
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Skull.h
00001 #ifndef SKULL_H 00002 #define SKULL_H 00003 #include "Entity.h" 00004 #define DASH_DELAY 120 00005 00006 /**Skull Class 00007 *@author Steven Mahasin 00008 *@brief Creates a Skull which inherits the Entity class, this is currently the only boss in the game. 00009 *@date May 2019 00010 */ 00011 class Skull : public Entity { 00012 private: 00013 // Member Variables 00014 /** 00015 * @brief the shadow of Skull has a separate size and offset, so it has to have such member variable 00016 */ 00017 SpriteSize _shadow; 00018 /** 00019 * @brief a status of wether the skull is dashing 00020 */ 00021 bool _dash; 00022 /** 00023 * @brief a counter to let the skull dash periodically 00024 */ 00025 int _dash_counter; 00026 /** 00027 * @brief an index to choose which velocity the Skull currently has when dashing 00028 */ 00029 int _velocity_index; 00030 00031 // Member Functions 00032 /** 00033 * @brief increase _frame.count which increases _frame.number to animate skull 00034 */ 00035 void increment_frames(); 00036 /** 00037 * @brief updates the offset of the skull so that it floats up and down periodically above the shadow (purely graphical) 00038 */ 00039 void update_offsets(); 00040 /** 00041 * @brief moves the skull towards the player, similar to headless 00042 * @param player_x @details player x-position 00043 * @param player_y @details player y-position 00044 */ 00045 void approaching_movement(float player_x, float player_y); 00046 /** 00047 * @brief moves the skull in a dashing manner 00048 */ 00049 void dash_movement(); 00050 00051 public: 00052 // Constructor 00053 Skull(float pos_x, float pos_y); 00054 00055 // Functions 00056 /** 00057 * @brief calls the function and conditions to move (both dashing and approaching) 00058 * @param x_value @details player x-position 00059 * @param y_value @details player y-position 00060 * @param map @details the 2d map array that dictates where there are walls or empty space 00061 * @param doorways @details an array that dictates which side of the wall has a doorway 00062 */ 00063 virtual void move(float x_value, float y_value, char * map, bool * doorways); // movement control and miscellaneous updates 00064 /** 00065 * @brief reduce _hp by damage 00066 * @param damage @details the amount of damage to be taken 00067 */ 00068 virtual void take_damage(int); 00069 /** 00070 * @brief a function of drawing the skull onto the screen 00071 * @param lcd @details the screen where the skull is drawn on 00072 */ 00073 virtual void draw(N5110 &lcd); 00074 }; 00075 00076 const float skull_velocity_pattern[7] = {0, 0, 0, 0.8, 1.6, 2}; 00077 00078 const char skull_sprite[4][2][23][21] = //skull_sprite[Face][mouthclose/mouthopen][Size_Y][Size_X] 00079 { 00080 { // Up 00081 { 00082 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00083 {0,0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0}, 00084 {0,0,0,1,1,2,1,2,2,2,2,2,2,2,2,2,1,1,0,0,0}, 00085 {0,0,1,2,2,2,2,1,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00086 {0,1,2,2,2,2,2,1,1,1,2,2,2,2,2,2,2,2,2,1,0}, 00087 {0,1,2,2,2,2,2,2,1,2,1,2,2,2,2,2,2,2,2,1,0}, 00088 {0,1,2,2,2,2,2,2,1,2,2,2,2,2,2,2,2,2,2,1,0}, 00089 {1,2,2,2,2,2,2,1,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00090 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00091 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00092 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00093 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00094 {1,2,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,2,1}, 00095 {0,1,2,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,2,1,0}, 00096 {0,1,2,2,1,1,1,1,2,2,2,2,2,1,1,1,1,2,2,1,0}, 00097 {0,0,1,2,1,1,1,1,1,2,2,2,1,1,1,1,1,2,1,0,0}, 00098 {0,0,1,2,2,1,1,1,2,2,2,2,2,1,1,1,2,2,1,0,0}, 00099 {0,0,0,1,2,2,1,1,1,2,2,2,1,1,1,2,2,1,0,0,0}, 00100 {0,0,0,0,1,2,2,1,1,1,1,1,1,1,2,2,1,0,0,0,0}, 00101 {0,0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0}, 00102 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00103 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00104 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00105 }, 00106 { 00107 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00108 {0,0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0}, 00109 {0,0,0,1,1,2,1,2,2,2,2,2,2,2,2,2,1,1,0,0,0}, 00110 {0,0,1,2,2,2,2,1,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00111 {0,1,2,2,2,2,2,1,1,1,2,2,2,2,2,2,2,2,2,1,0}, 00112 {0,1,2,2,2,2,2,2,1,2,1,2,2,2,2,2,2,2,2,1,0}, 00113 {0,1,2,2,2,2,2,2,1,2,2,2,2,2,2,2,2,2,2,1,0}, 00114 {1,2,2,2,2,2,2,1,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00115 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00116 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00117 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00118 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1}, 00119 {1,2,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,2,1}, 00120 {0,1,2,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,2,1,0}, 00121 {0,1,2,2,1,1,1,1,2,2,2,2,2,1,1,1,1,2,2,1,0}, 00122 {0,0,1,2,1,1,1,1,1,2,2,2,1,1,1,1,1,2,1,0,0}, 00123 {0,0,1,2,1,1,1,1,2,2,2,2,2,1,1,1,1,2,1,0,0}, 00124 {0,0,1,2,2,1,1,1,1,2,2,2,1,1,1,1,2,2,1,0,0}, 00125 {0,0,0,1,2,2,1,1,1,1,1,1,1,1,1,2,2,1,0,0,0}, 00126 {0,0,0,0,1,2,1,1,1,1,1,1,1,1,1,2,1,0,0,0,0}, 00127 {0,0,0,0,0,1,2,2,1,1,1,1,1,2,2,1,0,0,0,0,0}, 00128 {0,0,0,0,0,0,1,1,2,2,2,2,2,1,1,0,0,0,0,0,0}, 00129 {0,0,0,0,0,0,0,0,1,1,1,1,1,0,0,0,0,0,0,0,0}, 00130 } 00131 }, 00132 { // Right 00133 { 00134 {0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0}, 00135 {0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0,0}, 00136 {0,0,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,0,0,0,0}, 00137 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0,0}, 00138 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00139 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00140 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00141 {1,2,2,2,2,1,2,2,2,2,2,2,1,1,1,2,1,2,2,1,0}, 00142 {1,2,2,2,2,2,1,2,2,2,2,1,1,1,1,1,1,2,2,1,0}, 00143 {1,2,2,2,2,1,1,1,2,2,2,1,1,1,2,2,1,1,2,1,0}, 00144 {1,2,2,2,1,1,1,1,2,2,2,2,1,1,2,2,1,1,2,1,0}, 00145 {0,1,2,2,2,2,2,2,2,2,2,2,2,1,1,1,1,2,2,1,0}, 00146 {0,0,1,1,1,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00147 {0,0,0,0,0,1,2,2,2,2,1,2,2,2,2,2,2,1,1,0,0}, 00148 {0,0,0,0,0,0,1,2,2,2,1,1,2,1,2,2,2,1,1,0,0}, 00149 {0,0,0,0,0,0,1,2,2,2,2,1,1,1,1,1,2,2,1,0,0}, 00150 {0,0,0,0,0,0,1,1,2,2,2,2,2,1,2,1,1,1,1,0,0}, 00151 {0,0,0,0,0,0,0,1,1,2,2,2,2,2,2,1,2,1,0,0,0}, 00152 {0,0,0,0,0,0,0,0,0,1,1,1,2,2,2,2,2,1,0,0,0}, 00153 {0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,2,1,1,0,0,0}, 00154 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,0,0,0,0}, 00155 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00156 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00157 }, 00158 { 00159 {0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0,0}, 00160 {0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0,0}, 00161 {0,0,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,0,0,0,0}, 00162 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0,0}, 00163 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00164 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00165 {1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00166 {1,2,2,2,2,1,2,2,2,2,2,2,1,1,1,2,1,2,2,1,0}, 00167 {1,2,2,2,2,2,1,2,2,2,2,1,1,1,1,1,1,2,2,1,0}, 00168 {1,2,2,2,2,1,1,1,2,2,2,1,1,1,1,2,1,1,2,1,0}, 00169 {1,2,2,2,1,1,1,1,2,2,2,2,1,1,1,1,1,1,2,1,0}, 00170 {0,1,2,2,2,2,2,2,2,2,2,2,2,1,1,1,1,2,2,1,0}, 00171 {0,0,1,1,1,2,2,2,2,2,2,2,2,2,2,2,2,2,1,2,0}, 00172 {0,0,0,0,0,1,2,2,2,2,2,2,2,2,2,2,2,1,1,0,0}, 00173 {0,0,0,0,0,0,1,2,2,1,1,1,2,2,2,2,2,1,1,0,0}, 00174 {0,0,0,0,0,0,1,2,2,2,1,1,1,2,1,2,2,2,1,0,0}, 00175 {0,0,0,0,0,0,1,2,2,2,2,1,0,1,2,1,1,2,1,0,0}, 00176 {0,0,0,0,0,0,1,2,2,2,2,1,0,1,0,0,0,1,0,0,0}, 00177 {0,0,0,0,0,0,0,1,2,2,2,2,1,2,1,1,0,0,0,0,0}, 00178 {0,0,0,0,0,0,0,0,1,1,2,2,2,2,1,2,1,0,0,0,0}, 00179 {0,0,0,0,0,0,0,0,0,0,1,1,2,2,2,2,2,1,0,0,0}, 00180 {0,0,0,0,0,0,0,0,0,0,0,0,1,2,2,2,2,1,0,0,0}, 00181 {0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,0,0,0,0}, 00182 } 00183 }, 00184 { // Down 00185 { 00186 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00187 {0,0,0,0,0,1,1,2,2,2,2,1,1,2,1,1,0,0,0,0,0}, 00188 {0,0,0,1,1,2,2,2,2,2,2,2,1,1,2,2,1,1,0,0,0}, 00189 {0,0,1,2,2,2,2,2,2,2,2,2,2,1,1,1,2,2,1,0,0}, 00190 {0,1,2,2,2,2,2,2,2,2,2,2,1,2,2,2,2,2,2,1,0}, 00191 {0,1,2,2,2,2,2,1,2,2,2,2,2,1,2,2,2,2,2,1,0}, 00192 {0,1,2,2,1,1,1,1,2,2,2,2,2,1,1,1,1,2,2,1,0}, 00193 {1,2,2,1,1,1,2,1,2,2,2,2,2,1,2,1,1,1,2,2,1}, 00194 {1,2,2,1,1,2,2,2,1,2,2,2,1,2,2,2,1,1,2,2,1}, 00195 {1,2,2,1,1,2,2,1,2,2,2,2,2,1,2,2,1,1,2,2,1}, 00196 {1,2,2,2,1,1,1,2,2,2,1,2,2,2,1,1,1,2,2,2,1}, 00197 {1,2,2,2,2,2,2,2,2,1,1,1,2,2,2,2,2,2,2,2,1}, 00198 {1,2,1,2,2,2,2,2,2,1,2,1,2,2,2,2,2,2,1,2,1}, 00199 {0,1,2,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,2,1,0}, 00200 {0,1,2,2,1,1,1,2,1,2,1,2,1,2,1,1,1,2,2,1,0}, 00201 {0,0,1,2,1,1,1,1,1,1,1,1,1,1,1,1,1,2,1,0,0}, 00202 {0,0,1,2,2,1,1,2,1,2,1,2,1,2,1,1,2,2,1,0,0}, 00203 {0,0,0,1,2,2,1,2,2,2,2,2,2,2,1,2,2,1,0,0,0}, 00204 {0,0,0,0,1,2,2,2,2,2,2,2,2,2,2,2,1,0,0,0,0}, 00205 {0,0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0}, 00206 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00207 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00208 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00209 }, 00210 { 00211 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00212 {0,0,0,0,0,1,1,2,2,2,2,1,1,2,1,1,0,0,0,0,0}, 00213 {0,0,0,1,1,2,2,2,2,2,2,2,1,1,2,2,1,1,0,0,0}, 00214 {0,0,1,2,2,2,2,2,2,2,2,2,2,1,1,1,2,2,1,0,0}, 00215 {0,1,2,2,2,2,2,2,2,2,2,2,1,2,2,2,2,2,2,1,0}, 00216 {0,1,2,2,2,2,2,1,2,2,2,2,2,1,2,2,2,2,2,1,0}, 00217 {0,1,2,2,1,1,1,1,2,2,2,2,2,1,1,1,1,2,2,1,0}, 00218 {1,2,2,1,1,1,1,1,2,2,2,2,2,1,1,1,1,1,2,2,1}, 00219 {1,2,2,1,1,1,2,1,1,2,2,2,1,1,2,1,1,1,2,2,1}, 00220 {1,2,2,1,1,1,1,1,2,2,2,2,2,1,1,1,1,1,2,2,1}, 00221 {1,2,2,2,1,1,1,2,2,2,1,2,2,2,1,1,1,2,2,2,1}, 00222 {1,2,2,2,2,2,2,2,2,1,1,1,2,2,2,2,2,2,2,2,1}, 00223 {1,2,1,2,2,2,2,2,2,1,2,1,2,2,2,2,2,2,1,2,1}, 00224 {0,1,2,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,2,1,0}, 00225 {0,1,2,2,1,1,1,2,2,2,2,2,2,2,1,1,1,2,2,1,0}, 00226 {0,0,1,2,1,1,1,2,1,2,1,2,1,2,1,1,1,2,1,0,0}, 00227 {0,0,1,2,1,1,1,1,1,1,1,1,1,1,1,1,1,2,1,0,0}, 00228 {0,0,1,2,2,1,1,1,1,1,1,1,1,1,1,1,2,2,1,0,0}, 00229 {0,0,0,1,2,2,1,1,1,1,1,1,1,1,1,2,2,1,0,0,0}, 00230 {0,0,0,0,1,2,1,2,1,2,1,2,1,2,1,2,1,0,0,0,0}, 00231 {0,0,0,0,0,1,2,2,2,2,2,2,2,2,2,1,0,0,0,0,0}, 00232 {0,0,0,0,0,0,1,1,2,2,2,2,2,1,1,0,0,0,0,0,0}, 00233 {0,0,0,0,0,0,0,0,1,1,1,1,1,0,0,0,0,0,0,0,0}, 00234 } 00235 }, 00236 { // Left 00237 { 00238 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00239 {0,0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0}, 00240 {0,0,0,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,0,0,0}, 00241 {0,0,1,2,1,1,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00242 {0,1,2,2,2,2,1,1,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00243 {0,1,2,2,2,1,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0}, 00244 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0}, 00245 {1,2,2,1,2,1,1,1,2,2,2,2,2,2,1,2,2,2,2,1,0}, 00246 {1,2,2,1,1,1,1,1,1,2,2,2,2,1,2,2,2,2,2,1,0}, 00247 {1,2,1,1,2,2,1,1,1,2,2,2,1,1,1,2,2,2,2,1,0}, 00248 {1,2,1,1,2,2,1,1,2,2,2,2,1,1,1,1,2,2,2,1,0}, 00249 {1,2,2,1,1,1,1,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00250 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,1,1,1,0,0,0}, 00251 {0,1,1,2,2,2,2,2,2,1,2,2,2,2,1,0,0,0,0,0,0}, 00252 {0,1,1,2,2,2,1,2,1,1,2,2,2,1,0,0,0,0,0,0,0}, 00253 {0,1,2,2,1,1,1,1,1,2,2,2,2,1,0,0,0,0,0,0,0}, 00254 {0,1,1,1,1,2,1,2,2,2,2,2,1,1,0,0,0,0,0,0,0}, 00255 {0,0,1,2,1,2,2,2,2,2,2,1,1,0,0,0,0,0,0,0,0}, 00256 {0,0,1,2,2,2,2,2,1,1,1,0,0,0,0,0,0,0,0,0,0}, 00257 {0,0,1,1,2,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00258 {0,0,0,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00259 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00260 {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00261 }, 00262 { 00263 {0,0,0,0,0,0,0,1,1,1,1,1,1,1,0,0,0,0,0,0,0}, 00264 {0,0,0,0,0,1,1,2,2,2,2,2,2,2,1,1,0,0,0,0,0}, 00265 {0,0,0,1,1,2,2,2,2,2,2,2,2,2,2,2,1,1,0,0,0}, 00266 {0,0,1,2,1,1,2,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00267 {0,1,2,2,2,2,1,1,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00268 {0,1,2,2,2,1,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0}, 00269 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,1,0}, 00270 {1,2,2,1,2,1,1,1,2,2,2,2,2,2,1,2,2,2,2,1,0}, 00271 {1,2,2,1,1,1,1,1,1,2,2,2,2,1,2,2,2,2,2,1,0}, 00272 {1,2,1,1,2,1,1,1,1,2,2,2,1,1,1,2,2,2,2,1,0}, 00273 {1,2,1,1,1,1,1,1,2,2,2,2,1,1,1,1,2,2,2,1,0}, 00274 {1,2,2,1,1,1,1,2,2,2,2,2,2,2,2,2,2,2,1,0,0}, 00275 {0,1,2,2,2,2,2,2,2,2,2,2,2,2,2,1,1,1,0,0,0}, 00276 {0,1,1,2,2,2,2,2,2,2,2,2,2,2,1,0,0,0,0,0,0}, 00277 {0,1,1,2,2,2,2,2,1,1,1,2,2,1,0,0,0,0,0,0,0}, 00278 {0,1,2,2,2,1,2,1,1,1,2,2,2,1,0,0,0,0,0,0,0}, 00279 {0,1,2,1,1,0,1,0,1,2,2,2,2,1,0,0,0,0,0,0,0}, 00280 {0,0,1,0,0,0,1,0,1,2,2,2,2,1,0,0,0,0,0,0,0}, 00281 {0,0,0,0,1,1,2,1,2,2,2,2,1,0,0,0,0,0,0,0,0}, 00282 {0,0,0,1,2,1,2,2,2,2,1,1,0,0,0,0,0,0,0,0,0}, 00283 {0,0,1,2,2,2,2,2,1,1,0,0,0,0,0,0,0,0,0,0,0}, 00284 {0,0,1,2,2,2,2,1,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00285 {0,0,0,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0}, 00286 } 00287 }, 00288 00289 }; 00290 00291 const char skull_shadow_sprite[2][5][19] = 00292 { 00293 { 00294 {0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0}, 00295 {0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0}, 00296 {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1}, 00297 {0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0}, 00298 {0,0,0,0,1,1,1,1,1,1,1,1,1,1,1,0,0,0,0} 00299 }, 00300 { 00301 {0,0,0,0,0,1,1,1,1,1,1,1,1,1,0,0,0,0,0}, 00302 {0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0}, 00303 {0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0}, 00304 {0,0,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,0,0}, 00305 {0,0,0,0,0,1,1,1,1,1,1,1,1,1,0,0,0,0,0} 00306 } 00307 }; 00308 00309 #endif
Generated on Tue Jul 19 2022 23:32:07 by
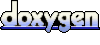