Remove Chip Select transitions. It affects other devices in the same bus.
Embed:
(wiki syntax)
Show/hide line numbers
ADXL345_I2C.cpp
00001 /** 00002 * @author Jose R. Padron 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * ADXL345, triple axis, digital interface, accelerometer. 00029 * 00030 * Datasheet: 00031 * 00032 * http://www.analog.com/static/imported-files/data_sheets/ADXL345.pdf 00033 */ 00034 00035 /** 00036 * Includes 00037 */ 00038 #include "ADXL345_I2C.h" 00039 00040 ADXL345_I2C::ADXL345_I2C(PinName sda, 00041 PinName scl, 00042 PinName cs) : i2c_(sda,scl), nCS_(cs) { 00043 00044 00045 nCS_ = 1; 00046 //100KHz, as specified by the datasheet. 00047 i2c_.frequency(100000); 00048 wait_us(500); 00049 00050 } 00051 00052 char ADXL345_I2C::getDevId(void) { 00053 00054 return oneByteRead(ADXL345_DEVID_REG); 00055 00056 } 00057 00058 int ADXL345_I2C::getTapThreshold(void) { 00059 00060 return oneByteRead(ADXL345_THRESH_TAP_REG); 00061 00062 } 00063 00064 void ADXL345_I2C::setTapThreshold(int threshold) { 00065 00066 oneByteWrite(ADXL345_THRESH_TAP_REG, threshold); 00067 00068 } 00069 00070 int ADXL345_I2C::getOffset(int axis) { 00071 00072 int address = 0; 00073 00074 if (axis == ADXL345_X) { 00075 address = ADXL345_OFSX_REG; 00076 } else if (axis == ADXL345_Y) { 00077 address = ADXL345_OFSY_REG; 00078 } else if (axis == ADXL345_Z) { 00079 address = ADXL345_OFSZ_REG; 00080 } 00081 00082 return oneByteRead(address); 00083 00084 } 00085 00086 void ADXL345_I2C::setOffset(int axis, char offset) { 00087 00088 char address = 0; 00089 00090 if (axis == ADXL345_X) { 00091 address = ADXL345_OFSX_REG; 00092 } else if (axis == ADXL345_Y) { 00093 address = ADXL345_OFSY_REG; 00094 } else if (axis == ADXL345_Z) { 00095 address = ADXL345_OFSZ_REG; 00096 } 00097 00098 return oneByteWrite(address, offset); 00099 00100 } 00101 00102 int ADXL345_I2C::getTapDuration(void) { 00103 00104 return oneByteRead(ADXL345_DUR_REG)*625; 00105 00106 } 00107 00108 void ADXL345_I2C::setTapDuration(int duration_us) { 00109 00110 int tapDuration = duration_us / 625; 00111 00112 oneByteWrite(ADXL345_DUR_REG, tapDuration); 00113 00114 } 00115 00116 float ADXL345_I2C::getTapLatency(void) { 00117 00118 return oneByteRead(ADXL345_LATENT_REG)*1.25; 00119 00120 } 00121 00122 void ADXL345_I2C::setTapLatency(int latency_ms) { 00123 00124 int tapLatency = latency_ms / 1.25; 00125 00126 oneByteWrite(ADXL345_LATENT_REG, tapLatency); 00127 00128 } 00129 00130 float ADXL345_I2C::getWindowTime(void) { 00131 00132 return oneByteRead(ADXL345_WINDOW_REG)*1.25; 00133 00134 } 00135 00136 void ADXL345_I2C::setWindowTime(int window_ms) { 00137 00138 int windowTime = window_ms / 1.25; 00139 00140 oneByteWrite(ADXL345_WINDOW_REG, windowTime); 00141 00142 } 00143 00144 int ADXL345_I2C::getActivityThreshold(void) { 00145 00146 return oneByteRead(ADXL345_THRESH_ACT_REG); 00147 00148 } 00149 00150 void ADXL345_I2C::setActivityThreshold(int threshold) { 00151 00152 oneByteWrite(ADXL345_THRESH_ACT_REG, threshold); 00153 00154 } 00155 00156 int ADXL345_I2C::getInactivityThreshold(void) { 00157 00158 return oneByteRead(ADXL345_THRESH_INACT_REG); 00159 00160 } 00161 00162 void ADXL345_I2C::setInactivityThreshold(int threshold) { 00163 00164 return oneByteWrite(ADXL345_THRESH_INACT_REG, threshold); 00165 00166 } 00167 00168 int ADXL345_I2C::getTimeInactivity(void) { 00169 00170 return oneByteRead(ADXL345_TIME_INACT_REG); 00171 00172 } 00173 00174 void ADXL345_I2C::setTimeInactivity(int timeInactivity) { 00175 00176 oneByteWrite(ADXL345_TIME_INACT_REG, timeInactivity); 00177 00178 } 00179 00180 int ADXL345_I2C::getActivityInactivityControl(void) { 00181 00182 return oneByteRead(ADXL345_ACT_INACT_CTL_REG); 00183 00184 } 00185 00186 void ADXL345_I2C::setActivityInactivityControl(int settings) { 00187 00188 oneByteWrite(ADXL345_ACT_INACT_CTL_REG, settings); 00189 00190 } 00191 00192 int ADXL345_I2C::getFreefallThreshold(void) { 00193 00194 return oneByteRead(ADXL345_THRESH_FF_REG); 00195 00196 } 00197 00198 void ADXL345_I2C::setFreefallThreshold(int threshold) { 00199 00200 oneByteWrite(ADXL345_THRESH_FF_REG, threshold); 00201 00202 } 00203 00204 int ADXL345_I2C::getFreefallTime(void) { 00205 00206 return oneByteRead(ADXL345_TIME_FF_REG)*5; 00207 00208 } 00209 00210 void ADXL345_I2C::setFreefallTime(int freefallTime_ms) { 00211 00212 int freefallTime = freefallTime_ms / 5; 00213 00214 oneByteWrite(ADXL345_TIME_FF_REG, freefallTime); 00215 00216 } 00217 00218 int ADXL345_I2C::getTapAxisControl(void) { 00219 00220 return oneByteRead(ADXL345_TAP_AXES_REG); 00221 00222 } 00223 00224 void ADXL345_I2C::setTapAxisControl(int settings) { 00225 00226 oneByteWrite(ADXL345_TAP_AXES_REG, settings); 00227 00228 } 00229 00230 int ADXL345_I2C::getTapSource(void) { 00231 00232 return oneByteRead(ADXL345_ACT_TAP_STATUS_REG); 00233 00234 } 00235 00236 void ADXL345_I2C::setPowerMode(char mode) { 00237 00238 //Get the current register contents, so we don't clobber the rate value. 00239 char registerContents = oneByteRead(ADXL345_BW_RATE_REG); 00240 00241 registerContents = (mode << 4) | registerContents; 00242 00243 oneByteWrite(ADXL345_BW_RATE_REG, registerContents); 00244 00245 } 00246 00247 int ADXL345_I2C::getPowerControl(void) { 00248 00249 return oneByteRead(ADXL345_POWER_CTL_REG); 00250 00251 } 00252 00253 void ADXL345_I2C::setPowerControl(int settings) { 00254 00255 oneByteWrite(ADXL345_POWER_CTL_REG, settings); 00256 00257 } 00258 00259 int ADXL345_I2C::getInterruptEnableControl(void) { 00260 00261 return oneByteRead(ADXL345_INT_ENABLE_REG); 00262 00263 } 00264 00265 void ADXL345_I2C::setInterruptEnableControl(int settings) { 00266 00267 oneByteWrite(ADXL345_INT_ENABLE_REG, settings); 00268 00269 } 00270 00271 int ADXL345_I2C::getInterruptMappingControl(void) { 00272 00273 return oneByteRead(ADXL345_INT_MAP_REG); 00274 00275 } 00276 00277 void ADXL345_I2C::setInterruptMappingControl(int settings) { 00278 00279 oneByteWrite(ADXL345_INT_MAP_REG, settings); 00280 00281 } 00282 00283 int ADXL345_I2C::getInterruptSource(void){ 00284 00285 return oneByteRead(ADXL345_INT_SOURCE_REG); 00286 00287 } 00288 00289 int ADXL345_I2C::getDataFormatControl(void){ 00290 00291 return oneByteRead(ADXL345_DATA_FORMAT_REG); 00292 00293 } 00294 00295 void ADXL345_I2C::setDataFormatControl(int settings){ 00296 00297 oneByteWrite(ADXL345_DATA_FORMAT_REG, settings); 00298 00299 } 00300 00301 void ADXL345_I2C::setDataRate(int rate) { 00302 00303 //Get the current register contents, so we don't clobber the power bit. 00304 char registerContents = oneByteRead(ADXL345_BW_RATE_REG); 00305 00306 registerContents &= 0x10; 00307 registerContents |= rate; 00308 00309 oneByteWrite(ADXL345_BW_RATE_REG, registerContents); 00310 00311 } 00312 00313 int ADXL345_I2C::getAx(){ 00314 00315 char buffer[2]; 00316 00317 TwoByteRead(ADXL345_DATAX0_REG, buffer); 00318 00319 return ((int)buffer[1] << 8 | (int)buffer[0]); 00320 } 00321 00322 00323 int ADXL345_I2C::getAy(){ 00324 00325 char buffer[2]; 00326 00327 TwoByteRead(ADXL345_DATAY0_REG, buffer); 00328 00329 return ((int)buffer[1] << 8 | (int)buffer[0]); 00330 } 00331 00332 int ADXL345_I2C::getAz(){ 00333 00334 char buffer[2]; 00335 00336 TwoByteRead(ADXL345_DATAZ0_REG, buffer); 00337 00338 return ((int)buffer[1] << 8 | (int)buffer[0]); 00339 } 00340 00341 00342 00343 void ADXL345_I2C::getOutput(int* readings){ 00344 00345 char buffer[2]; 00346 00347 TwoByteRead(ADXL345_DATAX0_REG, buffer); 00348 readings[0] = (int)buffer[1] << 8 | (int)buffer[0]; 00349 TwoByteRead(ADXL345_DATAY0_REG, buffer); 00350 readings[1] = (int)buffer[1] << 8 | (int)buffer[0]; 00351 TwoByteRead(ADXL345_DATAZ0_REG, buffer); 00352 readings[2] = (int)buffer[1] << 8 | (int)buffer[0]; 00353 00354 } 00355 00356 int ADXL345_I2C::getFifoControl(void){ 00357 00358 return oneByteRead(ADXL345_FIFO_CTL); 00359 00360 } 00361 00362 void ADXL345_I2C::setFifoControl(int settings){ 00363 00364 oneByteWrite(ADXL345_FIFO_STATUS, settings); 00365 00366 } 00367 00368 int ADXL345_I2C::getFifoStatus(void){ 00369 00370 return oneByteRead(ADXL345_FIFO_STATUS); 00371 00372 } 00373 00374 char ADXL345_I2C::oneByteRead(char address) { 00375 00376 00377 char rx[1]; 00378 char tx[1]; 00379 00380 // nCS_ = 1; 00381 tx[0]=address; 00382 00383 00384 i2c_.write(ADXL345_I2C_WRITE, tx,1); 00385 i2c_.read(ADXL345_I2C_READ,rx,1); 00386 00387 //nCS_ = 0; 00388 return rx[0]; 00389 00390 } 00391 00392 void ADXL345_I2C::oneByteWrite(char address, char data) { 00393 // nCS_ = 1; 00394 char tx[2]; 00395 00396 tx[0]=address; 00397 tx[1]=data; 00398 00399 i2c_.write(ADXL345_I2C_WRITE,tx,2); 00400 00401 //nCS_ = 0; 00402 } 00403 00404 void ADXL345_I2C::TwoByteRead(char startAddress, char* buffer) { 00405 00406 00407 // nCS_ = 1; 00408 //Send address to start reading from. 00409 char tx[1]; 00410 tx[0]=startAddress; 00411 i2c_.write(ADXL345_I2C_WRITE,tx,1); 00412 i2c_.read(ADXL345_I2C_READ,buffer,2); 00413 00414 //nCS_ = 0; 00415 } 00416 00417 void ADXL345_I2C::TwoByteWrite(char startAddress, char* buffer) { 00418 00419 //nCS_ = 1; 00420 //Send address to start reading from. 00421 char tx[1]; 00422 tx[0]=startAddress; 00423 i2c_.write(ADXL345_I2C_WRITE,tx,1); 00424 i2c_.write(ADXL345_I2C_WRITE,buffer,2); 00425 00426 00427 //nCS_ = 0; 00428 00429 }
Generated on Wed Jul 13 2022 16:44:29 by
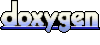