fork
Fork of nRF51822 by
Embed:
(wiki syntax)
Show/hide line numbers
nRF51Gap.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __NRF51822_GAP_H__ 00018 #define __NRF51822_GAP_H__ 00019 00020 #include "mbed.h" 00021 #include "ble/blecommon.h" 00022 #include "ble.h" 00023 #include "ble/GapAdvertisingParams.h" 00024 #include "ble/GapAdvertisingData.h" 00025 #include "ble/Gap.h" 00026 #include "ble/GapScanningParams.h" 00027 00028 #include "nrf_soc.h" 00029 #include "ble_radio_notification.h " 00030 #include "btle_security.h" 00031 00032 /**************************************************************************/ 00033 /*! 00034 \brief 00035 00036 */ 00037 /**************************************************************************/ 00038 class nRF51Gap : public Gap 00039 { 00040 public: 00041 static nRF51Gap &getInstance(); 00042 00043 /* Functions that must be implemented from Gap */ 00044 virtual ble_error_t setAddress(AddressType_t type, const Address_t address); 00045 virtual ble_error_t getAddress(AddressType_t *typeP, Address_t address); 00046 virtual ble_error_t setAdvertisingData(const GapAdvertisingData &, const GapAdvertisingData &); 00047 00048 virtual uint16_t getMinAdvertisingInterval(void) const {return ADVERTISEMENT_DURATION_UNITS_TO_MS(BLE_GAP_ADV_INTERVAL_MIN);} 00049 virtual uint16_t getMinNonConnectableAdvertisingInterval(void) const {return ADVERTISEMENT_DURATION_UNITS_TO_MS(BLE_GAP_ADV_NONCON_INTERVAL_MIN);} 00050 virtual uint16_t getMaxAdvertisingInterval(void) const {return ADVERTISEMENT_DURATION_UNITS_TO_MS(BLE_GAP_ADV_INTERVAL_MAX);} 00051 00052 virtual ble_error_t startAdvertising(const GapAdvertisingParams &); 00053 virtual ble_error_t stopAdvertising(void); 00054 virtual ble_error_t connect(const Address_t, Gap::AddressType_t peerAddrType, const ConnectionParams_t *connectionParams, const GapScanningParams *scanParams); 00055 virtual ble_error_t disconnect(Handle_t connectionHandle, DisconnectionReason_t reason); 00056 virtual ble_error_t disconnect(DisconnectionReason_t reason); 00057 00058 virtual ble_error_t setDeviceName(const uint8_t *deviceName); 00059 virtual ble_error_t getDeviceName(uint8_t *deviceName, unsigned *lengthP); 00060 virtual ble_error_t setAppearance(GapAdvertisingData::Appearance appearance); 00061 virtual ble_error_t getAppearance(GapAdvertisingData::Appearance *appearanceP); 00062 00063 virtual ble_error_t setTxPower(int8_t txPower); 00064 virtual void getPermittedTxPowerValues(const int8_t **valueArrayPP, size_t *countP); 00065 00066 void setConnectionHandle(uint16_t con_handle); 00067 uint16_t getConnectionHandle(void); 00068 00069 virtual ble_error_t getPreferredConnectionParams(ConnectionParams_t *params); 00070 virtual ble_error_t setPreferredConnectionParams(const ConnectionParams_t *params); 00071 virtual ble_error_t updateConnectionParams(Handle_t handle, const ConnectionParams_t *params); 00072 00073 virtual void onRadioNotification(RadioNotificationEventCallback_t callback) { 00074 Gap::onRadioNotification(callback); 00075 ble_radio_notification_init(NRF_APP_PRIORITY_HIGH, NRF_RADIO_NOTIFICATION_DISTANCE_800US, radioNotificationCallback); 00076 } 00077 00078 virtual ble_error_t startRadioScan(const GapScanningParams &scanningParams) { 00079 ble_gap_scan_params_t scanParams = { 00080 .active = scanningParams.getActiveScanning(), /**< If 1, perform active scanning (scan requests). */ 00081 .selective = 0, /**< If 1, ignore unknown devices (non whitelisted). */ 00082 .p_whitelist = NULL, /**< Pointer to whitelist, NULL if none is given. */ 00083 .interval = scanningParams.getInterval(), /**< Scan interval between 0x0004 and 0x4000 in 0.625ms units (2.5ms to 10.24s). */ 00084 .window = scanningParams.getWindow(), /**< Scan window between 0x0004 and 0x4000 in 0.625ms units (2.5ms to 10.24s). */ 00085 .timeout = scanningParams.getTimeout(), /**< Scan timeout between 0x0001 and 0xFFFF in seconds, 0x0000 disables timeout. */ 00086 }; 00087 00088 if (sd_ble_gap_scan_start(&scanParams) != NRF_SUCCESS) { 00089 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00090 } 00091 00092 return BLE_ERROR_NONE; 00093 } 00094 00095 virtual ble_error_t stopScan(void) { 00096 if (sd_ble_gap_scan_stop() == NRF_SUCCESS) { 00097 return BLE_ERROR_NONE; 00098 } 00099 00100 return BLE_STACK_BUSY; 00101 } 00102 00103 private: 00104 uint16_t m_connectionHandle; 00105 nRF51Gap() { 00106 m_connectionHandle = BLE_CONN_HANDLE_INVALID; 00107 } 00108 00109 nRF51Gap(nRF51Gap const &); 00110 void operator=(nRF51Gap const &); 00111 }; 00112 00113 #endif // ifndef __NRF51822_GAP_H__
Generated on Tue Jul 12 2022 18:08:54 by
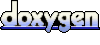