
CooCox 1.1.4 on mbed with simple blinky example
Embed:
(wiki syntax)
Show/hide line numbers
mbox.c
Go to the documentation of this file.
00001 /** 00002 ******************************************************************************* 00003 * @file mbox.c 00004 * @version V1.1.4 00005 * @date 2011.04.20 00006 * @brief Mailbox management implementation code of CooCox CoOS kernel. 00007 ******************************************************************************* 00008 * @copy 00009 * 00010 * INTERNAL FILE,DON'T PUBLIC. 00011 * 00012 * <h2><center>© COPYRIGHT 2009 CooCox </center></h2> 00013 ******************************************************************************* 00014 */ 00015 00016 /*---------------------------- Include ---------------------------------------*/ 00017 #include <coocox.h> 00018 00019 00020 #if CFG_MAILBOX_EN > 0 00021 00022 00023 /** 00024 ******************************************************************************* 00025 * @brief Create a mailbox 00026 * @param[in] sortType Mail box waiting list sort type. 00027 * @param[out] None 00028 * @retval E_CREATE_FAIL Create mailbox fail. 00029 * @retval others Create mailbox successful. 00030 * 00031 * @par Description 00032 * @details This function is called to create a mailbox. 00033 * @note 00034 ******************************************************************************* 00035 */ 00036 OS_EventID CoCreateMbox(U8 sortType) 00037 { 00038 P_ECB pecb; 00039 00040 /* Create a mailbox type event control block */ 00041 pecb = CreatEvent(EVENT_TYPE_MBOX,sortType,Co_NULL); 00042 if(pecb == Co_NULL) /* If failed to create event block */ 00043 { 00044 return E_CREATE_FAIL; 00045 } 00046 pecb->eventCounter = 0; 00047 return (pecb->id ); /* Create a mailbox successfully, return event ID */ 00048 } 00049 00050 00051 00052 /** 00053 ******************************************************************************* 00054 * @brief Delete a mailbox 00055 * @param[in] id Event ID. 00056 * @param[in] opt Delete option. 00057 * @param[out] None 00058 * @retval E_INVALID_ID Invalid event ID. 00059 * @retval E_INVALID_PARAMETER Invalid parameter. 00060 * @retval E_TASK_WAITTING Tasks waitting for the event,delete fail. 00061 * @retval E_OK Event deleted successful. 00062 * 00063 * @par Description 00064 * @details This function is called to delete a mailbox. 00065 * @note 00066 ******************************************************************************* 00067 */ 00068 StatusType CoDelMbox(OS_EventID id,U8 opt) 00069 { 00070 P_ECB pecb; 00071 00072 #if CFG_PAR_CHECKOUT_EN >0 00073 if(id >= CFG_MAX_EVENT) /* Judge id is valid or not? */ 00074 { 00075 return E_INVALID_ID; /* Id is invalid ,return error */ 00076 } 00077 #endif 00078 pecb = &EventTbl [id]; 00079 #if CFG_PAR_CHECKOUT_EN >0 00080 if(pecb->eventType != EVENT_TYPE_MBOX)/* Validate event control block type*/ 00081 { 00082 return E_INVALID_ID; /* The event is not mailbox */ 00083 } 00084 #endif 00085 return (DeleteEvent(pecb,opt)); /* Delete the mailbox event control block */ 00086 } 00087 00088 00089 00090 /** 00091 ******************************************************************************* 00092 * @brief Accept a mailbox 00093 * @param[in] id Event ID. 00094 * @param[out] perr A pointer to error code. 00095 * @retval Co_NULL 00096 * @retval A pointer to mailbox accepted. 00097 * 00098 * @par Description 00099 * @details This function is called to accept a mailbox. 00100 * @note 00101 ******************************************************************************* 00102 */ 00103 void* CoAcceptMail(OS_EventID id,StatusType* perr) 00104 { 00105 P_ECB pecb; 00106 void* pmail; 00107 #if CFG_PAR_CHECKOUT_EN >0 00108 if(id >= CFG_MAX_EVENT) 00109 { 00110 *perr = E_INVALID_ID; /* Invalid 'id' */ 00111 return Co_NULL; 00112 } 00113 #endif 00114 pecb = &EventTbl [id]; 00115 00116 #if CFG_PAR_CHECKOUT_EN >0 00117 if(pecb->eventType != EVENT_TYPE_MBOX)/* Invalid event control block type */ 00118 { 00119 *perr = E_INVALID_ID; 00120 return Co_NULL; 00121 } 00122 #endif 00123 OsSchedLock(); 00124 if(pecb->eventCounter == 1) /* If there is already a message */ 00125 { 00126 *perr = E_OK; 00127 pmail = pecb->eventPtr ; /* Get the message */ 00128 pecb->eventPtr = Co_NULL; /* Clear the mailbox */ 00129 pecb->eventCounter = 0; 00130 OsSchedUnlock(); 00131 return pmail; /* Return the message received */ 00132 } 00133 else /* If the mailbox is empty */ 00134 { 00135 OsSchedUnlock(); 00136 *perr = E_MBOX_EMPTY; /* Mailbox is empty,return Co_NULL */ 00137 return Co_NULL; 00138 } 00139 } 00140 00141 00142 00143 /** 00144 ******************************************************************************* 00145 * @brief Wait for a mailbox 00146 * @param[in] id Event ID. 00147 * @param[in] timeout The longest time for writting mail. 00148 * @param[out] perr A pointer to error code. 00149 * @retval Co_NULL 00150 * @retval A pointer to mailbox accept. 00151 * 00152 * @par Description 00153 * @details This function is called to wait a mailbox. 00154 * @note 00155 ******************************************************************************* 00156 */ 00157 void* CoPendMail(OS_EventID id,U32 timeout,StatusType* perr) 00158 { 00159 P_ECB pecb; 00160 void* pmail; 00161 P_OSTCB curTCB; 00162 00163 if(OSIntNesting > 0) /* If the caller is ISR */ 00164 { 00165 *perr = E_CALL; 00166 return Co_NULL; 00167 } 00168 00169 #if CFG_PAR_CHECKOUT_EN >0 00170 if(id >= CFG_MAX_EVENT) 00171 { 00172 *perr = E_INVALID_ID; /* Invalid 'id',retrun error */ 00173 return Co_NULL; 00174 } 00175 #endif 00176 00177 pecb = &EventTbl [id]; 00178 #if CFG_PAR_CHECKOUT_EN >0 00179 if(pecb->eventType != EVENT_TYPE_MBOX) 00180 { 00181 *perr = E_INVALID_ID; /* Invalid event type,not EVENT_TYPE_MBOX */ 00182 return Co_NULL; 00183 } 00184 #endif 00185 00186 if(OSSchedLock != 0) /* Judge schedule is locked or not? */ 00187 { 00188 *perr = E_OS_IN_LOCK; /* Schedule is locked */ 00189 return Co_NULL; /* return Co_NULL */ 00190 } 00191 OsSchedLock(); 00192 if( pecb->eventCounter == 1) /* If there is already a message */ 00193 { 00194 *perr = E_OK; 00195 pmail = pecb->eventPtr ; /* Get the message */ 00196 pecb->eventPtr = Co_NULL; /* Clear the mailbox */ 00197 pecb->eventCounter = 0; 00198 OsSchedUnlock(); 00199 return pmail; /* Return the message received */ 00200 } 00201 else /* If message is not available, task will pend */ 00202 { 00203 OsSchedUnlock(); 00204 curTCB = TCBRunning ; 00205 if(timeout == 0) /* If time-out is not configured */ 00206 { 00207 EventTaskToWait(pecb,curTCB); /* Block task until event occurs */ 00208 *perr = E_OK; 00209 00210 /* Have recived a message or the mailbox have been deleted */ 00211 pmail = curTCB->pmail ; 00212 curTCB->pmail = Co_NULL; 00213 return pmail; /* Return received message or Co_NULL */ 00214 } 00215 else /* If time-out is configured */ 00216 { 00217 OsSchedLock(); 00218 00219 /* Block task until event or timeout occurs */ 00220 EventTaskToWait(pecb,curTCB); 00221 InsertDelayList(curTCB,timeout); 00222 OsSchedUnlock(); 00223 if( curTCB->pmail == Co_NULL) /* Time-out occurred */ 00224 { 00225 *perr = E_TIMEOUT; 00226 return Co_NULL; 00227 } 00228 else /* Have recived a message or the mailbox have been deleted*/ 00229 { 00230 *perr = E_OK; 00231 pmail = curTCB->pmail ; 00232 curTCB->pmail = Co_NULL; 00233 return pmail; /* Return received message or Co_NULL */ 00234 } 00235 } 00236 } 00237 } 00238 00239 00240 /** 00241 ******************************************************************************* 00242 * @brief Post a mailbox 00243 * @param[in] id Event ID. 00244 * @param[in] pmail Pointer to mail that want to send. 00245 * @param[out] None 00246 * @retval E_INVALID_ID 00247 * @retval E_OK 00248 * 00249 * @par Description 00250 * @details This function is called to post a mail. 00251 * @note 00252 ******************************************************************************* 00253 */ 00254 StatusType CoPostMail(OS_EventID id,void* pmail) 00255 { 00256 P_ECB pecb; 00257 #if CFG_PAR_CHECKOUT_EN >0 00258 if(id >= CFG_MAX_EVENT) 00259 { 00260 return E_INVALID_ID; /* Invalid id,return error */ 00261 } 00262 #endif 00263 00264 pecb = &EventTbl [id]; 00265 #if CFG_PAR_CHECKOUT_EN >0 00266 if(pecb->eventType != EVENT_TYPE_MBOX)/* Validate event control block type*/ 00267 { 00268 return E_INVALID_ID; /* Event is not mailbox,return error*/ 00269 } 00270 #endif 00271 00272 if(pecb->eventCounter == 0) /* If mailbox doesn't already have a message*/ 00273 { 00274 OsSchedLock(); 00275 pecb->eventPtr = pmail; /* Place message in mailbox */ 00276 pecb->eventCounter = 1; 00277 EventTaskToRdy(pecb); /* Check waiting list */ 00278 OsSchedUnlock(); 00279 return E_OK; 00280 } 00281 else /* If there is already a message in mailbox */ 00282 { 00283 return E_MBOX_FULL; /* Mailbox is full,and return "E_MBOX_FULL" */ 00284 } 00285 } 00286 00287 /** 00288 ******************************************************************************* 00289 * @brief Post a mailbox in ISR 00290 * @param[in] id Event ID. 00291 * @param[in] pmail Pointer to mail that want to send. 00292 * @param[out] None 00293 * @retval E_INVALID_ID 00294 * @retval E_OK 00295 * 00296 * @par Description 00297 * @details This function is called to post a mail in ISR. 00298 * @note 00299 ******************************************************************************* 00300 */ 00301 #if CFG_MAX_SERVICE_REQUEST > 0 00302 StatusType isr_PostMail(OS_EventID id,void* pmail) 00303 { 00304 if(OSSchedLock > 0) /* If scheduler is locked,(the caller is ISR) */ 00305 { 00306 /* Insert the request into service request queue */ 00307 if(InsertInSRQ(MBOX_REQ,id,pmail) == Co_FALSE) 00308 { 00309 return E_SEV_REQ_FULL; /* If service request queue is full */ 00310 } 00311 else /* Operate successfully */ 00312 { 00313 return E_OK; 00314 } 00315 } 00316 else 00317 { 00318 return(CoPostMail(id,pmail)); /* Sends the message to the mailbox */ 00319 } 00320 } 00321 #endif 00322 00323 #endif 00324 00325
Generated on Tue Jul 12 2022 18:19:10 by
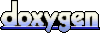