
CooCox 1.1.4 on mbed with simple blinky example
Embed:
(wiki syntax)
Show/hide line numbers
OsEvent.h
Go to the documentation of this file.
00001 /** 00002 ******************************************************************************* 00003 * @file OsEvent.h 00004 * @version V1.1.4 00005 * @date 2011.04.20 00006 * @brief Event management header file 00007 * @details This file including some defines and declares related to event 00008 * (semaphore,mailbox,queque) management. 00009 ******************************************************************************* 00010 * @copy 00011 * 00012 * INTERNAL FILE,DON'T PUBLIC. 00013 * 00014 * <h2><center>© COPYRIGHT 2009 CooCox </center></h2> 00015 ******************************************************************************* 00016 */ 00017 00018 00019 #ifndef _EVENT_H 00020 #define _EVENT_H 00021 00022 #define EVENT_TYPE_SEM (U8)0x01 /*!< Event type:Semaphore. */ 00023 #define EVENT_TYPE_MBOX (U8)0x02 /*!< Event type:Mailbox. */ 00024 #define EVENT_TYPE_QUEUE (U8)0x03 /*!< Event type:Queue. */ 00025 #define EVENT_TYPE_INVALID (U8)0x04 /*!< Invalid event type. */ 00026 00027 00028 /** 00029 * @struct EventCtrBlk event.h 00030 * @brief Event control block 00031 * @details This struct is use to manage event, 00032 * e.g. semaphore,mailbox,queue. 00033 */ 00034 typedef struct EventCtrBlk 00035 { 00036 void* eventPtr ; /*!< Point to mailbox or queue struct */ 00037 U8 id ; /*!< ECB id */ 00038 U8 eventType :4; /*!< Type of event */ 00039 U8 eventSortType :4; /*!< 0:FIFO 1: Preemptive by prio */ 00040 U16 eventCounter ; /*!< Counter of semaphore. */ 00041 U16 initialEventCounter ; /*!< Initial counter of semaphore. */ 00042 P_OSTCB eventTCBList ; /*!< Task waitting list. */ 00043 }ECB,*P_ECB; 00044 00045 /*---------------------------- Variable declare ------------------------------*/ 00046 extern ECB EventTbl [CFG_MAX_EVENT]; /*!< Table use to save TCB. */ 00047 00048 /*---------------------------- Function declare ------------------------------*/ 00049 /*!< Create a event */ 00050 extern P_ECB CreatEvent(U8 eventType,U8 eventSortType,void* eventPtr); 00051 00052 /*!< Remove a task from wait list */ 00053 extern void EventTaskToWait(P_ECB pecb,P_OSTCB ptcb); 00054 extern StatusType DeleteEvent(P_ECB pecb,U8 opt); /*!< Delete a event. */ 00055 extern void EventTaskToRdy(P_ECB pecb); /*!< Insert a task to ready list*/ 00056 extern void CreateEventList(void); /*!< Create a event list. */ 00057 extern void RemoveEventWaittingList(P_OSTCB ptcb); 00058 #endif
Generated on Tue Jul 12 2022 18:19:10 by
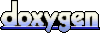