
CooCox 1.1.4 on mbed with simple blinky example
Embed:
(wiki syntax)
Show/hide line numbers
serviceReq.c
Go to the documentation of this file.
00001 /** 00002 ******************************************************************************* 00003 * @file serviceReq.c 00004 * @version V1.1.4 00005 * @date 2011.04.20 00006 * @brief servive request management implementation code of CooCox CoOS kernel. 00007 ******************************************************************************* 00008 * @copy 00009 * 00010 * INTERNAL FILE,DON'T PUBLIC. 00011 * 00012 * <h2><center>© COPYRIGHT 2009 CooCox </center></h2> 00013 ******************************************************************************* 00014 */ 00015 00016 00017 00018 /*---------------------------- Include ---------------------------------------*/ 00019 #include <coocox.h> 00020 00021 #if (CFG_TASK_WAITTING_EN > 0) || (CFG_TMR_EN >0) 00022 00023 #if CFG_MAX_SERVICE_REQUEST > 0 00024 /*---------------------------- Variable Define -------------------------------*/ 00025 SRQ ServiceReq = {0,0}; /*!< ISR server request queue */ 00026 #endif 00027 BOOL IsrReq = Co_FALSE; 00028 #if (CFG_TASK_WAITTING_EN > 0) 00029 BOOL TimeReq = Co_FALSE; /*!< Time delay dispose request */ 00030 #endif 00031 00032 #if CFG_TMR_EN > 0 00033 BOOL TimerReq = Co_FALSE; /*!< Timer dispose request */ 00034 #endif 00035 00036 /** 00037 ******************************************************************************* 00038 * @brief Insert into service requst queue 00039 * @param[in] type Service request type. 00040 * @param[in] id Service request event id,event id/flag id. 00041 * @param[in] arg Service request argument. 00042 * @param[out] None 00043 * 00044 * @retval Co_FALSE Successfully insert into service request queue. 00045 * @retval Co_TRUE Failure to insert into service request queue. 00046 * 00047 * @par Description 00048 * @details This function be called to insert a requst into service request 00049 * queue. 00050 * @note 00051 ******************************************************************************* 00052 */ 00053 #if (CFG_MAX_SERVICE_REQUEST > 0) 00054 BOOL InsertInSRQ(U8 type,U8 id,void* arg) 00055 { 00056 P_SQC pcell; 00057 U8 cnt; 00058 U8 heed; 00059 IRQ_DISABLE_SAVE(); 00060 if (ServiceReq .cnt >= CFG_MAX_SERVICE_REQUEST) 00061 { 00062 IRQ_ENABLE_RESTORE (); 00063 00064 return Co_FALSE; /* Error return */ 00065 } 00066 cnt = Inc8(&ServiceReq .cnt); 00067 heed = ServiceReq .head; 00068 IsrReq = Co_TRUE; 00069 pcell = &ServiceReq .cell[((cnt+heed)%CFG_MAX_SERVICE_REQUEST)];/*the tail */ 00070 pcell->type = type; /* Save service request type, */ 00071 pcell->id = id; /* event id */ 00072 pcell->arg = arg; /* and parameter */ 00073 IRQ_ENABLE_RESTORE (); 00074 00075 return Co_TRUE; /* Return OK */ 00076 } 00077 #endif 00078 00079 00080 00081 /** 00082 ******************************************************************************* 00083 * @brief Respond the request in the service request queue. 00084 * @param[in] None 00085 * @param[out] None 00086 * @retval None 00087 * 00088 * @par Description 00089 * @details This function be called to respond the request in the service 00090 * request queue. 00091 * @note 00092 ******************************************************************************* 00093 */ 00094 void RespondSRQ(void) 00095 { 00096 00097 #if CFG_MAX_SERVICE_REQUEST > 0 00098 SQC cell; 00099 00100 #endif 00101 00102 #if (CFG_TASK_WAITTING_EN > 0) 00103 if(TimeReq == Co_TRUE) /* Time delay request? */ 00104 { 00105 TimeDispose(); /* Yes,call handler */ 00106 TimeReq = Co_FALSE; /* Reset time delay request Co_FALSE */ 00107 } 00108 #endif 00109 #if CFG_TMR_EN > 0 00110 if(TimerReq == Co_TRUE) /* Timer request? */ 00111 { 00112 TmrDispose(); /* Yes,call handler */ 00113 TimerReq = Co_FALSE; /* Reset timer request Co_FALSE */ 00114 } 00115 #endif 00116 00117 #if CFG_MAX_SERVICE_REQUEST > 0 00118 00119 while (ServiceReq .cnt != 0) 00120 { 00121 IRQ_DISABLE_SAVE (); /* need to protect the following */ 00122 cell = ServiceReq .cell[ServiceReq .head]; /* extract one cell */ 00123 ServiceReq .head = (ServiceReq .head + 1) % /* move head (pop) */ 00124 CFG_MAX_SERVICE_REQUEST; 00125 ServiceReq .cnt--; 00126 IRQ_ENABLE_RESTORE (); /* now use the cell copy */ 00127 00128 switch(cell.type) /* Judge service request type */ 00129 { 00130 #if CFG_SEM_EN > 0 00131 case SEM_REQ: /* Semaphore post request,call handler*/ 00132 CoPostSem(cell.id); 00133 break; 00134 #endif 00135 #if CFG_MAILBOX_EN > 0 00136 case MBOX_REQ: /* Mailbox post request,call handler */ 00137 CoPostMail(cell.id, cell.arg); 00138 break; 00139 #endif 00140 #if CFG_FLAG_EN > 0 00141 case FLAG_REQ: /* Flag set request,call handler */ 00142 CoSetFlag(cell.id); 00143 break; 00144 #endif 00145 #if CFG_QUEUE_EN > 0 00146 case QUEUE_REQ: /* Queue post request,call handler */ 00147 CoPostQueueMail(cell.id, cell.arg); 00148 break; 00149 #endif 00150 default: /* Others,break */ 00151 break; 00152 } 00153 } 00154 #endif 00155 IRQ_DISABLE_SAVE (); /* need to protect the following */ 00156 00157 if (ServiceReq .cnt == 0) /* another item in the queue already? */ 00158 { 00159 IsrReq = Co_FALSE; /* queue still empty here */ 00160 } 00161 IRQ_ENABLE_RESTORE (); /* now it is done and return */ 00162 } 00163 00164 #endif 00165
Generated on Tue Jul 12 2022 18:19:10 by
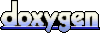