
CooCox 1.1.4 on mbed with simple blinky example
Embed:
(wiki syntax)
Show/hide line numbers
time.c
Go to the documentation of this file.
00001 /** 00002 ******************************************************************************* 00003 * @file time.c 00004 * @version V1.1.4 00005 * @date 2011.04.20 00006 * @brief time management implementation code of CooCox CoOS kernel. 00007 ******************************************************************************* 00008 * @copy 00009 * 00010 * INTERNAL FILE,DON'T PUBLIC. 00011 * 00012 * <h2><center>© COPYRIGHT 2009 CooCox </center></h2> 00013 ******************************************************************************* 00014 */ 00015 00016 00017 00018 /*---------------------------- Include ---------------------------------------*/ 00019 #include <coocox.h> 00020 00021 #if CFG_TASK_WAITTING_EN > 0 00022 00023 /*---------------------------- Variable Define -------------------------------*/ 00024 P_OSTCB DlyList = 0; /*!< Header pointer to the DELAY list.*/ 00025 00026 00027 /** 00028 ******************************************************************************* 00029 * @brief Insert into DELAY list 00030 * 00031 * @param[in] ptcb Task that want to insert into DELAY list. 00032 * @param[in] ticks Delay system ticks. 00033 * @param[out] None 00034 * @retval None. 00035 * 00036 * @par Description 00037 * @details This function is called to insert task into DELAY list. 00038 ******************************************************************************* 00039 */ 00040 void InsertDelayList(P_OSTCB ptcb,U32 ticks) 00041 { 00042 S32 deltaTicks; 00043 P_OSTCB dlyNext; 00044 00045 if(ticks == 0) /* Is delay tick == 0? */ 00046 return; /* Yes,do nothing,return */ 00047 if(DlyList == 0) /* Is no item in DELAY list? */ 00048 { 00049 ptcb->delayTick = ticks; /* Yes,set this as first item */ 00050 DlyList = ptcb; 00051 } 00052 else 00053 { 00054 /* No,find correct place ,and insert the task */ 00055 dlyNext = DlyList ; 00056 deltaTicks = ticks; /* Get task delay ticks */ 00057 00058 /* Find correct place */ 00059 while(dlyNext != 0) 00060 { 00061 /* Get delta ticks with previous item */ 00062 deltaTicks -= dlyNext->delayTick ; 00063 if(deltaTicks < 0) /* Is delta ticks<0? */ 00064 { 00065 /* Yes,get correct place */ 00066 if(dlyNext->TCBprev != 0) /* Is head item of DELAY list? */ 00067 { 00068 dlyNext->TCBprev ->TCBnext = ptcb; /* No,insert into */ 00069 ptcb->TCBprev = dlyNext->TCBprev ; 00070 ptcb->TCBnext = dlyNext; 00071 dlyNext->TCBprev = ptcb; 00072 } 00073 else /* Yes,set task as first item */ 00074 { 00075 ptcb->TCBnext = DlyList ; 00076 DlyList->TCBprev = ptcb; 00077 DlyList = ptcb; 00078 } 00079 ptcb->delayTick = ptcb->TCBnext ->delayTick +deltaTicks; 00080 ptcb->TCBnext ->delayTick -= ptcb->delayTick ; 00081 break; 00082 } 00083 /* Is last item in DELAY list? */ 00084 else if((deltaTicks >= 0) && (dlyNext->TCBnext == 0) ) 00085 { 00086 ptcb->TCBprev = dlyNext; /* Yes,insert into */ 00087 dlyNext->TCBnext = ptcb; 00088 ptcb->delayTick = deltaTicks; 00089 break; 00090 } 00091 dlyNext = dlyNext->TCBnext ; /* Get the next item in DELAY list */ 00092 } 00093 } 00094 00095 ptcb->state = TASK_WAITING; /* Set task status as TASK_WAITING */ 00096 TaskSchedReq = Co_TRUE; 00097 } 00098 00099 00100 /** 00101 ******************************************************************************* 00102 * @brief Remove from the DELAY list 00103 * @param[in] ptcb Task that want to remove from the DELAY list. 00104 * @param[out] None 00105 * @retval None 00106 * 00107 * @par Description 00108 * @details This function is called to remove task from the DELAY list. 00109 ******************************************************************************* 00110 */ 00111 void RemoveDelayList(P_OSTCB ptcb) 00112 { 00113 00114 /* Is there only one item in the DELAY list? */ 00115 if((ptcb->TCBprev == 0) && ( ptcb->TCBnext == 0)) 00116 { 00117 DlyList = 0; /* Yes,set DELAY list as Co_NULL */ 00118 } 00119 else if(ptcb->TCBprev == 0) /* Is the first item in DELAY list? */ 00120 { 00121 /* Yes,remove task from the DELAY list,and reset the list */ 00122 DlyList = ptcb->TCBnext ; 00123 ptcb->TCBnext ->delayTick += ptcb->delayTick ; 00124 ptcb->TCBnext ->TCBprev = 0; 00125 ptcb->TCBnext = 0; 00126 00127 } 00128 else if(ptcb->TCBnext == 0) /* Is the last item in DELAY list? */ 00129 { 00130 ptcb->TCBprev ->TCBnext = 0; /* Yes,remove task form DELAY list */ 00131 ptcb->TCBprev = 0; 00132 } 00133 else /* No, remove task from DELAY list */ 00134 { 00135 ptcb->TCBprev ->TCBnext = ptcb->TCBnext ; 00136 ptcb->TCBnext ->TCBprev = ptcb->TCBprev ; 00137 ptcb->TCBnext ->delayTick += ptcb->delayTick ; 00138 ptcb->TCBnext = 0; 00139 ptcb->TCBprev = 0; 00140 } 00141 ptcb->delayTick = INVALID_VALUE; /* Set task delay tick value as invalid */ 00142 } 00143 00144 /** 00145 ******************************************************************************* 00146 * @brief Get current ticks 00147 * @param[in] None 00148 * @param[out] None 00149 * @retval Return current system tick counter. 00150 * 00151 * @par Description 00152 * @details This function is called to obtain current system tick counter. 00153 ******************************************************************************* 00154 */ 00155 U64 CoGetOSTime(void) 00156 { 00157 return OSTickCnt ; /* Get system time(tick) */ 00158 } 00159 00160 00161 /** 00162 ******************************************************************************* 00163 * @brief Delay current task for specify ticks number 00164 * @param[in] ticks Specify system tick number which will delay. 00165 * @param[out] None 00166 * @retval E_CALL Error call in ISR. 00167 * @retval E_OK The current task was insert to DELAY list successful,it 00168 * will delay specify time. 00169 * @par Description 00170 * @details This function delay specify ticks for current task. 00171 * 00172 * @note This function be called in ISR,do nothing and return immediately. 00173 ******************************************************************************* 00174 */ 00175 StatusType CoTickDelay(U32 ticks) 00176 { 00177 if(OSIntNesting >0) /* Is call in ISR? */ 00178 { 00179 return E_CALL; /* Yes,error return */ 00180 } 00181 00182 if(ticks == INVALID_VALUE) /* Is tick==INVALID_VALUE? */ 00183 { 00184 return E_INVALID_PARAMETER; /* Yes,error return */ 00185 } 00186 if(ticks == 0) /* Is tick==0? */ 00187 { 00188 return E_OK; /* Yes,do nothing ,return OK */ 00189 } 00190 if(OSSchedLock != 0) /* Is OS lock? */ 00191 { 00192 return E_OS_IN_LOCK; /* Yes,error return */ 00193 } 00194 OsSchedLock(); /* Lock schedule */ 00195 InsertDelayList(TCBRunning ,ticks); /* Insert task in DELAY list */ 00196 OsSchedUnlock(); /* Unlock schedule,and call task schedule */ 00197 return E_OK; /* Return OK */ 00198 } 00199 00200 00201 /** 00202 ******************************************************************************* 00203 * @brief Reset task delay ticks 00204 * @param[in] ptcb Task that want to insert into DELAY list. 00205 * @param[in] ticks Specify system tick number which will delay . 00206 * @param[out] None 00207 * @retval E_CALL Error call in ISR. 00208 * @retval E_INVALID_ID Invalid task id. 00209 * @retval E_NOT_IN_DELAY_LIST Task not in delay list. 00210 * @retval E_OK The current task was inserted to DELAY list 00211 * successful,it will delay for specify time. 00212 * @par Description 00213 * @details This function delay specify ticks for current task. 00214 ******************************************************************************* 00215 */ 00216 StatusType CoResetTaskDelayTick(OS_TID taskID,U32 ticks) 00217 { 00218 P_OSTCB ptcb; 00219 00220 00221 #if CFG_PAR_CHECKOUT_EN >0 /* Check validity of parameter */ 00222 if(taskID >= CFG_MAX_USER_TASKS + SYS_TASK_NUM) 00223 { 00224 return E_INVALID_ID; 00225 } 00226 #endif 00227 00228 ptcb = &TCBTbl [taskID]; 00229 #if CFG_PAR_CHECKOUT_EN >0 00230 if(ptcb->stkPtr == Co_NULL) 00231 { 00232 return E_INVALID_ID; 00233 } 00234 #endif 00235 00236 if(ptcb->delayTick == INVALID_VALUE) /* Is tick==INVALID_VALUE? */ 00237 { 00238 return E_NOT_IN_DELAY_LIST; /* Yes,error return */ 00239 } 00240 OsSchedLock(); /* Lock schedule */ 00241 RemoveDelayList(ptcb); /* Remove task from the DELAY list */ 00242 00243 if(ticks == 0) /* Is delay tick==0? */ 00244 { 00245 InsertToTCBRdyList(ptcb); /* Insert task into the DELAY list */ 00246 } 00247 else 00248 { 00249 InsertDelayList(ptcb,ticks); /* No,insert task into DELAY list */ 00250 } 00251 OsSchedUnlock(); /* Unlock schedule,and call task schedule */ 00252 return E_OK; /* Return OK */ 00253 } 00254 00255 00256 /** 00257 ******************************************************************************* 00258 * @brief Delay current task for detail time 00259 * @param[in] hour Specify the number of hours. 00260 * @param[in] minute Specify the number of minutes. 00261 * @param[in] sec Specify the number of seconds. 00262 * @param[in] millsec Specify the number of millseconds. 00263 * @param[out] None 00264 * @retval E_CALL Error call in ISR. 00265 * @retval E_INVALID_PARAMETER Parameter passed was invalid,delay fail. 00266 * @retval E_OK The current task was inserted to DELAY list 00267 * successful,it will delay for specify time. 00268 * @par Description 00269 * @details This function delay specify time for current task. 00270 * 00271 * @note If this function called in ISR,do nothing and return immediately. 00272 ******************************************************************************* 00273 */ 00274 #if CFG_TIME_DELAY_EN >0 00275 StatusType CoTimeDelay(U8 hour,U8 minute,U8 sec,U16 millsec) 00276 { 00277 U32 ticks; 00278 #if CFG_PAR_CHECKOUT_EN >0 /* Check validity of parameter */ 00279 if(OSIntNesting > 0) 00280 { 00281 return E_CALL; 00282 } 00283 if((minute > 59)||(sec > 59)||(millsec > 999)) 00284 return E_INVALID_PARAMETER; 00285 #endif 00286 if(OSSchedLock != 0) /* Is OS lock? */ 00287 { 00288 return E_OS_IN_LOCK; /* Yes,error return */ 00289 } 00290 00291 /* Get tick counter from time */ 00292 ticks = ((hour*3600) + (minute*60) + (sec)) * (CFG_SYSTICK_FREQ)\ 00293 + (millsec*CFG_SYSTICK_FREQ + 500)/1000; 00294 00295 CoTickDelay(ticks); /* Call tick delay */ 00296 return E_OK; /* Return OK */ 00297 } 00298 #endif 00299 00300 00301 00302 00303 /** 00304 ******************************************************************************* 00305 * @brief Dispose time delay 00306 * @param[in] None 00307 * @param[out] None 00308 * @retval None 00309 * 00310 * @par Description 00311 * @details This function is called to dispose time delay of all task. 00312 ******************************************************************************* 00313 */ 00314 void TimeDispose(void) 00315 { 00316 P_OSTCB dlyList; 00317 00318 dlyList = DlyList ; /* Get first item of DELAY list */ 00319 while((dlyList != 0) && (dlyList->delayTick == 0) ) 00320 { 00321 00322 #if CFG_EVENT_EN > 0 00323 if(dlyList->eventID != INVALID_ID) /* Is task in event waiting list? */ 00324 { 00325 RemoveEventWaittingList(dlyList); /* Yes,remove task from list */ 00326 } 00327 #endif 00328 00329 #if CFG_FLAG_EN > 0 00330 if(dlyList->pnode != 0) /* Is task in flag waiting list? */ 00331 { 00332 RemoveLinkNode((P_FLAG_NODE)dlyList->pnode ); /* Yes,remove task from list */ 00333 } 00334 #endif 00335 dlyList->delayTick = INVALID_VALUE; /* Set delay tick value as invalid*/ 00336 DlyList = dlyList->TCBnext ; /* Get next item as the head of DELAY list*/ 00337 dlyList->TCBnext = 0; 00338 00339 InsertToTCBRdyList(dlyList); /* Insert task into READY list */ 00340 00341 dlyList = DlyList ; /* Get the first item of DELAY list */ 00342 if(dlyList != 0) /* Is DELAY list as Co_NULL? */ 00343 { 00344 dlyList->TCBprev = 0; /* No,initialize the first item */ 00345 } 00346 } 00347 } 00348 00349 00350 /** 00351 ******************************************************************************* 00352 * @brief Dispose time delay in ISR 00353 * @param[in] None 00354 * @param[out] None 00355 * @retval None 00356 * 00357 * @par Description 00358 * @details This function is called in systick interrupt to dispose time delay 00359 * of all task. 00360 ******************************************************************************* 00361 */ 00362 void isr_TimeDispose(void) 00363 { 00364 if(OSSchedLock > 1) /* Is schedule lock? */ 00365 { 00366 IsrReq = Co_TRUE; 00367 TimeReq = Co_TRUE; /* Yes,set time request Co_TRUE */ 00368 } 00369 else 00370 { 00371 TimeDispose(); /* No,call handler */ 00372 } 00373 } 00374 00375 00376 #endif
Generated on Tue Jul 12 2022 18:19:10 by
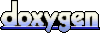