Device Driver for RAMTRON FM25W256 SPI-driven FRAM Uses SPI peripheral at 10MHz Based on fast SPI routines
fm25w256.cpp
00001 #include "fm25w256.h" 00002 #include "mbed.h" 00003 00004 /* =================================================================== 00005 routine: FM25W_SSP1_Init 00006 purpose: Configures the LPC1768's SSP1 peripheral for 00007 communication with Ramtron FM25W256 FRAM memory 00008 parameters: none 00009 returns: nothing 00010 date: 2012-04-05 00011 author: Stefan Guenther 00012 00013 * hardware connection scheme: * 00014 00015 mbed FRAM 00016 (LPC1768) 00017 --------+ +--------- 00018 | +Vcc _____ | 00019 | o +-----| 4k7 |---------+ !WP 00020 | | | | 00021 VCC +------+------+ | 00022 | | _____ | 00023 | +-----| 4k7 |-------- + !HOLD 00024 | <CLK> | 00025 P0.7 +--->--------------------------->---+ SCK 00026 | <MISO> | 00027 P0.8 +---<---------------------------<---+ SO 00028 | <MOSI> | 00029 P0.9 +--->--------------------------->---+ SI 00030 | <!SSL> | 00031 P0.6 +--->--------------------------->---+ !CS 00032 | | 00033 GND +------------------+----------------+ GND 00034 | | | 00035 --------+ o +--------- 00036 0V 00037 Keep traces as short as possible - SPI frequency is 10MHz! 00038 -------------------------------------------------------------------*/ 00039 void FM25W_SSP1_Init(void) 00040 { 00041 LPC_SC->PCLKSEL0|=(1<<20); //SSP clock = 1x System Clock 00042 LPC_SC->PCONP |= BIT10; //enable power to SSP1 peripheral 00043 //P0.6 -> SSEL 00044 //P0.7 -> SCK 00045 //P0.8 -> MISO 00046 //P0.9 -> MOSI 00047 LPC_PINCON->PINSEL0 &=~0xFC000; //reset GPIO functions for needed portpins 00048 LPC_PINCON->PINSEL0 |= 0xA8000; //set GPIO functions to SSP1 00049 LPC_SSP1->CPSR=11; //SSP clock divider (results in 10MHz SPI Clk) 00050 LPC_SSP1->CR0=7;//|0x80|0x40; //FRF=SPI, DSS=8, SPO=1, SPH=1 00051 LPC_SSP1->CR1 &=~BIT2; //SSP1 is master 00052 LPC_SSP1->CR1 |= BIT1; //enable SSP1 peripheral (SSE bit) 00053 LPC_PINCON->PINSEL0 &=~0x3000; //p8 [P0.6] is Slave Select (software driven) 00054 LPC_GPIO0->FIODIR |= BIT6; //P0.6 is output 00055 SSL1; 00056 } 00057 00058 00059 /* =================================================================== 00060 routine: FM25W_ReadByte 00061 purpose: Reads a single byte from specified memory address 00062 parameters: <iAddress> Memory address to read 00063 returns: Memory content 00064 date: 2012-04-05 00065 author: Stefan Guenther 00066 -------------------------------------------------------------------*/ 00067 unsigned char FM25W_ReadByte(unsigned int iAddress) 00068 { 00069 unsigned char retVal=0; 00070 while(!(LPC_SSP1->SR&BIT0)); //wait for TX FIFO to get empty 00071 SSL0; 00072 LPC_SSP1->DR = READ; 00073 LPC_SSP1->DR = (iAddress >> 8) & 0xFF; //Send top address byte to read from 00074 LPC_SSP1->DR = iAddress & 0xFF; //Send Middle address byte to read from 00075 LPC_SSP1->DR = 0; 00076 while(LPC_SSP1->SR&BIT4); //wait for TX to end 00077 while(LPC_SSP1->SR&BIT2) //read out RX FIFO 00078 retVal = LPC_SSP1->DR; //last FIFO entry is our data byte 00079 SSL1; 00080 return(retVal); 00081 } 00082 00083 /* =================================================================== 00084 routine: FM25W_WriteByte 00085 purpose: Writes a single byte to specified memory address 00086 parameters: <iAddress> Memory address to write to 00087 <cByte> Data to be written 00088 returns: nothing 00089 date: 2012-04-05 00090 author: Stefan Guenther 00091 -------------------------------------------------------------------*/ 00092 void FM25W_WriteByte(unsigned int iAddress, unsigned char cByte) 00093 { 00094 while(!(LPC_SSP1->SR&BIT0)); //wait for TX FIFO to get empty 00095 SSL0; 00096 LPC_SSP1->DR = WREN; //WREN has to be written prior to 00097 //each write access to FRAM 00098 while(LPC_SSP1->SR&BIT4); //wait for TX to end 00099 SSL1; 00100 while(LPC_SSP1->SR&BIT2) //clear RX FIFO 00101 if(LPC_SSP1->DR); 00102 SSL0; 00103 LPC_SSP1->DR = WRITE; 00104 LPC_SSP1->DR = (iAddress >> 8) & 0xFF; //Send top address byte to read from 00105 LPC_SSP1->DR = iAddress & 0xFF; //Send low address byte to read from 00106 LPC_SSP1->DR = cByte; 00107 while(LPC_SSP1->SR&BIT4); //wait for TX to end 00108 while(LPC_SSP1->SR&BIT2) //clear RX FIFO 00109 if(LPC_SSP1->DR); 00110 SSL1; 00111 } 00112 00113 /* =================================================================== 00114 routine: FM25W_WriteBlock 00115 purpose: Writes a block of data, starting at specified 00116 memory address 00117 parameters: <iAddress> First memory address to be written 00118 <*ptrBlock> Pointer to data block to be written 00119 <iCount> Length (in bytes) of data block 00120 returns: nothing 00121 date: 2012-04-05 00122 author: Stefan Guenther 00123 -------------------------------------------------------------------*/ 00124 void FM25W_WriteBlock(unsigned int iAddress, unsigned char *ptrBlock, unsigned int iCount) 00125 { 00126 int x; 00127 while(!(LPC_SSP1->SR&BIT0)); //wait for TX FIFO to get empty 00128 SSL0; 00129 LPC_SSP1->DR = WREN; //unlock FRAM for writing 00130 while(LPC_SSP1->SR&BIT4); //wait for TX to end 00131 SSL1; 00132 while(LPC_SSP1->SR&BIT2) //clear RX FIFO 00133 if(LPC_SSP1->DR); 00134 SSL0; 00135 LPC_SSP1->DR = WRITE; //send write command 00136 LPC_SSP1->DR = (iAddress >> 8) & 0xFF; //set start adress of block 00137 LPC_SSP1->DR = iAddress & 0xFF; 00138 for(x=0; x<iCount; x++) 00139 { 00140 while(!(LPC_SSP1->SR&BIT1)); //wait as TX FIFO is full 00141 00142 LPC_SSP1->DR = *ptrBlock; 00143 ptrBlock++; 00144 } 00145 while(LPC_SSP1->SR&BIT4); //wait for TX to end 00146 SSL1; 00147 while(LPC_SSP1->SR&BIT2) //clear RX FIFO 00148 if(LPC_SSP1->DR); 00149 } 00150 00151 /* =================================================================== 00152 routine: FM25W_ReadBlock 00153 purpose: Reads a block of data, starting at specified 00154 memory address 00155 parameters: <iAddress> First memory address to be read 00156 <*ptrBlock> Pointer to local RAM for data block 00157 <iCount> Length (in bytes) of data block to read 00158 returns: nothing 00159 date: 2012-04-05 00160 author: Stefan Guenther 00161 -------------------------------------------------------------------*/ 00162 void FM25W_ReadBlock(unsigned int iAddress, unsigned char *ptrBlock, unsigned int iCount) 00163 { 00164 int x; 00165 00166 while(LPC_SSP1->SR&BIT2) //clear RX FIFO 00167 if(LPC_SSP1->DR); 00168 while(!(LPC_SSP1->SR&BIT0)); //wait for TX FIFO to get empty 00169 00170 SSL0; 00171 00172 LPC_SSP1->DR = READ; 00173 LPC_SSP1->DR = (iAddress >> 8) & 0xFF; //send upper address byte to read from 00174 LPC_SSP1->DR = iAddress & 0xFF; //send lower address byte to read from 00175 while(LPC_SSP1->SR&BIT4); //wait for TX FIFO to get empty 00176 x = LPC_SSP1->DR; //clear RX FIFO 00177 x = LPC_SSP1->DR; 00178 x = LPC_SSP1->DR; 00179 00180 for(x=0; x<iCount; x++) 00181 { 00182 LPC_SSP1->DR = 0; //send first dummy byte 00183 while(LPC_SSP1->SR&BIT4); //wait for TX FIFO to get empty 00184 *ptrBlock = LPC_SSP1->DR; //last FIFO entry is our data byte 00185 ptrBlock++; 00186 } 00187 SSL1; 00188 while(LPC_SSP1->SR&BIT2) //clear RX FIFO 00189 if(LPC_SSP1->DR); 00190 } 00191
Generated on Wed Jul 20 2022 01:53:46 by
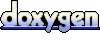