "a"; 00036 static uint8_t rx_len=2; 00037 uint8_t blinklow; 00038 uint8_t blinkperiod; 00039 uint8_t blinkrate; 00040 uint8_t blinkcount; 00041 00042 00043 GattCharacteristic txCharacteristic (uart_tx_uuid, txPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00044 00045 GattCharacteristic rxCharacteristic (uart_rx_uuid, rxPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00046 00047 GattCharacteristic *uartChars[] = {&txCharacteristic, &rxCharacteristic}; 00048 00049 GattService uartService(uart_base_uuid, uartChars, sizeof(uartChars) / sizeof(GattCharacteristic *)); 00050 00051 00052 00053 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00054 { 00055 pc.printf("Disconnected \r\n"); 00056 pc.printf("Restart advertising \r\n"); 00057 ble.startAdvertising(); 00058 } 00059 00060 // Serial Write from BLE Read 00061 void WrittenHandler(const GattWriteCallbackParams *Handler) 00062 { 00063 uint8_t buf[TXRX_BUF_LEN]; 00064 uint16_t bytesRead, index; 00065 00066 if (Handler->handle == txCharacteristic.getValueAttribute().getHandle()) 00067 { 00068 led1 = !led1; 00069 ble.readCharacteristicValue(txCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00070 memset(txPayload, 0, TXRX_BUF_LEN); 00071 memcpy(txPayload, buf, TXRX_BUF_LEN); 00072 if(bytesRead==0x01) { 00073 if(txPayload[0] == 0x41){ 00074 pc.printf("Fast \r\n"); 00075 blinkperiod = 5; 00076 } 00077 if(txPayload[0] == 0x42){ 00078 pc.printf("Slow \r\n"); 00079 blinkperiod = 20; 00080 } 00081 } 00082 pc.printf("BLE received = "); 00083 for(index=0; index<bytesRead; index++) 00084 { 00085 pc.putc(txPayload[index]); 00086 } 00087 pc.printf("\r\n"); 00088 } 00089 } 00090 00091 // BLE Write from Serial read 00092 void uartCB(void) 00093 { 00094 while(pc.readable()) 00095 { 00096 rx_buf[0] = pc.getc(); 00097 led1 = !led1; 00098 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), rx_buf, rx_len); 00099 pc.printf("Received sensor data transmitted on BLE "); 00100 pc.putc(rx_buf[0]); 00101 pc.printf(" \r\n"); 00102 } 00103 } 00104 00105 //void u//artCB(void) 00106 // { 00107 // while(pc.readable()) 00108 // { 00109 // rx_buf[rx_len++] = pc.getc(); 00110 // led1 = !led1; 00111 // if(rx_len>=4 || rx_buf[rx_len-1]=='\0' || rx_buf[rx_len-1]=='\n') 00112 // { 00113 // ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), rx_buf, rx_len); 00114 // pc.printf("Received sensor data transmitted on BLE "); 00115 // pc.putc(rx_len); 00116 // pc.printf("\r\n"); 00117 // rx_len = 0; 00118 // break; 00119 // } 00120 // } 00121 //} 00122 00123 void periodicCallback(void) 00124 { 00125 blinkcount = blinkcount + 1; 00126 if(blinkcount >= blinkperiod) 00127 { 00128 led1 = 0; 00129 blinkcount = 0; 00130 pc.printf("Blinked\r\n"); 00131 } 00132 else if(blinkcount > 1) 00133 { 00134 led1 = 1; 00135 } 00136 else 00137 { 00138 led1 = 0; 00139 } 00140 00141 } 00142 00143 int main(void) 00144 { 00145 led1 = 0; 00146 blinkcount = 0; 00147 blinkperiod = 20; 00148 Ticker ticker; 00149 ticker.attach(periodicCallback, 0.1); // blink LED every second 00150 ble.init(); 00151 ble.onDisconnection(disconnectionCallback); 00152 ble.onDataWritten(WrittenHandler); 00153 00154 pc.baud(9600); 00155 pc.printf("IoT Init \r\n"); 00156 00157 pc.attach( uartCB , pc.RxIrq); 00158 // setup advertising 00159 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00160 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00161 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00162 (const uint8_t *)"YSU_bluetooth", sizeof("YSU_bluetooth") - 1); 00163 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00164 (const uint8_t *)uart_base_uuid_rev, sizeof(uart_base_uuid)); 00165 // 100ms; in multiples of 0.625ms. 00166 ble.setAdvertisingInterval(160); 00167 00168 ble.addService(uartService); 00169 00170 ble.startAdvertising(); 00171 pc.printf("Advertising Started \r\n"); 00172 00173 while(1) 00174 { 00175 ble.waitForEvent(); 00176 } 00177 }
Generated on Thu Jul 14 2022 01:30:57 by
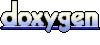