
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Headless.h
00001 #ifndef HEADLESS_H 00002 #define HEADLESS_H 00003 #include "Entity.h" 00004 00005 /**Headless Class 00006 @author Steven Mahasin 00007 @brief Creates a Headless which inherits the Entity class, this is one of the mobs that spawns in the normal rooms. 00008 @date May 2019 00009 */ 00010 class Headless : public Entity 00011 { 00012 00013 public: 00014 /** Constructor 00015 * @brief creates a headless at positions pos_x and pos_y 00016 * @param pos_x @details initialise _position.x 00017 * @param pos_y @details initialise _position.y 00018 */ 00019 Headless(float pos_x, float pos_y); 00020 00021 // Functions 00022 /** 00023 * @brief function moves the headless towards the player 00024 * @param x_value @details player x-position 00025 * @param y_value @details player y-position 00026 * @param map @details the 2d map array that dictates where there are walls or empty space 00027 * @param doorways @details an array that dictates which side of the wall has a doorway 00028 */ 00029 virtual void move(float player_x, float player_y, char * map, bool * doorways); 00030 /** 00031 * @brief reduce _hp by damage 00032 * @param damage @details the amount of damage to be taken 00033 */ 00034 virtual void take_damage(int damage); 00035 /** 00036 * @brief a virtual function of drawing the headless onto the screen 00037 * @param lcd @details the screen where the headless is drawn on 00038 */ 00039 virtual void draw(N5110 &lcd); 00040 00041 private: 00042 // Methods 00043 /** 00044 * @brief gets the sprite array 00045 * @return char pointer array of the corresponding snake sprite frame 00046 */ 00047 char * get_frame(); 00048 /** 00049 * @brief increase _frame.count which increases _frame.number to animate snake 00050 */ 00051 void increment_frame(); 00052 }; 00053 00054 const char sprite_headless[4][4][9][6] = { // Player [Face][SpriteAnimationFrame][Size_Y][Size_X] 00055 { 00056 // Up 00057 { 00058 {0,0,0,0,0,0,}, 00059 {1,0,0,0,0,0,}, 00060 {0,1,1,1,1,0,}, 00061 {1,0,1,1,0,1,}, 00062 {0,0,1,1,0,0,}, 00063 {0,0,1,1,0,0,}, 00064 {0,1,0,0,1,0,}, 00065 {0,1,0,0,1,0,}, 00066 {0,1,0,0,1,0,} 00067 }, 00068 { 00069 {0,0,0,0,0,0,}, 00070 {0,0,0,1,0,0,}, 00071 {0,1,1,1,1,0,}, 00072 {1,0,1,1,0,1,}, 00073 {0,0,1,1,0,0,}, 00074 {0,0,1,1,0,0,}, 00075 {0,1,0,0,1,0,}, 00076 {0,1,0,0,1,0,}, 00077 {0,0,0,0,1,0,} 00078 }, 00079 { 00080 {0,0,0,0,1,0,}, 00081 {0,0,1,0,0,0,}, 00082 {0,1,1,1,1,0,}, 00083 {1,0,1,1,0,1,}, 00084 {0,0,1,1,0,0,}, 00085 {0,0,1,1,0,0,}, 00086 {0,1,0,0,1,0,}, 00087 {0,1,0,0,1,0,}, 00088 {0,1,0,0,1,0,} 00089 }, 00090 { 00091 {0,1,0,0,0,0,}, 00092 {0,0,0,0,0,0,}, 00093 {0,1,1,1,1,0,}, 00094 {1,0,1,1,0,1,}, 00095 {0,0,1,1,0,0,}, 00096 {0,0,1,1,0,0,}, 00097 {0,1,0,0,1,0,}, 00098 {0,1,0,0,1,0,}, 00099 {0,1,0,0,0,0,} 00100 } 00101 }, 00102 { 00103 // Right 00104 { 00105 {0,0,0,0,0,0,}, 00106 {0,0,1,0,0,0,}, 00107 {0,0,1,1,0,0,}, 00108 {0,0,1,1,1,1,}, 00109 {0,0,1,1,0,0,}, 00110 {0,0,1,1,0,0,}, 00111 {0,0,1,0,0,0,}, 00112 {0,0,1,0,0,0,}, 00113 {0,0,1,1,0,0,} 00114 }, 00115 { 00116 {0,1,0,0,0,0,}, 00117 {0,0,0,1,0,0,}, 00118 {0,0,1,1,0,0,}, 00119 {0,0,1,1,1,1,}, 00120 {0,0,1,1,0,0,}, 00121 {0,0,1,1,0,0,}, 00122 {0,0,1,0,1,0,}, 00123 {0,0,1,0,1,0,}, 00124 {0,1,0,0,0,1,} 00125 }, 00126 { 00127 {0,0,0,0,1,0,}, 00128 {0,0,0,0,0,0,}, 00129 {0,0,1,1,0,0,}, 00130 {0,0,1,1,1,1,}, 00131 {0,0,1,1,0,0,}, 00132 {0,0,1,1,0,0,}, 00133 {0,0,1,0,0,0,}, 00134 {0,0,1,0,0,0,}, 00135 {0,0,1,1,0,0,} 00136 }, 00137 { 00138 {0,0,0,0,0,0,}, 00139 {0,0,0,0,0,1,}, 00140 {0,0,1,1,0,0,}, 00141 {0,0,1,1,1,1,}, 00142 {0,0,1,1,0,0,}, 00143 {0,0,1,1,0,0,}, 00144 {0,0,1,0,1,0,}, 00145 {0,0,1,0,1,0,}, 00146 {0,1,0,0,0,1,} 00147 } 00148 }, 00149 { 00150 // Down 00151 { 00152 {0,0,0,0,0,0,}, 00153 {1,0,0,0,0,0,}, 00154 {0,1,1,1,1,0,}, 00155 {1,0,1,1,0,1,}, 00156 {0,0,1,1,0,0,}, 00157 {0,0,1,1,0,0,}, 00158 {0,1,0,0,1,0,}, 00159 {0,1,0,0,1,0,}, 00160 {0,1,0,0,1,0,} 00161 }, 00162 { 00163 {0,0,0,0,0,0,}, 00164 {0,0,0,1,0,0,}, 00165 {0,1,1,1,1,0,}, 00166 {1,0,1,1,0,1,}, 00167 {0,0,1,1,0,0,}, 00168 {0,0,1,1,0,0,}, 00169 {0,1,0,0,1,0,}, 00170 {0,1,0,0,1,0,}, 00171 {0,0,0,0,1,0,} 00172 }, 00173 { 00174 {0,0,0,0,1,0,}, 00175 {0,0,1,0,0,0,}, 00176 {0,1,1,1,1,0,}, 00177 {1,0,1,1,0,1,}, 00178 {0,0,1,1,0,0,}, 00179 {0,0,1,1,0,0,}, 00180 {0,1,0,0,1,0,}, 00181 {0,1,0,0,1,0,}, 00182 {0,1,0,0,1,0,} 00183 }, 00184 { 00185 {0,1,0,0,0,0,}, 00186 {0,0,0,0,0,0,}, 00187 {0,1,1,1,1,0,}, 00188 {1,0,1,1,0,1,}, 00189 {0,0,1,1,0,0,}, 00190 {0,0,1,1,0,0,}, 00191 {0,1,0,0,1,0,}, 00192 {0,1,0,0,1,0,}, 00193 {0,1,0,0,0,0,} 00194 } 00195 }, 00196 { 00197 // Left 00198 { 00199 {0,0,0,0,0,0,}, 00200 {0,0,0,1,0,0,}, 00201 {0,0,1,1,0,0,}, 00202 {1,1,1,1,0,0,}, 00203 {0,0,1,1,0,0,}, 00204 {0,0,1,1,0,0,}, 00205 {0,0,0,1,0,0,}, 00206 {0,0,0,1,0,0,}, 00207 {0,0,1,1,0,0,} 00208 }, 00209 { 00210 {0,0,0,0,1,0,}, 00211 {0,0,1,0,0,0,}, 00212 {0,0,1,1,0,0,}, 00213 {1,1,1,1,0,0,}, 00214 {0,0,1,1,0,0,}, 00215 {0,0,1,1,0,0,}, 00216 {0,1,0,1,0,0,}, 00217 {0,1,0,1,0,0,}, 00218 {1,0,0,0,1,0,} 00219 }, 00220 { 00221 {0,1,0,0,0,0,}, 00222 {0,0,0,0,0,0,}, 00223 {0,0,1,1,0,0,}, 00224 {1,1,1,1,0,0,}, 00225 {0,0,1,1,0,0,}, 00226 {0,0,1,1,0,0,}, 00227 {0,0,0,1,0,0,}, 00228 {0,0,0,1,0,0,}, 00229 {0,0,1,1,0,0,} 00230 }, 00231 { 00232 {0,0,0,0,0,0,}, 00233 {1,0,0,0,0,0,}, 00234 {0,0,1,1,0,0,}, 00235 {1,1,1,1,0,0,}, 00236 {0,0,1,1,0,0,}, 00237 {0,0,1,1,0,0,}, 00238 {0,1,0,1,0,0,}, 00239 {0,1,0,1,0,0,}, 00240 {1,0,0,0,1,0,} 00241 } 00242 } 00243 }; 00244 00245 #endif
Generated on Tue Jul 19 2022 23:32:07 by
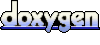