
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Snake.cpp
00001 #include "Snake.h" 00002 #include "math.h" 00003 #include <complex> 00004 00005 // Constructor 00006 Snake::Snake(float pos_x, float pos_y) 00007 { 00008 _hp = 4; 00009 _attack = 1; 00010 _face = 0; 00011 _prev_face = 0; 00012 _hp_drop_chance = 10; // out of 100 00013 00014 _hitbox.width = 4; 00015 _hitbox.height = 7; 00016 00017 _position.x = pos_x; 00018 _position.y = pos_y; 00019 00020 _sprite_size.width = 6; 00021 _sprite_size.height = 12; 00022 _sprite_size.offset_x = -1; 00023 _sprite_size.offset_y = -6; 00024 00025 _frame.count = 0; 00026 _frame.number = 0; 00027 _frame.max = 6; 00028 00029 _velocity = 0; 00030 _velocity_index = 0; 00031 } 00032 // Member Function 00033 void Snake::update_prev_face() 00034 { 00035 _prev_face = _face; 00036 } 00037 00038 // Member Mutator 00039 void Snake::update_hitbox(int _hitbox_width, int _hitbox_height, int _sprite_size_width, int _sprite_size_height, int _sprite_size_offset_x, int _sprite_size_offset_y, int max_frame) // Offset, Hitbox and Frame Count update 00040 { 00041 if (_prev_face != _face) { 00042 _frame.number = 0; // Resets animation everytime face changes 00043 _hitbox.width = _hitbox_width; 00044 _hitbox.height = _hitbox_height; 00045 00046 _sprite_size.width = _sprite_size_width; 00047 _sprite_size.height = _sprite_size_height; 00048 _sprite_size.offset_x = _sprite_size_offset_x; 00049 _sprite_size.offset_y = _sprite_size_offset_y; 00050 00051 _frame.max = max_frame; 00052 } 00053 } 00054 00055 // Functions 00056 void Snake::move(float player_x, float player_y, char * map, bool * doorways) 00057 { 00058 float diff_x = player_x - _position.x; 00059 float diff_y = player_y - _position.y; 00060 _velocity = snake_velocity_pattern[_velocity_index]; // Creating slithering effect, changing velocity of movement 00061 update_prev_face(); 00062 00063 // Setting Face 00064 update_face(diff_x, diff_y); 00065 00066 // Movement 00067 move_snake(); // Movement and updating _hitboxes 00068 00069 undo_move_x(entity_to_map_collision_test(_position.x, _prev_pos.y, map, doorways)); 00070 undo_move_y(entity_to_map_collision_test(_prev_pos.x, _position.y, map, doorways)); 00071 00072 increment_frame(); 00073 } 00074 00075 void Snake::update_face(float diff_x, float diff_y) // Depending on the displacement of player from snake, after a full slither effect, change the face 00076 { 00077 if (_velocity_index == 0) { 00078 if (abs(diff_x) > abs(diff_y)) { 00079 if (diff_x > 0) { 00080 _face = 1; 00081 } else { 00082 _face = 3; 00083 } 00084 } else { 00085 if (diff_y > 0) { 00086 _face = 2; 00087 } else { 00088 _face = 0; 00089 } 00090 } 00091 } 00092 } 00093 00094 void Snake::move_snake() // Moves the Snake according to velocity, updates the _hitboxes everytime it changes face 00095 { 00096 if (_face == 0) { 00097 _position.y -= _velocity; 00098 update_hitbox(4, 7, 6, 12, -1, -6, 6); 00099 } else if (_face == 1) { 00100 _position.x += _velocity; 00101 update_hitbox(7, 4, 12, 7, -6, -4, 4); 00102 } else if (_face == 2) { 00103 _position.y += _velocity; 00104 update_hitbox(4, 7, 6, 12, -1, -5, 6); 00105 } else if (_face == 3) { 00106 _position.x -= _velocity; 00107 update_hitbox(7, 4, 12, 7, 0, -4, 4); 00108 } 00109 } 00110 00111 void Snake::increment_frame() // Frame increment and velocity index increment 00112 { 00113 _frame.count++; 00114 if (_frame.count >= 10) { // Every 10 frames, sprite_frames increments and velocity_index increments 00115 _frame.count = 0; 00116 _velocity_index++; 00117 _frame.number++; 00118 if (_velocity_index >= 6) { // Velocity_index max; reset 00119 _velocity_index = 0; 00120 } 00121 if (_frame.number >= _frame.max) { // Frame.number max; reset 00122 _frame.number = 0; 00123 } 00124 } 00125 } 00126 00127 void Snake::take_damage(int damage) 00128 { 00129 _hp -= damage; 00130 } 00131 00132 char * Snake::get_frame() // Returns the frame needed 00133 { 00134 if(_face == 0) { 00135 return (char *) sprite_snake_y[0][_frame.number]; 00136 } else if(_face == 1) { 00137 return (char *) sprite_snake_x[0][_frame.number]; 00138 } else if(_face == 2) { 00139 return (char *) sprite_snake_y[1][_frame.number]; 00140 } else if(_face == 3) { 00141 return (char *) sprite_snake_x[1][_frame.number]; 00142 } 00143 return 0; 00144 } 00145 00146 void Snake::draw(N5110 &lcd) 00147 { 00148 lcd.drawSpriteTransparent(get_pos_x()+_sprite_size.offset_x, 00149 get_pos_y()+_sprite_size.offset_y, 00150 _sprite_size.height, 00151 _sprite_size.width, 00152 get_frame()); 00153 }
Generated on Tue Jul 19 2022 23:32:07 by
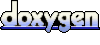