Add functions to read Ax, Ay and Az individually
Embed:
(wiki syntax)
Show/hide line numbers
ADXL345.cpp
00001 /** 00002 * @author Aaron Berk 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * ADXL345, triple axis, digital interface, accelerometer. 00029 * 00030 * Datasheet: 00031 * 00032 * http://www.analog.com/static/imported-files/data_sheets/ADXL345.pdf 00033 */ 00034 00035 /** 00036 * Includes 00037 */ 00038 #include "ADXL345.h" 00039 00040 ADXL345::ADXL345(PinName mosi, 00041 PinName miso, 00042 PinName sck, 00043 PinName cs) : spi_(mosi, miso, sck), nCS_(cs) { 00044 00045 //2MHz, allowing us to use the fastest data rates. 00046 spi_.frequency(2000000); 00047 spi_.format(8,3); 00048 00049 nCS_ = 1; 00050 00051 wait_us(500); 00052 00053 } 00054 00055 int ADXL345::getDevId(void) { 00056 00057 return oneByteRead(ADXL345_DEVID_REG); 00058 00059 } 00060 00061 int ADXL345::getTapThreshold(void) { 00062 00063 return oneByteRead(ADXL345_THRESH_TAP_REG); 00064 00065 } 00066 00067 void ADXL345::setTapThreshold(int threshold) { 00068 00069 oneByteWrite(ADXL345_THRESH_TAP_REG, threshold); 00070 00071 } 00072 00073 int ADXL345::getOffset(int axis) { 00074 00075 int address = 0; 00076 00077 if (axis == ADXL345_X) { 00078 address = ADXL345_OFSX_REG; 00079 } else if (axis == ADXL345_Y) { 00080 address = ADXL345_OFSY_REG; 00081 } else if (axis == ADXL345_Z) { 00082 address = ADXL345_OFSZ_REG; 00083 } 00084 00085 return oneByteRead(address); 00086 00087 } 00088 00089 void ADXL345::setOffset(int axis, char offset) { 00090 00091 int address = 0; 00092 00093 if (axis == ADXL345_X) { 00094 address = ADXL345_OFSX_REG; 00095 } else if (axis == ADXL345_Y) { 00096 address = ADXL345_OFSY_REG; 00097 } else if (axis == ADXL345_Z) { 00098 address = ADXL345_OFSZ_REG; 00099 } 00100 00101 return oneByteWrite(address, offset); 00102 00103 } 00104 00105 int ADXL345::getTapDuration(void) { 00106 00107 return oneByteRead(ADXL345_DUR_REG)*625; 00108 00109 } 00110 00111 void ADXL345::setTapDuration(int duration_us) { 00112 00113 int tapDuration = duration_us / 625; 00114 00115 oneByteWrite(ADXL345_DUR_REG, tapDuration); 00116 00117 } 00118 00119 float ADXL345::getTapLatency(void) { 00120 00121 return oneByteRead(ADXL345_LATENT_REG)*1.25; 00122 00123 } 00124 00125 void ADXL345::setTapLatency(int latency_ms) { 00126 00127 int tapLatency = latency_ms / 1.25; 00128 00129 oneByteWrite(ADXL345_LATENT_REG, tapLatency); 00130 00131 } 00132 00133 float ADXL345::getWindowTime(void) { 00134 00135 return oneByteRead(ADXL345_WINDOW_REG)*1.25; 00136 00137 } 00138 00139 void ADXL345::setWindowTime(int window_ms) { 00140 00141 int windowTime = window_ms / 1.25; 00142 00143 oneByteWrite(ADXL345_WINDOW_REG, windowTime); 00144 00145 } 00146 00147 int ADXL345::getActivityThreshold(void) { 00148 00149 return oneByteRead(ADXL345_THRESH_ACT_REG); 00150 00151 } 00152 00153 void ADXL345::setActivityThreshold(int threshold) { 00154 00155 oneByteWrite(ADXL345_THRESH_ACT_REG, threshold); 00156 00157 } 00158 00159 int ADXL345::getInactivityThreshold(void) { 00160 00161 return oneByteRead(ADXL345_THRESH_INACT_REG); 00162 00163 } 00164 00165 void ADXL345::setInactivityThreshold(int threshold) { 00166 00167 return oneByteWrite(ADXL345_THRESH_INACT_REG, threshold); 00168 00169 } 00170 00171 int ADXL345::getTimeInactivity(void) { 00172 00173 return oneByteRead(ADXL345_TIME_INACT_REG); 00174 00175 } 00176 00177 void ADXL345::setTimeInactivity(int timeInactivity) { 00178 00179 oneByteWrite(ADXL345_TIME_INACT_REG, timeInactivity); 00180 00181 } 00182 00183 int ADXL345::getActivityInactivityControl(void) { 00184 00185 return oneByteRead(ADXL345_ACT_INACT_CTL_REG); 00186 00187 } 00188 00189 void ADXL345::setActivityInactivityControl(int settings) { 00190 00191 oneByteWrite(ADXL345_ACT_INACT_CTL_REG, settings); 00192 00193 } 00194 00195 int ADXL345::getFreefallThreshold(void) { 00196 00197 return oneByteRead(ADXL345_THRESH_FF_REG); 00198 00199 } 00200 00201 void ADXL345::setFreefallThreshold(int threshold) { 00202 00203 oneByteWrite(ADXL345_THRESH_FF_REG, threshold); 00204 00205 } 00206 00207 int ADXL345::getFreefallTime(void) { 00208 00209 return oneByteRead(ADXL345_TIME_FF_REG)*5; 00210 00211 } 00212 00213 void ADXL345::setFreefallTime(int freefallTime_ms) { 00214 00215 int freefallTime = freefallTime_ms / 5; 00216 00217 oneByteWrite(ADXL345_TIME_FF_REG, freefallTime); 00218 00219 } 00220 00221 int ADXL345::getTapAxisControl(void) { 00222 00223 return oneByteRead(ADXL345_TAP_AXES_REG); 00224 00225 } 00226 00227 void ADXL345::setTapAxisControl(int settings) { 00228 00229 oneByteWrite(ADXL345_TAP_AXES_REG, settings); 00230 00231 } 00232 00233 int ADXL345::getTapSource(void) { 00234 00235 return oneByteRead(ADXL345_ACT_TAP_STATUS_REG); 00236 00237 } 00238 00239 void ADXL345::setPowerMode(char mode) { 00240 00241 //Get the current register contents, so we don't clobber the rate value. 00242 char registerContents = oneByteRead(ADXL345_BW_RATE_REG); 00243 00244 registerContents = (mode << 4) | registerContents; 00245 00246 oneByteWrite(ADXL345_BW_RATE_REG, registerContents); 00247 00248 } 00249 00250 int ADXL345::getPowerControl(void) { 00251 00252 return oneByteRead(ADXL345_POWER_CTL_REG); 00253 00254 } 00255 00256 void ADXL345::setPowerControl(int settings) { 00257 00258 oneByteWrite(ADXL345_POWER_CTL_REG, settings); 00259 00260 } 00261 00262 int ADXL345::getInterruptEnableControl(void) { 00263 00264 return oneByteRead(ADXL345_INT_ENABLE_REG); 00265 00266 } 00267 00268 void ADXL345::setInterruptEnableControl(int settings) { 00269 00270 oneByteWrite(ADXL345_INT_ENABLE_REG, settings); 00271 00272 } 00273 00274 int ADXL345::getInterruptMappingControl(void) { 00275 00276 return oneByteRead(ADXL345_INT_MAP_REG); 00277 00278 } 00279 00280 void ADXL345::setInterruptMappingControl(int settings) { 00281 00282 oneByteWrite(ADXL345_INT_MAP_REG, settings); 00283 00284 } 00285 00286 int ADXL345::getInterruptSource(void){ 00287 00288 return oneByteRead(ADXL345_INT_SOURCE_REG); 00289 00290 } 00291 00292 int ADXL345::getDataFormatControl(void){ 00293 00294 return oneByteRead(ADXL345_DATA_FORMAT_REG); 00295 00296 } 00297 00298 void ADXL345::setDataFormatControl(int settings){ 00299 00300 oneByteWrite(ADXL345_DATA_FORMAT_REG, settings); 00301 00302 } 00303 00304 void ADXL345::setDataRate(int rate) { 00305 00306 //Get the current register contents, so we don't clobber the power bit. 00307 char registerContents = oneByteRead(ADXL345_BW_RATE_REG); 00308 00309 registerContents &= 0x10; 00310 registerContents |= rate; 00311 00312 oneByteWrite(ADXL345_BW_RATE_REG, registerContents); 00313 00314 } 00315 00316 int ADXL345::getAx(){ 00317 00318 char buffer[2]; 00319 00320 multiByteRead(ADXL345_DATAX0_REG, buffer, 2); 00321 00322 return ((int)buffer[1] << 8 | (int)buffer[0]); 00323 } 00324 00325 00326 int ADXL345::getAy(){ 00327 00328 char buffer[2]; 00329 00330 multiByteRead(ADXL345_DATAY0_REG, buffer, 2); 00331 00332 return ((int)buffer[1] << 8 | (int)buffer[0]); 00333 } 00334 00335 int ADXL345::getAz(){ 00336 00337 char buffer[2]; 00338 00339 multiByteRead(ADXL345_DATAZ0_REG, buffer, 2); 00340 00341 return ((int)buffer[1] << 8 | (int)buffer[0]); 00342 } 00343 00344 00345 00346 void ADXL345::getOutput(int* readings){ 00347 00348 char buffer[6]; 00349 00350 multiByteRead(ADXL345_DATAX0_REG, buffer, 6); 00351 00352 readings[0] = (int)buffer[1] << 8 | (int)buffer[0]; 00353 readings[1] = (int)buffer[3] << 8 | (int)buffer[2]; 00354 readings[2] = (int)buffer[5] << 8 | (int)buffer[4]; 00355 00356 } 00357 00358 int ADXL345::getFifoControl(void){ 00359 00360 return oneByteRead(ADXL345_FIFO_CTL); 00361 00362 } 00363 00364 void ADXL345::setFifoControl(int settings){ 00365 00366 oneByteWrite(ADXL345_FIFO_STATUS, settings); 00367 00368 } 00369 00370 int ADXL345::getFifoStatus(void){ 00371 00372 return oneByteRead(ADXL345_FIFO_STATUS); 00373 00374 } 00375 00376 int ADXL345::oneByteRead(int address) { 00377 00378 int tx = (ADXL345_SPI_READ | (address & 0x3F)); 00379 int rx = 0; 00380 00381 nCS_ = 0; 00382 //Send address to read from. 00383 spi_.write(tx); 00384 //Read back contents of address. 00385 rx = spi_.write(0x00); 00386 nCS_ = 1; 00387 00388 return rx; 00389 00390 } 00391 00392 void ADXL345::oneByteWrite(int address, char data) { 00393 00394 int tx = (ADXL345_SPI_WRITE | (address & 0x3F)); 00395 00396 nCS_ = 0; 00397 //Send address to write to. 00398 spi_.write(tx); 00399 //Send data to be written. 00400 spi_.write(data); 00401 nCS_ = 1; 00402 00403 } 00404 00405 void ADXL345::multiByteRead(int startAddress, char* buffer, int size) { 00406 00407 int tx = (ADXL345_SPI_READ | ADXL345_MULTI_BYTE | (startAddress & 0x3F)); 00408 00409 nCS_ = 0; 00410 //Send address to start reading from. 00411 spi_.write(tx); 00412 00413 for (int i = 0; i < size; i++) { 00414 buffer[i] = spi_.write(0x00); 00415 } 00416 00417 nCS_ = 1; 00418 00419 } 00420 00421 void ADXL345::multiByteWrite(int startAddress, char* buffer, int size) { 00422 00423 int tx = (ADXL345_SPI_WRITE | ADXL345_MULTI_BYTE | (startAddress & 0x3F)); 00424 00425 nCS_ = 0; 00426 //Send address to start reading from. 00427 spi_.write(tx); 00428 00429 for (int i = 0; i < size; i++) { 00430 buffer[i] = spi_.write(0x00); 00431 } 00432 00433 nCS_ = 1; 00434 00435 }
Generated on Thu Jul 14 2022 20:07:13 by
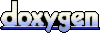