
CooCox 1.1.4 on mbed with simple blinky example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // CooCox RTOS v1.1.4 with simple example 00002 // Two LEDs will blink with 250ms and 500ms interval based on two seperate tasks 00003 // by electronix79, 29/July/2011 00004 // 00005 // Comments: Nice work by Eric Ebert which used CooCox RTOS v1.1.3 but all files of CoOS were in one main folder, 00006 // so I create new project with the same structure as in CooCox design and of cause I use the updated version 1.1.4 00007 00008 #include "mbed.h" 00009 00010 #include <CoOS.h> 00011 00012 DigitalOut led1(LED1), led4(LED4); 00013 00014 #define TASK_STACK_SIZE 128 /*!< Define task size */ 00015 00016 #define PRIORITY_TASK1 1 /* Priority of task 1 */ 00017 #define PRIORITY_TASK2 2 /* Priority of task 2 */ 00018 #define PRIORITY_TASK3 3 /* Priority of task 3 */ 00019 00020 OS_STK task1_stk[TASK_STACK_SIZE]; /*!< Define "task1" task stack */ 00021 OS_STK task2_stk[TASK_STACK_SIZE]; /*!< Define "task2" task stack */ 00022 OS_STK task3_stk[TASK_STACK_SIZE]; /*!< Define "task3" task stack */ 00023 00024 OS_TID task1_id; /*!< Task ID of 'task1'. */ 00025 OS_TID task2_id; /*!< Task ID of 'task2'. */ 00026 OS_TID task3_id; /*!< Task ID of 'task3'. */ 00027 00028 OS_MutexID mut_1; /*!< Save id of mutex. */ 00029 00030 void task1(void *); 00031 void task2(void *); 00032 void task3(void *); 00033 00034 void task1(void* pdata) 00035 { 00036 mut_1 = CoCreateMutex(); /* Create a mutex */ 00037 00038 task2_id = CoCreateTask(task2, (void *)0, PRIORITY_TASK2, &task2_stk[TASK_STACK_SIZE-1], TASK_STACK_SIZE); 00039 task3_id = CoCreateTask(task3, (void *)0, PRIORITY_TASK3, &task3_stk[TASK_STACK_SIZE-1], TASK_STACK_SIZE); 00040 00041 CoExitTask(); /* Delete 'task1' task. */ 00042 } 00043 00044 void task2(void* pdata) 00045 { 00046 for(;;) 00047 { 00048 CoEnterMutexSection(mut_1); /* Enter critical region */ 00049 led1 = 1; /* Turn On Led */ 00050 CoLeaveMutexSection(mut_1); /* Exit critical region */ 00051 CoTickDelay(250); /* Delay 250ms */ 00052 CoEnterMutexSection(mut_1); /* Enter critical region */ 00053 led1 = 0; /* Turn Off Led */ 00054 CoLeaveMutexSection(mut_1); /* Exit critical region */ 00055 CoTickDelay(250); /* Delay 250ms */ 00056 } 00057 } 00058 00059 void task3(void* pdata) 00060 { 00061 for(;;) 00062 { 00063 CoEnterMutexSection(mut_1); /* Enter critical region */ 00064 led4 = 1; /* Turn On Led */ 00065 CoLeaveMutexSection(mut_1); /* Exit critical region */ 00066 CoTickDelay(500); /* Delay 500ms */ 00067 CoEnterMutexSection(mut_1); /* Enter critical region */ 00068 led4 = 0; /* Turn Off Led */ 00069 CoLeaveMutexSection(mut_1); /* Exit critical region */ 00070 CoTickDelay(500); /* Delay 500ms */ 00071 } 00072 } 00073 00074 int main() 00075 { 00076 CoInitOS(); /*!< Initial CooCox CoOS */ 00077 00078 task1_id = CoCreateTask(task1, (void *)0, PRIORITY_TASK1, &task1_stk[TASK_STACK_SIZE-1], TASK_STACK_SIZE); 00079 00080 CoStartOS(); /*!< Start multitask */ 00081 00082 while (1); /*!< The code don't reach here */ 00083 }
Generated on Tue Jul 12 2022 18:19:10 by
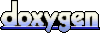