
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Bullets.h
00001 #ifndef BULLETS_H 00002 #define BULLETS_H 00003 #include "Entity.h" 00004 00005 /**Bullets Class 00006 @author Steven Mahasin 00007 @brief Creates a Bullet which inherits the Entity class, this will be a created projectile by the player class 00008 @date May 2019 00009 */ 00010 class Bullets : public Entity 00011 { 00012 00013 public: 00014 /** Constructor 00015 * @brief creates a bullet at positions pos_x and pos_y travelling at face dir 00016 * @param pos_x @details initialise _position.x 00017 * @param pos_y @details initialise _position.y 00018 * @param dir @details initialise _face 00019 */ 00020 Bullets(float pos_x, float pos_y, int dir); 00021 00022 // Functions 00023 /** 00024 * @brief function moves the bullet on it's face at a speed 00025 * @param speed @details the speed of the bullet 00026 * @param unused @details not used 00027 * @param map @details not used 00028 * @param doorways @details not used 00029 */ 00030 virtual void move(float speed, float unused, char * map, bool * doorways); 00031 /** 00032 * @brief draws the bullet onto the screen 00033 * @param lcd @details the screen where the bullet is drawn 00034 */ 00035 virtual void draw(N5110 &lcd); 00036 /** 00037 * @brief reduce _hp by damage 00038 * @param damage @details the amount of damage to be taken 00039 */ 00040 virtual void take_damage(int damage); 00041 /** 00042 * @brief checks if the bullet is out of bounds (hit wall or out of screen) 00043 * @param map @details the 2d map array that dictates where there are walls or empty space 00044 * @param doorways @details an array that dictates which side of the wall has a doorway 00045 * @returns true if bullet is out of bounds 00046 */ 00047 bool out_of_bounds_check(char * map, bool * doorways); 00048 }; 00049 00050 const char bullets_sprite[3][3] = {{1,1,1}, 00051 {1,1,1}, 00052 {1,1,1} 00053 }; 00054 00055 #endif
Generated on Tue Jul 19 2022 23:32:07 by
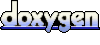