Class used to interface with the Nokia N5110 LCD.
Fork of N5110 by
N5110.cpp
00001 #include "mbed.h" 00002 #include "N5110.h" 00003 00004 // overloaded constructor includes power pin - LCD Vcc connected to GPIO pin 00005 // this constructor works fine with LPC1768 - enough current sourced from GPIO 00006 // to power LCD. Doesn't work well with K64F. 00007 N5110::N5110(PinName const pwrPin, 00008 PinName const scePin, 00009 PinName const rstPin, 00010 PinName const dcPin, 00011 PinName const mosiPin, 00012 PinName const sclkPin, 00013 PinName const ledPin) 00014 : 00015 _spi(new SPI(mosiPin,NC,sclkPin)), // create new SPI instance and initialise 00016 _led(new PwmOut(ledPin)), 00017 _pwr(new DigitalOut(pwrPin)), 00018 _sce(new DigitalOut(scePin)), 00019 _rst(new DigitalOut(rstPin)), 00020 _dc(new DigitalOut(dcPin)) 00021 {} 00022 00023 // overloaded constructor does not include power pin - LCD Vcc must be tied to +3V3 00024 // Best to use this with K64F as the GPIO hasn't sufficient output current to reliably 00025 // drive the LCD. 00026 N5110::N5110(PinName const scePin, 00027 PinName const rstPin, 00028 PinName const dcPin, 00029 PinName const mosiPin, 00030 PinName const sclkPin, 00031 PinName const ledPin) 00032 : 00033 _spi(new SPI(mosiPin,NC,sclkPin)), // create new SPI instance and initialise 00034 _led(new PwmOut(ledPin)), 00035 _pwr(NULL), // pwr not needed so null it to be safe 00036 _sce(new DigitalOut(scePin)), 00037 _rst(new DigitalOut(rstPin)), 00038 _dc(new DigitalOut(dcPin)) 00039 {} 00040 00041 N5110::~N5110() 00042 { 00043 delete _spi; 00044 00045 if(_pwr) { 00046 delete _pwr; 00047 } 00048 00049 delete _led; 00050 delete _sce; 00051 delete _rst; 00052 delete _dc; 00053 } 00054 00055 // initialise function - powers up and sends the initialisation commands 00056 void N5110::init() 00057 { 00058 turnOn(); // power up 00059 reset(); // reset LCD - must be done within 100 ms 00060 00061 initSPI(); 00062 // function set - extended 00063 sendCommand(0x20 | CMD_FS_ACTIVE_MODE | CMD_FS_HORIZONTAL_MODE | CMD_FS_EXTENDED_MODE); 00064 // Don't completely understand these parameters - they seem to work as they are 00065 // Consult the datasheet if you need to change them 00066 sendCommand(CMD_VOP_7V38); // operating voltage - these values are from Chris Yan's Library 00067 sendCommand(CMD_TC_TEMP_2); // temperature control 00068 sendCommand(CMD_BI_MUX_48); // changing this can sometimes improve the contrast on some displays 00069 00070 // function set - basic 00071 sendCommand(0x20 | CMD_FS_ACTIVE_MODE | CMD_FS_HORIZONTAL_MODE | CMD_FS_BASIC_MODE); 00072 normalMode(); // normal video mode by default 00073 sendCommand(CMD_DC_NORMAL_MODE); // black on white 00074 00075 clearRAM(); // RAM is undefined at power-up so clear 00076 clear(); // clear buffer 00077 setBrightness(0.5); 00078 } 00079 00080 // sets normal video mode (black on white) 00081 void N5110::normalMode() 00082 { 00083 sendCommand(CMD_DC_NORMAL_MODE); 00084 } 00085 00086 // sets normal video mode (white on black) 00087 void N5110::inverseMode() 00088 { 00089 sendCommand(CMD_DC_INVERT_VIDEO); 00090 } 00091 00092 // function to power up the LCD and backlight - only works when using GPIO to power 00093 void N5110::turnOn() 00094 { 00095 if (_pwr != NULL) { 00096 _pwr->write(1); // apply power 00097 } 00098 } 00099 00100 // function to power down LCD 00101 void N5110::turnOff() 00102 { 00103 clear(); // clear buffer 00104 refresh(); 00105 setBrightness(0.0); // turn backlight off 00106 clearRAM(); // clear RAM to ensure specified current consumption 00107 // send command to ensure we are in basic mode 00108 sendCommand(0x20 | CMD_FS_ACTIVE_MODE | CMD_FS_HORIZONTAL_MODE | CMD_FS_BASIC_MODE); 00109 // clear the display 00110 sendCommand(CMD_DC_CLEAR_DISPLAY); 00111 // enter the extended mode and power down 00112 sendCommand(0x20 | CMD_FS_POWER_DOWN_MODE | CMD_FS_HORIZONTAL_MODE | CMD_FS_EXTENDED_MODE); 00113 // small delay and then turn off the power pin 00114 wait_ms(10); 00115 00116 // if we are powering the LCD using the GPIO then make it low to turn off 00117 if (_pwr != NULL) { 00118 _pwr->write(0); // turn off power 00119 } 00120 00121 } 00122 00123 // function to change LED backlight brightness 00124 void N5110::setBrightness(float brightness) 00125 { 00126 // check whether brightness is within range 00127 if (brightness < 0.0f) 00128 brightness = 0.0f; 00129 if (brightness > 1.0f) 00130 brightness = 1.0f; 00131 // set PWM duty cycle 00132 _led->write(brightness); 00133 } 00134 00135 00136 // pulse the active low reset line 00137 void N5110::reset() 00138 { 00139 _rst->write(0); // reset the LCD 00140 _rst->write(1); 00141 } 00142 00143 // function to initialise SPI peripheral 00144 void N5110::initSPI() 00145 { 00146 _spi->format(8,1); // 8 bits, Mode 1 - polarity 0, phase 1 - base value of clock is 0, data captured on falling edge/propagated on rising edge 00147 _spi->frequency(4000000); // maximum of screen is 4 MHz 00148 } 00149 00150 // send a command to the display 00151 void N5110::sendCommand(unsigned char command) 00152 { 00153 _dc->write(0); // set DC low for command 00154 _sce->write(0); // set CE low to begin frame 00155 _spi->write(command); // send command 00156 _dc->write(1); // turn back to data by default 00157 _sce->write(1); // set CE high to end frame (expected for transmission of single byte) 00158 } 00159 00160 // send data to the display at the current XY address 00161 // dc is set to 1 (i.e. data) after sending a command and so should 00162 // be the default mode. 00163 void N5110::sendData(unsigned char data) 00164 { 00165 _sce->write(0); // set CE low to begin frame 00166 _spi->write(data); 00167 _sce->write(1); // set CE high to end frame (expected for transmission of single byte) 00168 } 00169 00170 // this function writes 0 to the 504 bytes to clear the RAM 00171 void N5110::clearRAM() 00172 { 00173 _sce->write(0); //set CE low to begin frame 00174 for(int i = 0; i < WIDTH * HEIGHT; i++) { // 48 x 84 bits = 504 bytes 00175 _spi->write(0x00); // send 0's 00176 } 00177 _sce->write(1); // set CE high to end frame 00178 } 00179 00180 // function to set the XY address in RAM for subsequenct data write 00181 void N5110::setXYAddress(unsigned int const x, 00182 unsigned int const y) 00183 { 00184 if (x<WIDTH && y<HEIGHT) { // check within range 00185 sendCommand(0x80 | x); // send addresses to display with relevant mask 00186 sendCommand(0x40 | y); 00187 } 00188 } 00189 00190 // These functions are used to set, clear and get the value of pixels in the display 00191 // Pixels are addressed in the range of 0 to 47 (y) and 0 to 83 (x). The refresh() 00192 // function must be called after set and clear in order to update the display 00193 void N5110::setPixel(unsigned int const x, 00194 unsigned int const y, 00195 bool const state) 00196 { 00197 if (x<WIDTH && y<HEIGHT) { // check within range 00198 // calculate bank and shift 1 to required position in the data byte 00199 if(state) buffer[x][y/8] |= (1 << y%8); 00200 else buffer[x][y/8] &= ~(1 << y%8); 00201 } 00202 } 00203 00204 void N5110::clearPixel(unsigned int const x, 00205 unsigned int const y) 00206 { 00207 if (x<WIDTH && y<HEIGHT) { // check within range 00208 // calculate bank and shift 1 to required position (using bit clear) 00209 buffer[x][y/8] &= ~(1 << y%8); 00210 } 00211 } 00212 00213 int N5110::getPixel(unsigned int const x, 00214 unsigned int const y) const 00215 { 00216 if (x<WIDTH && y<HEIGHT) { // check within range 00217 // return relevant bank and mask required bit 00218 00219 int pixel = (int) buffer[x][y/8] & (1 << y%8); 00220 00221 if (pixel) 00222 return 1; 00223 else 00224 return 0; 00225 } 00226 00227 return 0; 00228 00229 } 00230 00231 // function to refresh the display 00232 void N5110::refresh() 00233 { 00234 setXYAddress(0,0); // important to set address back to 0,0 before refreshing display 00235 // address auto increments after printing string, so buffer[0][0] will not coincide 00236 // with top-left pixel after priting string 00237 00238 _sce->write(0); //set CE low to begin frame 00239 00240 for(int j = 0; j < BANKS; j++) { // be careful to use correct order (j,i) for horizontal addressing 00241 for(int i = 0; i < WIDTH; i++) { 00242 _spi->write(buffer[i][j]); // send buffer 00243 } 00244 } 00245 _sce->write(1); // set CE high to end frame 00246 00247 } 00248 00249 // fills the buffer with random bytes. Can be used to test the display. 00250 // The rand() function isn't seeded so it probably creates the same pattern everytime 00251 void N5110::randomiseBuffer() 00252 { 00253 int i,j; 00254 for(j = 0; j < BANKS; j++) { // be careful to use correct order (j,i) for horizontal addressing 00255 for(i = 0; i < WIDTH; i++) { 00256 buffer[i][j] = rand()%256; // generate random byte 00257 } 00258 } 00259 00260 } 00261 00262 // function to print 5x7 font 00263 void N5110::printChar(char const c, 00264 unsigned int const x, 00265 unsigned int const y) 00266 { 00267 if (y<BANKS) { // check if printing in range of y banks 00268 00269 for (int i = 0; i < 5 ; i++ ) { 00270 int pixel_x = x+i; 00271 if (pixel_x > WIDTH-1) // ensure pixel isn't outside the buffer size (0 - 83) 00272 break; 00273 buffer[pixel_x][y] = font5x7[(c - 32)*5 + i]; 00274 // array is offset by 32 relative to ASCII, each character is 5 pixels wide 00275 } 00276 00277 } 00278 } 00279 00280 // function to print string at specified position 00281 void N5110::printString(const char *str, 00282 unsigned int const x, 00283 unsigned int const y) 00284 { 00285 if (y<BANKS) { // check if printing in range of y banks 00286 00287 int n = 0 ; // counter for number of characters in string 00288 // loop through string and print character 00289 while(*str) { 00290 00291 // writes the character bitmap data to the buffer, so that 00292 // text and pixels can be displayed at the same time 00293 for (int i = 0; i < 5 ; i++ ) { 00294 int pixel_x = x+i+n*6; 00295 if (pixel_x > WIDTH-1) // ensure pixel isn't outside the buffer size (0 - 83) 00296 break; 00297 buffer[pixel_x][y] = font5x7[(*str - 32)*5 + i]; 00298 } 00299 str++; // go to next character in string 00300 n++; // increment index 00301 } 00302 } 00303 } 00304 00305 // function to clear the screen buffer 00306 void N5110::clear() 00307 { 00308 memset(buffer,0,sizeof(buffer)); 00309 } 00310 00311 // function to plot array on display 00312 void N5110::plotArray(float const array[]) 00313 { 00314 for (int i=0; i<WIDTH; i++) { // loop through array 00315 // elements are normalised from 0.0 to 1.0, so multiply 00316 // by 47 to convert to pixel range, and subtract from 47 00317 // since top-left is 0,0 in the display geometry 00318 setPixel(i,47 - int(array[i]*47.0f)); 00319 } 00320 00321 } 00322 00323 // function to draw circle 00324 void N5110:: drawCircle(unsigned int const x0, 00325 unsigned int const y0, 00326 unsigned int const radius, 00327 FillType const fill) 00328 { 00329 // from http://en.wikipedia.org/wiki/Midpoint_circle_algorithm 00330 int x = radius; 00331 int y = 0; 00332 int radiusError = 1-x; 00333 00334 while(x >= y) { 00335 00336 // if transparent, just draw outline 00337 if (fill == FILL_TRANSPARENT) { 00338 setPixel( x + x0, y + y0); 00339 setPixel(-x + x0, y + y0); 00340 setPixel( y + x0, x + y0); 00341 setPixel(-y + x0, x + y0); 00342 setPixel(-y + x0, -x + y0); 00343 setPixel( y + x0, -x + y0); 00344 setPixel( x + x0, -y + y0); 00345 setPixel(-x + x0, -y + y0); 00346 } else { // drawing filled circle, so draw lines between points at same y value 00347 00348 int type = (fill==FILL_BLACK) ? 1:0; // black or white fill 00349 00350 drawLine(x+x0,y+y0,-x+x0,y+y0,type); 00351 drawLine(y+x0,x+y0,-y+x0,x+y0,type); 00352 drawLine(y+x0,-x+y0,-y+x0,-x+y0,type); 00353 drawLine(x+x0,-y+y0,-x+x0,-y+y0,type); 00354 } 00355 00356 y++; 00357 if (radiusError<0) { 00358 radiusError += 2 * y + 1; 00359 } else { 00360 x--; 00361 radiusError += 2 * (y - x) + 1; 00362 } 00363 } 00364 00365 } 00366 00367 void N5110::drawLine(unsigned int const x0, 00368 unsigned int const y0, 00369 unsigned int const x1, 00370 unsigned int const y1, 00371 unsigned int const type) 00372 { 00373 // Note that the ranges can be negative so we have to turn the input values 00374 // into signed integers first 00375 int const y_range = static_cast<int>(y1) - static_cast<int>(y0); 00376 int const x_range = static_cast<int>(x1) - static_cast<int>(x0); 00377 00378 // if dotted line, set step to 2, else step is 1 00379 unsigned int const step = (type==2) ? 2:1; 00380 00381 // make sure we loop over the largest range to get the most pixels on the display 00382 // for instance, if drawing a vertical line (x_range = 0), we need to loop down the y pixels 00383 // or else we'll only end up with 1 pixel in the x column 00384 if ( abs(x_range) > abs(y_range) ) { 00385 00386 // ensure we loop from smallest to largest or else for-loop won't run as expected 00387 unsigned int const start = x_range > 0 ? x0:x1; 00388 unsigned int const stop = x_range > 0 ? x1:x0; 00389 00390 // loop between x pixels 00391 for (unsigned int x = start; x<= stop ; x+=step) { 00392 // do linear interpolation 00393 int const dx = static_cast<int>(x)-static_cast<int>(x0); 00394 unsigned int const y = y0 + y_range * dx / x_range; 00395 00396 // If the line type is '0', this will clear the pixel 00397 // If it is '1' or '2', the pixel will be set 00398 setPixel(x,y, type); 00399 } 00400 } else { 00401 00402 // ensure we loop from smallest to largest or else for-loop won't run as expected 00403 unsigned int const start = y_range > 0 ? y0:y1; 00404 unsigned int const stop = y_range > 0 ? y1:y0; 00405 00406 for (unsigned int y = start; y<= stop ; y+=step) { 00407 // do linear interpolation 00408 int const dy = static_cast<int>(y)-static_cast<int>(y0); 00409 unsigned int const x = x0 + x_range * dy / y_range; 00410 00411 // If the line type is '0', this will clear the pixel 00412 // If it is '1' or '2', the pixel will be set 00413 setPixel(x,y, type); 00414 } 00415 } 00416 00417 } 00418 00419 void N5110::drawRect(unsigned int const x0, 00420 unsigned int const y0, 00421 unsigned int const width, 00422 unsigned int const height, 00423 FillType const fill) 00424 { 00425 if (fill == FILL_TRANSPARENT) { // transparent, just outline 00426 drawLine(x0,y0,x0+(width-1),y0,1); // top 00427 drawLine(x0,y0+(height-1),x0+(width-1),y0+(height-1),1); // bottom 00428 drawLine(x0,y0,x0,y0+(height-1),1); // left 00429 drawLine(x0+(width-1),y0,x0+(width-1),y0+(height-1),1); // right 00430 } else { // filled rectangle 00431 int type = (fill==FILL_BLACK) ? 1:0; // black or white fill 00432 for (int y = y0; y<y0+height; y++) { // loop through rows of rectangle 00433 drawLine(x0,y,x0+(width-1),y,type); // draw line across screen 00434 } 00435 } 00436 } 00437 00438 void N5110::drawSprite(int x0, 00439 int y0, 00440 int nrows, 00441 int ncols, 00442 int *sprite) 00443 { 00444 for (int i = 0; i < nrows; i++) { 00445 for (int j = 0 ; j < ncols ; j++) { 00446 00447 int pixel = *((sprite+i*ncols)+j); 00448 setPixel(x0+j,y0+i, pixel); 00449 } 00450 } 00451 }
Generated on Thu Jul 14 2022 02:23:06 by
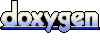