
for BLENano v1 AD8232
Dependencies: BLE_API mbed nRF51822
Fork of BLENano_SimpleTemplate by
main.cpp
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 /* 00021 * The application works with the BlueJelly.js 00022 * 00023 * http://jellyware.jp/kurage/ 00024 * https://github.com/electricbaka/bluejelly 00025 * 00026 */ 00027 00028 //====================================================================== 00029 //Grobal 00030 //====================================================================== 00031 //------------------------------------------------------------ 00032 //Include Header Files 00033 //------------------------------------------------------------ 00034 #include "mbed.h" 00035 #include "ble/BLE.h" 00036 00037 00038 //------------------------------------------------------------ 00039 //Definition 00040 //------------------------------------------------------------ 00041 #define TXRX_BUF_LEN 20 //max 20[byte] 00042 #define DEVICE_LOCAL_NAME "BlueJelly" 00043 #define ADVERTISING_INTERVAL 160 //160 * 0.625[ms] = 100[ms] 00044 #define TICKER_TIME 1000 //1000[us] = 1[ms] 00045 #define DIGITAL_OUT_PIN P0_9 00046 #define ANALOG_IN_PIN1 P0_4 00047 00048 //HeartRate 00049 int timer_num = 0; 00050 int threshold_v = 650; 00051 int threshold_t = 300; 00052 00053 00054 //------------------------------------------------------------ 00055 //Object generation 00056 //------------------------------------------------------------ 00057 BLE blenano; 00058 DigitalOut LED_SET(DIGITAL_OUT_PIN); 00059 AnalogIn ANALOG1(ANALOG_IN_PIN1); 00060 00061 00062 //------------------------------------------------------------ 00063 //Service & Characteristic Setting 00064 //------------------------------------------------------------ 00065 //Service UUID 00066 static const uint8_t base_uuid[] = { 0x71, 0x3D, 0x00, 0x00, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E } ; 00067 00068 //Characteristic UUID 00069 static const uint8_t tx_uuid[] = { 0x71, 0x3D, 0x00, 0x03, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E } ; 00070 static const uint8_t rx_uuid[] = { 0x71, 0x3D, 0x00, 0x02, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E } ; 00071 00072 //Characteristic Value 00073 uint8_t txPayload[TXRX_BUF_LEN] = {0,}; 00074 uint8_t rxPayload[TXRX_BUF_LEN] = {0,}; 00075 00076 //Characteristic Property Setting etc 00077 GattCharacteristic txCharacteristic (tx_uuid, txPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00078 GattCharacteristic rxCharacteristic (rx_uuid, rxPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY| GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00079 GattCharacteristic *myChars[] = {&txCharacteristic, &rxCharacteristic}; 00080 00081 //Service Setting 00082 GattService myService(base_uuid, myChars, sizeof(myChars) / sizeof(GattCharacteristic *)); 00083 00084 00085 //====================================================================== 00086 //onDisconnection 00087 //====================================================================== 00088 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00089 { 00090 blenano.startAdvertising(); 00091 } 00092 00093 00094 //====================================================================== 00095 //onDataWritten 00096 //====================================================================== 00097 void WrittenHandler(const GattWriteCallbackParams *Handler) 00098 { 00099 uint8_t buf[TXRX_BUF_LEN]; 00100 uint16_t bytesRead; 00101 00102 if (Handler->handle == txCharacteristic.getValueAttribute().getHandle()) 00103 { 00104 blenano.readCharacteristicValue(txCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00105 memset(txPayload, 0, TXRX_BUF_LEN); 00106 memcpy(txPayload, buf, TXRX_BUF_LEN); 00107 00108 if(buf[0] == 1) 00109 LED_SET = 1; 00110 else 00111 LED_SET = 0; 00112 } 00113 } 00114 00115 00116 //====================================================================== 00117 //onTimeout 00118 //====================================================================== 00119 void m_status_check_handle(void) 00120 { 00121 timer_num ++; 00122 } 00123 00124 00125 //====================================================================== 00126 //convert reverse UUID 00127 //====================================================================== 00128 void reverseUUID(const uint8_t* src, uint8_t* dst) 00129 { 00130 int i; 00131 00132 for(i=0;i<16;i++) 00133 dst[i] = src[15 - i]; 00134 } 00135 00136 00137 //====================================================================== 00138 //main 00139 //====================================================================== 00140 int main(void) 00141 { 00142 uint8_t base_uuid_rev[16]; 00143 00144 //Timer Setting [us] 00145 Ticker ticker; 00146 ticker.attach_us(m_status_check_handle, TICKER_TIME); 00147 00148 //BLE init 00149 blenano.init(); 00150 00151 //EventListener 00152 blenano.onDisconnection(disconnectionCallback); 00153 blenano.onDataWritten(WrittenHandler); 00154 00155 //------------------------------------------------------------ 00156 //setup advertising 00157 //------------------------------------------------------------ 00158 //Classic BT not support 00159 blenano.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00160 00161 //Connectable to Central 00162 blenano.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00163 00164 //Local Name 00165 blenano.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, 00166 (const uint8_t *)DEVICE_LOCAL_NAME, sizeof(DEVICE_LOCAL_NAME) - 1); 00167 00168 //GAP AdvertisingData 00169 reverseUUID(base_uuid, base_uuid_rev); 00170 blenano.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00171 (uint8_t *)base_uuid_rev, sizeof(base_uuid)); 00172 00173 //Advertising Interval 00174 blenano.setAdvertisingInterval(ADVERTISING_INTERVAL); 00175 00176 //Add Service 00177 blenano.addService(myService); 00178 00179 //Start Advertising 00180 blenano.startAdvertising(); 00181 00182 //------------------------------------------------------------ 00183 //Loop 00184 //------------------------------------------------------------ 00185 int timer_ms; 00186 00187 while(1) 00188 { 00189 blenano.waitForEvent(); 00190 00191 float s = ANALOG1; 00192 uint16_t value = s * 1024; 00193 00194 //--------------------------------------------------------------------------------------------- 00195 //Detect HeartRate Peak 00196 //--------------------------------------------------------------------------------------------- 00197 timer_ms = TICKER_TIME/1000 * timer_num; 00198 if(value >= threshold_v && timer_ms > threshold_t) 00199 { 00200 //clear timer_num 00201 timer_num = 0; 00202 00203 uint8_t buf[2]; 00204 buf[0] = (timer_ms >> 8); 00205 buf[1] = timer_ms; 00206 00207 //Send out 00208 blenano.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 2); 00209 } 00210 } 00211 }
Generated on Tue Jul 12 2022 20:45:56 by
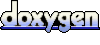