
CooCox 1.1.4 on mbed with simple blinky example
Embed:
(wiki syntax)
Show/hide line numbers
port.c
Go to the documentation of this file.
00001 /** 00002 ******************************************************************************* 00003 * @file port.c 00004 * @version V1.14 00005 * @date 2011.04.20 00006 * @brief Compiler adapter for CooCox CoOS kernel. 00007 ******************************************************************************* 00008 * @copy 00009 * 00010 * INTERNAL FILE,DON'T PUBLIC. 00011 * 00012 * <h2><center>© COPYRIGHT 2010 CooCox </center></h2> 00013 ******************************************************************************* 00014 */ 00015 00016 /*---------------------------- Include ---------------------------------------*/ 00017 #include <coocox.h> 00018 00019 00020 /** 00021 ****************************************************************************** 00022 * @brief Plus a byte integers and Saved into memory cell 00023 * @param[in] data byte integers. 00024 * @param[out] None 00025 * @retval Returns Original value. 00026 * 00027 * @par Description 00028 * @details This function is called to Plus a byte integers 00029 * and Saved into memory cell. 00030 ****************************************************************************** 00031 */ 00032 __asm U8 Inc8 (volatile U8 *data) 00033 { 00034 PUSH {R1} 00035 CPSID I 00036 LDRB R1,[R0] 00037 ADDS R1,#1 00038 STRB R1,[R0] 00039 CPSIE I 00040 SUBS R1,#1 00041 MOVS R0,R1 00042 POP {R1} 00043 BX LR 00044 ALIGN 00045 } 00046 00047 00048 /** 00049 ****************************************************************************** 00050 * @brief Decrease a byte integers and Saved into memory cell 00051 * @param[in] data byte integers. 00052 * @param[out] None 00053 * @retval Returns Original value. 00054 * 00055 * @par Description 00056 * @details This function is called to Decrease a byte integers 00057 * and Saved into memory cell. 00058 ****************************************************************************** 00059 */ 00060 __asm U8 Dec8 (volatile U8 *data) 00061 { 00062 PUSH {R1} 00063 CPSID I 00064 LDRB R1,[R0] 00065 SUBS R1,#1 00066 STRB R1,[R0] 00067 CPSIE I 00068 MOVS R0,R1 00069 POP {R1} 00070 BX LR 00071 ALIGN 00072 } 00073 00074 /** 00075 ****************************************************************************** 00076 * @brief ENABLE Interrupt 00077 * @param[in] None 00078 * @param[out] None 00079 * @retval None 00080 * 00081 * @par Description 00082 * @details This function is called to ENABLE Interrupt. 00083 ****************************************************************************** 00084 */ 00085 __asm void IRQ_ENABLE_RESTORE(void) 00086 { CPSIE I 00087 BX LR 00088 } 00089 00090 /** 00091 ****************************************************************************** 00092 * @brief Close Interrupt 00093 * @param[in] None 00094 * @param[out] None 00095 * @retval None 00096 * 00097 * @par Description 00098 * @details This function is called to close Interrupt. 00099 ****************************************************************************** 00100 */ 00101 __asm void IRQ_DISABLE_SAVE(void) 00102 { CPSID I 00103 BX LR 00104 } 00105 00106 /** 00107 ****************************************************************************** 00108 * @brief Set environment for Coocox OS running 00109 * @param[in] pstk stack pointer 00110 * @param[out] None 00111 * @retval None. 00112 * 00113 * @par Description 00114 * @details This function is called to Set environment 00115 * for Coocox OS running. 00116 ****************************************************************************** 00117 */ 00118 __asm void SetEnvironment(OS_STK *pstk) 00119 { 00120 SUBS R0,#28 00121 MSR PSP, R0 ; Mov new stack point to PSP 00122 BX LR 00123 ALIGN 00124 } 00125 00126 00127 /** 00128 ****************************************************************************** 00129 * @brief Do ready work to Switch Context for task change 00130 * @param[in] None 00131 * @param[out] None 00132 * @retval None. 00133 * 00134 * @par Description 00135 * @details This function is called to Do ready work to 00136 * Switch Context for task change 00137 ****************************************************************************** 00138 */ 00139 __asm void SwitchContext(void) 00140 { 00141 LDR R0, =0xE000ED04 ; Trigger the PendSV exception (causes context switch) 00142 LDR R1, =0x10000000 00143 STR R1, [R0] 00144 BX LR 00145 ALIGN 00146 } 00147 00148 00149 /** 00150 ****************************************************************************** 00151 * @brief Switch Context for task change 00152 * @param[in] None 00153 * @param[out] None 00154 * @retval None. 00155 * 00156 * @par Description 00157 * @details This function is called to Switch Context for task change. 00158 ****************************************************************************** 00159 */ 00160 #if CFG_CHIP_TYPE == 2 00161 extern "C" __asm void PendSV_Handler() 00162 { 00163 IMPORT TCBRunning 00164 IMPORT TCBNext 00165 IMPORT OSSchedLock 00166 LDR R3,=TCBRunning 00167 LDR R1,[R3] ; R1 == running tcb 00168 LDR R2,=TCBNext 00169 LDR R2,[R2] ; R2 == next tcb 00170 00171 CMP R1,R2 00172 BEQ exitPendSV 00173 MRS R0, PSP ; Get PSP point (can not use PUSH,in ISR,SP is MSP ) 00174 00175 SUBS R0,R0,#32 00176 STR R0,[R1] ; Save orig PSP 00177 ; Store r4-r11,r0 -= regCnt * 4,r0 is new stack 00178 ; top point (addr h->l r11,r10,...,r5,r4) 00179 STMIA R0!,{R4-R7} ; Save old context (R4-R7) 00180 MOV R4,R8 00181 MOV R5,R9 00182 MOV R6,R10 00183 MOV R7,R11 00184 STMIA R0!,{R4-R7} ; Save old context (R8-R11) 00185 00186 00187 popStk 00188 STR R2, [R3] ; TCBRunning = TCBNext ; 00189 LDR R0, [R2] ; Get SP of task that be switch into. 00190 00191 ADDS R0, R0,#16 00192 LDMIA R0!,{R4-R7} ; Restore new Context (R8-R11) 00193 MOV R8,R4 00194 MOV R9,R5 00195 MOV R10,R6 00196 MOV R11,R7 00197 SUBS R0,R0,#32 00198 LDMIA R0!,{R4-R7} ; Restore new Context (R4-R7) 00199 ADDS R0, R0,#16 00200 MSR PSP, R0 ; Mov new stack point to PSP 00201 00202 exitPendSV 00203 LDR R3,=OSSchedLock 00204 MOVS R0, #0x0 00205 STRB R0, [R3] 00206 MOVS R0,#4 00207 RSBS R0,#0 ; =0xFFFFFFFC,Ensure exception return uses process stack 00208 BX R0 ; Exit interrupt 00209 ALIGN 00210 } 00211 #endif 00212 00213 00214 #if CFG_CHIP_TYPE == 1 00215 extern "C" __asm void PendSV_Handler() 00216 { 00217 IMPORT TCBRunning 00218 IMPORT TCBNext 00219 IMPORT OSSchedLock 00220 LDR R3,=TCBRunning 00221 LDR R1,[R3] ; R1 == running tcb 00222 LDR R2,=TCBNext 00223 LDR R2,[R2] ; R2 == next tcb 00224 00225 CMP R1,R2 00226 BEQ exitPendSV 00227 MRS R0, PSP ; Get PSP point (can not use PUSH,in ISR,SP is MSP ) 00228 STMDB R0!,{R4-R11} ; Store r4-r11,r0 -= regCnt * 4,r0 is new stack 00229 ; top point (addr h->l r11,r10,...,r5,r4) 00230 STR R0,[R1] ; Save orig PSP 00231 popStk 00232 STR R2, [R3] ; TCBRunning = TCBNext ; 00233 LDR R0, [R2] ; Get SP of task that be switch into. 00234 LDMIA R0!,{R4-R11} ; POP (R4-R11),R0 += regCnt * 4 00235 MSR PSP, R0 ; Mov new stack point to PSP 00236 00237 exitPendSV 00238 LDR R3,=OSSchedLock 00239 MOVS R0, #0x0 00240 STRB R0, [R3] 00241 ORR LR, LR, #0x04 ; Ensure exception return uses process stack 00242 BX LR ; Exit interrupt 00243 00244 ALIGN 00245 } 00246 #endif 00247 00248
Generated on Tue Jul 12 2022 18:19:10 by
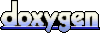